Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / TextTreeExtractElementUndoUnit.cs / 1 / TextTreeExtractElementUndoUnit.cs
//---------------------------------------------------------------------------- // // File: TextTreeExtractElementUndoUnit.cs // // Description: Undo unit for TextContainer.ExtractElement calls. // // History: // 03/03/2004 : benwest - Created // //--------------------------------------------------------------------------- using System; using MS.Internal; namespace System.Windows.Documents { // Undo unit for TextContainer.ExtractElement calls. internal class TextTreeExtractElementUndoUnit : TextTreeUndoUnit { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Creates a new undo unit instance. internal TextTreeExtractElementUndoUnit(TextContainer tree, TextTreeTextElementNode elementNode) : base(tree, elementNode.GetSymbolOffset(tree.Generation)) { _symbolCount = elementNode.SymbolCount; _type = elementNode.TextElement.GetType(); _localValues = LocalValueEnumeratorToArray(elementNode.TextElement.GetLocalValueEnumerator()); _resources = elementNode.TextElement.Resources; // Table requires additional work for storing its Columns collection if (elementNode.TextElement is Table) { _columns = TextTreeDeleteContentUndoUnit.SaveColumns((Table)elementNode.TextElement); } } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods // Called by the undo manager. Restores tree state to its condition // when the unit was created. Assumes the tree state matches conditions // just after the unit was created. public override void DoCore() { TextPointer start; TextPointer end; TextElement element; VerifyTreeContentHashCode(); start = new TextPointer(this.TextContainer, this.SymbolOffset, LogicalDirection.Forward); end = new TextPointer(this.TextContainer, this.SymbolOffset + _symbolCount - 2, LogicalDirection.Forward); // Insert a new element. element = (TextElement)Activator.CreateInstance(_type); element.Reposition(start, end); // Restore local resources element.Resources = _resources; // Move end into the scope of the new element. end.MoveToNextContextPosition(LogicalDirection.Backward); // Then restore local property values. // this.TextContainer.SetValues(end, ArrayToLocalValueEnumerator(_localValues)); if (element is Table) { TextTreeDeleteContentUndoUnit.RestoreColumns((Table)element, _columns); } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // Count of symbols covered by the extracted TextElement, including its 2 edges. private readonly int _symbolCount; // Type of the extracted TextElement. private readonly Type _type; // Collection of all local property values set on the extracted TextElement. private readonly PropertyRecord []_localValues; // Resources defined locally on the TextElement private readonly ResourceDictionary _resources; // TableColumns collection private readonly TableColumn[] _columns; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextTreeExtractElementUndoUnit.cs // // Description: Undo unit for TextContainer.ExtractElement calls. // // History: // 03/03/2004 : benwest - Created // //--------------------------------------------------------------------------- using System; using MS.Internal; namespace System.Windows.Documents { // Undo unit for TextContainer.ExtractElement calls. internal class TextTreeExtractElementUndoUnit : TextTreeUndoUnit { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Creates a new undo unit instance. internal TextTreeExtractElementUndoUnit(TextContainer tree, TextTreeTextElementNode elementNode) : base(tree, elementNode.GetSymbolOffset(tree.Generation)) { _symbolCount = elementNode.SymbolCount; _type = elementNode.TextElement.GetType(); _localValues = LocalValueEnumeratorToArray(elementNode.TextElement.GetLocalValueEnumerator()); _resources = elementNode.TextElement.Resources; // Table requires additional work for storing its Columns collection if (elementNode.TextElement is Table) { _columns = TextTreeDeleteContentUndoUnit.SaveColumns((Table)elementNode.TextElement); } } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods // Called by the undo manager. Restores tree state to its condition // when the unit was created. Assumes the tree state matches conditions // just after the unit was created. public override void DoCore() { TextPointer start; TextPointer end; TextElement element; VerifyTreeContentHashCode(); start = new TextPointer(this.TextContainer, this.SymbolOffset, LogicalDirection.Forward); end = new TextPointer(this.TextContainer, this.SymbolOffset + _symbolCount - 2, LogicalDirection.Forward); // Insert a new element. element = (TextElement)Activator.CreateInstance(_type); element.Reposition(start, end); // Restore local resources element.Resources = _resources; // Move end into the scope of the new element. end.MoveToNextContextPosition(LogicalDirection.Backward); // Then restore local property values. // this.TextContainer.SetValues(end, ArrayToLocalValueEnumerator(_localValues)); if (element is Table) { TextTreeDeleteContentUndoUnit.RestoreColumns((Table)element, _columns); } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // Count of symbols covered by the extracted TextElement, including its 2 edges. private readonly int _symbolCount; // Type of the extracted TextElement. private readonly Type _type; // Collection of all local property values set on the extracted TextElement. private readonly PropertyRecord []_localValues; // Resources defined locally on the TextElement private readonly ResourceDictionary _resources; // TableColumns collection private readonly TableColumn[] _columns; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
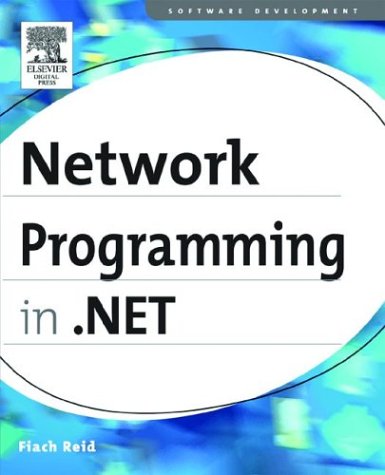
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditingCoordinator.cs
- WindowsSysHeader.cs
- BoundField.cs
- NativeRightsManagementAPIsStructures.cs
- GestureRecognitionResult.cs
- CodeSubDirectoriesCollection.cs
- ProgressChangedEventArgs.cs
- metadatamappinghashervisitor.cs
- CaseInsensitiveComparer.cs
- Int64KeyFrameCollection.cs
- SettingsBase.cs
- DetailsViewUpdatedEventArgs.cs
- KnowledgeBase.cs
- RewritingProcessor.cs
- RegexCapture.cs
- BinaryMessageFormatter.cs
- ElementProxy.cs
- ThreadNeutralSemaphore.cs
- GridViewCancelEditEventArgs.cs
- RoutedEventHandlerInfo.cs
- ObjectStateEntry.cs
- NetworkInformationPermission.cs
- ConnectionModeReader.cs
- SmiEventSink.cs
- ReadOnlyObservableCollection.cs
- ListViewDeleteEventArgs.cs
- CustomWebEventKey.cs
- FileUpload.cs
- ProgressChangedEventArgs.cs
- NavigationPropertyEmitter.cs
- ActivityExecutor.cs
- WeakReference.cs
- FunctionDefinition.cs
- XsdSchemaFileEditor.cs
- FixUpCollection.cs
- RefType.cs
- SafeMemoryMappedFileHandle.cs
- ProcessManager.cs
- ListDesigner.cs
- ApplicationManager.cs
- CultureInfoConverter.cs
- WebBrowserEvent.cs
- DataControlCommands.cs
- ThreadAbortException.cs
- DetailsViewModeEventArgs.cs
- WebPartEditorApplyVerb.cs
- PrintDialog.cs
- TriggerCollection.cs
- SqlCaseSimplifier.cs
- OperationAbortedException.cs
- ZipFileInfo.cs
- FormViewUpdateEventArgs.cs
- ConfigXmlWhitespace.cs
- SyntaxCheck.cs
- Stream.cs
- basenumberconverter.cs
- WindowsTokenRoleProvider.cs
- TheQuery.cs
- VirtualPathUtility.cs
- PlainXmlDeserializer.cs
- ResourceDescriptionAttribute.cs
- WizardForm.cs
- CodeGotoStatement.cs
- CreateRefExpr.cs
- DataSourceControl.cs
- ScrollPattern.cs
- SynchronizationLockException.cs
- OleDbTransaction.cs
- XslTransform.cs
- ToggleButtonAutomationPeer.cs
- TreeViewTemplateSelector.cs
- ActiveDesignSurfaceEvent.cs
- BehaviorEditorPart.cs
- SponsorHelper.cs
- DesignerToolboxInfo.cs
- IntSecurity.cs
- AttachedPropertiesService.cs
- XPathEmptyIterator.cs
- ByeOperationCD1AsyncResult.cs
- PolygonHotSpot.cs
- ConnectionStringSettings.cs
- DataServiceQuery.cs
- WebControlAdapter.cs
- DecimalConstantAttribute.cs
- Guid.cs
- TreeView.cs
- DataGridViewHitTestInfo.cs
- ListView.cs
- IssuedSecurityTokenProvider.cs
- TypeUsage.cs
- HtmlElementEventArgs.cs
- SparseMemoryStream.cs
- Timer.cs
- DataRelation.cs
- XmlSerializationGeneratedCode.cs
- TabletCollection.cs
- ActivityCodeGenerator.cs
- BuiltInPermissionSets.cs
- AutoCompleteStringCollection.cs
- ParagraphResult.cs