Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / AsyncMethodInvoker.cs / 1 / AsyncMethodInvoker.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Description; using System.Reflection; using System.ServiceModel.Diagnostics; using System.Threading; using System.Collections; using System.Diagnostics; class AsyncMethodInvoker : IOperationInvoker { MethodInfo beginMethod; MethodInfo endMethod; InvokeBeginDelegate invokeBeginDelegate; InvokeEndDelegate invokeEndDelegate; int inputParameterCount; int outputParameterCount; public AsyncMethodInvoker(MethodInfo beginMethod, MethodInfo endMethod) { if (beginMethod == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("beginMethod")); if (endMethod == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("endMethod")); this.beginMethod = beginMethod; this.endMethod = endMethod; } public MethodInfo BeginMethod { get { return this.beginMethod; } } public MethodInfo EndMethod { get { return this.endMethod; } } public bool IsSynchronous { get { return false; } } public object[] AllocateInputs() { return EmptyArray.Allocate(this.InputParameterCount); } public object Invoke(object instance, object[] inputs, out object[] outputs) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } void CreateActivityInfo(ref ServiceModelActivity activity, ref Activity boundActivity) { if (DiagnosticUtility.ShouldUseActivity) { activity = ServiceModelActivity.CreateAsyncActivity(); TraceUtility.UpdateAsyncOperationContextWithActivity(activity); boundActivity = ServiceModelActivity.BoundOperation(activity, true); } else if (TraceUtility.ShouldPropagateActivity) { Guid activityId = ActivityIdHeader.ExtractActivityId(OperationContext.Current.IncomingMessage); if (activityId != Guid.Empty) { boundActivity = Activity.CreateActivity(activityId); } TraceUtility.UpdateAsyncOperationContextWithActivity(activityId); } } public IAsyncResult InvokeBegin(object instance, object[] inputs, AsyncCallback callback, object state) { if (instance == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxNoServiceObject))); if (inputs == null) { if (this.InputParameterCount > 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxInputParametersToServiceNull, this.InputParameterCount))); } else if (inputs.Length != this.InputParameterCount) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxInputParametersToServiceInvalid, this.InputParameterCount, inputs.Length))); if (PerformanceCounters.PerformanceCountersEnabled) { PerformanceCounters.MethodCalled(beginMethod.Name.Substring(ServiceReflector.BeginMethodNamePrefix.Length)); } IAsyncResult returnValue; try { ServiceModelActivity activity = null; Activity boundActivity = null; this.CreateActivityInfo(ref activity, ref boundActivity); using (boundActivity) { if (DiagnosticUtility.ShouldUseActivity) { string activityName = null; if (this.endMethod == null) { activityName = SR.GetString(SR.ActivityExecuteMethod, this.beginMethod.DeclaringType.FullName, this.beginMethod.Name); } else { activityName = SR.GetString(SR.ActivityExecuteAsyncMethod, this.beginMethod.DeclaringType.FullName, this.beginMethod.Name, this.endMethod.DeclaringType.FullName, this.endMethod.Name); } ServiceModelActivity.Start(activity, activityName, ActivityType.ExecuteUserCode); } returnValue = this.InvokeBeginDelegate(instance, inputs, callback, state); } ServiceModelActivity.Stop(activity); } catch (System.Security.SecurityException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(AuthorizationBehavior.CreateAccessDeniedFaultException()); } catch (Exception e) { TraceUtility.TraceUserCodeException(e, this.beginMethod); throw; } return returnValue; } void GetActivityInfo(ref ServiceModelActivity activity, ref Activity boundOperation) { if (DiagnosticUtility.ShouldUseActivity || TraceUtility.ShouldPropagateActivity) { object activityInfo = TraceUtility.ExtractAsyncOperationContextActivity(); if (activityInfo != null) { if (DiagnosticUtility.ShouldUseActivity) { activity = activityInfo as ServiceModelActivity; boundOperation = ServiceModelActivity.BoundOperation(activity, true); } else if (TraceUtility.ShouldPropagateActivity) { if (activityInfo is Guid) { Guid activityId = (Guid)activityInfo; boundOperation = Activity.CreateActivity(activityId); } } } } } public object InvokeEnd(object instance, out object[] outputs, IAsyncResult result) { object returnVal; if (instance == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxNoServiceObject))); outputs = EmptyArray.Allocate(this.OutputParameterCount); bool callFailed = true; bool callFaulted = false; try { ServiceModelActivity activity = null; Activity boundOperation = null; this.GetActivityInfo(ref activity, ref boundOperation); using (boundOperation) { returnVal = this.InvokeEndDelegate(instance, outputs, result); callFailed = false; } ServiceModelActivity.Stop(activity); } catch (System.Security.SecurityException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(AuthorizationBehavior.CreateAccessDeniedFaultException()); } catch (System.ServiceModel.FaultException) { callFaulted = true; callFailed = false; throw; } finally { if (PerformanceCounters.PerformanceCountersEnabled) { if (callFailed) { PerformanceCounters.MethodReturnedError(endMethod.Name.Substring(ServiceReflector.EndMethodNamePrefix.Length)); } else if (callFaulted) { PerformanceCounters.MethodReturnedFault(endMethod.Name.Substring(ServiceReflector.EndMethodNamePrefix.Length)); } else { PerformanceCounters.MethodReturnedSuccess(endMethod.Name.Substring(ServiceReflector.EndMethodNamePrefix.Length)); } } } return returnVal; } InvokeBeginDelegate InvokeBeginDelegate { get { EnsureIsInitialized(); return invokeBeginDelegate; } } InvokeEndDelegate InvokeEndDelegate { get { EnsureIsInitialized(); return invokeEndDelegate; } } int InputParameterCount { get { EnsureIsInitialized(); return this.inputParameterCount; } } int OutputParameterCount { get { EnsureIsInitialized(); return this.outputParameterCount; } } void EnsureIsInitialized() { if (this.invokeBeginDelegate == null) { // Only pass locals byref because InvokerUtil may store temporary results in the byref. // If two threads both reference this.count, temporary results may interact. int inputParameterCount; InvokeBeginDelegate invokeBeginDelegate = new InvokerUtil().GenerateInvokeBeginDelegate(this.beginMethod, out inputParameterCount); this.inputParameterCount = inputParameterCount; int outputParameterCount; InvokeEndDelegate invokeEndDelegate = new InvokerUtil().GenerateInvokeEndDelegate(this.endMethod, out outputParameterCount); this.outputParameterCount = outputParameterCount; this.invokeEndDelegate = invokeEndDelegate; this.invokeBeginDelegate = invokeBeginDelegate; // must set this last due to race } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
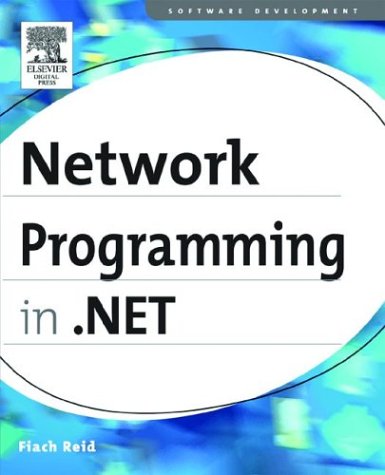
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceKey.cs
- SamlDoNotCacheCondition.cs
- LiteralControl.cs
- ToolboxCategoryItems.cs
- ProfileProvider.cs
- Html32TextWriter.cs
- ExtensionWindowHeader.cs
- LogLogRecordEnumerator.cs
- AuthenticationConfig.cs
- BrowserPolicyValidator.cs
- DataGridItemCollection.cs
- TextEndOfParagraph.cs
- EntityProxyTypeInfo.cs
- UriSection.cs
- VirtualDirectoryMapping.cs
- HelpEvent.cs
- SafeNativeMethods.cs
- FieldDescriptor.cs
- PiiTraceSource.cs
- EmptyControlCollection.cs
- XmlSerializerFactory.cs
- Journaling.cs
- XLinq.cs
- UIPropertyMetadata.cs
- NullableLongMinMaxAggregationOperator.cs
- ResourceDisplayNameAttribute.cs
- SettingsAttributes.cs
- Win32SafeHandles.cs
- ConvertEvent.cs
- URLIdentityPermission.cs
- ASCIIEncoding.cs
- PreProcessInputEventArgs.cs
- AutoGeneratedFieldProperties.cs
- DataGridViewColumnConverter.cs
- ErrorTableItemStyle.cs
- ContainerControl.cs
- AssemblyAssociatedContentFileAttribute.cs
- VisualStyleElement.cs
- ActivityStatusChangeEventArgs.cs
- TextBoxDesigner.cs
- EntityContainerAssociationSet.cs
- ParseHttpDate.cs
- TextParaLineResult.cs
- SqlDataSource.cs
- DefaultTextStore.cs
- ProfessionalColors.cs
- TypedElement.cs
- DataTableCollection.cs
- SimpleApplicationHost.cs
- WebRequestModuleElement.cs
- TextTreePropertyUndoUnit.cs
- DynamicDataManager.cs
- ToolStripHighContrastRenderer.cs
- OutgoingWebRequestContext.cs
- InternalResources.cs
- Int32AnimationUsingKeyFrames.cs
- WebPartTransformer.cs
- ScriptResourceInfo.cs
- BooleanStorage.cs
- WebBrowserNavigatedEventHandler.cs
- ReachSerializerAsync.cs
- RectValueSerializer.cs
- GridViewColumnCollection.cs
- BypassElement.cs
- NativeCppClassAttribute.cs
- ReferenceSchema.cs
- WindowPattern.cs
- TextDecorationLocationValidation.cs
- XmlBinaryReader.cs
- MexTcpBindingCollectionElement.cs
- Dictionary.cs
- DelayedRegex.cs
- CustomSignedXml.cs
- OutOfMemoryException.cs
- BitmapEncoder.cs
- Input.cs
- BitmapEffectState.cs
- EntityContainer.cs
- ReadOnlyTernaryTree.cs
- TileBrush.cs
- ImageConverter.cs
- RegionIterator.cs
- OverlappedContext.cs
- ConnectionAcceptor.cs
- OrderedDictionary.cs
- WorkerRequest.cs
- ThemeDictionaryExtension.cs
- HtmlInputButton.cs
- ToolStripKeyboardHandlingService.cs
- Size.cs
- DataGridView.cs
- XmlWrappingReader.cs
- EllipseGeometry.cs
- DynamicFilterExpression.cs
- BuildManager.cs
- DescendantOverDescendantQuery.cs
- ControlBuilder.cs
- CallbackValidatorAttribute.cs
- SynchronizedInputPattern.cs
- TimeSpanValidator.cs