Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / ColorConvertedBitmapExtension.cs / 1 / ColorConvertedBitmapExtension.cs
/****************************************************************************\ * * File: ColorConvertedBitmapExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.IO; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Reflection; using MS.Internal; namespace System.Windows { ////// Class for Xaml markup extension for ColorConvertedBitmap with non-embedded profile. /// [MarkupExtensionReturnType(typeof(ColorConvertedBitmap))] public class ColorConvertedBitmapExtension : MarkupExtension { ////// Constructor that takes no parameters /// public ColorConvertedBitmapExtension() { } ////// Constructor that takes the markup for a "{ColorConvertedBitmap image source.icc destination.icc}" /// public ColorConvertedBitmapExtension( object image) { if (image == null) { throw new ArgumentNullException("image"); } string[] tokens = ((string)image).Split(new char[] { ' ' }); foreach (string str in tokens) { if (str.Length > 0) { if (_image == null) { _image = str; } else if (_sourceProfile == null) { _sourceProfile = str; } else if (_destinationProfile == null) { _destinationProfile = str; } else { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionSyntax)); } } } } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For ColorConvertedBitmapExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_image == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceImage)); } if (_sourceProfile == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceProfile)); } // Save away the BaseUri. IUriContext uriContext = serviceProvider.GetService(typeof(IUriContext)) as IUriContext; if( uriContext == null ) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IUriContext" )); } _baseUri = uriContext.BaseUri; Uri imageUri = GetResolvedUri(_image); Uri sourceProfileUri = GetResolvedUri(_sourceProfile); Uri destinationProfileUri = GetResolvedUri(_destinationProfile); ColorContext sourceContext = new ColorContext(sourceProfileUri); ColorContext destinationContext = destinationProfileUri != null ? new ColorContext(destinationProfileUri) : new ColorContext(PixelFormats.Default); BitmapDecoder decoder = BitmapDecoder.Create( imageUri, BitmapCreateOptions.IgnoreColorProfile | BitmapCreateOptions.IgnoreImageCache, BitmapCacheOption.None ); BitmapSource bitmap = decoder.Frames[0]; FormatConvertedBitmap formatConverted = new FormatConvertedBitmap(bitmap, PixelFormats.Bgra32, null, 0.0); object result = formatConverted; try { ColorConvertedBitmap colorConverted = new ColorConvertedBitmap(formatConverted, sourceContext, destinationContext, PixelFormats.Bgra32); result= colorConverted; } catch (FileFormatException) { // Gracefully ignore non-matching profile // If the file contains a bad color context, we catch the exception here // since color transform isn't possible // with the given color context. } return result; } private Uri GetResolvedUri(string uri) { if (uri == null) { return null; } return new Uri(_baseUri,uri); } string _image; string _sourceProfile; Uri _baseUri; string _destinationProfile; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: ColorConvertedBitmapExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.IO; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Reflection; using MS.Internal; namespace System.Windows { ////// Class for Xaml markup extension for ColorConvertedBitmap with non-embedded profile. /// [MarkupExtensionReturnType(typeof(ColorConvertedBitmap))] public class ColorConvertedBitmapExtension : MarkupExtension { ////// Constructor that takes no parameters /// public ColorConvertedBitmapExtension() { } ////// Constructor that takes the markup for a "{ColorConvertedBitmap image source.icc destination.icc}" /// public ColorConvertedBitmapExtension( object image) { if (image == null) { throw new ArgumentNullException("image"); } string[] tokens = ((string)image).Split(new char[] { ' ' }); foreach (string str in tokens) { if (str.Length > 0) { if (_image == null) { _image = str; } else if (_sourceProfile == null) { _sourceProfile = str; } else if (_destinationProfile == null) { _destinationProfile = str; } else { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionSyntax)); } } } } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For ColorConvertedBitmapExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_image == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceImage)); } if (_sourceProfile == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceProfile)); } // Save away the BaseUri. IUriContext uriContext = serviceProvider.GetService(typeof(IUriContext)) as IUriContext; if( uriContext == null ) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IUriContext" )); } _baseUri = uriContext.BaseUri; Uri imageUri = GetResolvedUri(_image); Uri sourceProfileUri = GetResolvedUri(_sourceProfile); Uri destinationProfileUri = GetResolvedUri(_destinationProfile); ColorContext sourceContext = new ColorContext(sourceProfileUri); ColorContext destinationContext = destinationProfileUri != null ? new ColorContext(destinationProfileUri) : new ColorContext(PixelFormats.Default); BitmapDecoder decoder = BitmapDecoder.Create( imageUri, BitmapCreateOptions.IgnoreColorProfile | BitmapCreateOptions.IgnoreImageCache, BitmapCacheOption.None ); BitmapSource bitmap = decoder.Frames[0]; FormatConvertedBitmap formatConverted = new FormatConvertedBitmap(bitmap, PixelFormats.Bgra32, null, 0.0); object result = formatConverted; try { ColorConvertedBitmap colorConverted = new ColorConvertedBitmap(formatConverted, sourceContext, destinationContext, PixelFormats.Bgra32); result= colorConverted; } catch (FileFormatException) { // Gracefully ignore non-matching profile // If the file contains a bad color context, we catch the exception here // since color transform isn't possible // with the given color context. } return result; } private Uri GetResolvedUri(string uri) { if (uri == null) { return null; } return new Uri(_baseUri,uri); } string _image; string _sourceProfile; Uri _baseUri; string _destinationProfile; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
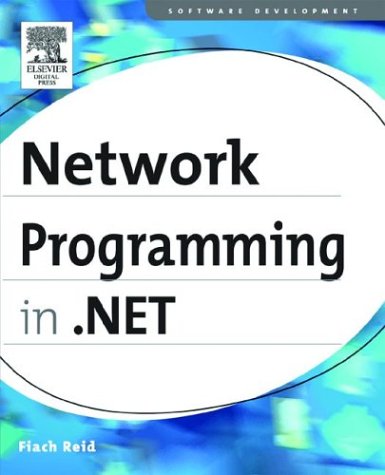
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexCompilationInfo.cs
- RelatedEnd.cs
- RijndaelManagedTransform.cs
- GorillaCodec.cs
- CodeNamespaceImport.cs
- _NativeSSPI.cs
- CodeAccessSecurityEngine.cs
- SafeArrayTypeMismatchException.cs
- NodeFunctions.cs
- DropDownList.cs
- AnchorEditor.cs
- CngProvider.cs
- AjaxFrameworkAssemblyAttribute.cs
- HttpCapabilitiesSectionHandler.cs
- DataServiceKeyAttribute.cs
- UnsafeNativeMethods.cs
- DrawingContextDrawingContextWalker.cs
- ImageSource.cs
- Brush.cs
- StatusBarPanel.cs
- WizardStepCollectionEditor.cs
- GridViewCellAutomationPeer.cs
- DefaultSection.cs
- FontUnit.cs
- ParserHooks.cs
- Main.cs
- TypeConverterHelper.cs
- SystemUnicastIPAddressInformation.cs
- QueryInterceptorAttribute.cs
- DataGridViewBindingCompleteEventArgs.cs
- DataGridRow.cs
- Set.cs
- HttpWriter.cs
- WebPartExportVerb.cs
- PrintPreviewGraphics.cs
- SiteMapNodeItemEventArgs.cs
- ToolStripSeparatorRenderEventArgs.cs
- GuidConverter.cs
- IgnoreFlushAndCloseStream.cs
- SizeAnimationClockResource.cs
- ChangeInterceptorAttribute.cs
- BasePropertyDescriptor.cs
- shaperfactoryquerycachekey.cs
- Message.cs
- SiteMapSection.cs
- Int32EqualityComparer.cs
- DBConnection.cs
- datacache.cs
- _BaseOverlappedAsyncResult.cs
- MouseCaptureWithinProperty.cs
- PowerModeChangedEventArgs.cs
- HostingEnvironmentException.cs
- Rect.cs
- RbTree.cs
- XmlWriterSettings.cs
- DataExchangeServiceBinder.cs
- WS2007HttpBindingElement.cs
- SetState.cs
- CorePropertiesFilter.cs
- Maps.cs
- LinkedResourceCollection.cs
- Predicate.cs
- VersionPair.cs
- wgx_commands.cs
- XmlObjectSerializerReadContextComplex.cs
- EditorZone.cs
- KeyedCollection.cs
- HtmlShim.cs
- ReferenceConverter.cs
- DataBindingList.cs
- CultureMapper.cs
- TemplatedMailWebEventProvider.cs
- NestedContainer.cs
- AutomationPropertyInfo.cs
- CallContext.cs
- ProxyFragment.cs
- TableRow.cs
- EntityDataSourceDataSelection.cs
- HttpListener.cs
- ItemContainerProviderWrapper.cs
- EventBuilder.cs
- PrintPreviewControl.cs
- TextComposition.cs
- XmlAutoDetectWriter.cs
- xmlglyphRunInfo.cs
- followingquery.cs
- TraceProvider.cs
- RSAPKCS1KeyExchangeFormatter.cs
- RectangleGeometry.cs
- ServiceDurableInstance.cs
- QueryOutputWriter.cs
- TextChange.cs
- MetabaseSettingsIis7.cs
- IPEndPoint.cs
- AuthenticationException.cs
- ListItemParagraph.cs
- String.cs
- SqlMethodAttribute.cs
- ImageMap.cs
- FixedDocument.cs