Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / RoutedEventHandlerInfo.cs / 1 / RoutedEventHandlerInfo.cs
using System; namespace System.Windows { ////// Container for handler instance and other /// invocation preferences for this handler /// instance /// ////// RoutedEventHandlerInfo constitutes the /// handler instance and flag that indicates if /// or not this handler must be invoked for /// already handled events //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] public struct RoutedEventHandlerInfo { #region Construction ////// /// /// This class needs to be public because it is /// used by ContentElement in the Framework /// to store Instance EventHandlers /// /// Construtor for RoutedEventHandlerInfo /// /// /// Non-null handler /// /// /// Flag that indicates if or not the handler must /// be invoked for already handled events /// internal RoutedEventHandlerInfo(Delegate handler, bool handledEventsToo) { _handler = handler; _handledEventsToo = handledEventsToo; } #endregion Construction #region Operations ////// Returns associated handler instance /// public Delegate Handler { get {return _handler;} } ////// Returns HandledEventsToo Flag /// public bool InvokeHandledEventsToo { get {return _handledEventsToo;} } // Invokes handler instance as per specified // invocation preferences internal void InvokeHandler(object target, RoutedEventArgs routedEventArgs) { if ((routedEventArgs.Handled == false) || (_handledEventsToo == true)) { if (_handler is RoutedEventHandler) { // Generic RoutedEventHandler is called directly here since // we don't need the InvokeEventHandler override to cast to // the proper type - we know what it is. ((RoutedEventHandler)_handler)(target, routedEventArgs); } else { // NOTE: Cannot call protected method InvokeEventHandler directly routedEventArgs.InvokeHandler(_handler, target); } } } ////// Is the given object equivalent to the current one /// public override bool Equals(object obj) { if (obj == null || !(obj is RoutedEventHandlerInfo)) return false; return Equals((RoutedEventHandlerInfo)obj); } ////// Is the given RoutedEventHandlerInfo equals the current /// public bool Equals(RoutedEventHandlerInfo handlerInfo) { return _handler == handlerInfo._handler && _handledEventsToo == handlerInfo._handledEventsToo; } ////// Serves as a hash function for a particular type, suitable for use in /// hashing algorithms and data structures like a hash table /// public override int GetHashCode() { return base.GetHashCode(); } ////// Equals operator overload /// public static bool operator== (RoutedEventHandlerInfo handlerInfo1, RoutedEventHandlerInfo handlerInfo2) { return handlerInfo1.Equals(handlerInfo2); } ////// NotEquals operator overload /// public static bool operator!= (RoutedEventHandlerInfo handlerInfo1, RoutedEventHandlerInfo handlerInfo2) { return !handlerInfo1.Equals(handlerInfo2); } ////// Cleanup all the references within the data /// /* Commented out to avoid "uncalled private code" fxcop violation internal void Clear() { _handler = null; _handledEventsToo = false; } */ #endregion Operations #region Data private Delegate _handler; private bool _handledEventsToo; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows { ////// Container for handler instance and other /// invocation preferences for this handler /// instance /// ////// RoutedEventHandlerInfo constitutes the /// handler instance and flag that indicates if /// or not this handler must be invoked for /// already handled events //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] public struct RoutedEventHandlerInfo { #region Construction ////// /// /// This class needs to be public because it is /// used by ContentElement in the Framework /// to store Instance EventHandlers /// /// Construtor for RoutedEventHandlerInfo /// /// /// Non-null handler /// /// /// Flag that indicates if or not the handler must /// be invoked for already handled events /// internal RoutedEventHandlerInfo(Delegate handler, bool handledEventsToo) { _handler = handler; _handledEventsToo = handledEventsToo; } #endregion Construction #region Operations ////// Returns associated handler instance /// public Delegate Handler { get {return _handler;} } ////// Returns HandledEventsToo Flag /// public bool InvokeHandledEventsToo { get {return _handledEventsToo;} } // Invokes handler instance as per specified // invocation preferences internal void InvokeHandler(object target, RoutedEventArgs routedEventArgs) { if ((routedEventArgs.Handled == false) || (_handledEventsToo == true)) { if (_handler is RoutedEventHandler) { // Generic RoutedEventHandler is called directly here since // we don't need the InvokeEventHandler override to cast to // the proper type - we know what it is. ((RoutedEventHandler)_handler)(target, routedEventArgs); } else { // NOTE: Cannot call protected method InvokeEventHandler directly routedEventArgs.InvokeHandler(_handler, target); } } } ////// Is the given object equivalent to the current one /// public override bool Equals(object obj) { if (obj == null || !(obj is RoutedEventHandlerInfo)) return false; return Equals((RoutedEventHandlerInfo)obj); } ////// Is the given RoutedEventHandlerInfo equals the current /// public bool Equals(RoutedEventHandlerInfo handlerInfo) { return _handler == handlerInfo._handler && _handledEventsToo == handlerInfo._handledEventsToo; } ////// Serves as a hash function for a particular type, suitable for use in /// hashing algorithms and data structures like a hash table /// public override int GetHashCode() { return base.GetHashCode(); } ////// Equals operator overload /// public static bool operator== (RoutedEventHandlerInfo handlerInfo1, RoutedEventHandlerInfo handlerInfo2) { return handlerInfo1.Equals(handlerInfo2); } ////// NotEquals operator overload /// public static bool operator!= (RoutedEventHandlerInfo handlerInfo1, RoutedEventHandlerInfo handlerInfo2) { return !handlerInfo1.Equals(handlerInfo2); } ////// Cleanup all the references within the data /// /* Commented out to avoid "uncalled private code" fxcop violation internal void Clear() { _handler = null; _handledEventsToo = false; } */ #endregion Operations #region Data private Delegate _handler; private bool _handledEventsToo; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
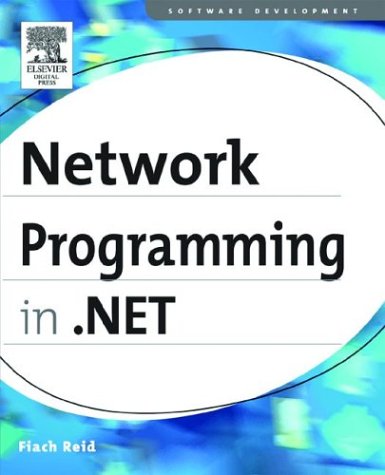
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintControllerWithStatusDialog.cs
- FontStretchConverter.cs
- XmlNodeComparer.cs
- COM2FontConverter.cs
- InputLangChangeEvent.cs
- Icon.cs
- CapabilitiesRule.cs
- Choices.cs
- LocalizeDesigner.cs
- FunctionCommandText.cs
- SByteStorage.cs
- WebPartConnectionsDisconnectVerb.cs
- DataGridView.cs
- OleTxTransaction.cs
- SimpleHandlerBuildProvider.cs
- EventDescriptorCollection.cs
- RequestSecurityTokenResponse.cs
- NullExtension.cs
- CrossSiteScriptingValidation.cs
- FigureHelper.cs
- WebServiceParameterData.cs
- XmlBaseWriter.cs
- TraceSource.cs
- SafeNativeMethodsCLR.cs
- IERequestCache.cs
- IdentityHolder.cs
- DataSourceControlBuilder.cs
- CodePageEncoding.cs
- RecordManager.cs
- CryptoApi.cs
- CreateUserWizardStep.cs
- HttpApplicationFactory.cs
- EditorAttribute.cs
- WsatConfiguration.cs
- WebPartUserCapability.cs
- StateFinalizationActivity.cs
- CheckPair.cs
- OrderingInfo.cs
- SchemaTableOptionalColumn.cs
- Int64.cs
- infer.cs
- CharEnumerator.cs
- Set.cs
- ProbeMatchesMessageCD1.cs
- TimeSpanMinutesConverter.cs
- FormViewUpdatedEventArgs.cs
- WorkflowDefinitionContext.cs
- OleDbException.cs
- PerformanceCounterManager.cs
- LogExtent.cs
- EntityCommandExecutionException.cs
- JoinSymbol.cs
- CustomAttributeFormatException.cs
- ApplicationActivator.cs
- AtomicFile.cs
- ipaddressinformationcollection.cs
- Int32Rect.cs
- TemplateControlCodeDomTreeGenerator.cs
- FontWeightConverter.cs
- FusionWrap.cs
- GuidConverter.cs
- EventSetter.cs
- InputProviderSite.cs
- SectionVisual.cs
- MappingModelBuildProvider.cs
- MailBnfHelper.cs
- Latin1Encoding.cs
- BoolLiteral.cs
- TextEditorSelection.cs
- FilteredReadOnlyMetadataCollection.cs
- _AutoWebProxyScriptEngine.cs
- DrawToolTipEventArgs.cs
- LinqToSqlWrapper.cs
- SqlCaseSimplifier.cs
- DependencyObject.cs
- SiteMap.cs
- ExceptionValidationRule.cs
- DocumentSequence.cs
- UpDownEvent.cs
- NavigationProperty.cs
- ToolboxItemImageConverter.cs
- DesignerObjectListAdapter.cs
- ObjectTag.cs
- fixedPageContentExtractor.cs
- QueryResultOp.cs
- HttpListener.cs
- ZoomPercentageConverter.cs
- DataSourceGeneratorException.cs
- ToolStripContentPanelRenderEventArgs.cs
- HandlerMappingMemo.cs
- TypeLoadException.cs
- JsonMessageEncoderFactory.cs
- HttpWriter.cs
- AssemblyAttributesGoHere.cs
- BrowserTree.cs
- Pens.cs
- ValidatingPropertiesEventArgs.cs
- ToolStripItemBehavior.cs
- RecommendedAsConfigurableAttribute.cs
- RightsManagementPermission.cs