Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / ObjectViewQueryResultData.cs / 1 / ObjectViewQueryResultData.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata; using System.Data.Metadata.Edm; using System.Data.Objects.DataClasses; using System.Diagnostics; namespace System.Data.Objects { ////// Manages a binding list constructed from query results. /// ////// Type of the elements in the binding list. /// ////// The binding list is initialized from query results. /// If the binding list can be modified, /// objects are added or removed from the ObjectStateManager (via the ObjectContext). /// internal sealed class ObjectViewQueryResultData: IObjectViewData { private List _bindingList; /// /// ObjectContext used to add or delete objects when the list can be modified. /// private ObjectContext _objectContext; ////// If the TElement type is an Entity type of some kind, /// this field specifies the entity set to add entity objects. /// private EntitySet _entitySet; private bool _canEditItems; private bool _canModifyList; ////// Construct a new instance of the ObjectViewQueryResultData class using the supplied query results. /// /// /// Result of object query execution used to populate the binding list. /// /// /// ObjectContext used to add or remove items. /// If the binding list can be modified, this parameter should not be null. /// /// /// True if items should not be allowed to be added or removed from the binding list. /// Note that other conditions may prevent the binding list from being modified, so a value of false /// supplied for this parameter doesn't necessarily mean that the list will be writable. /// /// /// If the TElement type is an Entity type of some kind, /// this field specifies the entity set to add entity objects. /// internal ObjectViewQueryResultData(IEnumerable queryResults, ObjectContext objectContext, bool forceReadOnlyList, EntitySet entitySet) { bool canTrackItemChanges = typeof(IEntityWithChangeTracker).IsAssignableFrom(typeof(TElement)); _objectContext = objectContext; _entitySet = entitySet; _canEditItems = canTrackItemChanges; _canModifyList = !forceReadOnlyList && canTrackItemChanges && _objectContext != null; _bindingList = new List(); foreach (TElement element in queryResults) { _bindingList.Add(element); } } /// /// Throw an exception is an entity set was not specified for this instance. /// private void EnsureEntitySet() { if (_entitySet == null) { throw EntityUtil.CannotResolveTheEntitySetforGivenEntity(typeof(TElement)); } } #region IObjectViewDataMembers public IList List { get { return _bindingList; } } public bool AllowNew { get { return _canModifyList && _entitySet != null; } } public bool AllowEdit { get { return _canEditItems; } } public bool AllowRemove { get { return _canModifyList; } } public bool FiresEventOnAdd { get { return false; } } public bool FiresEventOnRemove { get { return true; } } public bool FiresEventOnClear { get { return false; } } public void EnsureCanAddNew() { EnsureEntitySet(); } public int Add(TElement item, bool isAddNew) { EnsureEntitySet(); Debug.Assert(_objectContext != null, "ObjectContext is null."); Debug.Assert(item is IEntityWithChangeTracker, "Item to add is not an IEntityWithChangeTracker."); // If called for AddNew operation, add item to binding list, pending addition to ObjectContext. if (!isAddNew) { _objectContext.AddObject(TypeHelpers.GetFullName(_entitySet), item); } _bindingList.Add(item); return _bindingList.Count - 1; } public void CommitItemAt(int index) { EnsureEntitySet(); Debug.Assert(_objectContext != null, "ObjectContext is null."); TElement item = _bindingList[index]; _objectContext.AddObject(TypeHelpers.GetFullName(_entitySet), item); } public void Clear() { while (0 < _bindingList.Count) { TElement entity = _bindingList[_bindingList.Count - 1]; Remove(entity, false); } } public bool Remove(TElement item, bool isCancelNew) { bool removed; Debug.Assert(_objectContext != null, "ObjectContext is null."); if (isCancelNew) { // Item was previously added to binding list, but not ObjectContext. removed = _bindingList.Remove(item); } else { ObjectStateEntry stateEntry = _objectContext.ObjectStateManager.FindObjectStateEntry(item); if (stateEntry != null) { stateEntry.Delete(); // OnCollectionChanged event will be fired, where the binding list will be updated. removed = true; } else { removed = false; } } return removed; } public ListChangedEventArgs OnCollectionChanged(object sender, CollectionChangeEventArgs e, ObjectViewListener listener) { ListChangedEventArgs changeArgs = null; // Since event is coming from cache and it might be shared amoung different queries // we have to check to see if correct event is being handled. if (e.Element.GetType().IsAssignableFrom(typeof(TElement)) && _bindingList.Contains((TElement)(e.Element))) { TElement item = (TElement)e.Element; int itemIndex = _bindingList.IndexOf(item); if (itemIndex >= 0) // Ignore entities that we don't know about. { // Only process "remove" events. Debug.Assert(e.Action != CollectionChangeAction.Refresh, "Cache should never fire with refresh, it does not have clear"); if (e.Action == CollectionChangeAction.Remove) { _bindingList.Remove(item); listener.UnregisterEntityEvents(item); changeArgs = new ListChangedEventArgs(ListChangedType.ItemDeleted, itemIndex /* newIndex*/, -1 /* oldIndex*/); } } } return changeArgs; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata; using System.Data.Metadata.Edm; using System.Data.Objects.DataClasses; using System.Diagnostics; namespace System.Data.Objects { ////// Manages a binding list constructed from query results. /// ////// Type of the elements in the binding list. /// ////// The binding list is initialized from query results. /// If the binding list can be modified, /// objects are added or removed from the ObjectStateManager (via the ObjectContext). /// internal sealed class ObjectViewQueryResultData: IObjectViewData { private List _bindingList; /// /// ObjectContext used to add or delete objects when the list can be modified. /// private ObjectContext _objectContext; ////// If the TElement type is an Entity type of some kind, /// this field specifies the entity set to add entity objects. /// private EntitySet _entitySet; private bool _canEditItems; private bool _canModifyList; ////// Construct a new instance of the ObjectViewQueryResultData class using the supplied query results. /// /// /// Result of object query execution used to populate the binding list. /// /// /// ObjectContext used to add or remove items. /// If the binding list can be modified, this parameter should not be null. /// /// /// True if items should not be allowed to be added or removed from the binding list. /// Note that other conditions may prevent the binding list from being modified, so a value of false /// supplied for this parameter doesn't necessarily mean that the list will be writable. /// /// /// If the TElement type is an Entity type of some kind, /// this field specifies the entity set to add entity objects. /// internal ObjectViewQueryResultData(IEnumerable queryResults, ObjectContext objectContext, bool forceReadOnlyList, EntitySet entitySet) { bool canTrackItemChanges = typeof(IEntityWithChangeTracker).IsAssignableFrom(typeof(TElement)); _objectContext = objectContext; _entitySet = entitySet; _canEditItems = canTrackItemChanges; _canModifyList = !forceReadOnlyList && canTrackItemChanges && _objectContext != null; _bindingList = new List(); foreach (TElement element in queryResults) { _bindingList.Add(element); } } /// /// Throw an exception is an entity set was not specified for this instance. /// private void EnsureEntitySet() { if (_entitySet == null) { throw EntityUtil.CannotResolveTheEntitySetforGivenEntity(typeof(TElement)); } } #region IObjectViewDataMembers public IList List { get { return _bindingList; } } public bool AllowNew { get { return _canModifyList && _entitySet != null; } } public bool AllowEdit { get { return _canEditItems; } } public bool AllowRemove { get { return _canModifyList; } } public bool FiresEventOnAdd { get { return false; } } public bool FiresEventOnRemove { get { return true; } } public bool FiresEventOnClear { get { return false; } } public void EnsureCanAddNew() { EnsureEntitySet(); } public int Add(TElement item, bool isAddNew) { EnsureEntitySet(); Debug.Assert(_objectContext != null, "ObjectContext is null."); Debug.Assert(item is IEntityWithChangeTracker, "Item to add is not an IEntityWithChangeTracker."); // If called for AddNew operation, add item to binding list, pending addition to ObjectContext. if (!isAddNew) { _objectContext.AddObject(TypeHelpers.GetFullName(_entitySet), item); } _bindingList.Add(item); return _bindingList.Count - 1; } public void CommitItemAt(int index) { EnsureEntitySet(); Debug.Assert(_objectContext != null, "ObjectContext is null."); TElement item = _bindingList[index]; _objectContext.AddObject(TypeHelpers.GetFullName(_entitySet), item); } public void Clear() { while (0 < _bindingList.Count) { TElement entity = _bindingList[_bindingList.Count - 1]; Remove(entity, false); } } public bool Remove(TElement item, bool isCancelNew) { bool removed; Debug.Assert(_objectContext != null, "ObjectContext is null."); if (isCancelNew) { // Item was previously added to binding list, but not ObjectContext. removed = _bindingList.Remove(item); } else { ObjectStateEntry stateEntry = _objectContext.ObjectStateManager.FindObjectStateEntry(item); if (stateEntry != null) { stateEntry.Delete(); // OnCollectionChanged event will be fired, where the binding list will be updated. removed = true; } else { removed = false; } } return removed; } public ListChangedEventArgs OnCollectionChanged(object sender, CollectionChangeEventArgs e, ObjectViewListener listener) { ListChangedEventArgs changeArgs = null; // Since event is coming from cache and it might be shared amoung different queries // we have to check to see if correct event is being handled. if (e.Element.GetType().IsAssignableFrom(typeof(TElement)) && _bindingList.Contains((TElement)(e.Element))) { TElement item = (TElement)e.Element; int itemIndex = _bindingList.IndexOf(item); if (itemIndex >= 0) // Ignore entities that we don't know about. { // Only process "remove" events. Debug.Assert(e.Action != CollectionChangeAction.Refresh, "Cache should never fire with refresh, it does not have clear"); if (e.Action == CollectionChangeAction.Remove) { _bindingList.Remove(item); listener.UnregisterEntityEvents(item); changeArgs = new ListChangedEventArgs(ListChangedType.ItemDeleted, itemIndex /* newIndex*/, -1 /* oldIndex*/); } } } return changeArgs; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
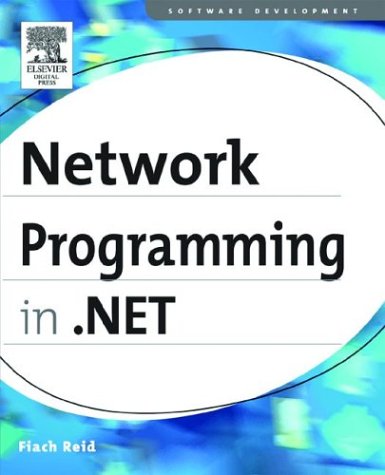
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOLocalFileHeader.cs
- CurrentChangingEventArgs.cs
- Pkcs7Recipient.cs
- ListItemParagraph.cs
- ValidatingReaderNodeData.cs
- SimpleApplicationHost.cs
- RowCache.cs
- ArcSegment.cs
- SessionSwitchEventArgs.cs
- DesigntimeLicenseContextSerializer.cs
- InternalDispatchObject.cs
- OracleDataAdapter.cs
- ToolStripActionList.cs
- OptimalBreakSession.cs
- ArrayConverter.cs
- NamedPipeConnectionPool.cs
- Pair.cs
- PropertyDescriptorCollection.cs
- FixedDocumentSequencePaginator.cs
- MiniMapControl.xaml.cs
- PropertyIDSet.cs
- CellNormalizer.cs
- wgx_exports.cs
- ClipboardProcessor.cs
- NamespaceDecl.cs
- XmlFileEditor.cs
- codemethodreferenceexpression.cs
- CultureNotFoundException.cs
- AssemblyBuilder.cs
- RenderDataDrawingContext.cs
- NodeInfo.cs
- AmbientLight.cs
- securestring.cs
- QilInvokeLateBound.cs
- DesignerContextDescriptor.cs
- CalendarKeyboardHelper.cs
- ComponentSerializationService.cs
- SettingsPropertyValue.cs
- FamilyTypefaceCollection.cs
- ConfigurationElementCollection.cs
- SQLRoleProvider.cs
- CodeVariableReferenceExpression.cs
- EditingMode.cs
- IChannel.cs
- DocumentScope.cs
- Fonts.cs
- DescendantBaseQuery.cs
- AttributeProviderAttribute.cs
- Atom10ItemFormatter.cs
- Utils.cs
- KeysConverter.cs
- TriggerCollection.cs
- FrameworkObject.cs
- Schema.cs
- FormViewPageEventArgs.cs
- OleDbParameter.cs
- ProcessThread.cs
- ExpandCollapseProviderWrapper.cs
- TextPattern.cs
- MembershipValidatePasswordEventArgs.cs
- WindowCollection.cs
- DataGridViewRowHeaderCell.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- CacheDependency.cs
- followingquery.cs
- ComplexBindingPropertiesAttribute.cs
- XPathParser.cs
- ProfilePropertySettingsCollection.cs
- ToolStripItemCollection.cs
- Decimal.cs
- EncodingInfo.cs
- MailAddress.cs
- GroupBoxRenderer.cs
- DataServices.cs
- DeviceContext.cs
- Utils.cs
- WindowShowOrOpenTracker.cs
- BaseValidator.cs
- ArraySegment.cs
- ValidateNames.cs
- DocumentSchemaValidator.cs
- InvalidPipelineStoreException.cs
- ICollection.cs
- Pointer.cs
- TiffBitmapDecoder.cs
- SchemaTableColumn.cs
- PointLight.cs
- XmlHierarchyData.cs
- compensatingcollection.cs
- UnknownExceptionActionHelper.cs
- SspiHelper.cs
- _CookieModule.cs
- ToolbarAUtomationPeer.cs
- Knowncolors.cs
- TypeElement.cs
- LinqDataSource.cs
- MetadataItemEmitter.cs
- ErrorTableItemStyle.cs
- DelegatingConfigHost.cs
- ImageDrawing.cs