Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / Atom10ItemFormatter.cs / 1 / Atom10ItemFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Text; using System.Xml; using System.Xml.Serialization; using System.Diagnostics.CodeAnalysis; using System.Xml.Schema; [XmlRoot(ElementName = Atom10Constants.EntryTag, Namespace = Atom10Constants.Atom10Namespace)] public class Atom10ItemFormatter : SyndicationItemFormatter, IXmlSerializable { Atom10FeedFormatter feedSerializer; Type itemType; bool preserveAttributeExtensions; bool preserveElementExtensions; public Atom10ItemFormatter() : this(typeof(SyndicationItem)) { } public Atom10ItemFormatter(Type itemTypeToCreate) : base() { if (itemTypeToCreate == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("itemTypeToCreate"); } if (!typeof(SyndicationItem).IsAssignableFrom(itemTypeToCreate)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("itemTypeToCreate", SR2.GetString(SR2.InvalidObjectTypePassed, "itemTypeToCreate", "SyndicationItem")); } this.feedSerializer = new Atom10FeedFormatter(); this.feedSerializer.PreserveAttributeExtensions = this.preserveAttributeExtensions = true; this.feedSerializer.PreserveElementExtensions = this.preserveElementExtensions = true; this.itemType = itemTypeToCreate; } public Atom10ItemFormatter(SyndicationItem itemToWrite) : base(itemToWrite) { // No need to check that the parameter passed is valid - it is checked by the c'tor of the base class this.feedSerializer = new Atom10FeedFormatter(); this.feedSerializer.PreserveAttributeExtensions = this.preserveAttributeExtensions = true; this.feedSerializer.PreserveElementExtensions = this.preserveElementExtensions = true; this.itemType = itemToWrite.GetType(); } public bool PreserveAttributeExtensions { get { return this.preserveAttributeExtensions; } set { this.preserveAttributeExtensions = value; this.feedSerializer.PreserveAttributeExtensions = value; } } public bool PreserveElementExtensions { get { return this.preserveElementExtensions; } set { this.preserveElementExtensions = value; this.feedSerializer.PreserveElementExtensions = value; } } public override string Version { get { return SyndicationVersions.Atom10; } } protected Type ItemType { get { return this.itemType; } } public override bool CanRead(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return reader.IsStartElement(Atom10Constants.EntryTag, Atom10Constants.Atom10Namespace); } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] XmlSchema IXmlSerializable.GetSchema() { return null; } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] void IXmlSerializable.ReadXml(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } SyndicationFeedFormatter.TraceItemReadBegin(); ReadItem(reader); SyndicationFeedFormatter.TraceItemReadEnd(); } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] void IXmlSerializable.WriteXml(XmlWriter writer) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } SyndicationFeedFormatter.TraceItemWriteBegin(); WriteItem(writer); SyndicationFeedFormatter.TraceItemWriteEnd(); } public override void ReadFrom(XmlReader reader) { SyndicationFeedFormatter.TraceItemReadBegin(); if (!CanRead(reader)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.UnknownItemXml, reader.LocalName, reader.NamespaceURI))); } ReadItem(reader); SyndicationFeedFormatter.TraceItemReadEnd(); } public override void WriteTo(XmlWriter writer) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } SyndicationFeedFormatter.TraceItemWriteBegin(); writer.WriteStartElement(Atom10Constants.EntryTag, Atom10Constants.Atom10Namespace); WriteItem(writer); writer.WriteEndElement(); SyndicationFeedFormatter.TraceItemWriteEnd(); } protected override SyndicationItem CreateItemInstance() { return SyndicationItemFormatter.CreateItemInstance(this.itemType); } void ReadItem(XmlReader reader) { SetItem(CreateItemInstance()); feedSerializer.ReadItemFrom(XmlDictionaryReader.CreateDictionaryReader(reader), this.Item); } void WriteItem(XmlWriter writer) { if (this.Item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.ItemFormatterDoesNotHaveItem))); } XmlDictionaryWriter w = XmlDictionaryWriter.CreateDictionaryWriter(writer); feedSerializer.WriteItemContents(w, this.Item); } } [XmlRoot(ElementName = Atom10Constants.EntryTag, Namespace = Atom10Constants.Atom10Namespace)] public class Atom10ItemFormatter: Atom10ItemFormatter where TSyndicationItem : SyndicationItem, new () { // constructors public Atom10ItemFormatter() : base(typeof(TSyndicationItem)) { } public Atom10ItemFormatter(TSyndicationItem itemToWrite) : base(itemToWrite) { } protected override SyndicationItem CreateItemInstance() { return new TSyndicationItem(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
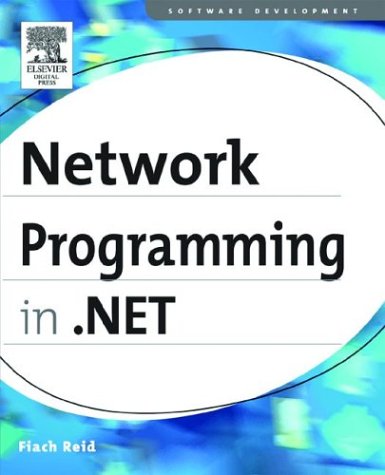
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlReflectionImporter.cs
- TextSpan.cs
- ObjectNavigationPropertyMapping.cs
- ListDictionary.cs
- processwaithandle.cs
- Preprocessor.cs
- GridViewUpdateEventArgs.cs
- UndirectedGraph.cs
- FigureHelper.cs
- NameTable.cs
- DelegateSerializationHolder.cs
- CharStorage.cs
- CollectionChangedEventManager.cs
- SafeFileMappingHandle.cs
- ScopelessEnumAttribute.cs
- Evidence.cs
- StateFinalizationDesigner.cs
- ObjectStateFormatter.cs
- AutoFocusStyle.xaml.cs
- CustomCredentialPolicy.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WebPartDescriptionCollection.cs
- UnsafeNativeMethods.cs
- SchemaTableColumn.cs
- ModuleElement.cs
- WebPartTracker.cs
- InternalBase.cs
- PopOutPanel.cs
- SynchronizedInputProviderWrapper.cs
- StrongName.cs
- ObjectMemberMapping.cs
- WebCategoryAttribute.cs
- Utils.cs
- HtmlGenericControl.cs
- GroupBoxRenderer.cs
- BitVector32.cs
- WebPartDescriptionCollection.cs
- CreateUserWizardDesigner.cs
- ServiceHostingEnvironment.cs
- HttpCachePolicy.cs
- RayHitTestParameters.cs
- Clipboard.cs
- StylusCaptureWithinProperty.cs
- TextSpan.cs
- PointLight.cs
- Dynamic.cs
- PartialClassGenerationTaskInternal.cs
- PreparingEnlistment.cs
- URLIdentityPermission.cs
- PopupControlService.cs
- Site.cs
- _NegoState.cs
- WindowsScroll.cs
- EncoderNLS.cs
- RichTextBoxDesigner.cs
- TemplateAction.cs
- Point3DConverter.cs
- MD5.cs
- WebRequestModuleElement.cs
- CodeTypeMemberCollection.cs
- RTLAwareMessageBox.cs
- SafeCancelMibChangeNotify.cs
- WizardPanelChangingEventArgs.cs
- DataServiceQueryOfT.cs
- InfoCardAsymmetricCrypto.cs
- InvalidCastException.cs
- Cloud.cs
- LayoutManager.cs
- PageContentCollection.cs
- NativeCppClassAttribute.cs
- DateTimeSerializationSection.cs
- ExpressionBuilderCollection.cs
- XmlILConstructAnalyzer.cs
- Effect.cs
- VariableAction.cs
- BinaryCommonClasses.cs
- WorkflowInstanceExtensionProvider.cs
- TextBoxBaseDesigner.cs
- InputGestureCollection.cs
- BevelBitmapEffect.cs
- BindingMemberInfo.cs
- MenuCommands.cs
- AssemblyAttributesGoHere.cs
- DataGridViewRowHeaderCell.cs
- _TimerThread.cs
- cookieexception.cs
- MulticastIPAddressInformationCollection.cs
- Activation.cs
- PkcsMisc.cs
- RuleInfoComparer.cs
- SamlSubject.cs
- _TimerThread.cs
- ProfileParameter.cs
- Splitter.cs
- ByteStack.cs
- EntityDesignerDataSourceView.cs
- BaseResourcesBuildProvider.cs
- VariantWrapper.cs
- NumberFormatInfo.cs
- Site.cs