Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextEmbeddedObject.cs / 1 / TextEmbeddedObject.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextEmbeddedObject.cs // // Contents: Definition of text embedded object // // Spec: http://avalon/text/DesignDocsAndSpecs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Windows.Media; using MS.Internal.TextFormatting; namespace System.Windows.Media.TextFormatting { ////// Provide definition for a kind of text content in which measuring, hittesting /// and drawing of the entire content is done in whole. Example of that kind of /// content is a button in the middle of the line. /// public abstract class TextEmbeddedObject : TextRun { ////// Line break condition before text object /// public abstract LineBreakCondition BreakBefore { get; } ////// Line break condition after text object /// public abstract LineBreakCondition BreakAfter { get; } ////// Flag indicates whether text object has fixed size regardless of where /// it is placed within a line /// public abstract bool HasFixedSize { get; } ////// Get text object measurement metrics that will fit within the specified /// remaining width of the paragraph /// /// remaining paragraph width ///text object metrics public abstract TextEmbeddedObjectMetrics Format( double remainingParagraphWidth ); ////// Get computed bounding box of text object /// /// run is drawn from right to left /// run is drawn with its side parallel to baseline ///computed bounding box size of text object public abstract Rect ComputeBoundingBox( bool rightToLeft, bool sideways ); ////// Draw text object /// /// drawing context /// origin where the object is drawn /// run is drawn from right to left /// run is drawn with its side parallel to baseline public abstract void Draw( DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways ); } ////// Text object properties /// public class TextEmbeddedObjectMetrics { private double _width; private double _height; private double _baseline; ////// Construct a text object size /// /// object width /// object height /// object baseline in ratio relative to run height public TextEmbeddedObjectMetrics( double width, double height, double baseline ) { _width = width; _height = height; _baseline = baseline; } ////// Object width /// public double Width { get { return _width; } } ////// Object height /// ///public double Height { get { return _height; } } /// /// Object baseline in ratio relative to run height /// public double Baseline { get { return _baseline; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextEmbeddedObject.cs // // Contents: Definition of text embedded object // // Spec: http://avalon/text/DesignDocsAndSpecs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Windows.Media; using MS.Internal.TextFormatting; namespace System.Windows.Media.TextFormatting { ////// Provide definition for a kind of text content in which measuring, hittesting /// and drawing of the entire content is done in whole. Example of that kind of /// content is a button in the middle of the line. /// public abstract class TextEmbeddedObject : TextRun { ////// Line break condition before text object /// public abstract LineBreakCondition BreakBefore { get; } ////// Line break condition after text object /// public abstract LineBreakCondition BreakAfter { get; } ////// Flag indicates whether text object has fixed size regardless of where /// it is placed within a line /// public abstract bool HasFixedSize { get; } ////// Get text object measurement metrics that will fit within the specified /// remaining width of the paragraph /// /// remaining paragraph width ///text object metrics public abstract TextEmbeddedObjectMetrics Format( double remainingParagraphWidth ); ////// Get computed bounding box of text object /// /// run is drawn from right to left /// run is drawn with its side parallel to baseline ///computed bounding box size of text object public abstract Rect ComputeBoundingBox( bool rightToLeft, bool sideways ); ////// Draw text object /// /// drawing context /// origin where the object is drawn /// run is drawn from right to left /// run is drawn with its side parallel to baseline public abstract void Draw( DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways ); } ////// Text object properties /// public class TextEmbeddedObjectMetrics { private double _width; private double _height; private double _baseline; ////// Construct a text object size /// /// object width /// object height /// object baseline in ratio relative to run height public TextEmbeddedObjectMetrics( double width, double height, double baseline ) { _width = width; _height = height; _baseline = baseline; } ////// Object width /// public double Width { get { return _width; } } ////// Object height /// ///public double Height { get { return _height; } } /// /// Object baseline in ratio relative to run height /// public double Baseline { get { return _baseline; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
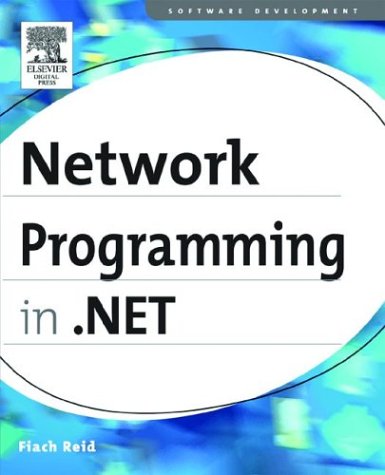
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegionInfo.cs
- SimpleType.cs
- WindowsRichEdit.cs
- Pair.cs
- Storyboard.cs
- CompilationUtil.cs
- PromptBuilder.cs
- DrawListViewColumnHeaderEventArgs.cs
- CodeMemberEvent.cs
- DateTimeConstantAttribute.cs
- PersistenceMetadataNamespace.cs
- SafePEFileHandle.cs
- ControlPropertyNameConverter.cs
- DictionarySurrogate.cs
- SimpleHandlerBuildProvider.cs
- TableCell.cs
- PasswordPropertyTextAttribute.cs
- LinkArea.cs
- DataSourceSelectArguments.cs
- UnauthorizedAccessException.cs
- VariableModifiersHelper.cs
- TimeManager.cs
- TextViewSelectionProcessor.cs
- CodeComment.cs
- Invariant.cs
- WizardPanel.cs
- TableLayoutStyle.cs
- X509Certificate2.cs
- HtmlInputReset.cs
- SerializationStore.cs
- DesignerSerializerAttribute.cs
- ConnectorRouter.cs
- XmlnsCompatibleWithAttribute.cs
- BitmapMetadataEnumerator.cs
- Point3D.cs
- WebContentFormatHelper.cs
- log.cs
- DesignerOptionService.cs
- PostBackTrigger.cs
- QuadraticBezierSegment.cs
- CroppedBitmap.cs
- DynamicPropertyHolder.cs
- GeometryConverter.cs
- RoleManagerSection.cs
- BrushConverter.cs
- BitmapScalingModeValidation.cs
- ToolboxItemAttribute.cs
- AutoGeneratedFieldProperties.cs
- FileDialog_Vista_Interop.cs
- ClientConfigurationHost.cs
- StringFunctions.cs
- HtmlElementEventArgs.cs
- EventEntry.cs
- CodeTypeMemberCollection.cs
- CustomAttribute.cs
- JsonDeserializer.cs
- control.ime.cs
- TreeViewCancelEvent.cs
- GridViewDeletedEventArgs.cs
- unsafenativemethodsother.cs
- XmlSchemaChoice.cs
- AccessViolationException.cs
- TransformerInfo.cs
- PersonalizationDictionary.cs
- HttpCacheVaryByContentEncodings.cs
- CommunicationException.cs
- TypeConverterAttribute.cs
- OleDbFactory.cs
- HMACRIPEMD160.cs
- BuildProviderAppliesToAttribute.cs
- RegexCompilationInfo.cs
- WebHttpDispatchOperationSelector.cs
- UseAttributeSetsAction.cs
- UxThemeWrapper.cs
- PixelFormatConverter.cs
- DataSourceSelectArguments.cs
- WebPartCancelEventArgs.cs
- TextTreeFixupNode.cs
- RelationHandler.cs
- XmlJsonWriter.cs
- LinqDataSourceSelectEventArgs.cs
- StreamGeometry.cs
- CompilerGlobalScopeAttribute.cs
- ReachPrintTicketSerializer.cs
- DescendantBaseQuery.cs
- TemplateXamlTreeBuilder.cs
- SafeSecurityHandles.cs
- TypeListConverter.cs
- OptimalTextSource.cs
- ListSortDescription.cs
- AdapterUtil.cs
- DataControlReference.cs
- ColumnPropertiesGroup.cs
- SqlConnectionString.cs
- TextCompositionManager.cs
- SortDescriptionCollection.cs
- serverconfig.cs
- mediaeventshelper.cs
- WebPartEditVerb.cs
- InputMethodStateChangeEventArgs.cs