Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapMetadataEnumerator.cs / 1 / BitmapMetadataEnumerator.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapMetadataEnumerator.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { ////// An enumerator that iterates over the children of a timeline. /// internal struct BitmapMetadataEnumerator : IEnumerator, IEnumerator { #region External interface #region IEnumerator interface #region Properties /// /// Gets the current element in the collection. /// ////// The current element in the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] object IEnumerator.Current { get { return Current; } } #endregion // Properties #region Methods ////// Advances the enumerator to the next element of the collection. /// ////// True if the enumerator was successfully advanced to the next element; /// false if the enumerator has passed the end of the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] public bool MoveNext() { if (_fStarted && _current == null) { return false; } _fStarted = true; IntPtr ppStr = IntPtr.Zero; Int32 celtFetched = 0; try { int hr = UnsafeNativeMethods.EnumString.Next( _enumeratorHandle, 1, ref ppStr, ref celtFetched); if (HRESULT.IsWindowsCodecError(hr)) { _current = null; return false; } HRESULT.Check(hr); if (celtFetched == 0) { _current = null; return false; } else { _current = Marshal.PtrToStringUni(ppStr); } } finally { if (ppStr != IntPtr.Zero) { Marshal.FreeCoTaskMem(ppStr); ppStr = IntPtr.Zero; } } return true; } ////// Sets the enumerator to its initial position, which is before the first element /// in the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] public void Reset() { HRESULT.Check(UnsafeNativeMethods.EnumString.Reset(_enumeratorHandle)); _current = null; _fStarted = false; } #endregion // Methods #endregion // IEnumerator interface #region Properties ////// The current timeline referenced by this enumerator. /// public String Current { get { if (_current == null) { if (!_fStarted) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Enumerator_NotStarted)); } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Enumerator_ReachedEnd)); } } return _current; } } ////// /// void IDisposable.Dispose() { // Do nothing - Required by the IEnumerable contract. } #endregion // Properties #endregion // External interface #region Internal implementation #region Construction ////// Creates an enumerator iterates over the children of the specified container. /// /// /// Handle to a metadata query reader/writer /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal BitmapMetadataEnumerator(SafeMILHandle metadataHandle) { Debug.Assert(metadataHandle != null && !metadataHandle.IsInvalid); HRESULT.Check(UnsafeNativeMethods.WICMetadataQueryReader.GetEnumerator( metadataHandle, out _enumeratorHandle)); _current = null; _fStarted = false; } #endregion // Construction #region Methods #endregion // Methods #region Data ////// Critical - pointer to an unmanaged object that methods are called on. /// [SecurityCritical] private SafeMILHandle _enumeratorHandle; private String _current; private bool _fStarted; #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapMetadataEnumerator.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { ////// An enumerator that iterates over the children of a timeline. /// internal struct BitmapMetadataEnumerator : IEnumerator, IEnumerator { #region External interface #region IEnumerator interface #region Properties /// /// Gets the current element in the collection. /// ////// The current element in the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] object IEnumerator.Current { get { return Current; } } #endregion // Properties #region Methods ////// Advances the enumerator to the next element of the collection. /// ////// True if the enumerator was successfully advanced to the next element; /// false if the enumerator has passed the end of the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] public bool MoveNext() { if (_fStarted && _current == null) { return false; } _fStarted = true; IntPtr ppStr = IntPtr.Zero; Int32 celtFetched = 0; try { int hr = UnsafeNativeMethods.EnumString.Next( _enumeratorHandle, 1, ref ppStr, ref celtFetched); if (HRESULT.IsWindowsCodecError(hr)) { _current = null; return false; } HRESULT.Check(hr); if (celtFetched == 0) { _current = null; return false; } else { _current = Marshal.PtrToStringUni(ppStr); } } finally { if (ppStr != IntPtr.Zero) { Marshal.FreeCoTaskMem(ppStr); ppStr = IntPtr.Zero; } } return true; } ////// Sets the enumerator to its initial position, which is before the first element /// in the collection. /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] public void Reset() { HRESULT.Check(UnsafeNativeMethods.EnumString.Reset(_enumeratorHandle)); _current = null; _fStarted = false; } #endregion // Methods #endregion // IEnumerator interface #region Properties ////// The current timeline referenced by this enumerator. /// public String Current { get { if (_current == null) { if (!_fStarted) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Enumerator_NotStarted)); } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Enumerator_ReachedEnd)); } } return _current; } } ////// /// void IDisposable.Dispose() { // Do nothing - Required by the IEnumerable contract. } #endregion // Properties #endregion // External interface #region Internal implementation #region Construction ////// Creates an enumerator iterates over the children of the specified container. /// /// /// Handle to a metadata query reader/writer /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal BitmapMetadataEnumerator(SafeMILHandle metadataHandle) { Debug.Assert(metadataHandle != null && !metadataHandle.IsInvalid); HRESULT.Check(UnsafeNativeMethods.WICMetadataQueryReader.GetEnumerator( metadataHandle, out _enumeratorHandle)); _current = null; _fStarted = false; } #endregion // Construction #region Methods #endregion // Methods #region Data ////// Critical - pointer to an unmanaged object that methods are called on. /// [SecurityCritical] private SafeMILHandle _enumeratorHandle; private String _current; private bool _fStarted; #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
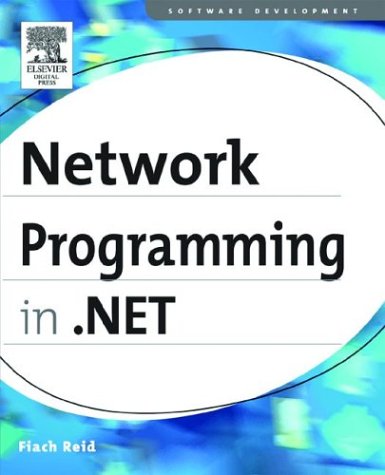
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PolicyVersion.cs
- LocationSectionRecord.cs
- Delegate.cs
- XmlNodeChangedEventManager.cs
- HtmlImage.cs
- OperatorExpressions.cs
- DataSourceXmlSerializationAttribute.cs
- InputLanguage.cs
- ProcessInfo.cs
- ManagedIStream.cs
- WebEventCodes.cs
- BreakSafeBase.cs
- VectorAnimationBase.cs
- PrimaryKeyTypeConverter.cs
- XmlSchemaComplexContentRestriction.cs
- FtpRequestCacheValidator.cs
- SystemWebSectionGroup.cs
- TextEndOfParagraph.cs
- WeakReferenceEnumerator.cs
- ADConnectionHelper.cs
- TaiwanCalendar.cs
- _LocalDataStoreMgr.cs
- TimelineGroup.cs
- UnmanagedHandle.cs
- PersonalizationStateInfoCollection.cs
- XmlAtomicValue.cs
- XmlCharCheckingWriter.cs
- EmissiveMaterial.cs
- EntityTemplateFactory.cs
- ADMembershipUser.cs
- IApplicationTrustManager.cs
- WebBrowser.cs
- CSharpCodeProvider.cs
- ProxyAssemblyNotLoadedException.cs
- EncryptedPackage.cs
- NameSpaceExtractor.cs
- Error.cs
- SecurityTokenSerializer.cs
- ChtmlLinkAdapter.cs
- FactoryId.cs
- sqlnorm.cs
- HtmlInputButton.cs
- StreamGeometryContext.cs
- UnsafeNativeMethodsTablet.cs
- CodeMemberProperty.cs
- StrongNameUtility.cs
- CacheEntry.cs
- InstancePersistenceCommand.cs
- RightsController.cs
- ResolveNameEventArgs.cs
- FixedSOMFixedBlock.cs
- TreeViewImageKeyConverter.cs
- CalendarDateChangedEventArgs.cs
- WebConfigurationFileMap.cs
- ParsedRoute.cs
- AccessKeyManager.cs
- Task.cs
- CharAnimationBase.cs
- FaultReasonText.cs
- AssemblyInfo.cs
- DataKey.cs
- CryptoStream.cs
- SelectionItemPattern.cs
- EventLogEntryCollection.cs
- ExtendedPropertyCollection.cs
- StandardBindingCollectionElement.cs
- WindowsGraphics2.cs
- ToolStripOverflowButton.cs
- AppDomainFactory.cs
- XamlTemplateSerializer.cs
- EditingMode.cs
- ServiceReference.cs
- ToolStripPanelRenderEventArgs.cs
- GlobalEventManager.cs
- HtmlControlPersistable.cs
- ColorComboBox.cs
- TextStore.cs
- IncrementalReadDecoders.cs
- UniqueEventHelper.cs
- TrustSection.cs
- VSWCFServiceContractGenerator.cs
- MailWebEventProvider.cs
- ToolboxCategory.cs
- PrefixQName.cs
- Select.cs
- Zone.cs
- ReliabilityContractAttribute.cs
- ISSmlParser.cs
- SearchForVirtualItemEventArgs.cs
- DetailsViewUpdatedEventArgs.cs
- UniqueConstraint.cs
- MimeTypeMapper.cs
- XamlVector3DCollectionSerializer.cs
- KeyFrames.cs
- TreeViewCancelEvent.cs
- ContentPosition.cs
- HashAlgorithm.cs
- QuaternionRotation3D.cs
- Enlistment.cs
- TemplatePartAttribute.cs