Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / AspNet / EntityModelBuildProvider.cs / 2 / EntityModelBuildProvider.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.CodeDom; using System.CodeDom.Compiler; using System.Globalization; using System.IO; using System.Web; using System.Web.Hosting; using System.Web.Compilation; using System.Xml; using System.Data.Entity.Design; using System.Data.Metadata.Edm; namespace System.Data.Entity.Design.AspNet { ////// The ASP .NET Build provider for the CSDL in ADO .NET /// /// [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] public class EntityModelBuildProvider : System.Web.Compilation.BuildProvider { ////// Default constructor /// public EntityModelBuildProvider() { } ////// We want ASP .NET to always reset the app domain when we have to rebuild /// /// ///public override BuildProviderResultFlags GetResultFlags(CompilerResults results) { return BuildProviderResultFlags.ShutdownAppDomainOnChange; } /// /// /// /// public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // look at the assembly builder to see which language we should use in the App_Code directory EntityClassGenerator generator = null; if (assemblyBuilder.CodeDomProvider.FileExtension.ToLowerInvariant() == "cs") { generator = new EntityClassGenerator(LanguageOption.GenerateCSharpCode); } else { generator = new EntityClassGenerator(LanguageOption.GenerateVBCode); } // generate the code for our CSDL file IListerrors = null; using (XmlReader input = XmlReader.Create(VirtualPathProvider.OpenFile(base.VirtualPath))) { using (StringWriter output = new StringWriter(CultureInfo.InvariantCulture)) { // Read from input and generate into output, put errors in a class member errors = generator.GenerateCode(input, output); if (errors.Count == 0) { output.Flush(); assemblyBuilder.AddCodeCompileUnit(this, new CodeSnippetCompileUnit(output.ToString())); } } } // if there are errors, package this data into XmlExceptions and throw this // if we are in VS, the ASP .NET stack will place this information in the error pane // if we are in the ASP .NET runtime, it will use this information to build the error page if (errors != null && errors.Count > 0) { XmlException inner = null; XmlException outer = null; foreach (EdmSchemaError error in errors) { outer = new XmlException(error.Message, inner, error.Line, error.Column); inner = outer; } throw outer; } BuildProviderUtils.AddArtifactReference(assemblyBuilder, this, base.VirtualPath); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.CodeDom; using System.CodeDom.Compiler; using System.Globalization; using System.IO; using System.Web; using System.Web.Hosting; using System.Web.Compilation; using System.Xml; using System.Data.Entity.Design; using System.Data.Metadata.Edm; namespace System.Data.Entity.Design.AspNet { ////// The ASP .NET Build provider for the CSDL in ADO .NET /// /// [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] public class EntityModelBuildProvider : System.Web.Compilation.BuildProvider { ////// Default constructor /// public EntityModelBuildProvider() { } ////// We want ASP .NET to always reset the app domain when we have to rebuild /// /// ///public override BuildProviderResultFlags GetResultFlags(CompilerResults results) { return BuildProviderResultFlags.ShutdownAppDomainOnChange; } /// /// /// /// public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // look at the assembly builder to see which language we should use in the App_Code directory EntityClassGenerator generator = null; if (assemblyBuilder.CodeDomProvider.FileExtension.ToLowerInvariant() == "cs") { generator = new EntityClassGenerator(LanguageOption.GenerateCSharpCode); } else { generator = new EntityClassGenerator(LanguageOption.GenerateVBCode); } // generate the code for our CSDL file IListerrors = null; using (XmlReader input = XmlReader.Create(VirtualPathProvider.OpenFile(base.VirtualPath))) { using (StringWriter output = new StringWriter(CultureInfo.InvariantCulture)) { // Read from input and generate into output, put errors in a class member errors = generator.GenerateCode(input, output); if (errors.Count == 0) { output.Flush(); assemblyBuilder.AddCodeCompileUnit(this, new CodeSnippetCompileUnit(output.ToString())); } } } // if there are errors, package this data into XmlExceptions and throw this // if we are in VS, the ASP .NET stack will place this information in the error pane // if we are in the ASP .NET runtime, it will use this information to build the error page if (errors != null && errors.Count > 0) { XmlException inner = null; XmlException outer = null; foreach (EdmSchemaError error in errors) { outer = new XmlException(error.Message, inner, error.Line, error.Column); inner = outer; } throw outer; } BuildProviderUtils.AddArtifactReference(assemblyBuilder, this, base.VirtualPath); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
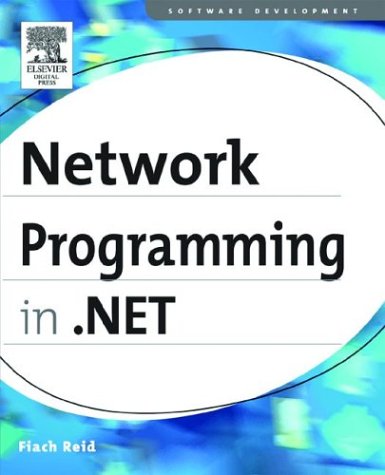
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingMemoryStreamFactory.cs
- EntityDataSourceEntitySetNameItem.cs
- BindingsCollection.cs
- Models.cs
- TimeIntervalCollection.cs
- DataView.cs
- ToolStripDropDown.cs
- ResXBuildProvider.cs
- RightsManagementManager.cs
- PriorityItem.cs
- LoadGrammarCompletedEventArgs.cs
- EventToken.cs
- WebPartConnectVerb.cs
- TitleStyle.cs
- EntityConnection.cs
- XmlReflectionImporter.cs
- ApplicationGesture.cs
- BitVec.cs
- Size3D.cs
- PeerApplication.cs
- ReversePositionQuery.cs
- _SslSessionsCache.cs
- Attributes.cs
- ZoneButton.cs
- LinqDataSourceInsertEventArgs.cs
- TreeBuilder.cs
- HttpHandlerActionCollection.cs
- ExtenderProvidedPropertyAttribute.cs
- XmlUtil.cs
- UriExt.cs
- HybridDictionary.cs
- BitmapEffectCollection.cs
- FixedSOMContainer.cs
- LexicalChunk.cs
- CommonDialog.cs
- DependencyPropertyChangedEventArgs.cs
- WindowClosedEventArgs.cs
- UnauthorizedAccessException.cs
- CalendarAutomationPeer.cs
- ModelTreeEnumerator.cs
- CompoundFileIOPermission.cs
- DataSourceXmlSubItemAttribute.cs
- BindingContext.cs
- LazyTextWriterCreator.cs
- ShellProvider.cs
- Win32SafeHandles.cs
- DocumentGridContextMenu.cs
- MeasurementDCInfo.cs
- WebServiceFaultDesigner.cs
- XamlInterfaces.cs
- ThreadExceptionEvent.cs
- BamlLocalizableResourceKey.cs
- SizeConverter.cs
- GridPattern.cs
- Misc.cs
- BaseTreeIterator.cs
- Permission.cs
- WebPartDescription.cs
- RawStylusSystemGestureInputReport.cs
- XmlNamedNodeMap.cs
- RadioButtonDesigner.cs
- FormsAuthenticationModule.cs
- ParserHooks.cs
- UniqueEventHelper.cs
- EntityConnectionStringBuilderItem.cs
- BindingsCollection.cs
- OutputChannel.cs
- RectAnimationClockResource.cs
- ControlAdapter.cs
- ProviderManager.cs
- DrawingContextDrawingContextWalker.cs
- DescriptionCreator.cs
- Pkcs7Signer.cs
- CompositeCollectionView.cs
- SqlClientWrapperSmiStream.cs
- TrustDriver.cs
- ConfigurationHelpers.cs
- TypedAsyncResult.cs
- WebPartCollection.cs
- AssemblyAttributesGoHere.cs
- DeadCharTextComposition.cs
- RefreshResponseInfo.cs
- XmlLanguage.cs
- PeerTransportSecuritySettings.cs
- ScriptingScriptResourceHandlerSection.cs
- wgx_sdk_version.cs
- SizeConverter.cs
- NetworkCredential.cs
- BaseDataList.cs
- EntityDesignerDataSourceView.cs
- ContentPropertyAttribute.cs
- EpmSourceTree.cs
- UnsupportedPolicyOptionsException.cs
- Pick.cs
- RuntimeHandles.cs
- ChannelAcceptor.cs
- BrowserCapabilitiesFactoryBase.cs
- WindowsListViewGroup.cs
- WizardStepBase.cs
- HelloMessageCD1.cs