Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Security / permissions / WebBrowserPermission.cs / 1305600 / WebBrowserPermission.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The WebBrowserPermission controls the ability to create the WebBrowsercontrol. // In avalon - this control creates the ability for frames to navigate to html. // // // History: // 05/18/05: marka Created. //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.IO; using System.Runtime.Serialization; using System.Collections; using System.Globalization; using System.Diagnostics.CodeAnalysis; using System.Windows; using MS.Internal.WindowsBase; namespace System.Security.Permissions { ////// Enum of permission levels. /// public enum WebBrowserPermissionLevel { ////// WebBrowser not allowed /// None, ////// Safe. Can create webbrowser with some restrictions. /// Safe, ////// Unrestricted. Can create webbrowser with no restrictions. /// Unrestricted } ////// The WebBrowserPermission controls the ability to create the WebBrowsercontrol. /// In avalon - this permission grants the ability for frames to navigate to html. /// The levels for this permission are : /// None - not able to navigate frames to HTML. /// Safe - able to navigate frames to HTML safely. This means that /// there are several mitigations in effect. Namely. /// Popup mitigation - unable to place an avalon popup over the weboc. /// SiteLock - the WebOC can only be navigated to site of origin. /// Url-Action-lockdown - the security settings of the weboc are reduced. /// Unrestricted - able to navigate the weboc with no restrictions. /// [Serializable()] sealed public class WebBrowserPermission : CodeAccessPermission, IUnrestrictedPermission { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// WebBrowserPermission ctor. /// public WebBrowserPermission() { _webBrowserPermissionLevel = WebBrowserPermissionLevel.Safe; } ////// WebBrowserPermission ctor. /// public WebBrowserPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { _webBrowserPermissionLevel = WebBrowserPermissionLevel.Unrestricted; } else if (state == PermissionState.None) { _webBrowserPermissionLevel = WebBrowserPermissionLevel.None; } else { throw new ArgumentException( SR.Get(SRID.InvalidPermissionState) ); } } ////// WebBrowserPermission ctor. /// public WebBrowserPermission(WebBrowserPermissionLevel webBrowserPermissionLevel) { WebBrowserPermission.VerifyWebBrowserPermissionLevel(webBrowserPermissionLevel); this._webBrowserPermissionLevel = webBrowserPermissionLevel; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods // // IUnrestrictedPermission implementation // ////// Is this an unrestricted permisison ? /// public bool IsUnrestricted() { return _webBrowserPermissionLevel == WebBrowserPermissionLevel.Unrestricted ; } // // CodeAccessPermission implementation // ////// Is this a subsetOf the target ? /// public override bool IsSubsetOf(IPermission target) { if (target == null) { return _webBrowserPermissionLevel == WebBrowserPermissionLevel.None; } WebBrowserPermission operand = target as WebBrowserPermission ; if ( operand != null ) { return this._webBrowserPermissionLevel <= operand._webBrowserPermissionLevel; } else { throw new ArgumentException(SR.Get(SRID.TargetNotWebBrowserPermissionLevel)); } } ////// Return the intersection with the target /// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } WebBrowserPermission operand = target as WebBrowserPermission ; if ( operand != null ) { WebBrowserPermissionLevel intersectLevel = _webBrowserPermissionLevel < operand._webBrowserPermissionLevel ? _webBrowserPermissionLevel : operand._webBrowserPermissionLevel; if (intersectLevel == WebBrowserPermissionLevel.None) { return null; } else { return new WebBrowserPermission(intersectLevel); } } else { throw new ArgumentException(SR.Get(SRID.TargetNotWebBrowserPermissionLevel)); } } ////// Return the Union with the target /// public override IPermission Union(IPermission target) { if (target == null) { return this.Copy(); } WebBrowserPermission operand = target as WebBrowserPermission ; if ( operand != null ) { WebBrowserPermissionLevel unionLevel = _webBrowserPermissionLevel > operand._webBrowserPermissionLevel ? _webBrowserPermissionLevel : operand._webBrowserPermissionLevel; if (unionLevel == WebBrowserPermissionLevel.None) { return null; } else { return new WebBrowserPermission(unionLevel); } } else { throw new ArgumentException(SR.Get(SRID.TargetNotWebBrowserPermissionLevel)); } } ////// Copy this permission. /// public override IPermission Copy() { return new WebBrowserPermission(this._webBrowserPermissionLevel); } ////// Return an XML instantiation of this permisison. /// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().AssemblyQualifiedName); securityElement.AddAttribute("version", "1"); if (IsUnrestricted()) { securityElement.AddAttribute("Unrestricted", Boolean.TrueString); } else { securityElement.AddAttribute("Level", _webBrowserPermissionLevel.ToString()); } return securityElement; } ////// Create a permission from XML /// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { throw new ArgumentNullException("securityElement"); } String className = securityElement.Attribute("class"); if (className == null || className.IndexOf(this.GetType().FullName, StringComparison.Ordinal) == -1) { throw new ArgumentNullException("securityElement"); } String unrestricted = securityElement.Attribute("Unrestricted"); if (unrestricted != null && Boolean.Parse(unrestricted)) { _webBrowserPermissionLevel = WebBrowserPermissionLevel.Unrestricted; return; } this._webBrowserPermissionLevel = WebBrowserPermissionLevel.None; String level = securityElement.Attribute("Level"); if (level != null) { _webBrowserPermissionLevel = (WebBrowserPermissionLevel)Enum.Parse(typeof(WebBrowserPermissionLevel), level); } else { throw new ArgumentException(SR.Get(SRID.BadXml,"level")); // bad XML } } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Current permission level. /// public WebBrowserPermissionLevel Level { get { return _webBrowserPermissionLevel; } set { WebBrowserPermission.VerifyWebBrowserPermissionLevel(value); _webBrowserPermissionLevel = value; } } #endregion Public Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods internal static void VerifyWebBrowserPermissionLevel(WebBrowserPermissionLevel level) { if (level < WebBrowserPermissionLevel.None || level > WebBrowserPermissionLevel.Unrestricted ) { throw new ArgumentException(SR.Get(SRID.InvalidPermissionLevel)); } } #endregion Private Methods // // Private fields: // private WebBrowserPermissionLevel _webBrowserPermissionLevel; } ////// Imperative attribute to create a WebBrowserPermission. /// [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] sealed public class WebBrowserPermissionAttribute : CodeAccessSecurityAttribute { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Imperative attribute to create a WebBrowserPermission. /// public WebBrowserPermissionAttribute(SecurityAction action) : base(action) { } #endregion Constructors //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Create a WebBrowserPermisison. /// public override IPermission CreatePermission() { if (Unrestricted) { return new WebBrowserPermission(PermissionState.Unrestricted); } else { return new WebBrowserPermission(_webBrowserPermissionLevel); } } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Current permission level. /// public WebBrowserPermissionLevel Level { get { return _webBrowserPermissionLevel; } set { WebBrowserPermission.VerifyWebBrowserPermissionLevel(value); _webBrowserPermissionLevel = value; } } #endregion Public Properties // // Private fields: // private WebBrowserPermissionLevel _webBrowserPermissionLevel; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
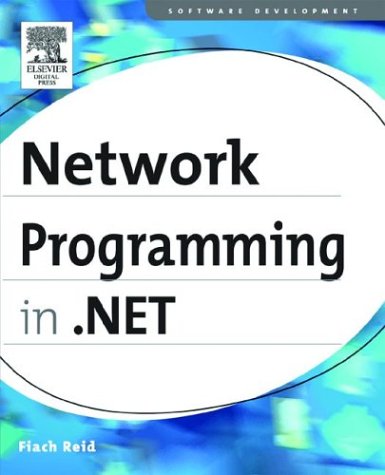
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataExporter.cs
- ResourceContainer.cs
- TextContainerChangedEventArgs.cs
- ConnectionModeReader.cs
- LinkedResourceCollection.cs
- DetailsViewDeleteEventArgs.cs
- ReadingWritingEntityEventArgs.cs
- CellNormalizer.cs
- Brushes.cs
- PageRanges.cs
- ComponentDispatcherThread.cs
- RandomNumberGenerator.cs
- StyleSheetComponentEditor.cs
- ChangePasswordDesigner.cs
- BaseHashHelper.cs
- TableLayoutStyleCollection.cs
- WindowsSlider.cs
- FontStyles.cs
- InternalControlCollection.cs
- OutputCacheSettingsSection.cs
- Visual3D.cs
- NegotiationTokenAuthenticatorStateCache.cs
- UndoManager.cs
- RegexMatchCollection.cs
- RemoteX509AsymmetricSecurityKey.cs
- TextEditorMouse.cs
- UrlMappingsSection.cs
- HwndHost.cs
- XmlSchemaType.cs
- DrawingDrawingContext.cs
- ProgressBar.cs
- DataGridViewCellCollection.cs
- ReferenceEqualityComparer.cs
- TextEditorCharacters.cs
- MessagePropertyAttribute.cs
- EntityModelBuildProvider.cs
- X509SecurityTokenAuthenticator.cs
- _BaseOverlappedAsyncResult.cs
- FormViewCommandEventArgs.cs
- BindingsSection.cs
- BmpBitmapEncoder.cs
- DataReceivedEventArgs.cs
- PartialTrustHelpers.cs
- FormatStringEditor.cs
- JavaScriptSerializer.cs
- ConstNode.cs
- ObjectParameter.cs
- IODescriptionAttribute.cs
- ContentValidator.cs
- FieldCollectionEditor.cs
- Vector3DIndependentAnimationStorage.cs
- DynamicRendererThreadManager.cs
- SiteMembershipCondition.cs
- XmlTypeAttribute.cs
- DataGridViewTextBoxCell.cs
- DetailsViewCommandEventArgs.cs
- FormViewUpdateEventArgs.cs
- ExtendedPropertyDescriptor.cs
- ExceptionList.cs
- CompensatableTransactionScopeActivity.cs
- Splitter.cs
- RuntimeHelpers.cs
- AdornerDecorator.cs
- SQLResource.cs
- FastPropertyAccessor.cs
- SamlSecurityToken.cs
- BlockCollection.cs
- ListMarkerSourceInfo.cs
- FieldBuilder.cs
- SqlSelectStatement.cs
- FormClosedEvent.cs
- DbDeleteCommandTree.cs
- MergePropertyDescriptor.cs
- ToolBarButtonClickEvent.cs
- GuidConverter.cs
- Char.cs
- InlineCategoriesDocument.cs
- COM2PropertyPageUITypeConverter.cs
- FormView.cs
- CrossSiteScriptingValidation.cs
- BamlBinaryWriter.cs
- FormViewDeleteEventArgs.cs
- SqlBinder.cs
- MsmqMessage.cs
- LocatorPartList.cs
- MexNamedPipeBindingCollectionElement.cs
- Knowncolors.cs
- HttpDictionary.cs
- PropertyInformation.cs
- EventLogger.cs
- SHA1Managed.cs
- ParameterCollection.cs
- FormConverter.cs
- DropDownList.cs
- SurrogateDataContract.cs
- ProcessModule.cs
- PartitionerStatic.cs
- PerfCounters.cs
- RadioButtonDesigner.cs
- EntityPropertyMappingAttribute.cs