Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / WmfPlaceableFileHeader.cs / 1 / WmfPlaceableFileHeader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Diagnostics; using System; using System.Drawing; using System.Runtime.InteropServices; ////// /// Defines an Placeable Metafile. /// [StructLayout(LayoutKind.Sequential)] public sealed class WmfPlaceableFileHeader { int key = unchecked((int)0x9aC6CDD7); short hmf; short bboxLeft; short bboxTop; short bboxRight; short bboxBottom; short inch; int reserved; short checksum; ////// /// Indicates the presence of a placeable /// metafile header. /// public int Key { get { return key; } set { key = value; } } ////// /// Stores the handle of the metafile in /// memory. /// public short Hmf { get { return hmf; } set { hmf = value; } } ////// /// The x-coordinate of the upper-left corner /// of the bounding rectangle of the metafile image on the output device. /// public short BboxLeft { get { return bboxLeft; } set { bboxLeft = value; } } ////// /// public short BboxTop { get { return bboxTop; } set { bboxTop = value; } } ////// The y-coordinate of the upper-left corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// public short BboxRight { get { return bboxRight; } set { bboxRight = value; } } ////// The x-coordinate of the lower-right corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// public short BboxBottom { get { return bboxBottom; } set { bboxBottom = value; } } ////// The y-coordinate of the lower-right corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// Indicates the number of twips per inch. /// public short Inch { get { return inch; } set { inch = value; } } ////// /// Reserved. Do not use. /// public int Reserved { get { return reserved; } set { reserved = value; } } ////// /// public short Checksum { get { return checksum; } set { checksum = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Indicates the checksum value for the /// previous ten WORDs in the header. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Diagnostics; using System; using System.Drawing; using System.Runtime.InteropServices; ////// /// Defines an Placeable Metafile. /// [StructLayout(LayoutKind.Sequential)] public sealed class WmfPlaceableFileHeader { int key = unchecked((int)0x9aC6CDD7); short hmf; short bboxLeft; short bboxTop; short bboxRight; short bboxBottom; short inch; int reserved; short checksum; ////// /// Indicates the presence of a placeable /// metafile header. /// public int Key { get { return key; } set { key = value; } } ////// /// Stores the handle of the metafile in /// memory. /// public short Hmf { get { return hmf; } set { hmf = value; } } ////// /// The x-coordinate of the upper-left corner /// of the bounding rectangle of the metafile image on the output device. /// public short BboxLeft { get { return bboxLeft; } set { bboxLeft = value; } } ////// /// public short BboxTop { get { return bboxTop; } set { bboxTop = value; } } ////// The y-coordinate of the upper-left corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// public short BboxRight { get { return bboxRight; } set { bboxRight = value; } } ////// The x-coordinate of the lower-right corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// public short BboxBottom { get { return bboxBottom; } set { bboxBottom = value; } } ////// The y-coordinate of the lower-right corner of the bounding rectangle of the /// metafile image on the output device. /// ////// /// Indicates the number of twips per inch. /// public short Inch { get { return inch; } set { inch = value; } } ////// /// Reserved. Do not use. /// public int Reserved { get { return reserved; } set { reserved = value; } } ////// /// public short Checksum { get { return checksum; } set { checksum = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Indicates the checksum value for the /// previous ten WORDs in the header. /// ///
Link Menu
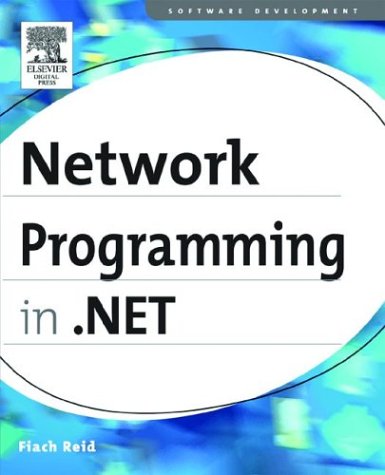
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlCaseSimplifier.cs
- SkewTransform.cs
- DecoderReplacementFallback.cs
- ToolStripHighContrastRenderer.cs
- FixedPageAutomationPeer.cs
- UserPreferenceChangingEventArgs.cs
- SHA1Managed.cs
- Border.cs
- WebPartChrome.cs
- OrthographicCamera.cs
- PackageRelationshipSelector.cs
- WebPartEditorApplyVerb.cs
- LayoutDump.cs
- MediaTimeline.cs
- XdrBuilder.cs
- ConfigViewGenerator.cs
- EnvelopedPkcs7.cs
- DocumentPageView.cs
- HwndStylusInputProvider.cs
- ViewSimplifier.cs
- SelectionEditingBehavior.cs
- WebPartCollection.cs
- HtmlElementCollection.cs
- HttpTransportSecurityElement.cs
- Int32EqualityComparer.cs
- DataServiceHost.cs
- WindowsAuthenticationEventArgs.cs
- SimpleHandlerBuildProvider.cs
- TrackingStringDictionary.cs
- UnsafeNativeMethods.cs
- Vector3DCollectionValueSerializer.cs
- WindowsSpinner.cs
- ColorMap.cs
- BitmapImage.cs
- BamlRecordReader.cs
- ConfigXmlCDataSection.cs
- AppDomainFactory.cs
- DataGridViewRowPrePaintEventArgs.cs
- QueryOutputWriter.cs
- SafeProcessHandle.cs
- LineBreak.cs
- Tag.cs
- XmlSchemaParticle.cs
- RequestBringIntoViewEventArgs.cs
- PerformanceCounterManager.cs
- XhtmlBasicTextViewAdapter.cs
- MarkupObject.cs
- cache.cs
- AttachedPropertyBrowsableAttribute.cs
- MachineKeyConverter.cs
- TextTabProperties.cs
- RelAssertionDirectKeyIdentifierClause.cs
- CfgParser.cs
- DebugView.cs
- SelectionListComponentEditor.cs
- Margins.cs
- StrongNameKeyPair.cs
- ResourceReferenceExpressionConverter.cs
- ResourceIDHelper.cs
- FastEncoderStatics.cs
- Merger.cs
- NullableFloatMinMaxAggregationOperator.cs
- TextEffectResolver.cs
- Selection.cs
- Single.cs
- InvariantComparer.cs
- SimpleTypesSurrogate.cs
- Expander.cs
- ArglessEventHandlerProxy.cs
- XslCompiledTransform.cs
- BitmapPalettes.cs
- ControlBuilder.cs
- AutoGeneratedField.cs
- CodeParameterDeclarationExpressionCollection.cs
- CodeDomSerializer.cs
- ProxyManager.cs
- PeerNode.cs
- DocumentViewerBaseAutomationPeer.cs
- DynamicDiscoveryDocument.cs
- TagPrefixInfo.cs
- ConvertEvent.cs
- HttpWebRequestElement.cs
- SqlServer2KCompatibilityCheck.cs
- GridItem.cs
- UpDownEvent.cs
- RelationshipDetailsCollection.cs
- SqlDataReader.cs
- HashMembershipCondition.cs
- IdentityManager.cs
- IteratorFilter.cs
- NativeMethods.cs
- TypeDescriptionProviderAttribute.cs
- IntegrationExceptionEventArgs.cs
- Solver.cs
- OrthographicCamera.cs
- SqlRowUpdatedEvent.cs
- MaskInputRejectedEventArgs.cs
- EntityCollection.cs
- HitTestWithGeometryDrawingContextWalker.cs
- NullReferenceException.cs