Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / RichTextBoxDesigner.cs / 1 / RichTextBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.RichTextBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Reflection; using System.Windows.Forms; ////// /// The RichTextBoxDesigner provides rich designtime behavior for the /// RichTextBox control. /// internal class RichTextBoxDesigner : TextBoxBaseDesigner { private DesignerActionListCollection _actionLists; ////// Called when the designer is intialized. This allows the designer to provide some /// meaningful default values in the control. The default implementation of this /// sets the control's text to its name. /// public override void InitializeNewComponent(IDictionary defaultValues) { base.InitializeNewComponent(defaultValues); // Disable DragDrop at design time. // Control control = Control; if (control != null && control.Handle != IntPtr.Zero) { NativeMethods.RevokeDragDrop(control.Handle); // DragAcceptFiles(control.Handle, false); } } public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); _actionLists.Add(new RichTextBoxActionList(this)); } return _actionLists; } } ////// /// Allows a designer to filter the set of properties /// the component it is designing will expose through the /// TypeDescriptor object. This method is called /// immediately before its corresponding "Post" method. /// If you are overriding this method you should call /// the base implementation before you perform your own /// filtering. /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; // Handle shadowed properties // string[] shadowProps = new string[] { "Text" }; Attribute[] empty = new Attribute[0]; for (int i = 0; i < shadowProps.Length; i++) { prop = (PropertyDescriptor)properties[shadowProps[i]]; if (prop != null) { properties[shadowProps[i]] = TypeDescriptor.CreateProperty(typeof(RichTextBoxDesigner), prop, empty); } } } ////// Accessor for Text. We need to replace "\r\n" with "\n" in the designer before deciding whether /// the old value & new value match. /// private string Text { get { return Control.Text; } set { string oldText = Control.Text; if (value != null) { value = value.Replace("\r\n", "\n"); } if (oldText != value) { Control.Text = value; } } } } internal class RichTextBoxActionList : DesignerActionList { private RichTextBoxDesigner _designer; public RichTextBoxActionList(RichTextBoxDesigner designer) : base(designer.Component) { _designer = designer; } public void EditLines() { EditorServiceContext.EditValue(_designer, Component, "Lines"); } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); items.Add(new DesignerActionMethodItem(this, "EditLines", SR.GetString(SR.EditLinesDisplayName), SR.GetString(SR.LinksCategoryName), SR.GetString(SR.EditLinesDescription), true)); return items; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
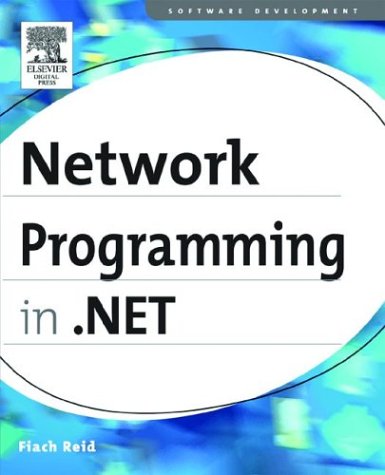
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _RequestCacheProtocol.cs
- IssuanceTokenProviderState.cs
- BufferedReceiveManager.cs
- AssemblyAttributes.cs
- BaseContextMenu.cs
- ResXDataNode.cs
- DependencySource.cs
- PeerContact.cs
- BufferBuilder.cs
- TypeLibConverter.cs
- InvokeMethodActivity.cs
- ListSourceHelper.cs
- VideoDrawing.cs
- UnsafeNativeMethods.cs
- AlternateViewCollection.cs
- TabletDeviceInfo.cs
- ValidationRule.cs
- PrintDialog.cs
- TextServicesContext.cs
- MessageHeaderInfoTraceRecord.cs
- CharacterBufferReference.cs
- DataGridColumnCollection.cs
- PtsPage.cs
- QuotaExceededException.cs
- CompositeDataBoundControl.cs
- WebPermission.cs
- TreeView.cs
- HttpRequest.cs
- figurelength.cs
- PersonalizationProviderCollection.cs
- mediaeventshelper.cs
- DataChangedEventManager.cs
- DateTimeStorage.cs
- Activator.cs
- SerializationAttributes.cs
- TemplateNameScope.cs
- EventlogProvider.cs
- LinkGrep.cs
- SimpleColumnProvider.cs
- XXXInfos.cs
- StringComparer.cs
- CompositionTarget.cs
- HtmlTable.cs
- SqlProvider.cs
- GeneralTransform.cs
- WebPartConnectionsCloseVerb.cs
- Int32Storage.cs
- StringBuilder.cs
- ICspAsymmetricAlgorithm.cs
- CssTextWriter.cs
- HostedTransportConfigurationBase.cs
- Vector3DCollection.cs
- LinqDataSourceView.cs
- DbUpdateCommandTree.cs
- InteropAutomationProvider.cs
- BaseParaClient.cs
- TextContainerHelper.cs
- PtsCache.cs
- ContextMenuStripActionList.cs
- BaseValidator.cs
- WebExceptionStatus.cs
- FilterableAttribute.cs
- MultipartContentParser.cs
- FontDriver.cs
- WebConvert.cs
- ItemDragEvent.cs
- Selection.cs
- DragStartedEventArgs.cs
- ColorBlend.cs
- GeneralTransform3DTo2DTo3D.cs
- MetadataLocation.cs
- DesignerTransactionCloseEvent.cs
- RuleSettings.cs
- TemplateDefinition.cs
- FormViewRow.cs
- FreezableDefaultValueFactory.cs
- NullableFloatAverageAggregationOperator.cs
- wgx_exports.cs
- XamlRtfConverter.cs
- MailAddressParser.cs
- CaseInsensitiveHashCodeProvider.cs
- SpecularMaterial.cs
- CheckBox.cs
- FlowNode.cs
- _Connection.cs
- ConnectionPoint.cs
- ServiceModelStringsVersion1.cs
- WindowsScrollBar.cs
- PropertyItemInternal.cs
- SID.cs
- StringValidatorAttribute.cs
- _AutoWebProxyScriptWrapper.cs
- ProcessInfo.cs
- HttpConfigurationContext.cs
- GridEntryCollection.cs
- SortAction.cs
- WindowsScroll.cs
- AdRotator.cs
- SecurityTokenSerializer.cs
- DataObjectSettingDataEventArgs.cs