Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / ScaleTransform3D.cs / 1 / ScaleTransform3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class ScaleTransform3D : AffineTransform3D { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new ScaleTransform3D Clone() { return (ScaleTransform3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new ScaleTransform3D CloneCurrentValue() { return (ScaleTransform3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ScaleXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleXValue = (double)e.NewValue; target.PropertyChanged(ScaleXProperty); } private static void ScaleYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleYValue = (double)e.NewValue; target.PropertyChanged(ScaleYProperty); } private static void ScaleZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleZValue = (double)e.NewValue; target.PropertyChanged(ScaleZProperty); } private static void CenterXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterXValue = (double)e.NewValue; target.PropertyChanged(CenterXProperty); } private static void CenterYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterYValue = (double)e.NewValue; target.PropertyChanged(CenterYProperty); } private static void CenterZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterZValue = (double)e.NewValue; target.PropertyChanged(CenterZProperty); } #region Public Properties ////// ScaleX - double. Default value is 1.0. /// public double ScaleX { get { ReadPreamble(); return _cachedScaleXValue; } set { SetValueInternal(ScaleXProperty, value); } } ////// ScaleY - double. Default value is 1.0. /// public double ScaleY { get { ReadPreamble(); return _cachedScaleYValue; } set { SetValueInternal(ScaleYProperty, value); } } ////// ScaleZ - double. Default value is 1.0. /// public double ScaleZ { get { ReadPreamble(); return _cachedScaleZValue; } set { SetValueInternal(ScaleZProperty, value); } } ////// CenterX - double. Default value is 0.0. /// public double CenterX { get { ReadPreamble(); return _cachedCenterXValue; } set { SetValueInternal(CenterXProperty, value); } } ////// CenterY - double. Default value is 0.0. /// public double CenterY { get { ReadPreamble(); return _cachedCenterYValue; } set { SetValueInternal(CenterYProperty, value); } } ////// CenterZ - double. Default value is 0.0. /// public double CenterZ { get { ReadPreamble(); return _cachedCenterZValue; } set { SetValueInternal(CenterZProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new ScaleTransform3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hScaleXAnimations = GetAnimationResourceHandle(ScaleXProperty, channel); DUCE.ResourceHandle hScaleYAnimations = GetAnimationResourceHandle(ScaleYProperty, channel); DUCE.ResourceHandle hScaleZAnimations = GetAnimationResourceHandle(ScaleZProperty, channel); DUCE.ResourceHandle hCenterXAnimations = GetAnimationResourceHandle(CenterXProperty, channel); DUCE.ResourceHandle hCenterYAnimations = GetAnimationResourceHandle(CenterYProperty, channel); DUCE.ResourceHandle hCenterZAnimations = GetAnimationResourceHandle(CenterZProperty, channel); // Pack & send command packet DUCE.MILCMD_SCALETRANSFORM3D data; unsafe { data.Type = MILCMD.MilCmdScaleTransform3D; data.Handle = _duceResource.GetHandle(channel); if (hScaleXAnimations.IsNull) { data.scaleX = ScaleX; } data.hScaleXAnimations = hScaleXAnimations; if (hScaleYAnimations.IsNull) { data.scaleY = ScaleY; } data.hScaleYAnimations = hScaleYAnimations; if (hScaleZAnimations.IsNull) { data.scaleZ = ScaleZ; } data.hScaleZAnimations = hScaleZAnimations; if (hCenterXAnimations.IsNull) { data.centerX = CenterX; } data.hCenterXAnimations = hCenterXAnimations; if (hCenterYAnimations.IsNull) { data.centerY = CenterY; } data.hCenterYAnimations = hCenterYAnimations; if (hCenterZAnimations.IsNull) { data.centerZ = CenterZ; } data.hCenterZAnimations = hCenterZAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SCALETRANSFORM3D)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SCALETRANSFORM3D)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the ScaleTransform3D.ScaleX property. /// public static readonly DependencyProperty ScaleXProperty; ////// The DependencyProperty for the ScaleTransform3D.ScaleY property. /// public static readonly DependencyProperty ScaleYProperty; ////// The DependencyProperty for the ScaleTransform3D.ScaleZ property. /// public static readonly DependencyProperty ScaleZProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterX property. /// public static readonly DependencyProperty CenterXProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterY property. /// public static readonly DependencyProperty CenterYProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterZ property. /// public static readonly DependencyProperty CenterZProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields private double _cachedScaleXValue = 1.0; private double _cachedScaleYValue = 1.0; private double _cachedScaleZValue = 1.0; private double _cachedCenterXValue = 0.0; private double _cachedCenterYValue = 0.0; private double _cachedCenterZValue = 0.0; internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_ScaleX = 1.0; internal const double c_ScaleY = 1.0; internal const double c_ScaleZ = 1.0; internal const double c_CenterX = 0.0; internal const double c_CenterY = 0.0; internal const double c_CenterZ = 0.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static ScaleTransform3D() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(ScaleTransform3D); ScaleXProperty = RegisterProperty("ScaleX", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ScaleYProperty = RegisterProperty("ScaleY", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ScaleZProperty = RegisterProperty("ScaleZ", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterXProperty = RegisterProperty("CenterX", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterYProperty = RegisterProperty("CenterY", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterZProperty = RegisterProperty("CenterZ", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class ScaleTransform3D : AffineTransform3D { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new ScaleTransform3D Clone() { return (ScaleTransform3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new ScaleTransform3D CloneCurrentValue() { return (ScaleTransform3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ScaleXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleXValue = (double)e.NewValue; target.PropertyChanged(ScaleXProperty); } private static void ScaleYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleYValue = (double)e.NewValue; target.PropertyChanged(ScaleYProperty); } private static void ScaleZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleZValue = (double)e.NewValue; target.PropertyChanged(ScaleZProperty); } private static void CenterXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterXValue = (double)e.NewValue; target.PropertyChanged(CenterXProperty); } private static void CenterYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterYValue = (double)e.NewValue; target.PropertyChanged(CenterYProperty); } private static void CenterZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterZValue = (double)e.NewValue; target.PropertyChanged(CenterZProperty); } #region Public Properties ////// ScaleX - double. Default value is 1.0. /// public double ScaleX { get { ReadPreamble(); return _cachedScaleXValue; } set { SetValueInternal(ScaleXProperty, value); } } ////// ScaleY - double. Default value is 1.0. /// public double ScaleY { get { ReadPreamble(); return _cachedScaleYValue; } set { SetValueInternal(ScaleYProperty, value); } } ////// ScaleZ - double. Default value is 1.0. /// public double ScaleZ { get { ReadPreamble(); return _cachedScaleZValue; } set { SetValueInternal(ScaleZProperty, value); } } ////// CenterX - double. Default value is 0.0. /// public double CenterX { get { ReadPreamble(); return _cachedCenterXValue; } set { SetValueInternal(CenterXProperty, value); } } ////// CenterY - double. Default value is 0.0. /// public double CenterY { get { ReadPreamble(); return _cachedCenterYValue; } set { SetValueInternal(CenterYProperty, value); } } ////// CenterZ - double. Default value is 0.0. /// public double CenterZ { get { ReadPreamble(); return _cachedCenterZValue; } set { SetValueInternal(CenterZProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new ScaleTransform3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hScaleXAnimations = GetAnimationResourceHandle(ScaleXProperty, channel); DUCE.ResourceHandle hScaleYAnimations = GetAnimationResourceHandle(ScaleYProperty, channel); DUCE.ResourceHandle hScaleZAnimations = GetAnimationResourceHandle(ScaleZProperty, channel); DUCE.ResourceHandle hCenterXAnimations = GetAnimationResourceHandle(CenterXProperty, channel); DUCE.ResourceHandle hCenterYAnimations = GetAnimationResourceHandle(CenterYProperty, channel); DUCE.ResourceHandle hCenterZAnimations = GetAnimationResourceHandle(CenterZProperty, channel); // Pack & send command packet DUCE.MILCMD_SCALETRANSFORM3D data; unsafe { data.Type = MILCMD.MilCmdScaleTransform3D; data.Handle = _duceResource.GetHandle(channel); if (hScaleXAnimations.IsNull) { data.scaleX = ScaleX; } data.hScaleXAnimations = hScaleXAnimations; if (hScaleYAnimations.IsNull) { data.scaleY = ScaleY; } data.hScaleYAnimations = hScaleYAnimations; if (hScaleZAnimations.IsNull) { data.scaleZ = ScaleZ; } data.hScaleZAnimations = hScaleZAnimations; if (hCenterXAnimations.IsNull) { data.centerX = CenterX; } data.hCenterXAnimations = hCenterXAnimations; if (hCenterYAnimations.IsNull) { data.centerY = CenterY; } data.hCenterYAnimations = hCenterYAnimations; if (hCenterZAnimations.IsNull) { data.centerZ = CenterZ; } data.hCenterZAnimations = hCenterZAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SCALETRANSFORM3D)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SCALETRANSFORM3D)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the ScaleTransform3D.ScaleX property. /// public static readonly DependencyProperty ScaleXProperty; ////// The DependencyProperty for the ScaleTransform3D.ScaleY property. /// public static readonly DependencyProperty ScaleYProperty; ////// The DependencyProperty for the ScaleTransform3D.ScaleZ property. /// public static readonly DependencyProperty ScaleZProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterX property. /// public static readonly DependencyProperty CenterXProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterY property. /// public static readonly DependencyProperty CenterYProperty; ////// The DependencyProperty for the ScaleTransform3D.CenterZ property. /// public static readonly DependencyProperty CenterZProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields private double _cachedScaleXValue = 1.0; private double _cachedScaleYValue = 1.0; private double _cachedScaleZValue = 1.0; private double _cachedCenterXValue = 0.0; private double _cachedCenterYValue = 0.0; private double _cachedCenterZValue = 0.0; internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_ScaleX = 1.0; internal const double c_ScaleY = 1.0; internal const double c_ScaleZ = 1.0; internal const double c_CenterX = 0.0; internal const double c_CenterY = 0.0; internal const double c_CenterZ = 0.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static ScaleTransform3D() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(ScaleTransform3D); ScaleXProperty = RegisterProperty("ScaleX", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ScaleYProperty = RegisterProperty("ScaleY", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ScaleZProperty = RegisterProperty("ScaleZ", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(ScaleZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterXProperty = RegisterProperty("CenterX", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterYProperty = RegisterProperty("CenterY", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); CenterZProperty = RegisterProperty("CenterZ", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(CenterZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
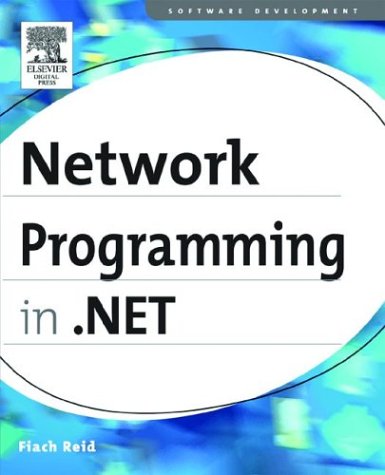
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionStringEditor.cs
- Reference.cs
- CalendarDay.cs
- ContentPropertyAttribute.cs
- documentsequencetextview.cs
- ConstantCheck.cs
- SchemaTypeEmitter.cs
- AudioBase.cs
- ImageMap.cs
- Region.cs
- SignatureToken.cs
- ActivityDesignerAccessibleObject.cs
- SuppressMessageAttribute.cs
- ScrollProviderWrapper.cs
- LicFileLicenseProvider.cs
- PostBackTrigger.cs
- Oid.cs
- ObjectHandle.cs
- ObjectView.cs
- ScrollProperties.cs
- ItemsPanelTemplate.cs
- ButtonPopupAdapter.cs
- _UncName.cs
- ResXResourceReader.cs
- OpCodes.cs
- ConfigurationPermission.cs
- RoleExceptions.cs
- SortableBindingList.cs
- _ShellExpression.cs
- TimelineClockCollection.cs
- Dump.cs
- SBCSCodePageEncoding.cs
- ZipIOExtraFieldElement.cs
- Oid.cs
- DynamicArgumentDialog.cs
- WebPartTracker.cs
- Renderer.cs
- SqlStatistics.cs
- QilReplaceVisitor.cs
- StandardOleMarshalObject.cs
- DesignTable.cs
- _DomainName.cs
- EnumConverter.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- FontFamilyValueSerializer.cs
- Table.cs
- IImplicitResourceProvider.cs
- ContextMenuService.cs
- Regex.cs
- ArcSegment.cs
- DesignerRegionCollection.cs
- RuleInfoComparer.cs
- DetailsViewUpdateEventArgs.cs
- SoapInteropTypes.cs
- ToolStripGrip.cs
- PrivilegeNotHeldException.cs
- ByteAnimationUsingKeyFrames.cs
- InstancePersistenceEvent.cs
- RadioButton.cs
- PerformanceCounterLib.cs
- AnnouncementEventArgs.cs
- ProxyWebPartManager.cs
- ChannelManagerHelpers.cs
- ObjectDataSourceView.cs
- DTCTransactionManager.cs
- PersonalizationState.cs
- GraphicsContext.cs
- SynchronizationScope.cs
- RichTextBox.cs
- Calendar.cs
- TCPClient.cs
- WebConfigurationFileMap.cs
- TextEffectResolver.cs
- Image.cs
- CmsInterop.cs
- ElementMarkupObject.cs
- PropertyChangedEventManager.cs
- ToolStripItem.cs
- GrowingArray.cs
- DESCryptoServiceProvider.cs
- CategoryEditor.cs
- CaseInsensitiveComparer.cs
- ListItemCollection.cs
- FormView.cs
- SymbolType.cs
- SqlDataSourceQueryEditor.cs
- CorrelationService.cs
- dtdvalidator.cs
- RenderOptions.cs
- DetailsViewDeleteEventArgs.cs
- StringFunctions.cs
- EFTableProvider.cs
- VisualStyleInformation.cs
- WindowsStreamSecurityElement.cs
- ReadOnlyCollection.cs
- BamlStream.cs
- IdentityModelDictionary.cs
- TimerElapsedEvenArgs.cs
- HtmlCalendarAdapter.cs
- ColorConvertedBitmap.cs