Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / Mail / MultiAsyncResult.cs / 1 / MultiAsyncResult.cs
namespace System.Net.Mime { using System; internal class MultiAsyncResult : LazyAsyncResult { int outstanding; object context; internal MultiAsyncResult(object context, AsyncCallback callback, object state) : base(context,state,callback) { this.context = context; } internal object Context { get { return this.context; } } internal void Enter() { Increment(); } internal void Leave() { Decrement(); } internal void Leave(object result) { this.Result = result; Decrement(); } void Decrement() { if (System.Threading.Interlocked.Decrement(ref this.outstanding) == -1) { base.InvokeCallback(Result); } } void Increment() { System.Threading.Interlocked.Increment(ref this.outstanding); } internal void CompleteSequence() { Decrement(); } internal static object End(IAsyncResult result) { MultiAsyncResult thisPtr = (MultiAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.Result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Net.Mime { using System; internal class MultiAsyncResult : LazyAsyncResult { int outstanding; object context; internal MultiAsyncResult(object context, AsyncCallback callback, object state) : base(context,state,callback) { this.context = context; } internal object Context { get { return this.context; } } internal void Enter() { Increment(); } internal void Leave() { Decrement(); } internal void Leave(object result) { this.Result = result; Decrement(); } void Decrement() { if (System.Threading.Interlocked.Decrement(ref this.outstanding) == -1) { base.InvokeCallback(Result); } } void Increment() { System.Threading.Interlocked.Increment(ref this.outstanding); } internal void CompleteSequence() { Decrement(); } internal static object End(IAsyncResult result) { MultiAsyncResult thisPtr = (MultiAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.Result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
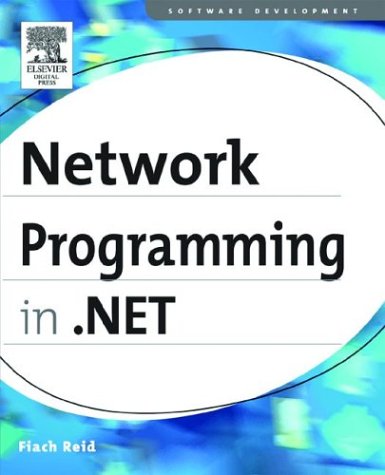
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RowTypePropertyElement.cs
- ResourceReader.cs
- FacetValueContainer.cs
- Vector3DAnimation.cs
- XmlAttribute.cs
- HtmlInputButton.cs
- ScrollProviderWrapper.cs
- TypeNameConverter.cs
- PointConverter.cs
- RsaElement.cs
- EventEntry.cs
- AdornerPresentationContext.cs
- TaskScheduler.cs
- ReaderWriterLockWrapper.cs
- ProxyWebPartManagerDesigner.cs
- HtmlInputRadioButton.cs
- ToolStripSystemRenderer.cs
- EditorPart.cs
- PointAnimation.cs
- GACIdentityPermission.cs
- ResourcesChangeInfo.cs
- AdCreatedEventArgs.cs
- Pair.cs
- SelectionProviderWrapper.cs
- MetadataItem_Static.cs
- XmlSerializerSection.cs
- MonthChangedEventArgs.cs
- MTConfigUtil.cs
- ResourceSet.cs
- ServiceContractViewControl.cs
- Timeline.cs
- InvokeMethodActivityDesigner.cs
- CollectionBuilder.cs
- CodeCommentStatement.cs
- MsmqIntegrationProcessProtocolHandler.cs
- AssemblyInfo.cs
- DrawToolTipEventArgs.cs
- CultureInfoConverter.cs
- WebPartMenu.cs
- EntityClassGenerator.cs
- BitArray.cs
- ProcessExitedException.cs
- SQLString.cs
- Size.cs
- BinHexDecoder.cs
- TraceSection.cs
- GroupBoxAutomationPeer.cs
- securitycriticaldataClass.cs
- TransformPattern.cs
- MasterPage.cs
- Policy.cs
- TraceSection.cs
- DecoderNLS.cs
- FacetDescriptionElement.cs
- WebBrowserSiteBase.cs
- ChannelSinkStacks.cs
- CheckedPointers.cs
- ArcSegment.cs
- EventlogProvider.cs
- WebPartConnectionsCloseVerb.cs
- ProcessThreadDesigner.cs
- IdentityNotMappedException.cs
- DependencyObject.cs
- StringConcat.cs
- MissingSatelliteAssemblyException.cs
- HelpKeywordAttribute.cs
- FlowLayoutPanel.cs
- PatternMatcher.cs
- Substitution.cs
- XmlWhitespace.cs
- SimpleHandlerFactory.cs
- SimpleTypeResolver.cs
- OracleBinary.cs
- HiddenFieldPageStatePersister.cs
- DependentList.cs
- validation.cs
- ReaderWriterLock.cs
- ToolboxItemAttribute.cs
- BrowserInteropHelper.cs
- URLIdentityPermission.cs
- ItemsControl.cs
- DbException.cs
- CategoryAttribute.cs
- Menu.cs
- TriggerActionCollection.cs
- Binding.cs
- IncrementalCompileAnalyzer.cs
- XPathNodeInfoAtom.cs
- ClassGenerator.cs
- BaseTemplatedMobileComponentEditor.cs
- DbReferenceCollection.cs
- _ConnectStream.cs
- EntityUtil.cs
- ImmutableObjectAttribute.cs
- ComAdminWrapper.cs
- OutputCacheSettings.cs
- LineGeometry.cs
- CollectionChangeEventArgs.cs
- QilList.cs
- ToolboxDataAttribute.cs