Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Log / System / IO / Log / LogReservationCollection.cs / 1305376 / LogReservationCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IO.Log { using System; using System.IO.Log; // NOTE: The reservations in the collection do not account for // header sizes, out of necessity. The reservations made // with the record sequence *do* account for header sizes. // // In order to maintain sanity, the record sequence only // deals with reservations that include the header // size. i.e., if it goes into CLFS, it has the header size // appended to it. // // So that means: If we receive a size from the collection, // add the header size to it before returning it. If we // receive a size from our code, subtract the header size // before giving it to the collection. // // Keep this straight, or else. // sealed class LogReservationCollection : ReservationCollection { LogRecordSequence recordSequence; internal LogReservationCollection(LogRecordSequence sequence) { this.recordSequence = sequence; } internal LogRecordSequence RecordSequence { get { return this.recordSequence; } } internal long GetMatchingReservation(long size) { // Reservation coming from CLFS, subtract record header // size. // size -= LogLogRecordHeader.Size; size = GetBestMatchingReservation(size); if (size == -1) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ReservationNotFound()); // Reservation coming from the collection, add record // header size. // size += LogLogRecordHeader.Size; return size; } internal void InternalAddReservation(long size) { // Reservation coming from CLFS, remove record header // size. // size -= LogLogRecordHeader.Size; ReservationMade(size); } protected override void MakeReservation(long size) { if (size < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentOutOfRange("size")); // Reservation coming from collection, add record header // size. // size += LogLogRecordHeader.Size; long aligned; UnsafeNativeMethods.AlignReservedLogSingle( this.recordSequence.MarshalContext, size, out aligned); UnsafeNativeMethods.AllocReservedLog( this.recordSequence.MarshalContext, 1, ref aligned); } protected override void FreeReservation(long size) { if (size < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentOutOfRange("size")); lock(this.recordSequence.LogStore.SyncRoot) { SafeMarshalContext context = this.recordSequence.InternalMarshalContext ; if(context == null || context.IsInvalid) { return; } // Reservation coming from collection, add record header // size. // size += LogLogRecordHeader.Size; long aligned; UnsafeNativeMethods.AlignReservedLogSingle( context, size, out aligned); // Adjustment must be negative, otherwise it's considered // a "set". (Yuck.) // aligned = -aligned; UnsafeNativeMethods.FreeReservedLog( context, 1, ref aligned); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IO.Log { using System; using System.IO.Log; // NOTE: The reservations in the collection do not account for // header sizes, out of necessity. The reservations made // with the record sequence *do* account for header sizes. // // In order to maintain sanity, the record sequence only // deals with reservations that include the header // size. i.e., if it goes into CLFS, it has the header size // appended to it. // // So that means: If we receive a size from the collection, // add the header size to it before returning it. If we // receive a size from our code, subtract the header size // before giving it to the collection. // // Keep this straight, or else. // sealed class LogReservationCollection : ReservationCollection { LogRecordSequence recordSequence; internal LogReservationCollection(LogRecordSequence sequence) { this.recordSequence = sequence; } internal LogRecordSequence RecordSequence { get { return this.recordSequence; } } internal long GetMatchingReservation(long size) { // Reservation coming from CLFS, subtract record header // size. // size -= LogLogRecordHeader.Size; size = GetBestMatchingReservation(size); if (size == -1) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ReservationNotFound()); // Reservation coming from the collection, add record // header size. // size += LogLogRecordHeader.Size; return size; } internal void InternalAddReservation(long size) { // Reservation coming from CLFS, remove record header // size. // size -= LogLogRecordHeader.Size; ReservationMade(size); } protected override void MakeReservation(long size) { if (size < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentOutOfRange("size")); // Reservation coming from collection, add record header // size. // size += LogLogRecordHeader.Size; long aligned; UnsafeNativeMethods.AlignReservedLogSingle( this.recordSequence.MarshalContext, size, out aligned); UnsafeNativeMethods.AllocReservedLog( this.recordSequence.MarshalContext, 1, ref aligned); } protected override void FreeReservation(long size) { if (size < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentOutOfRange("size")); lock(this.recordSequence.LogStore.SyncRoot) { SafeMarshalContext context = this.recordSequence.InternalMarshalContext ; if(context == null || context.IsInvalid) { return; } // Reservation coming from collection, add record header // size. // size += LogLogRecordHeader.Size; long aligned; UnsafeNativeMethods.AlignReservedLogSingle( context, size, out aligned); // Adjustment must be negative, otherwise it's considered // a "set". (Yuck.) // aligned = -aligned; UnsafeNativeMethods.FreeReservedLog( context, 1, ref aligned); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
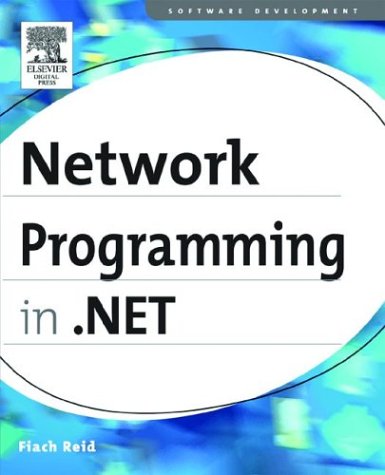
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandleRef.cs
- CultureInfoConverter.cs
- _StreamFramer.cs
- CalendarDataBindingHandler.cs
- ExpressionBindingCollection.cs
- ProfileManager.cs
- QueryRewriter.cs
- PartitionResolver.cs
- ErrorProvider.cs
- SecureStringHasher.cs
- BrowserCapabilitiesCodeGenerator.cs
- OdbcTransaction.cs
- SafeHandles.cs
- BehaviorEditorPart.cs
- UseAttributeSetsAction.cs
- StylusEditingBehavior.cs
- SyncMethodInvoker.cs
- VirtualPath.cs
- COM2EnumConverter.cs
- ConfigXmlCDataSection.cs
- DataGridViewColumnHeaderCell.cs
- ProcessProtocolHandler.cs
- SQLInt32.cs
- Solver.cs
- OpCodes.cs
- SeverityFilter.cs
- SelectedDatesCollection.cs
- ReferenceTypeElement.cs
- MasterPage.cs
- ModelItemExtensions.cs
- PageTheme.cs
- SignedInfo.cs
- ExtendedProtectionPolicyTypeConverter.cs
- UriTemplateLiteralQueryValue.cs
- MenuTracker.cs
- SpellerStatusTable.cs
- LayoutTableCell.cs
- FunctionNode.cs
- Descriptor.cs
- ConfigurationValue.cs
- TextOutput.cs
- Internal.cs
- LongValidatorAttribute.cs
- TagPrefixAttribute.cs
- DataSourceHelper.cs
- DataGridViewSelectedCellCollection.cs
- WebBrowserNavigatedEventHandler.cs
- PingOptions.cs
- UTF32Encoding.cs
- PopOutPanel.cs
- Tag.cs
- TokenizerHelper.cs
- DesignerDataView.cs
- RuleProcessor.cs
- MaterialCollection.cs
- AppSettingsExpressionBuilder.cs
- NCryptSafeHandles.cs
- __Error.cs
- Rect3DValueSerializer.cs
- _AutoWebProxyScriptEngine.cs
- UnknownWrapper.cs
- AtomPub10CategoriesDocumentFormatter.cs
- EventListener.cs
- GlyphsSerializer.cs
- TemplateBaseAction.cs
- JournalNavigationScope.cs
- ColorDialog.cs
- System.Data_BID.cs
- TabItemAutomationPeer.cs
- SoapUnknownHeader.cs
- XmlSchemaImport.cs
- remotingproxy.cs
- SafeNativeMethods.cs
- MaskInputRejectedEventArgs.cs
- XslAstAnalyzer.cs
- InkCanvasSelectionAdorner.cs
- PointLight.cs
- ObjectItemAttributeAssemblyLoader.cs
- IUnknownConstantAttribute.cs
- XsltLibrary.cs
- WinFormsSecurity.cs
- BehaviorEditorPart.cs
- NegotiationTokenProvider.cs
- BaseComponentEditor.cs
- _TransmitFileOverlappedAsyncResult.cs
- TrustManager.cs
- Menu.cs
- StringCollectionMarkupSerializer.cs
- SqlExpressionNullability.cs
- SingleObjectCollection.cs
- ActivityBindForm.Designer.cs
- DesignerGeometryHelper.cs
- Group.cs
- ToolboxItemAttribute.cs
- XmlLangPropertyAttribute.cs
- InvokeGenerator.cs
- DependencyPropertyHelper.cs
- InertiaRotationBehavior.cs
- SwitchLevelAttribute.cs
- RoleGroupCollection.cs