Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / CheckedPointers.cs / 1 / CheckedPointers.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Checked pointers for various types // // History: // 05/09/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Security; using MS.Internal.Shaping; using MS.Internal.FontCache; // // The file contains wrapper structs for various pointer types. // This is to allow us passing these pointers safely in layout code and provides // some bound checking. Only construction and probing into these pointers are security critical. // namespace MS.Internal { ////// Checked pointer for (Char*) /// internal struct CheckedCharPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedCharPointer(char * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(char)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe char * Probe(int offset, int length) { return (char*) _checkedPointer.Probe(offset * sizeof(char), length * sizeof(char)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (int*) /// internal struct CheckedIntPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedIntPointer(int * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(int)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe int * Probe(int offset, int length) { return (int *) _checkedPointer.Probe(offset * sizeof(int), length * sizeof(int)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (ushort*) /// internal struct CheckedUShortPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedUShortPointer(ushort * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(ushort)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe ushort * Probe(int offset, int length) { return (ushort *) _checkedPointer.Probe(offset * sizeof(ushort), length * sizeof(ushort)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (GlyphOffset*) /// internal struct CheckedGlyphOffsetPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphOffsetPointer(GlyphOffset* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphOffset)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe GlyphOffset * Probe(int offset, int length) { return (GlyphOffset *) _checkedPointer.Probe(offset * sizeof(GlyphOffset), length * sizeof(GlyphOffset)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (GlyphShapingProperties*) /// internal struct CheckedGlyphShapingPropertiesPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphShapingPropertiesPointer(GlyphShapingProperties* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphShapingProperties)); } ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphShapingPropertiesPointer(uint* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphShapingProperties)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe GlyphShapingProperties * Probe(int offset, int length) { return (GlyphShapingProperties *) _checkedPointer.Probe(offset * sizeof(GlyphShapingProperties), length * sizeof(GlyphShapingProperties)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (CharacterShapingProperties*) /// internal struct CheckedCharacterShapingPropertiesPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedCharacterShapingPropertiesPointer(CharacterShapingProperties* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(CharacterShapingProperties)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe CharacterShapingProperties * Probe(int offset, int length) { return (CharacterShapingProperties *) _checkedPointer.Probe(offset * sizeof(CharacterShapingProperties), length * sizeof(CharacterShapingProperties)); } private CheckedPointer _checkedPointer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Checked pointers for various types // // History: // 05/09/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Security; using MS.Internal.Shaping; using MS.Internal.FontCache; // // The file contains wrapper structs for various pointer types. // This is to allow us passing these pointers safely in layout code and provides // some bound checking. Only construction and probing into these pointers are security critical. // namespace MS.Internal { ////// Checked pointer for (Char*) /// internal struct CheckedCharPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedCharPointer(char * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(char)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe char * Probe(int offset, int length) { return (char*) _checkedPointer.Probe(offset * sizeof(char), length * sizeof(char)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (int*) /// internal struct CheckedIntPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedIntPointer(int * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(int)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe int * Probe(int offset, int length) { return (int *) _checkedPointer.Probe(offset * sizeof(int), length * sizeof(int)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (ushort*) /// internal struct CheckedUShortPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedUShortPointer(ushort * pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(ushort)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe ushort * Probe(int offset, int length) { return (ushort *) _checkedPointer.Probe(offset * sizeof(ushort), length * sizeof(ushort)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (GlyphOffset*) /// internal struct CheckedGlyphOffsetPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphOffsetPointer(GlyphOffset* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphOffset)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe GlyphOffset * Probe(int offset, int length) { return (GlyphOffset *) _checkedPointer.Probe(offset * sizeof(GlyphOffset), length * sizeof(GlyphOffset)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (GlyphShapingProperties*) /// internal struct CheckedGlyphShapingPropertiesPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphShapingPropertiesPointer(GlyphShapingProperties* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphShapingProperties)); } ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedGlyphShapingPropertiesPointer(uint* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(GlyphShapingProperties)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe GlyphShapingProperties * Probe(int offset, int length) { return (GlyphShapingProperties *) _checkedPointer.Probe(offset * sizeof(GlyphShapingProperties), length * sizeof(GlyphShapingProperties)); } private CheckedPointer _checkedPointer; } ////// Checked pointer for (CharacterShapingProperties*) /// internal struct CheckedCharacterShapingPropertiesPointer { ////// Critical - The method takes unsafe pointer /// [SecurityCritical] internal unsafe CheckedCharacterShapingPropertiesPointer(CharacterShapingProperties* pointer, int length) { _checkedPointer = new CheckedPointer(pointer, length * sizeof(CharacterShapingProperties)); } ////// Critical - The method returns unsafe pointer /// [SecurityCritical] internal unsafe CharacterShapingProperties * Probe(int offset, int length) { return (CharacterShapingProperties *) _checkedPointer.Probe(offset * sizeof(CharacterShapingProperties), length * sizeof(CharacterShapingProperties)); } private CheckedPointer _checkedPointer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
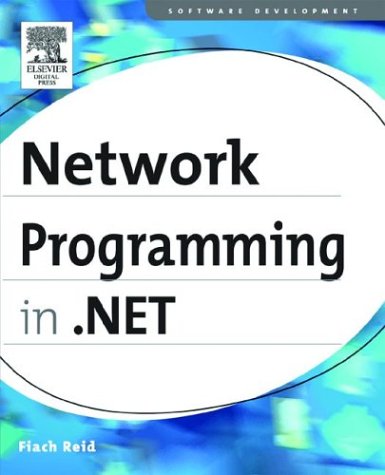
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputScope.cs
- BinaryFormatter.cs
- SpotLight.cs
- PackWebResponse.cs
- IResourceProvider.cs
- SpeakProgressEventArgs.cs
- SingleResultAttribute.cs
- ResourceDictionaryCollection.cs
- ParameterCollection.cs
- PermissionSetEnumerator.cs
- ColumnMapVisitor.cs
- XmlSerializer.cs
- TextDecorations.cs
- SystemIcmpV6Statistics.cs
- WebPartVerbCollection.cs
- DbConnectionFactory.cs
- SchemaCollectionPreprocessor.cs
- BaseDataList.cs
- ObjectQuery_EntitySqlExtensions.cs
- PageAdapter.cs
- AppearanceEditorPart.cs
- SQLInt16Storage.cs
- EdmTypeAttribute.cs
- GenericPrincipal.cs
- PackageRelationship.cs
- ColorConvertedBitmap.cs
- DesignerDataSourceView.cs
- SecurityRuntime.cs
- SqlDependencyUtils.cs
- storepermission.cs
- TokenBasedSetEnumerator.cs
- MethodSet.cs
- SqlWebEventProvider.cs
- Base64Decoder.cs
- WebColorConverter.cs
- ConfigurationProperty.cs
- ToolStripOverflow.cs
- SerializationFieldInfo.cs
- InstanceValue.cs
- StoreUtilities.cs
- ArraySortHelper.cs
- ImageUrlEditor.cs
- ArrayConverter.cs
- CodeGen.cs
- TableLayoutPanelCellPosition.cs
- SessionStateContainer.cs
- SQLByte.cs
- rsa.cs
- TreeBuilder.cs
- DynamicControlParameter.cs
- WebCategoryAttribute.cs
- RawStylusActions.cs
- ScriptDescriptor.cs
- ObjectViewQueryResultData.cs
- CompressionTracing.cs
- DocumentsTrace.cs
- AsyncSerializedWorker.cs
- _BufferOffsetSize.cs
- CompilerParameters.cs
- BindingsCollection.cs
- WebPartMovingEventArgs.cs
- ListCollectionView.cs
- COM2EnumConverter.cs
- DesignerActionVerbItem.cs
- ProfileServiceManager.cs
- SerialErrors.cs
- Item.cs
- SpeechRecognizer.cs
- ItemCheckedEvent.cs
- ImmComposition.cs
- OleDbPermission.cs
- DetectRunnableInstancesTask.cs
- BooleanStorage.cs
- PopupRootAutomationPeer.cs
- EntityContainerEmitter.cs
- SettingsProperty.cs
- DefaultBindingPropertyAttribute.cs
- WebPartConnectionsCancelEventArgs.cs
- RelationalExpressions.cs
- WebEventTraceProvider.cs
- XmlDataDocument.cs
- HwndTarget.cs
- XmlHelper.cs
- ColumnCollection.cs
- InputLanguageCollection.cs
- DataSet.cs
- CompositeCollectionView.cs
- Hashtable.cs
- BamlReader.cs
- Timer.cs
- ScriptControl.cs
- StreamSecurityUpgradeAcceptorBase.cs
- SByte.cs
- CodeMemberEvent.cs
- ObjectDataSourceMethodEventArgs.cs
- BackStopAuthenticationModule.cs
- EtwTrace.cs
- List.cs
- DefinitionUpdate.cs
- RequestSecurityTokenForRemoteTokenFactory.cs