Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / ValidationResult.cs / 1 / ValidationResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // A ValidationResult is the result of call to ValidationRule.Validate // // See specs at http://avalon/connecteddata/M5%20Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Controls { ////// A ValidationResult is the result of call to ValidationRule.Validate /// public class ValidationResult { ////// Constructor /// public ValidationResult(bool isValid, object errorContent) { _isValid = isValid; _errorContent = errorContent; } ////// Whether or not the ValidationRule that was checked is valid. /// public bool IsValid { get { return _isValid; } } ////// Additional information regarding the cause of the invalid /// state of the binding that was just checked. /// public object ErrorContent { get { return _errorContent; } } ////// Returns a valid ValidationResult /// public static ValidationResult ValidResult { get { return s_valid; } } ////// Compares the parameters for value equality /// /// left operand /// right operand ///true if the values are equal public static bool operator == (ValidationResult left, ValidationResult right) { return Object.Equals(left, right); } ////// Compares the parameters for value inequality /// /// left operand /// right operand ///true if the values are not equal public static bool operator != (ValidationResult left, ValidationResult right) { return !Object.Equals(left, right); } ////// By-value comparison of ValidationResult /// ////// This method is also used indirectly from the operator overrides. /// /// ValidationResult to be compared against this ValidationRule ///true if obj is ValidationResult and has the same values public override bool Equals(object obj) { // A cheaper alternative to Object.ReferenceEquals() is used here for better perf if (obj == (object)this) { return true; } else { ValidationResult vr = obj as ValidationResult; if (vr != null) { return (IsValid == vr.IsValid) && (ErrorContent == vr.ErrorContent); } } return false; } ////// Hash function for ValidationResult /// ///hash code for the current ValidationResult public override int GetHashCode() { return IsValid.GetHashCode() ^ ((ErrorContent == null) ? int.MinValue : ErrorContent).GetHashCode(); } private bool _isValid; private object _errorContent; private static readonly ValidationResult s_valid = new ValidationResult(true, null); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // A ValidationResult is the result of call to ValidationRule.Validate // // See specs at http://avalon/connecteddata/M5%20Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Controls { ////// A ValidationResult is the result of call to ValidationRule.Validate /// public class ValidationResult { ////// Constructor /// public ValidationResult(bool isValid, object errorContent) { _isValid = isValid; _errorContent = errorContent; } ////// Whether or not the ValidationRule that was checked is valid. /// public bool IsValid { get { return _isValid; } } ////// Additional information regarding the cause of the invalid /// state of the binding that was just checked. /// public object ErrorContent { get { return _errorContent; } } ////// Returns a valid ValidationResult /// public static ValidationResult ValidResult { get { return s_valid; } } ////// Compares the parameters for value equality /// /// left operand /// right operand ///true if the values are equal public static bool operator == (ValidationResult left, ValidationResult right) { return Object.Equals(left, right); } ////// Compares the parameters for value inequality /// /// left operand /// right operand ///true if the values are not equal public static bool operator != (ValidationResult left, ValidationResult right) { return !Object.Equals(left, right); } ////// By-value comparison of ValidationResult /// ////// This method is also used indirectly from the operator overrides. /// /// ValidationResult to be compared against this ValidationRule ///true if obj is ValidationResult and has the same values public override bool Equals(object obj) { // A cheaper alternative to Object.ReferenceEquals() is used here for better perf if (obj == (object)this) { return true; } else { ValidationResult vr = obj as ValidationResult; if (vr != null) { return (IsValid == vr.IsValid) && (ErrorContent == vr.ErrorContent); } } return false; } ////// Hash function for ValidationResult /// ///hash code for the current ValidationResult public override int GetHashCode() { return IsValid.GetHashCode() ^ ((ErrorContent == null) ? int.MinValue : ErrorContent).GetHashCode(); } private bool _isValid; private object _errorContent; private static readonly ValidationResult s_valid = new ValidationResult(true, null); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
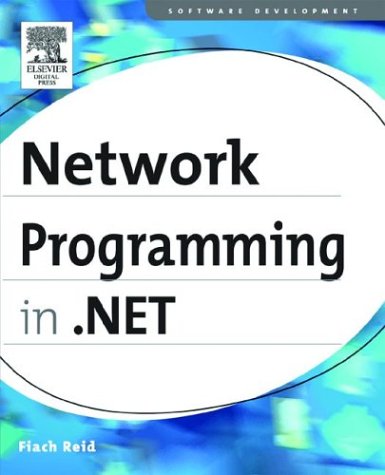
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilLoop.cs
- TextTrailingCharacterEllipsis.cs
- keycontainerpermission.cs
- ViewgenGatekeeper.cs
- MtomMessageEncodingBindingElement.cs
- PlatformNotSupportedException.cs
- _TLSstream.cs
- InvalidCommandTreeException.cs
- ClientSettingsProvider.cs
- FixedTextView.cs
- ListManagerBindingsCollection.cs
- CurrencyWrapper.cs
- SoapHttpTransportImporter.cs
- WindowsGraphicsCacheManager.cs
- TextEditorMouse.cs
- ButtonField.cs
- TimeSpanOrInfiniteConverter.cs
- DataTable.cs
- NamespaceMapping.cs
- TextServicesHost.cs
- DoubleConverter.cs
- LongValidatorAttribute.cs
- RoutingSection.cs
- XMLDiffLoader.cs
- StateItem.cs
- ListenerHandler.cs
- BasicHttpMessageSecurityElement.cs
- CanonicalXml.cs
- TextFindEngine.cs
- SerializationObjectManager.cs
- CaseStatement.cs
- EpmContentSerializerBase.cs
- Configuration.cs
- InlineObject.cs
- TreeBuilder.cs
- SupportsEventValidationAttribute.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- CodeRemoveEventStatement.cs
- IpcServerChannel.cs
- WindowsNonControl.cs
- AsyncOperationManager.cs
- FileLevelControlBuilderAttribute.cs
- ServiceAppDomainAssociationProvider.cs
- CodeConstructor.cs
- _LocalDataStore.cs
- TextParagraphCache.cs
- LayoutTableCell.cs
- TextTreeUndoUnit.cs
- AsymmetricSignatureFormatter.cs
- HandleExceptionArgs.cs
- BooleanToVisibilityConverter.cs
- TextTreeUndoUnit.cs
- RuntimeConfigLKG.cs
- CodeNamespace.cs
- XmlMemberMapping.cs
- RawAppCommandInputReport.cs
- EncoderReplacementFallback.cs
- LinearQuaternionKeyFrame.cs
- COM2ComponentEditor.cs
- CodeBlockBuilder.cs
- Canvas.cs
- PathStreamGeometryContext.cs
- DecimalStorage.cs
- ReflectPropertyDescriptor.cs
- UnauthorizedAccessException.cs
- FormsAuthentication.cs
- RegexWorker.cs
- WindowsToolbarItemAsMenuItem.cs
- Visitor.cs
- ValuePatternIdentifiers.cs
- ProfileModule.cs
- ButtonPopupAdapter.cs
- ListItemCollection.cs
- SystemColors.cs
- CreateUserErrorEventArgs.cs
- DataSetUtil.cs
- ZoneMembershipCondition.cs
- WizardPanel.cs
- DataListItemEventArgs.cs
- HttpProfileGroupBase.cs
- Hashtable.cs
- ObjectReaderCompiler.cs
- FreeIndexList.cs
- ObjectDataSourceStatusEventArgs.cs
- DeliveryRequirementsAttribute.cs
- ToolStripContentPanel.cs
- _IPv4Address.cs
- WebPartTransformerCollection.cs
- ScrollItemPatternIdentifiers.cs
- CollectionViewGroupInternal.cs
- MessageQueueEnumerator.cs
- ToolStripPanelRenderEventArgs.cs
- MethodSet.cs
- ConfigurationSectionGroupCollection.cs
- AsyncDataRequest.cs
- DesignerActionVerbList.cs
- AttachedPropertiesService.cs
- OwnerDrawPropertyBag.cs
- BitmapCodecInfo.cs
- PassportIdentity.cs