Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ChooseAction.cs / 1305376 / ChooseAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ChooseAction : ContainerAction { internal override void Compile(Compiler compiler) { CompileAttributes(compiler); if (compiler.Recurse()) { CompileConditions(compiler); compiler.ToParent(); } } private void CompileConditions(Compiler compiler) { NavigatorInput input = compiler.Input; bool when = false; bool otherwise = false; do { switch (input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Ref.Equal(nspace, input.Atoms.UriXsl)) { IfAction action = null; if (Ref.Equal(name, input.Atoms.When)) { if (otherwise) { throw XsltException.Create(Res.Xslt_WhenAfterOtherwise); } action = compiler.CreateIfAction(IfAction.ConditionType.ConditionWhen); when = true; } else if (Ref.Equal(name, input.Atoms.Otherwise)) { if (otherwise) { throw XsltException.Create(Res.Xslt_DupOtherwise); } action = compiler.CreateIfAction(IfAction.ConditionType.ConditionOtherwise); otherwise = true; } else { throw compiler.UnexpectedKeyword(); } AddAction(action); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, "choose"); } } while (compiler.Advance()); if (! when) { throw XsltException.Create(Res.Xslt_NoWhen); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ChooseAction : ContainerAction { internal override void Compile(Compiler compiler) { CompileAttributes(compiler); if (compiler.Recurse()) { CompileConditions(compiler); compiler.ToParent(); } } private void CompileConditions(Compiler compiler) { NavigatorInput input = compiler.Input; bool when = false; bool otherwise = false; do { switch (input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Ref.Equal(nspace, input.Atoms.UriXsl)) { IfAction action = null; if (Ref.Equal(name, input.Atoms.When)) { if (otherwise) { throw XsltException.Create(Res.Xslt_WhenAfterOtherwise); } action = compiler.CreateIfAction(IfAction.ConditionType.ConditionWhen); when = true; } else if (Ref.Equal(name, input.Atoms.Otherwise)) { if (otherwise) { throw XsltException.Create(Res.Xslt_DupOtherwise); } action = compiler.CreateIfAction(IfAction.ConditionType.ConditionOtherwise); otherwise = true; } else { throw compiler.UnexpectedKeyword(); } AddAction(action); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, "choose"); } } while (compiler.Advance()); if (! when) { throw XsltException.Create(Res.Xslt_NoWhen); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
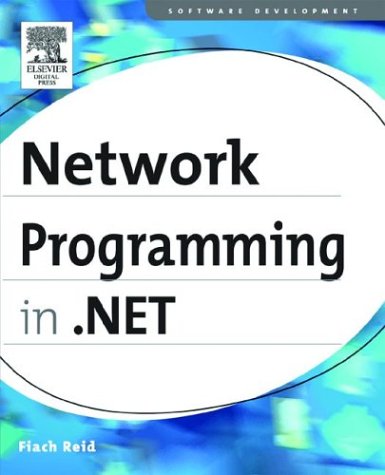
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeAttributeDeclaration.cs
- DrawingAttributes.cs
- ScriptResourceHandler.cs
- OutputCacheSettings.cs
- CollectionViewGroupRoot.cs
- TableChangeProcessor.cs
- WindowProviderWrapper.cs
- URI.cs
- KeyFrames.cs
- TemporaryBitmapFile.cs
- SoapExtension.cs
- MenuItem.cs
- LayoutUtils.cs
- HtmlSelect.cs
- SelectionChangedEventArgs.cs
- ElementFactory.cs
- SpeechRecognitionEngine.cs
- D3DImage.cs
- DesignTimeTemplateParser.cs
- TypeReference.cs
- StringBuilder.cs
- ButtonBaseAdapter.cs
- ControlUtil.cs
- TaskFileService.cs
- MDIClient.cs
- RefreshPropertiesAttribute.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- StorageMappingFragment.cs
- TypeInitializationException.cs
- ParallelTimeline.cs
- PenCursorManager.cs
- AssemblyAssociatedContentFileAttribute.cs
- UInt16.cs
- PassportAuthenticationEventArgs.cs
- ObjectItemCachedAssemblyLoader.cs
- StringAnimationUsingKeyFrames.cs
- AssemblyName.cs
- DetailsViewDeletedEventArgs.cs
- HttpStreamXmlDictionaryWriter.cs
- VectorCollection.cs
- UpdateCommand.cs
- EndEvent.cs
- DefaultValueAttribute.cs
- ScrollBarRenderer.cs
- TableCellCollection.cs
- DependentList.cs
- Certificate.cs
- WebBrowserDocumentCompletedEventHandler.cs
- AsyncPostBackErrorEventArgs.cs
- ColorContextHelper.cs
- MissingManifestResourceException.cs
- SqlAggregateChecker.cs
- WebControlsSection.cs
- SqlInternalConnectionSmi.cs
- TextDpi.cs
- UserControlBuildProvider.cs
- ReadOnlyAttribute.cs
- XmlConvert.cs
- WindowsPrincipal.cs
- EventLogPermissionEntry.cs
- BlurEffect.cs
- DataGridViewCellStyleChangedEventArgs.cs
- StringUtil.cs
- HMACSHA384.cs
- CodePageUtils.cs
- ConfigurationLocationCollection.cs
- TokenBasedSet.cs
- XsltContext.cs
- WebPartUtil.cs
- UriScheme.cs
- WebRequestModuleElement.cs
- AspProxy.cs
- COM2ColorConverter.cs
- Schema.cs
- FileEnumerator.cs
- WebConfigurationManager.cs
- DoubleCollectionConverter.cs
- EventManager.cs
- PeerApplicationLaunchInfo.cs
- TypeListConverter.cs
- ButtonBase.cs
- MSAAWinEventWrap.cs
- DataObjectMethodAttribute.cs
- HostingEnvironmentSection.cs
- WebPartEventArgs.cs
- RadioButtonFlatAdapter.cs
- TreeBuilder.cs
- XmlAtomicValue.cs
- CodeObject.cs
- ContentValidator.cs
- SizeConverter.cs
- StackSpiller.Generated.cs
- ListView.cs
- DocumentScope.cs
- CheckBoxList.cs
- isolationinterop.cs
- NullExtension.cs
- TextElement.cs
- GenericRootAutomationPeer.cs
- StrongNameIdentityPermission.cs