Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Command / InputBinding.cs / 1 / InputBinding.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; // SecurityCritical, TreatAsSafe using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.ComponentModel; namespace System.Windows.Input { ////// InputBinding - InputGesture and ICommand combination /// Used to specify the binding between Gesture and Command at Element level. /// public class InputBinding : DependencyObject, ICommandSource { #region Constructor ////// Default Constructor - needed to allow markup creation /// protected InputBinding() { } ////// Constructor /// /// Command /// Input Gesture ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical] public InputBinding(ICommand command, InputGesture gesture) { if (command == null) throw new ArgumentNullException("command"); if (gesture == null) throw new ArgumentNullException("gesture"); // Check before assignment to avoid continuation CheckSecureCommand(command, gesture); _command = command; _gesture = gesture; } #endregion Constructor //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Command Object associated /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return _command; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(value, _gesture); _command = value; } } } ////// A parameter for the command. /// public object CommandParameter { get { return _commandParameter; } set { lock (_dataLock) { _commandParameter = value; } } } ////// Where the command should be raised. /// public IInputElement CommandTarget { get { return _commandTarget; } set { lock (_dataLock) { _commandTarget = value; } } } ////// InputGesture associated with the Command /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// public virtual InputGesture Gesture { // We would like to make this getter non-virtual but that's not legal // in C#. Luckily there is no security issue with leaving it virtual. get { return _gesture; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(_command, value); _gesture = value; } } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Critical - determines if a command will later make an /// assert. This is critical to be right, because /// later we assume that the binding was protected. /// TreatAsSafe - Demand() is not an unsafe operation /// [SecurityCritical, SecurityTreatAsSafe] void CheckSecureCommand(ICommand command, InputGesture gesture) { ISecureCommand secure = command as ISecureCommand; if (secure != null) { secure.UserInitiatedPermission.Demand(); } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private ICommand _command = null ; private InputGesture _gesture = null ; private object _commandParameter; private IInputElement _commandTarget; internal static object _dataLock = new object(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
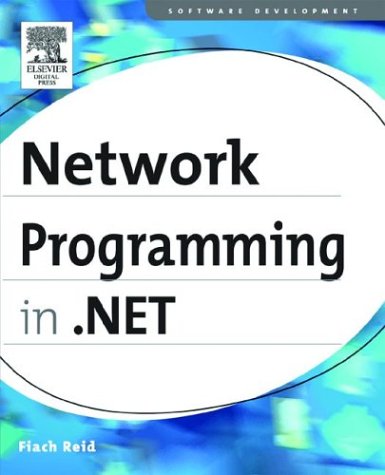
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeToken.cs
- TextAutomationPeer.cs
- CurrentChangedEventManager.cs
- DockProviderWrapper.cs
- TextEditorTables.cs
- EtwTrace.cs
- BamlLocalizationDictionary.cs
- CodeExpressionStatement.cs
- GuidTagList.cs
- TextServicesDisplayAttributePropertyRanges.cs
- ScrollItemPatternIdentifiers.cs
- MethodImplAttribute.cs
- DocumentPageViewAutomationPeer.cs
- Crypto.cs
- XmlObjectSerializerReadContextComplex.cs
- QualifiedCellIdBoolean.cs
- ThreadPool.cs
- DesignColumnCollection.cs
- IResourceProvider.cs
- WebMethodAttribute.cs
- SocketPermission.cs
- WebConvert.cs
- TrackingConditionCollection.cs
- TextWriterTraceListener.cs
- FullTrustAssembliesSection.cs
- ImageUrlEditor.cs
- indexingfiltermarshaler.cs
- AsyncCompletedEventArgs.cs
- XmlILModule.cs
- WebPartConnectVerb.cs
- CompositeActivityValidator.cs
- ObjectDataSourceEventArgs.cs
- MultipartContentParser.cs
- TextEditorThreadLocalStore.cs
- RemoveStoryboard.cs
- ResourceProperty.cs
- HwndSubclass.cs
- RoutedEvent.cs
- RichTextBoxAutomationPeer.cs
- EditorPartChrome.cs
- SqlNode.cs
- CalculatedColumn.cs
- DataGridViewSortCompareEventArgs.cs
- GridViewDeletedEventArgs.cs
- CodeAttributeDeclaration.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- ElementAction.cs
- SmiGettersStream.cs
- MatrixTransform3D.cs
- BaseParser.cs
- AnnotationResource.cs
- ElementAction.cs
- XmlSchemaGroupRef.cs
- BitmapEffectDrawing.cs
- FamilyMap.cs
- SchemaLookupTable.cs
- XPathDocumentIterator.cs
- StreamReader.cs
- NetMsmqSecurityElement.cs
- SessionSwitchEventArgs.cs
- KnownIds.cs
- EdmValidator.cs
- _ConnectOverlappedAsyncResult.cs
- RpcCryptoContext.cs
- SourceFileBuildProvider.cs
- TableLayoutColumnStyleCollection.cs
- PenLineCapValidation.cs
- InsufficientMemoryException.cs
- AssemblyAttributes.cs
- TextComposition.cs
- WeakReadOnlyCollection.cs
- WorkItem.cs
- HttpApplication.cs
- TaskFormBase.cs
- WindowManager.cs
- DockEditor.cs
- AnnotationAuthorChangedEventArgs.cs
- LambdaCompiler.Binary.cs
- PolicyChain.cs
- WorkflowTimerService.cs
- EventDriven.cs
- CompileXomlTask.cs
- DocumentSequenceHighlightLayer.cs
- DataSourceControl.cs
- NameTable.cs
- MatrixTransform3D.cs
- ADConnectionHelper.cs
- ManifestSignedXml.cs
- SymmetricAlgorithm.cs
- PropertyManager.cs
- WindowsToolbarItemAsMenuItem.cs
- SortQuery.cs
- NativeRightsManagementAPIsStructures.cs
- SafeNativeMethods.cs
- ReadWriteControlDesigner.cs
- ForceCopyBuildProvider.cs
- WebPartHelpVerb.cs
- SmtpFailedRecipientException.cs
- AspNetCompatibilityRequirementsAttribute.cs
- DbParameterHelper.cs