Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / TextBoxBaseDesigner.cs / 1 / TextBoxBaseDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Design.Behavior; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; ////// /// internal class TextBoxBaseDesigner : ControlDesigner { public TextBoxBaseDesigner() { AutoResizeHandles = true; } ////// Provides a designer that can design components /// that extend TextBoxBase. ////// /// Adds a baseline SnapLine to the list of SnapLines related /// to this control. /// public override IList SnapLines { get { ArrayList snapLines = base.SnapLines as ArrayList; int baseline = DesignerUtils.GetTextBaseline(Control, System.Drawing.ContentAlignment.TopLeft); BorderStyle borderStyle = BorderStyle.Fixed3D; PropertyDescriptor prop = TypeDescriptor.GetProperties(Component)["BorderStyle"]; if (prop != null) { borderStyle = (BorderStyle)prop.GetValue(Component); } if (borderStyle == BorderStyle.None) { baseline += 0; } else if (borderStyle == BorderStyle.FixedSingle) { baseline += 2; } else if (borderStyle == BorderStyle.Fixed3D) { baseline += 3; } else { Debug.Fail("Unknown borderstyle"); baseline += 0; } snapLines.Add(new SnapLine(SnapLineType.Baseline, baseline, SnapLinePriority.Medium)); return snapLines; } } private string Text { get { return Control.Text; } set { Control.Text = value; // This fixes bug #48462. If the text box is not wide enough to display all of the text, // then we want to display the first portion at design-time. We can ensure this by // setting the selection to (0, 0). // ((TextBoxBase)Control).Select(0, 0); } } private bool ShouldSerializeText() { return TypeDescriptor.GetProperties(typeof(TextBoxBase))["Text"].ShouldSerializeValue(Component); } [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] private void ResetText() { Control.Text = ""; } ////// /// We override this so we can clear the text field set by controldesigner. /// public override void InitializeNewComponent(IDictionary defaultValues) { base.InitializeNewComponent(defaultValues); PropertyDescriptor textProp = TypeDescriptor.GetProperties(Component)["Text"]; if (textProp != null && textProp.PropertyType == typeof(string) && !textProp.IsReadOnly && textProp.IsBrowsable) { textProp.SetValue(Component, ""); } } protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop; // Handle shadowed properties // string[] shadowProps = new string[] { "Text", }; Attribute[] empty = new Attribute[0]; for (int i = 0; i < shadowProps.Length; i++) { prop = (PropertyDescriptor)properties[shadowProps[i]]; if (prop != null) { properties[shadowProps[i]] = TypeDescriptor.CreateProperty(typeof(TextBoxBaseDesigner), prop, empty); } } } ////// /// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = base.SelectionRules; object component = Component; rules |= SelectionRules.AllSizeable; PropertyDescriptor prop = TypeDescriptor.GetProperties(component)["Multiline"]; if (prop != null) { Object value = prop.GetValue(component); if (value is bool && (bool)value == false) { PropertyDescriptor propAuto = TypeDescriptor.GetProperties(component)["AutoSize"]; if (propAuto != null) { Object auto = propAuto.GetValue(component); //VSWhidbey #369288 if (auto is bool && (bool)auto == true) { rules &= ~(SelectionRules.TopSizeable | SelectionRules.BottomSizeable); } } } } return rules; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
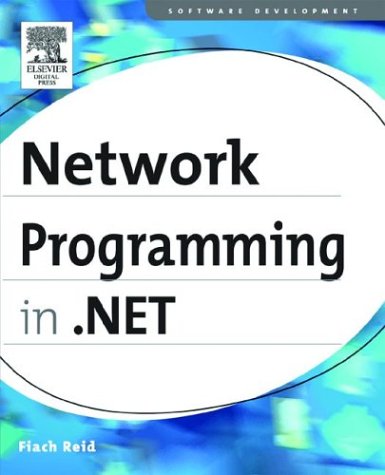
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LineInfo.cs
- DirectoryNotFoundException.cs
- ResourceManager.cs
- HandlerMappingMemo.cs
- Transform3DGroup.cs
- NumericUpDownAccelerationCollection.cs
- DurationConverter.cs
- FocusManager.cs
- DataGridViewComboBoxColumn.cs
- CheckBoxField.cs
- StatusBarDrawItemEvent.cs
- MergeFilterQuery.cs
- TransactionManagerProxy.cs
- KeyValueSerializer.cs
- CodeDomLocalizationProvider.cs
- CompilationUnit.cs
- UnaryOperationBinder.cs
- TagMapInfo.cs
- Internal.cs
- FacetValueContainer.cs
- LocalTransaction.cs
- ProjectionRewriter.cs
- WindowsTitleBar.cs
- ScriptResourceDefinition.cs
- DataServiceRequestArgs.cs
- XMLDiffLoader.cs
- XamlVector3DCollectionSerializer.cs
- BatchWriter.cs
- SqlCacheDependencyDatabaseCollection.cs
- TemplateControlCodeDomTreeGenerator.cs
- DesignerDataSourceView.cs
- RemoveStoryboard.cs
- SplineKeyFrames.cs
- WebBrowserDocumentCompletedEventHandler.cs
- WizardStepBase.cs
- odbcmetadatafactory.cs
- FileDialog_Vista.cs
- WmpBitmapEncoder.cs
- PathSegment.cs
- GlyphInfoList.cs
- MetaModel.cs
- MembershipSection.cs
- InputLangChangeEvent.cs
- ToolStripOverflow.cs
- TopClause.cs
- DES.cs
- StructureChangedEventArgs.cs
- ToolStripProgressBar.cs
- FileDialog.cs
- InkCanvasSelectionAdorner.cs
- PartialList.cs
- CreateInstanceBinder.cs
- DescendantQuery.cs
- ConfigXmlText.cs
- CacheVirtualItemsEvent.cs
- UndirectedGraph.cs
- ToolboxItemCollection.cs
- ContractListAdapter.cs
- Int32Animation.cs
- SafeEventLogWriteHandle.cs
- ExpandCollapsePattern.cs
- NamedPipeConnectionPool.cs
- cache.cs
- DataSetMappper.cs
- WorkflowItemsPresenter.cs
- EntityKey.cs
- basevalidator.cs
- CorrelationResolver.cs
- FeatureSupport.cs
- DataTrigger.cs
- RelationshipManager.cs
- processwaithandle.cs
- WindowsGraphics2.cs
- ResourceDescriptionAttribute.cs
- TimeSpanConverter.cs
- XsltException.cs
- DiscoveryExceptionDictionary.cs
- ContractListAdapter.cs
- WebConfigurationManager.cs
- filewebrequest.cs
- PropertyReferenceSerializer.cs
- DataGrid.cs
- ConfigurationProperty.cs
- PathGradientBrush.cs
- DataPagerField.cs
- HiddenField.cs
- ApplicationFileCodeDomTreeGenerator.cs
- TableLayoutSettingsTypeConverter.cs
- SpeechSynthesizer.cs
- StorageModelBuildProvider.cs
- WebControl.cs
- TextEditorTyping.cs
- CapabilitiesPattern.cs
- DynamicDataRouteHandler.cs
- RealizationDrawingContextWalker.cs
- CompiledQueryCacheEntry.cs
- SingleStorage.cs
- Int16Animation.cs
- PropertyItem.cs
- ExtenderProvidedPropertyAttribute.cs