Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Annotations / Anchoring / TextViewSelectionProcessor.cs / 1305600 / TextViewSelectionProcessor.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A SelectionProcessor subclass which produces locator parts that select // all anchors that intersect with the text in an element's TextView. // // History: // 11/29/2006: rruiz: Created //----------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Windows.Annotations; using System.Windows.Annotations.Storage; using System.Windows.Controls; using System.Windows.Documents; using System.Xml; using MS.Utility; using System.Windows.Media; using System.Windows.Controls.Primitives; using MS.Internal.Documents; using MS.Internal.PtsHost; namespace MS.Internal.Annotations.Anchoring { ////// internal class TextViewSelectionProcessor : SelectionProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of TextViewSelectionProcessor. /// public TextViewSelectionProcessor() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Not implemented for this selection processor. /// /// selection to merge /// other selection to merge /// always set to null because DocumentPageViews cannot be merged ///always returns false because DocumentPageViewss cannot be merged public override bool MergeSelections(Object selection1, Object selection2, out Object newSelection) { newSelection = null; return false; } ////// Simply returns the selection passed in which should be an element. /// /// the selection to examine ///a list containing the selection ///selection is null ///selection is of wrong type public override IListGetSelectedNodes(Object selection) { // Verify selection is a service provider that provides ITextView VerifySelection(selection); return new DependencyObject[] { (DependencyObject)selection }; } /// /// Simply returns the selection which should be an element. /// /// the selection to examine ///the selection itself ///selection is null ///selection is of wrong type public override UIElement GetParent(Object selection) { // Verify selection is a service provider that provides ITextView VerifySelection(selection); return (UIElement)selection; } ////// Gets the anchor point for the selection. This isn't a runtime selection /// and therefore shouldn't be anchored to. This processor returns a point of /// (Double.NaN, Double.NaN). /// /// the selection to examine ///selection is null ///selection is of wrong type public override Point GetAnchorPoint(Object selection) { // Verify selection is a DocumentPageView VerifySelection(selection); // Returns the point (Nan,Nan) return new Point(double.NaN,double.NaN); } ////// Creates one or more locator parts representing the portion /// of 'startNode' spanned by 'selection'. /// /// the selection that is being processed /// the node the locator parts should be in the /// context of ///one or more locator parts representing the portion of 'startNode' spanned /// by 'selection' ///startNode or selection is null ///selection is of the wrong type public override IListGenerateLocatorParts(Object selection, DependencyObject startNode) { if (startNode == null) throw new ArgumentNullException("startNode"); List res = null; ITextView textView = VerifySelection(selection); res = new List (1); int startOffset; int endOffset; if (textView != null && textView.IsValid) { GetTextViewTextRange(textView, out startOffset, out endOffset); } else { // This causes no content to be loaded startOffset = -1; endOffset = -1; } ContentLocatorPart part = new ContentLocatorPart(TextSelectionProcessor.CharacterRangeElementName);// DocumentPageViewLocatorPart(); part.NameValuePairs.Add(TextSelectionProcessor.CountAttribute, 1.ToString(NumberFormatInfo.InvariantInfo)); part.NameValuePairs.Add(TextSelectionProcessor.SegmentAttribute + 0.ToString(NumberFormatInfo.InvariantInfo), startOffset.ToString(NumberFormatInfo.InvariantInfo) + TextSelectionProcessor.Separator[0] + endOffset.ToString(NumberFormatInfo.InvariantInfo)); part.NameValuePairs.Add(TextSelectionProcessor.IncludeOverlaps, Boolean.TrueString); res.Add(part); return res; } /// /// This processor doesn't resolve ContentLocatorParts. It simply returns null. /// /// locator part specifying data to be spanned /// the node to be spanned by the created /// selection /// always set to AttachmentLevel.Unresolved ///always returns null; this processor does not resolve ContentLocatorParts ///locatorPart or startNode are null public override Object ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out AttachmentLevel attachmentLevel) { if (locatorPart == null) throw new ArgumentNullException("locatorPart"); if (startNode == null) throw new ArgumentNullException("startNode"); attachmentLevel = AttachmentLevel.Unresolved; // ContentLocator Parts generated by this selection processor cannot be resolved return null; } ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can generate. This processor /// does not resolve these ContentLocatorParts - only generates them. /// public override XmlQualifiedName[] GetLocatorPartTypes() { return (XmlQualifiedName[])LocatorPartTypeNames.Clone(); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //----------------------------------------------------- //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Returns the TextRange representing the visible contents of the ITextView. /// Also returns the same information in terms of the offsets to the start and end. /// /// ITextView to get visible contents of /// offset into the document representing start of visible content /// offset into the document representing end of visible content ///TextRange spanning all visible content internal static TextRange GetTextViewTextRange(ITextView textView, out int startOffset, out int endOffset) { Debug.Assert(textView != null); // These are the default in case we don't find any content in the DocumentPageView startOffset = int.MinValue; endOffset = 0; TextRange textRange = null; IListsegments = textView.TextSegments; if (segments != null && segments.Count > 0) { ITextPointer start = segments[0].Start; ITextPointer end = segments[segments.Count - 1].End; startOffset = end.TextContainer.Start.GetOffsetToPosition(start); endOffset = end.TextContainer.Start.GetOffsetToPosition(end); textRange = new TextRange(start, end); } return textRange; } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// Verify the selection is of the correct type. /// /// selection to verify ///the selection cast to the necessary type private ITextView VerifySelection(object selection) { if (selection == null) throw new ArgumentNullException("selection"); IServiceProvider provider = selection as IServiceProvider; if (provider == null) throw new ArgumentException(SR.Get(SRID.SelectionMustBeServiceProvider),"selection"); ITextView textView = provider.GetService(typeof(ITextView)) as ITextView; return textView; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // ContentLocator part types understood by this processor private static readonly XmlQualifiedName[] LocatorPartTypeNames = new XmlQualifiedName[0]; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
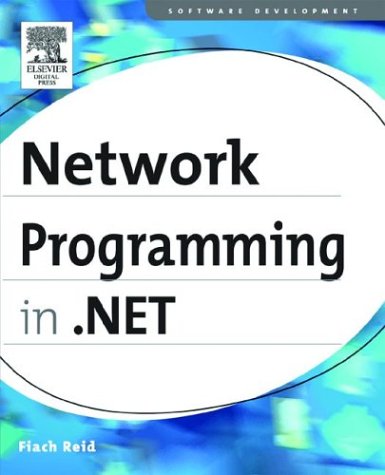
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyBuilderData.cs
- PermissionSet.cs
- WrapPanel.cs
- XmlRawWriter.cs
- TimeZoneInfo.cs
- CustomWebEventKey.cs
- MenuItemCollectionEditorDialog.cs
- SqlDataSourceEnumerator.cs
- FileDialog_Vista.cs
- XmlQueryTypeFactory.cs
- CellParaClient.cs
- MSHTMLHost.cs
- QueryCursorEventArgs.cs
- HandledEventArgs.cs
- HMACMD5.cs
- SelectionProviderWrapper.cs
- VerbConverter.cs
- COM2Properties.cs
- XmlDataSourceNodeDescriptor.cs
- DiffuseMaterial.cs
- TextTreeText.cs
- IChannel.cs
- UIElement.cs
- OleDbConnectionFactory.cs
- AdornerHitTestResult.cs
- EntityContainer.cs
- BroadcastEventHelper.cs
- ProcessModule.cs
- DataSourceHelper.cs
- BasicHttpBindingCollectionElement.cs
- IndentedTextWriter.cs
- FileRecordSequenceHelper.cs
- SelectionProcessor.cs
- ZipIOLocalFileHeader.cs
- Parser.cs
- SrgsOneOf.cs
- Matrix.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- MulticastNotSupportedException.cs
- MessageQueueConverter.cs
- RpcResponse.cs
- PassportPrincipal.cs
- _BufferOffsetSize.cs
- DataServicePagingProviderWrapper.cs
- namescope.cs
- ApplicationHost.cs
- LoginCancelEventArgs.cs
- ConfigXmlSignificantWhitespace.cs
- SequentialOutput.cs
- NGCUIElementCollectionSerializerAsync.cs
- ExpandSegment.cs
- FtpRequestCacheValidator.cs
- CalendarButtonAutomationPeer.cs
- KnownColorTable.cs
- DiffuseMaterial.cs
- DefinitionBase.cs
- XPathSingletonIterator.cs
- DataSetUtil.cs
- TablePattern.cs
- MultiByteCodec.cs
- EventlogProvider.cs
- _UriTypeConverter.cs
- TemplateDefinition.cs
- SafeEventLogReadHandle.cs
- TextWriterEngine.cs
- ExpressionReplacer.cs
- XmlAnyAttributeAttribute.cs
- ApplicationTrust.cs
- SerializationInfoEnumerator.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- ImageInfo.cs
- DefaultSerializationProviderAttribute.cs
- SamlAuthorizationDecisionStatement.cs
- MonitoringDescriptionAttribute.cs
- ThumbAutomationPeer.cs
- GPRECT.cs
- XComponentModel.cs
- ApplicationSettingsBase.cs
- GridViewSelectEventArgs.cs
- Events.cs
- ScanQueryOperator.cs
- HttpStreamXmlDictionaryWriter.cs
- StyleModeStack.cs
- DependencyObjectPropertyDescriptor.cs
- WebMessageEncoderFactory.cs
- DataGridItemCollection.cs
- KeySplineConverter.cs
- TTSEngineProxy.cs
- ContextStack.cs
- LongValidator.cs
- CapabilitiesUse.cs
- ValidationSummary.cs
- DeadCharTextComposition.cs
- SQLUtility.cs
- MaskedTextBox.cs
- BaseDataListActionList.cs
- DataGridColumnHeaderItemAutomationPeer.cs
- OracleDataAdapter.cs
- ColorKeyFrameCollection.cs