Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / TemplateKey.cs / 1 / TemplateKey.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Base class for DataTemplateKey, TableTemplateKey. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.ComponentModel; using System.Windows.Markup; using MS.Internal.Data; // DataBindEngine.EnglishUSCulture namespace System.Windows { ///The TemplateKey object is used as the resource key for data templates [TypeConverter(typeof(TemplateKeyConverter))] public abstract class TemplateKey : ResourceKey, ISupportInitialize { ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType) { _dataType = null; // still needs to be initialized _templateType = templateType; } ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType, object dataType) { Exception ex = ValidateDataType(dataType, "dataType"); if (ex != null) throw ex; _dataType = dataType; _templateType = templateType; } #region ISupportInitialize ///Begin Initialization void ISupportInitialize.BeginInit() { _initializing = true; } ///End Initialization, verify that internal state is consistent void ISupportInitialize.EndInit() { if (_dataType == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "DataType", this.GetType().Name)); } _initializing = false; } #endregion ISupportInitialize ////// The type for which the template is designed. This is either /// a Type (for object data), or a string (for XML data). In the latter /// case the string denotes the XML tag name. /// public object DataType { get { return _dataType; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "DataType", this.GetType().Name)); if (_dataType != null && value != _dataType) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "DataType", this.GetType().Name)); Exception ex = ValidateDataType(value, "value"); if (ex != null) throw ex; _dataType = value; } } ///Override of Object.GetHashCode() public override int GetHashCode() { // note that the hash code can change, but only during intialization // and only once (DataType can only be changed once, from null to // non-null, and that can only happen during [Begin/End]Init). // Technically this is still a violation of the "constant during // lifetime" rule, however in practice this is acceptable. It is // very unlikely that someone will put a TemplateKey into a hashtable // before it is initialized. int hashcode = (int)_templateType; if (_dataType != null) { hashcode += _dataType.GetHashCode(); } return hashcode; } ///Override of Object.Equals() public override bool Equals(object o) { TemplateKey key = o as TemplateKey; if (key != null) { return _templateType == key._templateType && Object.Equals(_dataType, key._dataType); } return false; } ///Override of Object.ToString() public override string ToString() { Type type = DataType as Type; return (DataType != null) ? String.Format(DataBindEngine.EnglishUSCulture, "{0}({1})", this.GetType().Name, DataType) : String.Format(DataBindEngine.EnglishUSCulture, "{0}(null)", this.GetType().Name); } ////// Allows SystemResources to know which assembly the template might be defined in. /// public override Assembly Assembly { get { Type type = _dataType as Type; if (type != null) { return type.Assembly; } return null; } } ///The different types of templates that use TemplateKey protected enum TemplateType { ///DataTemplate DataTemplate, ///TableTemplate TableTemplate, } // Validate against these rules // 1. dataType must not be null (except at initialization, which is tested at EndInit) // 2. dataType must be either a Type (object data) or a string (XML tag name) // 3. dataType cannot be typeof(Object) internal static Exception ValidateDataType(object dataType, string argName) { Exception result = null; if (dataType == null) { result = new ArgumentNullException(argName); } else if (!(dataType is Type) && !(dataType is String)) { result = new ArgumentException(SR.Get(SRID.MustBeTypeOrString, dataType.GetType().Name), argName); } else if (dataType == typeof(Object)) { result = new ArgumentException(SR.Get(SRID.DataTypeCannotBeObject), argName); } return result; } object _dataType; TemplateType _templateType; bool _initializing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Base class for DataTemplateKey, TableTemplateKey. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.ComponentModel; using System.Windows.Markup; using MS.Internal.Data; // DataBindEngine.EnglishUSCulture namespace System.Windows { ///The TemplateKey object is used as the resource key for data templates [TypeConverter(typeof(TemplateKeyConverter))] public abstract class TemplateKey : ResourceKey, ISupportInitialize { ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType) { _dataType = null; // still needs to be initialized _templateType = templateType; } ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType, object dataType) { Exception ex = ValidateDataType(dataType, "dataType"); if (ex != null) throw ex; _dataType = dataType; _templateType = templateType; } #region ISupportInitialize ///Begin Initialization void ISupportInitialize.BeginInit() { _initializing = true; } ///End Initialization, verify that internal state is consistent void ISupportInitialize.EndInit() { if (_dataType == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "DataType", this.GetType().Name)); } _initializing = false; } #endregion ISupportInitialize ////// The type for which the template is designed. This is either /// a Type (for object data), or a string (for XML data). In the latter /// case the string denotes the XML tag name. /// public object DataType { get { return _dataType; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "DataType", this.GetType().Name)); if (_dataType != null && value != _dataType) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "DataType", this.GetType().Name)); Exception ex = ValidateDataType(value, "value"); if (ex != null) throw ex; _dataType = value; } } ///Override of Object.GetHashCode() public override int GetHashCode() { // note that the hash code can change, but only during intialization // and only once (DataType can only be changed once, from null to // non-null, and that can only happen during [Begin/End]Init). // Technically this is still a violation of the "constant during // lifetime" rule, however in practice this is acceptable. It is // very unlikely that someone will put a TemplateKey into a hashtable // before it is initialized. int hashcode = (int)_templateType; if (_dataType != null) { hashcode += _dataType.GetHashCode(); } return hashcode; } ///Override of Object.Equals() public override bool Equals(object o) { TemplateKey key = o as TemplateKey; if (key != null) { return _templateType == key._templateType && Object.Equals(_dataType, key._dataType); } return false; } ///Override of Object.ToString() public override string ToString() { Type type = DataType as Type; return (DataType != null) ? String.Format(DataBindEngine.EnglishUSCulture, "{0}({1})", this.GetType().Name, DataType) : String.Format(DataBindEngine.EnglishUSCulture, "{0}(null)", this.GetType().Name); } ////// Allows SystemResources to know which assembly the template might be defined in. /// public override Assembly Assembly { get { Type type = _dataType as Type; if (type != null) { return type.Assembly; } return null; } } ///The different types of templates that use TemplateKey protected enum TemplateType { ///DataTemplate DataTemplate, ///TableTemplate TableTemplate, } // Validate against these rules // 1. dataType must not be null (except at initialization, which is tested at EndInit) // 2. dataType must be either a Type (object data) or a string (XML tag name) // 3. dataType cannot be typeof(Object) internal static Exception ValidateDataType(object dataType, string argName) { Exception result = null; if (dataType == null) { result = new ArgumentNullException(argName); } else if (!(dataType is Type) && !(dataType is String)) { result = new ArgumentException(SR.Get(SRID.MustBeTypeOrString, dataType.GetType().Name), argName); } else if (dataType == typeof(Object)) { result = new ArgumentException(SR.Get(SRID.DataTypeCannotBeObject), argName); } return result; } object _dataType; TemplateType _templateType; bool _initializing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
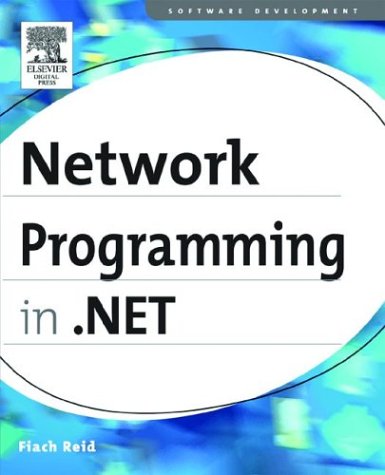
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RSAOAEPKeyExchangeDeformatter.cs
- _OverlappedAsyncResult.cs
- PropertyCollection.cs
- MenuRendererStandards.cs
- XmlBinaryReader.cs
- DataGridViewComboBoxColumn.cs
- SmiMetaDataProperty.cs
- SqlServer2KCompatibilityAnnotation.cs
- ApplicationId.cs
- HandlerBase.cs
- ResourceDefaultValueAttribute.cs
- Gdiplus.cs
- SortFieldComparer.cs
- UriTemplateTable.cs
- MetadataArtifactLoaderComposite.cs
- Expression.cs
- XmlAttributeProperties.cs
- SiteMapProvider.cs
- TransformPattern.cs
- MD5CryptoServiceProvider.cs
- WizardForm.cs
- BitmapPalettes.cs
- AggregateNode.cs
- FileFormatException.cs
- ShaperBuffers.cs
- TextEffect.cs
- PersonalizationStateInfoCollection.cs
- ValidateNames.cs
- CheckBoxFlatAdapter.cs
- _UriTypeConverter.cs
- LayoutManager.cs
- Utils.cs
- SymLanguageType.cs
- SendKeys.cs
- Light.cs
- DispatcherFrame.cs
- VariableExpressionConverter.cs
- ComboBoxItem.cs
- Tablet.cs
- ObjectStateEntry.cs
- GatewayDefinition.cs
- AsymmetricAlgorithm.cs
- ResXResourceWriter.cs
- CharacterBufferReference.cs
- MarkerProperties.cs
- Vector3DConverter.cs
- Activator.cs
- RelationshipFixer.cs
- UpDownBase.cs
- TextCharacters.cs
- AnnotationService.cs
- FactoryRecord.cs
- HttpHandlerAction.cs
- NotSupportedException.cs
- GenericEnumerator.cs
- XmlSchemaValidationException.cs
- TypeListConverter.cs
- XmlSchemaFacet.cs
- ObjectDisposedException.cs
- Mutex.cs
- MaskInputRejectedEventArgs.cs
- ConnectionConsumerAttribute.cs
- TypeLoadException.cs
- CacheVirtualItemsEvent.cs
- FixedPosition.cs
- DataMemberListEditor.cs
- WebEventTraceProvider.cs
- PassportPrincipal.cs
- Style.cs
- FormConverter.cs
- IntSecurity.cs
- HtmlHistory.cs
- DataControlButton.cs
- InvalidAsynchronousStateException.cs
- OleDbConnectionFactory.cs
- TargetControlTypeCache.cs
- PermissionListSet.cs
- DataGridViewMethods.cs
- PriorityChain.cs
- KernelTypeValidation.cs
- XamlFilter.cs
- EventRouteFactory.cs
- DeferrableContentConverter.cs
- AssemblyCache.cs
- LabelEditEvent.cs
- MaskedTextProvider.cs
- LocationSectionRecord.cs
- AddIn.cs
- filewebresponse.cs
- BatchParser.cs
- PermissionSet.cs
- SQLInt64.cs
- SetterBaseCollection.cs
- WindowsFont.cs
- RenderContext.cs
- ObjectAnimationBase.cs
- SpeakCompletedEventArgs.cs
- DescendentsWalker.cs
- DesignBindingPicker.cs
- ColorPalette.cs