Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Automation / Peers / DocumentViewerBaseAutomationPeer.cs / 1 / DocumentViewerBaseAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentViewerBaseAutomationPeer.cs // // Description: AutomationPeer associated with DocumentViewerBase. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Windows.Controls.Primitives; // DocumentViewerBase using System.Windows.Documents; // IDocumentPaginatorSource namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with DocumentViewerBase. /// public class DocumentViewerBaseAutomationPeer : FrameworkElementAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public DocumentViewerBaseAutomationPeer(DocumentViewerBase owner) : base(owner) { } ////// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Text) { // Make sure that Automation children are created. this.GetChildren(); // Re-expose TextPattern from hosted document. if (_documentPeer != null) { _documentPeer.EventsSource = this; returnValue = _documentPeer.GetPattern(patternInterface); } } return returnValue; } ////// /// ////// /// AutomationPeer associated with DocumentViewerBase returns an AutomationPeer /// for hosted Document and for elements in the style. /// protected override ListGetChildrenCore() { // Get children for all elements in the style. List children = base.GetChildrenCore(); // Add AutomationPeer associated with the document. // Make it the first child of the collection. AutomationPeer documentPeer = GetDocumentAutomationPeer(); if (_documentPeer != documentPeer) { if (_documentPeer != null) { _documentPeer.OnDisconnected(); } _documentPeer = documentPeer as DocumentAutomationPeer; } if (documentPeer != null) { if (children == null) { children = new List (); } children.Add(documentPeer); } return children; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Document; } ////// /// protected override string GetClassNameCore() { return "DocumentViewer"; } ////// /// Retrieves AutomationPeer for the document. /// ///private AutomationPeer GetDocumentAutomationPeer() { AutomationPeer documentPeer = null; IDocumentPaginatorSource document = ((DocumentViewerBase)Owner).Document; if (document != null) { if (document is UIElement) { documentPeer = UIElementAutomationPeer.CreatePeerForElement((UIElement)document); } else if (document is ContentElement) { documentPeer = ContentElementAutomationPeer.CreatePeerForElement((ContentElement)document); } } return documentPeer; } private DocumentAutomationPeer _documentPeer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentViewerBaseAutomationPeer.cs // // Description: AutomationPeer associated with DocumentViewerBase. // //--------------------------------------------------------------------------- using System.Collections.Generic; // List using System.Windows.Controls.Primitives; // DocumentViewerBase using System.Windows.Documents; // IDocumentPaginatorSource namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with DocumentViewerBase. /// public class DocumentViewerBaseAutomationPeer : FrameworkElementAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public DocumentViewerBaseAutomationPeer(DocumentViewerBase owner) : base(owner) { } ////// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Text) { // Make sure that Automation children are created. this.GetChildren(); // Re-expose TextPattern from hosted document. if (_documentPeer != null) { _documentPeer.EventsSource = this; returnValue = _documentPeer.GetPattern(patternInterface); } } return returnValue; } ////// /// ////// /// AutomationPeer associated with DocumentViewerBase returns an AutomationPeer /// for hosted Document and for elements in the style. /// protected override ListGetChildrenCore() { // Get children for all elements in the style. List children = base.GetChildrenCore(); // Add AutomationPeer associated with the document. // Make it the first child of the collection. AutomationPeer documentPeer = GetDocumentAutomationPeer(); if (_documentPeer != documentPeer) { if (_documentPeer != null) { _documentPeer.OnDisconnected(); } _documentPeer = documentPeer as DocumentAutomationPeer; } if (documentPeer != null) { if (children == null) { children = new List (); } children.Add(documentPeer); } return children; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Document; } ////// /// protected override string GetClassNameCore() { return "DocumentViewer"; } ////// /// Retrieves AutomationPeer for the document. /// ///private AutomationPeer GetDocumentAutomationPeer() { AutomationPeer documentPeer = null; IDocumentPaginatorSource document = ((DocumentViewerBase)Owner).Document; if (document != null) { if (document is UIElement) { documentPeer = UIElementAutomationPeer.CreatePeerForElement((UIElement)document); } else if (document is ContentElement) { documentPeer = ContentElementAutomationPeer.CreatePeerForElement((ContentElement)document); } } return documentPeer; } private DocumentAutomationPeer _documentPeer; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
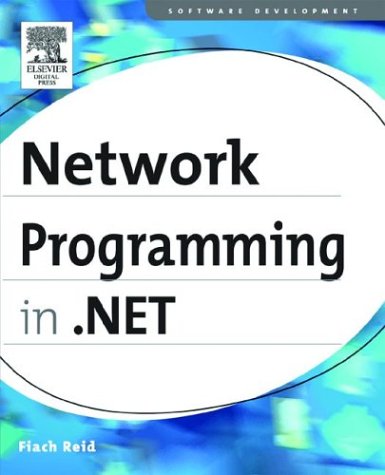
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParsedAttributeCollection.cs
- SQLDateTimeStorage.cs
- TimeSpan.cs
- RowVisual.cs
- MILUtilities.cs
- WorkflowTerminatedException.cs
- SchemaCollectionCompiler.cs
- DataGridViewCellStyleBuilderDialog.cs
- XmlTextReader.cs
- _ListenerAsyncResult.cs
- LiteralControl.cs
- XmlIgnoreAttribute.cs
- System.Data_BID.cs
- HashRepartitionStream.cs
- FullTextState.cs
- WebBrowserHelper.cs
- ExtendedTransformFactory.cs
- MetafileHeaderEmf.cs
- ZoneMembershipCondition.cs
- QuaternionConverter.cs
- InstanceDescriptor.cs
- Size.cs
- MapPathBasedVirtualPathProvider.cs
- InfoCardTraceRecord.cs
- SurrogateDataContract.cs
- ToolStripOverflow.cs
- SignerInfo.cs
- CellTreeNode.cs
- HandlerFactoryCache.cs
- StickyNoteHelper.cs
- BitHelper.cs
- X500Name.cs
- GridEntryCollection.cs
- ImageInfo.cs
- SmiEventSink_Default.cs
- DependencyProperty.cs
- XmlSchemas.cs
- DropDownList.cs
- DecimalKeyFrameCollection.cs
- ModifierKeysConverter.cs
- TimeSpan.cs
- FormatSettings.cs
- InstanceCollisionException.cs
- InplaceBitmapMetadataWriter.cs
- BooleanFunctions.cs
- DataBoundControlDesigner.cs
- ExpressionPrefixAttribute.cs
- MemoryStream.cs
- DataPointer.cs
- SuppressedPackageProperties.cs
- PropertyCondition.cs
- ProtocolsConfigurationHandler.cs
- CommunicationObjectManager.cs
- SqlDataReaderSmi.cs
- hresults.cs
- VersionPair.cs
- ProcessThread.cs
- BitArray.cs
- PolyBezierSegmentFigureLogic.cs
- CodeGeneratorAttribute.cs
- DbProviderFactoriesConfigurationHandler.cs
- NativeObjectSecurity.cs
- NamedObject.cs
- ILGenerator.cs
- DynamicArgumentDesigner.xaml.cs
- SiteMapNode.cs
- IntegerValidatorAttribute.cs
- XmlSerializableServices.cs
- PassportPrincipal.cs
- ActiveXSite.cs
- GridViewHeaderRowPresenter.cs
- StylusShape.cs
- FileDialogCustomPlacesCollection.cs
- SelectionRange.cs
- RestHandler.cs
- TextTreeRootTextBlock.cs
- WebAdminConfigurationHelper.cs
- CustomWebEventKey.cs
- CssClassPropertyAttribute.cs
- XmlUTF8TextWriter.cs
- KnownColorTable.cs
- DocumentViewerAutomationPeer.cs
- PolicyChain.cs
- WindowsImpersonationContext.cs
- StaticDataManager.cs
- TransformerConfigurationWizardBase.cs
- MetadataSerializer.cs
- LayoutTable.cs
- WebPartEditorApplyVerb.cs
- ToolBarPanel.cs
- EventMappingSettings.cs
- ProcessModule.cs
- GeometryDrawing.cs
- ConnectionPoolManager.cs
- CachingHintValidation.cs
- ObjectResult.cs
- StatusBarPanelClickEvent.cs
- DataGridViewLayoutData.cs
- DataControlReference.cs
- PersonalizationAdministration.cs