Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Annotations / Anchoring / SelectionProcessor.cs / 1 / SelectionProcessor.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SelectionProcessor is an abstract class defining the API for // creating and processing selections in the annotation framework. // SelectionProcessors are responsible for creating locator parts representing // a selection and for creating selections based on a locator part. // Spec: http://team/sites/ag/Specifications/Anchoring%20Namespace%20Spec.doc // // History: // 12/01/2002: magedz: Created - based on architectural discussions and design // by axelk, rruiz, magedz // 04/01/2003: rruiz: Updated file as part of integrating into working system // 07/21/2003: rruiz: Ported to WCP tree. // 08/18/2003: rruiz: Updated to Anchoring Namespace spec. // 05/07/2004: ssimova: Removed Xpath expressions // 01/07/2004: rruiz: Removed LocatorManager property. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Annotations; using System.Windows.Media; using MS.Utility; using System.Xml; namespace MS.Internal.Annotations.Anchoring { ////// SelectionProcessor is an abstract class defining the API for /// creating and processing selections in the annotation framework. /// SelectionProcessors are responsible for creating locator parts representing /// a selection and for creating selections based on a locator part. /// internal abstract class SelectionProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of SelectionProcessor. /// protected SelectionProcessor() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Merges the two selections into one, if possible. /// /// selection to merge /// other selection to merge /// new selection that contains the data from both /// selection1 and selection2 ///true if the selections were merged, false otherwise /// ///selection1 or selection2 are /// null public abstract bool MergeSelections(Object selection1, Object selection2, out Object newSelection); ////// Gets the tree elements spanned by the selection. /// /// the selection to examine ///a list of elements spanned by the selection; never returns /// null ///selection is null ///selection is of wrong type public abstract IListGetSelectedNodes(Object selection); /// /// Gets the parent element of this selection. /// /// the selection to examine ///the parent element of the selection; can be null ///selection is null ///selection is of wrong type public abstract UIElement GetParent(Object selection); ////// Gets the anchor point for the selection /// /// the selection to examine ///the anchor point of the selection; can be null ///selection is null ///selection is of wrong type public abstract Point GetAnchorPoint(Object selection); ////// Creates one or more locator parts representing the portion /// of 'startNode' spanned by 'selection'. /// /// the selection that is being processed /// the node the locator parts should be in the /// context of ///one or more locator parts representing the portion of 'startNode' spanned /// by 'selection' ///startNode or selection is null ///startNode is not a DependencyObject or /// selection is of the wrong type public abstract IListGenerateLocatorParts(Object selection, DependencyObject startNode); /// /// Creates a selection object spanning the portion of 'startNode' /// specified by 'locatorPart'. /// /// locator part specifying data to be spanned /// the node to be spanned by the created /// selection /// describes the level of resolution reached when resolving the locator part ///a selection spanning the portion of 'startNode' specified by /// 'locatorPart' ///locatorPart or startNode are /// null ///locatorPart is of the incorrect type public abstract Object ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out AttachmentLevel attachmentLevel); ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can resolve/generate. /// public abstract XmlQualifiedName[] GetLocatorPartTypes(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //----------------------------------------------------- } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SelectionProcessor is an abstract class defining the API for // creating and processing selections in the annotation framework. // SelectionProcessors are responsible for creating locator parts representing // a selection and for creating selections based on a locator part. // Spec: http://team/sites/ag/Specifications/Anchoring%20Namespace%20Spec.doc // // History: // 12/01/2002: magedz: Created - based on architectural discussions and design // by axelk, rruiz, magedz // 04/01/2003: rruiz: Updated file as part of integrating into working system // 07/21/2003: rruiz: Ported to WCP tree. // 08/18/2003: rruiz: Updated to Anchoring Namespace spec. // 05/07/2004: ssimova: Removed Xpath expressions // 01/07/2004: rruiz: Removed LocatorManager property. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Annotations; using System.Windows.Media; using MS.Utility; using System.Xml; namespace MS.Internal.Annotations.Anchoring { ////// SelectionProcessor is an abstract class defining the API for /// creating and processing selections in the annotation framework. /// SelectionProcessors are responsible for creating locator parts representing /// a selection and for creating selections based on a locator part. /// internal abstract class SelectionProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of SelectionProcessor. /// protected SelectionProcessor() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Merges the two selections into one, if possible. /// /// selection to merge /// other selection to merge /// new selection that contains the data from both /// selection1 and selection2 ///true if the selections were merged, false otherwise /// ///selection1 or selection2 are /// null public abstract bool MergeSelections(Object selection1, Object selection2, out Object newSelection); ////// Gets the tree elements spanned by the selection. /// /// the selection to examine ///a list of elements spanned by the selection; never returns /// null ///selection is null ///selection is of wrong type public abstract IListGetSelectedNodes(Object selection); /// /// Gets the parent element of this selection. /// /// the selection to examine ///the parent element of the selection; can be null ///selection is null ///selection is of wrong type public abstract UIElement GetParent(Object selection); ////// Gets the anchor point for the selection /// /// the selection to examine ///the anchor point of the selection; can be null ///selection is null ///selection is of wrong type public abstract Point GetAnchorPoint(Object selection); ////// Creates one or more locator parts representing the portion /// of 'startNode' spanned by 'selection'. /// /// the selection that is being processed /// the node the locator parts should be in the /// context of ///one or more locator parts representing the portion of 'startNode' spanned /// by 'selection' ///startNode or selection is null ///startNode is not a DependencyObject or /// selection is of the wrong type public abstract IListGenerateLocatorParts(Object selection, DependencyObject startNode); /// /// Creates a selection object spanning the portion of 'startNode' /// specified by 'locatorPart'. /// /// locator part specifying data to be spanned /// the node to be spanned by the created /// selection /// describes the level of resolution reached when resolving the locator part ///a selection spanning the portion of 'startNode' specified by /// 'locatorPart' ///locatorPart or startNode are /// null ///locatorPart is of the incorrect type public abstract Object ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out AttachmentLevel attachmentLevel); ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can resolve/generate. /// public abstract XmlQualifiedName[] GetLocatorPartTypes(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //----------------------------------------------------- } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
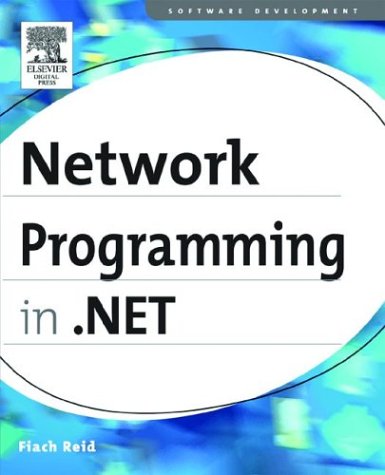
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _OverlappedAsyncResult.cs
- SignedXml.cs
- WizardPanelChangingEventArgs.cs
- ImportedNamespaceContextItem.cs
- CriticalHandle.cs
- ColumnMapTranslator.cs
- NewExpression.cs
- ToolStripDropDownItem.cs
- TcpTransportBindingElement.cs
- Camera.cs
- ModuleElement.cs
- WhiteSpaceTrimStringConverter.cs
- TextInfo.cs
- SliderAutomationPeer.cs
- SchemaNamespaceManager.cs
- XmlHierarchicalDataSourceView.cs
- OdbcConnection.cs
- CaseStatement.cs
- XmlUtf8RawTextWriter.cs
- XmlSchemaInfo.cs
- CodeSnippetTypeMember.cs
- BrowserTree.cs
- HMACRIPEMD160.cs
- EdmItemCollection.cs
- WebServiceClientProxyGenerator.cs
- CompositeTypefaceMetrics.cs
- PaintValueEventArgs.cs
- Animatable.cs
- GeneralTransform3DCollection.cs
- SharedConnectionListener.cs
- DataTableReader.cs
- SerializerProvider.cs
- AnnotationHelper.cs
- RoleGroup.cs
- XPathPatternBuilder.cs
- InputLanguage.cs
- MasterPageBuildProvider.cs
- DynamicField.cs
- PartBasedPackageProperties.cs
- DomainUpDown.cs
- XmlLoader.cs
- Triplet.cs
- WebPartsPersonalizationAuthorization.cs
- _ShellExpression.cs
- Encoder.cs
- RichTextBoxContextMenu.cs
- ItemsPresenter.cs
- PageStatePersister.cs
- PropertyCollection.cs
- Timer.cs
- TextContainerChangedEventArgs.cs
- RegexCode.cs
- BeginEvent.cs
- AccessText.cs
- DataGridBeginningEditEventArgs.cs
- unsafenativemethodstextservices.cs
- JulianCalendar.cs
- ResizeGrip.cs
- ConnectionManagementElement.cs
- OdbcConnectionOpen.cs
- ContractUtils.cs
- BypassElement.cs
- ButtonBase.cs
- HttpStreamMessage.cs
- NestedContainer.cs
- DataBoundControlHelper.cs
- UniqueEventHelper.cs
- Encoder.cs
- SHA512Managed.cs
- Component.cs
- FormsAuthenticationTicket.cs
- sqlser.cs
- PhoneCallDesigner.cs
- GridViewItemAutomationPeer.cs
- FeedUtils.cs
- DBDataPermission.cs
- shaper.cs
- InternalPolicyElement.cs
- PartialCachingAttribute.cs
- DoubleCollectionConverter.cs
- SqlSupersetValidator.cs
- Propagator.Evaluator.cs
- ImageConverter.cs
- ImageUrlEditor.cs
- RTLAwareMessageBox.cs
- XPathAncestorQuery.cs
- CryptoStream.cs
- DataGridViewCellEventArgs.cs
- FocusTracker.cs
- TrackingProfileSerializer.cs
- FastEncoder.cs
- ListBase.cs
- WebExceptionStatus.cs
- Int32.cs
- BooleanKeyFrameCollection.cs
- PageRequestManager.cs
- BaseDataList.cs
- StdValidatorsAndConverters.cs
- XmlSchemaCollection.cs
- DiscreteKeyFrames.cs