Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Configuration / ScriptingScriptResourceHandlerSection.cs / 1 / ScriptingScriptResourceHandlerSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Security.Permissions; using System.Web; using System.Web.Configuration; using System.Web.Script.Serialization; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] public sealed class ScriptingScriptResourceHandlerSection : ConfigurationSection { private static readonly ConfigurationProperty _propEnableCaching = new ConfigurationProperty("enableCaching", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableCompression = new ConfigurationProperty("enableCompression", typeof(bool), true, ConfigurationPropertyOptions.None); private static ConfigurationPropertyCollection _properties = BuildProperties(); private static ConfigurationPropertyCollection BuildProperties() { ConfigurationPropertyCollection props = new ConfigurationPropertyCollection(); props.Add(_propEnableCaching); props.Add(_propEnableCompression); return props; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enableCaching", DefaultValue = true)] public bool EnableCaching { get { return (bool)base[_propEnableCaching]; } set { base[_propEnableCaching] = value; } } [ConfigurationProperty("enableCompression", DefaultValue = true)] public bool EnableCompression { get { return (bool)base[_propEnableCompression]; } set { base[_propEnableCompression] = value; } } internal static class ApplicationSettings { private volatile static bool s_sectionLoaded; private static bool s_enableCaching; private static bool s_enableCompression; private static void EnsureSectionLoaded() { if (!s_sectionLoaded) { ScriptingScriptResourceHandlerSection section = (ScriptingScriptResourceHandlerSection) WebConfigurationManager.GetWebApplicationSection("system.web.extensions/scripting/scriptResourceHandler"); if (section != null) { s_enableCaching = section.EnableCaching; s_enableCompression = section.EnableCompression; } else { s_enableCaching = (bool)_propEnableCaching.DefaultValue; s_enableCompression = (bool)_propEnableCompression.DefaultValue; } s_sectionLoaded = true; } } internal static bool EnableCaching { get { EnsureSectionLoaded(); return s_enableCaching; } } internal static bool EnableCompression { get { EnsureSectionLoaded(); return s_enableCompression; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Security.Permissions; using System.Web; using System.Web.Configuration; using System.Web.Script.Serialization; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] public sealed class ScriptingScriptResourceHandlerSection : ConfigurationSection { private static readonly ConfigurationProperty _propEnableCaching = new ConfigurationProperty("enableCaching", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableCompression = new ConfigurationProperty("enableCompression", typeof(bool), true, ConfigurationPropertyOptions.None); private static ConfigurationPropertyCollection _properties = BuildProperties(); private static ConfigurationPropertyCollection BuildProperties() { ConfigurationPropertyCollection props = new ConfigurationPropertyCollection(); props.Add(_propEnableCaching); props.Add(_propEnableCompression); return props; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enableCaching", DefaultValue = true)] public bool EnableCaching { get { return (bool)base[_propEnableCaching]; } set { base[_propEnableCaching] = value; } } [ConfigurationProperty("enableCompression", DefaultValue = true)] public bool EnableCompression { get { return (bool)base[_propEnableCompression]; } set { base[_propEnableCompression] = value; } } internal static class ApplicationSettings { private volatile static bool s_sectionLoaded; private static bool s_enableCaching; private static bool s_enableCompression; private static void EnsureSectionLoaded() { if (!s_sectionLoaded) { ScriptingScriptResourceHandlerSection section = (ScriptingScriptResourceHandlerSection) WebConfigurationManager.GetWebApplicationSection("system.web.extensions/scripting/scriptResourceHandler"); if (section != null) { s_enableCaching = section.EnableCaching; s_enableCompression = section.EnableCompression; } else { s_enableCaching = (bool)_propEnableCaching.DefaultValue; s_enableCompression = (bool)_propEnableCompression.DefaultValue; } s_sectionLoaded = true; } } internal static bool EnableCaching { get { EnsureSectionLoaded(); return s_enableCaching; } } internal static bool EnableCompression { get { EnsureSectionLoaded(); return s_enableCompression; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
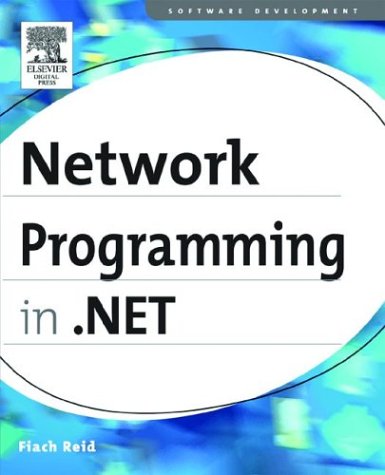
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HwndSourceParameters.cs
- RuntimeResourceSet.cs
- Random.cs
- XmlSchemaImport.cs
- ImageUrlEditor.cs
- StylusPlugInCollection.cs
- ConfigurationElementProperty.cs
- listviewsubitemcollectioneditor.cs
- GPPOINT.cs
- AssemblyCache.cs
- CompModSwitches.cs
- SafeCertificateContext.cs
- DesignBinding.cs
- MailWebEventProvider.cs
- List.cs
- ControlDesigner.cs
- XmlQuerySequence.cs
- ValueUnavailableException.cs
- XomlCompiler.cs
- Visual3D.cs
- TransformedBitmap.cs
- DSASignatureDeformatter.cs
- ADMembershipUser.cs
- FixedSOMPageConstructor.cs
- MemberRelationshipService.cs
- TypeLibConverter.cs
- RenderDataDrawingContext.cs
- FontStyle.cs
- ReferentialConstraint.cs
- ReferencedCollectionType.cs
- BamlReader.cs
- StandardBindingCollectionElement.cs
- TypeSystem.cs
- CLSCompliantAttribute.cs
- Message.cs
- EffectiveValueEntry.cs
- Internal.cs
- XmlQualifiedNameTest.cs
- TypefaceCollection.cs
- ProcessModule.cs
- TextAnchor.cs
- EntityDataSourceUtil.cs
- LicenseException.cs
- OdbcUtils.cs
- ReadOnlyHierarchicalDataSourceView.cs
- _LocalDataStoreMgr.cs
- MouseButton.cs
- Select.cs
- LogExtentCollection.cs
- ExtensibleClassFactory.cs
- QuestionEventArgs.cs
- DataServiceQueryException.cs
- ReflectionUtil.cs
- WebPartTracker.cs
- SoapMessage.cs
- smtppermission.cs
- ChannelPoolSettingsElement.cs
- RegexParser.cs
- DashStyle.cs
- PreviewControlDesigner.cs
- Assert.cs
- ISFClipboardData.cs
- CacheEntry.cs
- AutomationElementCollection.cs
- Process.cs
- TrackingRecord.cs
- UrlAuthFailedErrorFormatter.cs
- XmlIlVisitor.cs
- EdmFunctions.cs
- _AutoWebProxyScriptEngine.cs
- XmlSchemaSimpleTypeUnion.cs
- VirtualPathUtility.cs
- PropertyReference.cs
- ComplexBindingPropertiesAttribute.cs
- XmlSchemaExternal.cs
- DataTableTypeConverter.cs
- MasterPage.cs
- SqlPersonalizationProvider.cs
- BinaryObjectInfo.cs
- DialogBaseForm.cs
- WebPartManagerInternals.cs
- SessionParameter.cs
- WindowsSlider.cs
- BulletedListEventArgs.cs
- SectionUpdates.cs
- UserNameSecurityToken.cs
- FormsAuthenticationUser.cs
- PtsPage.cs
- GridViewColumnCollection.cs
- PointValueSerializer.cs
- WebConfigurationManager.cs
- MarshalDirectiveException.cs
- ReflectPropertyDescriptor.cs
- StyleCollection.cs
- ArgumentNullException.cs
- WmfPlaceableFileHeader.cs
- CharUnicodeInfo.cs
- PeerNameResolver.cs
- ControlAdapter.cs
- FixedSOMTable.cs