Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Internal / SequentialUshortCollection.cs / 1305600 / SequentialUshortCollection.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollectionfor a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollection for a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
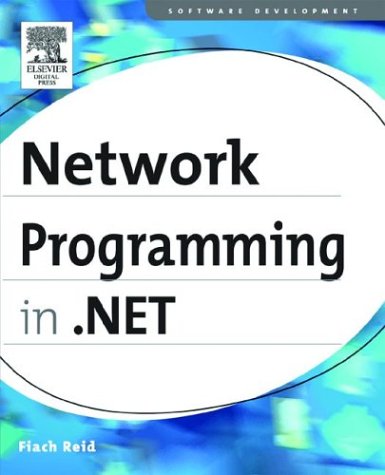
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadPool.cs
- ComponentConverter.cs
- SpeechUI.cs
- UriParserTemplates.cs
- DesignTimeTemplateParser.cs
- UserControlFileEditor.cs
- XPathConvert.cs
- ListItemsPage.cs
- SimpleBitVector32.cs
- EndOfStreamException.cs
- ToolConsole.cs
- Single.cs
- BreakSafeBase.cs
- ImpersonationOption.cs
- MediaSystem.cs
- SafeNativeMethods.cs
- TextContainerHelper.cs
- Int16Storage.cs
- HtmlInputButton.cs
- CollectionEditor.cs
- WindowsListViewGroupHelper.cs
- NoneExcludedImageIndexConverter.cs
- MimePart.cs
- LogReservationCollection.cs
- AuthenticationException.cs
- PlatformCulture.cs
- RectIndependentAnimationStorage.cs
- TrackingParameters.cs
- RuntimeEnvironment.cs
- TouchPoint.cs
- SqlServer2KCompatibilityCheck.cs
- MarshalByRefObject.cs
- XmlObjectSerializerReadContextComplexJson.cs
- DictionaryEditChange.cs
- EntityDataSourceDesignerHelper.cs
- EntityTypeEmitter.cs
- FigureHelper.cs
- ReturnEventArgs.cs
- FormsAuthenticationModule.cs
- LoadedOrUnloadedOperation.cs
- Brushes.cs
- IsolatedStorageFileStream.cs
- GridViewDeleteEventArgs.cs
- ExecutionEngineException.cs
- WmlPhoneCallAdapter.cs
- DataObjectCopyingEventArgs.cs
- SubstitutionResponseElement.cs
- PeerNode.cs
- WpfWebRequestHelper.cs
- Select.cs
- IsolatedStorageException.cs
- SamlAdvice.cs
- Int32Rect.cs
- MultiByteCodec.cs
- GroupItemAutomationPeer.cs
- Dump.cs
- ValueSerializer.cs
- SQLDouble.cs
- DependencyPropertyDescriptor.cs
- ExecutedRoutedEventArgs.cs
- Rules.cs
- HwndSubclass.cs
- SafePipeHandle.cs
- ProtocolsConfigurationEntry.cs
- CalloutQueueItem.cs
- RelationshipConstraintValidator.cs
- WindowsToolbar.cs
- JumpPath.cs
- CopyAttributesAction.cs
- SecurityValidationBehavior.cs
- NotCondition.cs
- InternalTypeHelper.cs
- CodeTryCatchFinallyStatement.cs
- EdmPropertyAttribute.cs
- ElementFactory.cs
- _NTAuthentication.cs
- Parser.cs
- ScrollViewerAutomationPeer.cs
- SrgsOneOf.cs
- StringExpressionSet.cs
- Point4D.cs
- MULTI_QI.cs
- SqlTriggerAttribute.cs
- CodeArrayCreateExpression.cs
- IProducerConsumerCollection.cs
- StringComparer.cs
- Source.cs
- ClientTargetSection.cs
- UIElementIsland.cs
- ToolStripDesignerUtils.cs
- ConditionalAttribute.cs
- PipelineModuleStepContainer.cs
- ContainerParagraph.cs
- PermissionSet.cs
- StrongTypingException.cs
- BinaryReader.cs
- ToolStripSeparator.cs
- ScrollViewerAutomationPeer.cs
- SQLDouble.cs
- PeerInputChannel.cs