Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Build / MS / Internal / Tasks / TaskFileService.cs / 1 / TaskFileService.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // File service for the input source files in a build task. // // The service does something like below: // // return stream for a given source file, // get last modification time in memory or disk. // // or get the link metadata part of a source file. // // It returns above information no matter the task is hosted // inside VS or not. // // History: // 01/24/2007, [....] created it // 03/28/2007, [....] added support of Exists, Delete, PutFileContents, IsRealBuild // //--------------------------------------------------------------------------- using System; using System.IO; using Microsoft.Build.Utilities; using System.Diagnostics; using System.Text; namespace MS.Internal { // // Declaration of interface ITaskFileService // internal interface ITaskFileService { // // Get content stream for a given source file // Stream GetContent(string srcFile); // // Get the last modificatin time for a given file. // DateTime GetLastChangeTime(string srcFile); // // Checks to see if file exists // bool Exists(string fileName); // // Deletes the specified file // void Delete(string fileName); // // Save content stream for the given destination file // void WriteFile(byte[] contentArray, string destinationFile); // // Determines if this is a real build operation or an // intellisense build operation // bool IsRealBuild {get;} } // // TaskFileService class // // This class can be used by different build tasks to get source file // service, the instance is created by those build tasks when task's // Execute( ) method is called. // internal class TaskFileService : MarshalByRefObject, ITaskFileService { // // Ctor // public TaskFileService(Task buildTask) { _buildTask = buildTask; _hostFileManager = null; _isRealBuild = null; } #region ITaskFileService // // Get content stream for a given source file, the content could // come from memory or disk based on the build host environment. // // It is the caller's responsibility to close the stream. // public Stream GetContent(string srcFile) { Stream fileStream = null; if (String.IsNullOrEmpty(srcFile)) { throw new ArgumentNullException("srcFile"); } if (HostFileManager != null) { // // Build Host environment has a FileManager, use it to get // file content from edit buffer in memory. GetFileContents // removes the BOM before returning the string. // string strFileContent = HostFileManager.GetFileContents(srcFile); // IVsMsBuildTaskFileManager.GetFileContents should never return null. // GetBytes might throw when input is null, but that should be fine. // // For xaml file, UTF8 is the standard encoding // UTF8Encoding utf8Encoding = new UTF8Encoding(); byte[] baFileContent = utf8Encoding.GetBytes(strFileContent); fileStream = new MemoryStream(baFileContent); } else { fileStream = File.OpenRead(srcFile); } return fileStream; } // // Get the last modificatin time for a given file. // public DateTime GetLastChangeTime(string srcFile) { DateTime lastChangeDT = new DateTime(0); if (String.IsNullOrEmpty(srcFile)) { throw new ArgumentNullException("srcFile"); } if (HostFileManager != null) { long fileTime = HostFileManager.GetFileLastChangeTime(srcFile); lastChangeDT = DateTime.FromFileTime(fileTime); } else { lastChangeDT = File.GetLastWriteTime(srcFile); } return lastChangeDT; } // // Checks to see if file exists // public bool Exists(string fileName) { bool fileExists = false; if (fileName == null) { throw new ArgumentNullException("fileName"); } if (HostFileManager != null) { fileExists = HostFileManager.Exists(fileName, IsRealBuild); } else { fileExists = File.Exists(fileName); } return fileExists; } // // Deletes the specified file // public void Delete(string fileName) { if (fileName == null) { throw new ArgumentNullException("fileName"); } if (HostFileManager != null) { HostFileManager.Delete(fileName); } else { File.Delete(fileName); } } // // Save content stream for the given destination file // UTF8 BOM should not be added to the contentArray. This // method adds BOM before writing file to disk. // public void WriteFile(byte[] contentArray, string destinationFile) { if (String.IsNullOrEmpty(destinationFile)) { throw new ArgumentNullException("destinationFile"); } if (contentArray == null) { throw new ArgumentNullException("contentArray"); } UTF8Encoding utf8Encoding = new UTF8Encoding(); string contentStr = utf8Encoding.GetString(contentArray); if (HostFileManager != null) { // PutGeneratedFileContents adds BOM to the file when saving // to memory or disk. HostFileManager.PutGeneratedFileContents(destinationFile, contentStr); } else { // Add BOM for UTF8Encoding since the input contentArray is not supposed to // have it already. using (StreamWriter sw = new StreamWriter(destinationFile, false, new UTF8Encoding(true))) { sw.WriteLine(contentStr); } } } // // Tells if this is an intellisense build or a real build. // Caching the result since this is called a lot of times. // public bool IsRealBuild { get { if (_isRealBuild == null) { bool isRealBuild = true; if (HostFileManager != null) { isRealBuild = HostFileManager.IsRealBuildOperation(); } _isRealBuild = isRealBuild; } return _isRealBuild.Value; } } #endregion ITaskFileService #region private property // // Get the FileManager implemented by the Host system // private IVsMSBuildTaskFileManager HostFileManager { get { if (_hostFileManager == null) { if (_buildTask != null && _buildTask.HostObject != null) { _hostFileManager = _buildTask.HostObject as IVsMSBuildTaskFileManager; } } return _hostFileManager; } } #endregion private property #region private data field private Task _buildTask; private IVsMSBuildTaskFileManager _hostFileManager; private Nullable_isRealBuild; #endregion private data field } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
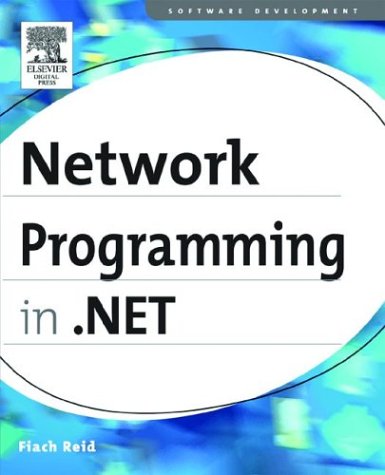
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpModuleAction.cs
- PeerNearMe.cs
- DataTableExtensions.cs
- _SslState.cs
- MdiWindowListStrip.cs
- ConfigurationPropertyAttribute.cs
- IteratorDescriptor.cs
- ItemsControl.cs
- UIAgentMonitor.cs
- LassoSelectionBehavior.cs
- CounterCreationDataCollection.cs
- StreamResourceInfo.cs
- WorkflowDispatchContext.cs
- ExternalException.cs
- coordinatorfactory.cs
- SqlDependencyListener.cs
- Int32Rect.cs
- Trace.cs
- Axis.cs
- ImageClickEventArgs.cs
- ErrorRuntimeConfig.cs
- CodeDOMUtility.cs
- DecimalStorage.cs
- OpacityConverter.cs
- LayoutTable.cs
- ParameterRetriever.cs
- SortedList.cs
- Section.cs
- ConfigurationManagerHelper.cs
- SessionStateContainer.cs
- ErrorLog.cs
- AccessDataSourceDesigner.cs
- CodeTypeConstructor.cs
- DefaultValueTypeConverter.cs
- DecimalAnimation.cs
- RoleService.cs
- WebControl.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- ContentControl.cs
- IgnorePropertiesAttribute.cs
- NonPrimarySelectionGlyph.cs
- DbParameterCollectionHelper.cs
- SiteMap.cs
- HtmlButton.cs
- DataGridViewColumnCollectionEditor.cs
- FormatterConverter.cs
- ValidationRuleCollection.cs
- Funcletizer.cs
- XmlSchemaException.cs
- ProfileEventArgs.cs
- X509Chain.cs
- SimpleModelProvider.cs
- ResolveMatches11.cs
- IdentityModelDictionary.cs
- RemoteWebConfigurationHost.cs
- WebHttpBinding.cs
- XmlRootAttribute.cs
- DataControlButton.cs
- ClientApiGenerator.cs
- DefaultEventAttribute.cs
- Exceptions.cs
- SuppressMergeCheckAttribute.cs
- TableAutomationPeer.cs
- DecimalConstantAttribute.cs
- ToolbarAUtomationPeer.cs
- BindingContext.cs
- XmlEncoding.cs
- AmbientProperties.cs
- ByteStreamMessage.cs
- srgsitem.cs
- DataGridSortCommandEventArgs.cs
- Attributes.cs
- StrongNamePublicKeyBlob.cs
- ViewUtilities.cs
- OracleColumn.cs
- SubstitutionResponseElement.cs
- FontUnit.cs
- TextEditorCharacters.cs
- EditorZoneBase.cs
- HMACSHA384.cs
- SQLDateTime.cs
- WebPartCloseVerb.cs
- Context.cs
- SignedPkcs7.cs
- SrgsSemanticInterpretationTag.cs
- HttpHandlerActionCollection.cs
- ClickablePoint.cs
- RegionData.cs
- SystemIcons.cs
- RawAppCommandInputReport.cs
- AdRotator.cs
- InputBinding.cs
- RevocationPoint.cs
- GridViewAutomationPeer.cs
- DataGridViewRowStateChangedEventArgs.cs
- NetworkInterface.cs
- XmlTextAttribute.cs
- WebExceptionStatus.cs
- UnmanagedMarshal.cs
- OdbcConnectionString.cs