Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / RegexWorker.cs / 2 / RegexWorker.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class for browser capabilities object: just a read-only dictionary * holder that supports Init() * * */ using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web.RegularExpressions; using System.Web.Util; namespace System.Web.Configuration { // [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RegexWorker { internal static readonly Regex RefPat = new BrowserCapsRefRegex(); private Hashtable _groups; private HttpBrowserCapabilities _browserCaps; public RegexWorker(HttpBrowserCapabilities browserCaps) { _browserCaps = browserCaps; } private string Lookup(string from) { MatchCollection matches = RefPat.Matches(from); // shortcut for no reference case if (matches.Count == 0) { return from; } StringBuilder sb = new StringBuilder(); int startIndex = 0; foreach (Match match in matches) { int length = match.Index - startIndex; sb.Append(from.Substring(startIndex, length)); startIndex = match.Index + match.Length; string groupName = match.Groups["name"].Value; string result = null; if (_groups != null) { result = (String)_groups[groupName]; } if (result == null) { result = _browserCaps[groupName]; } sb.Append(result); } sb.Append(from, startIndex, from.Length - startIndex); string output = sb.ToString(); // Return null instead of empty string since empty string is used to override values. if (output.Length == 0) { return null; } return output; } public string this[string key] { get { return Lookup(key); } } public bool ProcessRegex(string target, string regexExpression) { if(target == null) { target = String.Empty; } Regex regex = new Regex(regexExpression, RegexOptions.ExplicitCapture); Match match = regex.Match(target); if(match.Success == false) { return false; } string[] groups = regex.GetGroupNames(); if (groups.Length > 0) { if (_groups == null) { _groups = new Hashtable(); } for (int i = 0; i < groups.Length; i++) { _groups[groups[i]] = match.Groups[i].Value; } } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
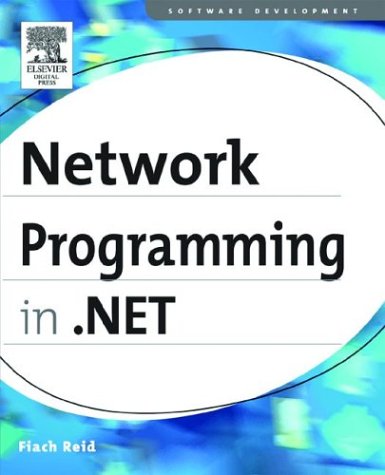
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryObjectInfo.cs
- IgnoreSectionHandler.cs
- DataPagerFieldCollection.cs
- ImageAttributes.cs
- SchemaNames.cs
- DispatcherOperation.cs
- CollectionViewGroupInternal.cs
- CompatibleIComparer.cs
- XslTransformFileEditor.cs
- WebBrowsableAttribute.cs
- StatusStrip.cs
- RIPEMD160.cs
- BasicExpressionVisitor.cs
- XmlSiteMapProvider.cs
- ToolboxService.cs
- LinqDataSourceContextEventArgs.cs
- SmiMetaData.cs
- Baml2006ReaderFrame.cs
- EntityDataSourceDataSelection.cs
- OrderedEnumerableRowCollection.cs
- ParallelTimeline.cs
- ReceiveCompletedEventArgs.cs
- XamlPointCollectionSerializer.cs
- _LazyAsyncResult.cs
- MergeFailedEvent.cs
- MdImport.cs
- HtmlTableCell.cs
- EventInfo.cs
- NumberSubstitution.cs
- XmlSchemaObjectTable.cs
- ThrowOnMultipleAssignment.cs
- MetadataArtifactLoaderResource.cs
- XsltArgumentList.cs
- PaperSource.cs
- SafeNativeMethodsOther.cs
- ConfigUtil.cs
- SelectedPathEditor.cs
- ServiceRoute.cs
- Win32Native.cs
- ToolStripMenuItemDesigner.cs
- BitmapEffectInput.cs
- ValueQuery.cs
- LogStore.cs
- ExpressionCopier.cs
- IDispatchConstantAttribute.cs
- SafeFileMappingHandle.cs
- ListViewEditEventArgs.cs
- DebugTrace.cs
- ConnectionsZone.cs
- ThemeDirectoryCompiler.cs
- RoutingBehavior.cs
- DictionaryGlobals.cs
- Track.cs
- OptimizedTemplateContentHelper.cs
- EdmComplexTypeAttribute.cs
- sqlinternaltransaction.cs
- EncryptedKeyIdentifierClause.cs
- CommandManager.cs
- BamlStream.cs
- ServiceDiscoveryElement.cs
- MulticastDelegate.cs
- MdiWindowListItemConverter.cs
- ExpressionVisitor.cs
- FontStretches.cs
- OdbcInfoMessageEvent.cs
- GridItem.cs
- LambdaSerializationException.cs
- ListViewDeleteEventArgs.cs
- GiveFeedbackEvent.cs
- OleDbMetaDataFactory.cs
- XmlSchema.cs
- TextTrailingWordEllipsis.cs
- ObjectStateEntryDbDataRecord.cs
- FamilyCollection.cs
- SelectedDatesCollection.cs
- DoubleLinkList.cs
- LicenseException.cs
- Matrix.cs
- UrlAuthorizationModule.cs
- IgnorePropertiesAttribute.cs
- CommandField.cs
- TextReturnReader.cs
- GetChildSubtree.cs
- WCFServiceClientProxyGenerator.cs
- TableAutomationPeer.cs
- WebPartManager.cs
- FullTrustAssemblyCollection.cs
- MethodImplAttribute.cs
- Overlapped.cs
- AutomationIdentifierGuids.cs
- DesignerOptions.cs
- XmlSchemaExternal.cs
- ArrayTypeMismatchException.cs
- FontDifferentiator.cs
- XPathScanner.cs
- ProcessProtocolHandler.cs
- TreeBuilderXamlTranslator.cs
- JapaneseCalendar.cs
- XmlSchemaAny.cs
- StateDesignerConnector.cs