Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewSelectedCellCollection.cs / 1 / DataGridViewSelectedCellCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IListRepresents a collection of selected ///objects in the /// control. implementation ] public class DataGridViewSelectedCellCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedCellCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewCell this[int index] { get { return (DataGridViewCell) this.items[index]; } } /// /// /// internal int Add(DataGridViewCell dataGridViewCell) { Debug.Assert(!Contains(dataGridViewCell)); return this.items.Add(dataGridViewCell); } /* Not used for now internal void AddRange(DataGridViewCell[] dataGridViewCells) { Debug.Assert(dataGridViewCells != null); foreach(DataGridViewCell dataGridViewCell in dataGridViewCells) { Debug.Assert(!Contains(dataGridViewCell)); this.items.Add(dataGridViewCell); } } internal void AddCellCollection(DataGridViewSelectedCellCollection dataGridViewCells) { Debug.Assert(dataGridViewCells != null); foreach(DataGridViewCell dataGridViewCell in dataGridViewCells) { Debug.Assert(!Contains(dataGridViewCell)); this.items.Add(dataGridViewCell); } } */ ///Adds a ///to this collection. /// /// internal void AddCellLinkedList(DataGridViewCellLinkedList dataGridViewCells) { Debug.Assert(dataGridViewCells != null); foreach (DataGridViewCell dataGridViewCell in dataGridViewCells) { Debug.Assert(!Contains(dataGridViewCell)); this.items.Add(dataGridViewCell); } } ///Adds all the ///objects from the provided linked list to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewCell dataGridViewCell) { return this.items.IndexOf(dataGridViewCell) != -1; } ///public void CopyTo(DataGridViewCell[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewCell dataGridViewCell) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
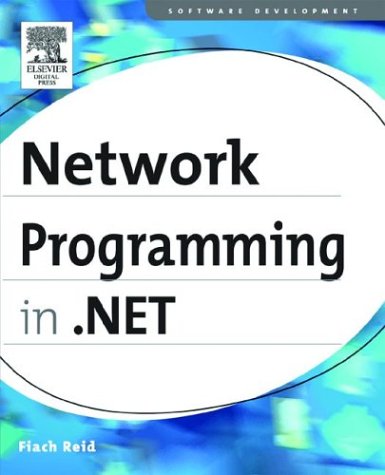
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeMethods.cs
- XamlRtfConverter.cs
- DataColumnMappingCollection.cs
- X509ThumbprintKeyIdentifierClause.cs
- SHA1Cng.cs
- GlyphRun.cs
- PartitionedStream.cs
- BStrWrapper.cs
- SpeechAudioFormatInfo.cs
- PeerEndPoint.cs
- TagMapCollection.cs
- VerificationAttribute.cs
- EventWaitHandleSecurity.cs
- CharacterString.cs
- CodeDefaultValueExpression.cs
- HtmlWindowCollection.cs
- EnvelopedPkcs7.cs
- EntityWrapper.cs
- basecomparevalidator.cs
- SoapWriter.cs
- ObjectSet.cs
- XamlWriter.cs
- DataSpaceManager.cs
- EpmSyndicationContentDeSerializer.cs
- AsymmetricAlgorithm.cs
- Bidi.cs
- WebBrowser.cs
- SerializationInfoEnumerator.cs
- ToolStripItemGlyph.cs
- TextServicesPropertyRanges.cs
- Form.cs
- ZipQueryOperator.cs
- PersonalizationEntry.cs
- SourceItem.cs
- TrackBar.cs
- DSASignatureDeformatter.cs
- UInt32.cs
- SystemWebCachingSectionGroup.cs
- LocalizedNameDescriptionPair.cs
- DirectoryInfo.cs
- DataGridViewRowHeaderCell.cs
- OpCopier.cs
- DropTarget.cs
- StateMachineTimers.cs
- Vector3DAnimationBase.cs
- StrongNamePublicKeyBlob.cs
- CuspData.cs
- SQLInt32.cs
- IisTraceWebEventProvider.cs
- DrawingAttributesDefaultValueFactory.cs
- MDIWindowDialog.cs
- SQLDateTime.cs
- ReadWriteSpinLock.cs
- EnvironmentPermission.cs
- StringValueSerializer.cs
- RsaSecurityTokenAuthenticator.cs
- MemoryRecordBuffer.cs
- FormCollection.cs
- XmlChoiceIdentifierAttribute.cs
- ISFClipboardData.cs
- SchemaNames.cs
- ObjectConverter.cs
- MenuAdapter.cs
- FlatButtonAppearance.cs
- HttpModule.cs
- precedingsibling.cs
- ImageButton.cs
- TimeSpanMinutesConverter.cs
- SpellCheck.cs
- PenLineJoinValidation.cs
- TextCollapsingProperties.cs
- LogicalChannel.cs
- DateTime.cs
- FixedElement.cs
- TrackBar.cs
- HttpValueCollection.cs
- InputMethodStateChangeEventArgs.cs
- ToolStripDropDownClosedEventArgs.cs
- SchemeSettingElement.cs
- ListCollectionView.cs
- ListManagerBindingsCollection.cs
- QilTypeChecker.cs
- XslAst.cs
- EndOfStreamException.cs
- ContextProperty.cs
- IgnoreDeviceFilterElementCollection.cs
- BehaviorEditorPart.cs
- Compiler.cs
- PageAsyncTask.cs
- OdbcConnectionOpen.cs
- HttpCapabilitiesEvaluator.cs
- X509ChainElement.cs
- JoinElimination.cs
- SoapIgnoreAttribute.cs
- XamlStackWriter.cs
- RuntimeConfigLKG.cs
- TCEAdapterGenerator.cs
- MailSettingsSection.cs
- MultipartContentParser.cs
- xsdvalidator.cs