Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / FacetValueContainer.cs / 1 / FacetValueContainer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// This Class is never expected to be used except for by the FacetValues class. /// /// The purpose of this class is to allow strong type checking by the compiler while setting facet values which /// are typically stored as Object because they can either on of these things /// /// 1. null /// 2. scalar type (bool, int, byte) /// 3. Unbounded object /// /// without this class it would be very easy to accidentally set precision to an int when it really is supposed to be /// a byte value. Also you would be able to set the facet value to any Object derived class (ANYTHING!!!) when really only /// null and Unbounded are allowed besides an actual scalar value. The magic of the class happens in the implicit constructors with /// allow patterns like /// /// new FacetValues( MaxLength = EdmConstants.UnboundedValue, Nullable = true}; /// /// and these are type checked at compile time /// ///internal struct FacetValueContainer { T _value; bool _hasValue; bool _isUnbounded; internal T Value { set { _isUnbounded = false; _hasValue = true; _value = value; } } private void SetUnbounded() { _isUnbounded = true; _hasValue = true; } // don't add an implicit conversion from object because it will kill the compile time type checking. public static implicit operator FacetValueContainer (System.Data.Metadata.Edm.EdmConstants.Unbounded unbounded) { Debug.Assert(object.ReferenceEquals(unbounded, EdmConstants.UnboundedValue), "you must pass the unbounded value. If you are trying to set null, use the T parameter overload"); FacetValueContainer container = new FacetValueContainer (); container.SetUnbounded(); return container; } public static implicit operator FacetValueContainer (T value) { FacetValueContainer container = new FacetValueContainer (); container.Value = value; return container; } internal object GetValueAsObject() { Debug.Assert(_hasValue, "Don't get the value if it has not been set"); if (_isUnbounded) { return EdmConstants.UnboundedValue; } else { return (object)_value; } } internal bool HasValue { get { return _hasValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// This Class is never expected to be used except for by the FacetValues class. /// /// The purpose of this class is to allow strong type checking by the compiler while setting facet values which /// are typically stored as Object because they can either on of these things /// /// 1. null /// 2. scalar type (bool, int, byte) /// 3. Unbounded object /// /// without this class it would be very easy to accidentally set precision to an int when it really is supposed to be /// a byte value. Also you would be able to set the facet value to any Object derived class (ANYTHING!!!) when really only /// null and Unbounded are allowed besides an actual scalar value. The magic of the class happens in the implicit constructors with /// allow patterns like /// /// new FacetValues( MaxLength = EdmConstants.UnboundedValue, Nullable = true}; /// /// and these are type checked at compile time /// ///internal struct FacetValueContainer { T _value; bool _hasValue; bool _isUnbounded; internal T Value { set { _isUnbounded = false; _hasValue = true; _value = value; } } private void SetUnbounded() { _isUnbounded = true; _hasValue = true; } // don't add an implicit conversion from object because it will kill the compile time type checking. public static implicit operator FacetValueContainer (System.Data.Metadata.Edm.EdmConstants.Unbounded unbounded) { Debug.Assert(object.ReferenceEquals(unbounded, EdmConstants.UnboundedValue), "you must pass the unbounded value. If you are trying to set null, use the T parameter overload"); FacetValueContainer container = new FacetValueContainer (); container.SetUnbounded(); return container; } public static implicit operator FacetValueContainer (T value) { FacetValueContainer container = new FacetValueContainer (); container.Value = value; return container; } internal object GetValueAsObject() { Debug.Assert(_hasValue, "Don't get the value if it has not been set"); if (_isUnbounded) { return EdmConstants.UnboundedValue; } else { return (object)_value; } } internal bool HasValue { get { return _hasValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
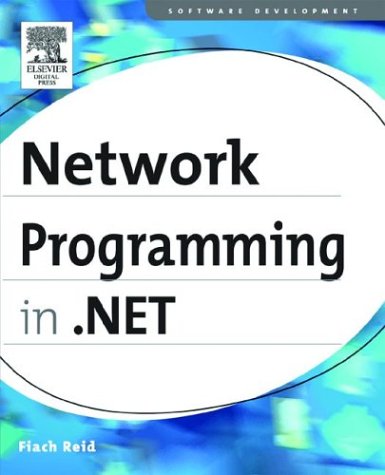
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreeDeleteContentUndoUnit.cs
- SoapUnknownHeader.cs
- SiteMapHierarchicalDataSourceView.cs
- DLinqDataModelProvider.cs
- DataTableReaderListener.cs
- RsaSecurityTokenParameters.cs
- RecognizedWordUnit.cs
- GridViewHeaderRowPresenter.cs
- CodeTypeMemberCollection.cs
- ResourceSet.cs
- Options.cs
- AutoResizedEvent.cs
- XmlRawWriter.cs
- XmlPreloadedResolver.cs
- BitStream.cs
- NativeDirectoryServicesQueryAPIs.cs
- IdentityManager.cs
- MarginCollapsingState.cs
- InputBuffer.cs
- FontStretches.cs
- TextEvent.cs
- PathFigureCollectionValueSerializer.cs
- ApplicationException.cs
- StringAnimationBase.cs
- ObfuscateAssemblyAttribute.cs
- FrameworkTextComposition.cs
- DispatcherOperation.cs
- WorkItem.cs
- StrokeCollectionDefaultValueFactory.cs
- ClientRolePrincipal.cs
- ReferencedType.cs
- XsltLoader.cs
- GregorianCalendar.cs
- ScrollableControl.cs
- InvokePattern.cs
- smtpconnection.cs
- DuplicateWaitObjectException.cs
- ProxyOperationRuntime.cs
- MDIControlStrip.cs
- ScriptResourceInfo.cs
- hwndwrapper.cs
- WindowsStatic.cs
- WaitHandleCannotBeOpenedException.cs
- TextEditorTyping.cs
- DataTable.cs
- ActiveXContainer.cs
- Bezier.cs
- AuthenticationException.cs
- ResourceDictionaryCollection.cs
- CapabilitiesRule.cs
- ListViewSortEventArgs.cs
- HeaderedContentControl.cs
- TemplateKey.cs
- InputGestureCollection.cs
- PointCollectionValueSerializer.cs
- PropertyDescriptor.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- TextBoxAutomationPeer.cs
- HtmlInputText.cs
- ClientEndpointLoader.cs
- FormsAuthenticationModule.cs
- Msec.cs
- UserControlParser.cs
- DataGridViewIntLinkedList.cs
- SecondaryIndexList.cs
- Splitter.cs
- LiteralLink.cs
- FlowDocumentFormatter.cs
- WindowsListViewItemCheckBox.cs
- ChameleonKey.cs
- safex509handles.cs
- TableRow.cs
- X509UI.cs
- GreenMethods.cs
- MaskDescriptors.cs
- RenderData.cs
- Drawing.cs
- DataMemberAttribute.cs
- WpfXamlType.cs
- RuntimeArgument.cs
- DocumentGridPage.cs
- StrongNameUtility.cs
- CompilerTypeWithParams.cs
- StrongNameHelpers.cs
- AspCompat.cs
- MtomMessageEncodingBindingElement.cs
- IdentityNotMappedException.cs
- ChangeDirector.cs
- OleDbCommand.cs
- PersonalizationEntry.cs
- FileStream.cs
- AsmxEndpointPickerExtension.cs
- CodeDelegateCreateExpression.cs
- Math.cs
- Errors.cs
- CodeRemoveEventStatement.cs
- WindowsListViewGroupHelper.cs
- ADMembershipUser.cs
- PtsHost.cs
- XmlAttributeCache.cs