Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RpcCryptoContext.cs / 1 / RpcCryptoContext.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.ComponentModel; using System.IO; using System.Runtime.InteropServices; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // The context class for perfoming RpcCrypto operations. // internal class RpcCryptoContext : IDisposable { string m_portName; string m_contextKey; IntPtr m_idlHandle; object m_syncRoot; bool m_open; bool m_disposed; // // Summary: // Creates a new RpcCryptoContext // // Arguments: // portName: The name of the port // contextKey: The name of the context // public RpcCryptoContext( string portName, string contextKey ) { m_portName = portName; m_contextKey = contextKey; m_syncRoot = new object(); m_disposed = false; } // // Summary: // DTOR // ~RpcCryptoContext() { if( !m_disposed ) { ((IDisposable)this).Dispose(); } } // // Summary: // Gets the ContextKye. // public string ContextKey { get{ return m_contextKey; } } // // Summary: // Gets the native IdlHandle // public IntPtr InterfaceHandle { get{ return m_idlHandle; } } // // Summary: // Indicates if the context is still open. // public bool IsOpen { get{ return m_open; } } // // Summary: // Opens the context. // public void Open() { ThrowIfDisposed(); if( !m_open ) { try { m_idlHandle = NativeMcppMethods.RpcCryptoOpen( m_portName ); } catch( Win32Exception we ) { throw IDT.ThrowHelperError( new CommunicationException( null, we ) ); } m_open = true; } } // // Summary: // Closes the context. // public void Close() { if( m_open ) { try { NativeMcppMethods.RpcCryptoClose( m_idlHandle, m_contextKey ); } catch( Win32Exception we ) { throw IDT.ThrowHelperError( new CommunicationException( null, we ) ); } m_idlHandle = IntPtr.Zero; m_open = false; } } // // Summary: // Disposes the object if not alreay done. // void IDisposable.Dispose() { if( !m_disposed ) { lock( m_syncRoot ) { if( !m_disposed ) { m_disposed = true; try { Close(); } catch( CommunicationException ie ) { // // In case the RPC interface on the agent had already shutdown, // we will get this exception from Close( ) above. Dispose is NOT // expected to throw, so catch this specific exception here. // string msg = ie.Message; IDT.TraceDebug( "Ignoring failure in RpcCryptoContext Close(): " + msg ); } m_portName = null; m_contextKey = null; m_idlHandle = IntPtr.Zero; GC.SuppressFinalize( this ); } } } } void ThrowIfDisposed() { if( m_disposed ) { throw IDT.ThrowHelperError( new ObjectDisposedException( "RpcCryptoContext" ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
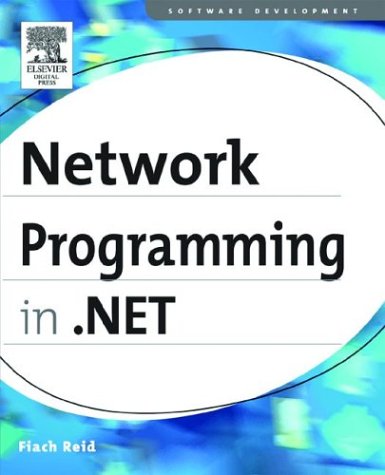
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vector3DCollectionConverter.cs
- SequentialUshortCollection.cs
- DbParameterCollectionHelper.cs
- DataGridViewRowCollection.cs
- UntypedNullExpression.cs
- ClientScriptManager.cs
- MetaModel.cs
- TypedReference.cs
- ControlUtil.cs
- CompositeControlDesigner.cs
- ErrorTableItemStyle.cs
- SelectionRange.cs
- MissingMethodException.cs
- ToolStripButton.cs
- XPathDocumentIterator.cs
- WindowsGraphics.cs
- EventProxy.cs
- _BasicClient.cs
- ItemMap.cs
- ServicesUtilities.cs
- ExpanderAutomationPeer.cs
- bidPrivateBase.cs
- WebBrowserNavigatedEventHandler.cs
- DBProviderConfigurationHandler.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ToolBarOverflowPanel.cs
- HtmlAnchor.cs
- NullableConverter.cs
- OneOfConst.cs
- ComContractElementCollection.cs
- RadioButtonPopupAdapter.cs
- ProfessionalColors.cs
- VirtualDirectoryMapping.cs
- ListControlDataBindingHandler.cs
- CollectionViewGroupInternal.cs
- SecuritySessionServerSettings.cs
- HashAlgorithm.cs
- TypeGeneratedEventArgs.cs
- NullableDoubleSumAggregationOperator.cs
- RedBlackList.cs
- ProxyElement.cs
- ScalarRestriction.cs
- UnsafeNativeMethods.cs
- PropertyConverter.cs
- DataStreams.cs
- Brushes.cs
- OleDbRowUpdatedEvent.cs
- DataBoundControlHelper.cs
- ClientOptions.cs
- DoubleAnimationUsingPath.cs
- DataRelationCollection.cs
- DataRelation.cs
- OleDbStruct.cs
- LinkConverter.cs
- IncrementalHitTester.cs
- MembershipValidatePasswordEventArgs.cs
- HelpEvent.cs
- AspNetSynchronizationContext.cs
- TextElementEnumerator.cs
- StreamSecurityUpgradeInitiatorBase.cs
- DoubleLinkListEnumerator.cs
- ToolTip.cs
- WinFormsUtils.cs
- DataGridViewLayoutData.cs
- RoutedEventHandlerInfo.cs
- DataList.cs
- Vector3dCollection.cs
- ScrollEvent.cs
- AuthorizationRuleCollection.cs
- Profiler.cs
- DeferredElementTreeState.cs
- BinaryNode.cs
- TargetConverter.cs
- PrintPageEvent.cs
- OdbcException.cs
- NTAccount.cs
- Single.cs
- NumberEdit.cs
- DrawTreeNodeEventArgs.cs
- Symbol.cs
- VectorAnimationBase.cs
- CLRBindingWorker.cs
- BinaryObjectReader.cs
- XmlSchemaCollection.cs
- TextBox.cs
- SourceFileInfo.cs
- InternalDispatchObject.cs
- DataGridHeaderBorder.cs
- SqlParameterizer.cs
- ElementFactory.cs
- DataGridViewButtonColumn.cs
- DecodeHelper.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- TypeNameParser.cs
- AudioException.cs
- CollectionViewSource.cs
- RegularExpressionValidator.cs
- ListViewAutomationPeer.cs
- SelectedDatesCollection.cs
- BlockCollection.cs