Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Security / RightsManagement / LocalizedNameDescriptionPair.cs / 1305600 / LocalizedNameDescriptionPair.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents an immutable pair of Strings (Name, Description) // That are generally used to represent name and description of an unsigned publish license // (a.k.a. template). Unsigned Publish License has property called LocalizedNameDescriptionDictionary // which holds a map of a local Id to a Name Description pair, in order to support scenarios of // building locale specific template browsing applications. // // History: // 11/14/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// LocalizedNameDescriptionPair class represent an immutable (Name, Description) pair of strings. This is /// a basic building block for structures that need to express locale specific information about /// Unsigned Publish Licenses. /// ////// Critical: This class exposes access to methods that eventually do one or more of the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attrbiute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class LocalizedNameDescriptionPair { ////// Constructor for the read only LocalizedNameDescriptionPair class. It takes values for Name and Description as parameters. /// public LocalizedNameDescriptionPair(string name, string description) { SecurityHelper.DemandRightsManagementPermission(); if (name == null) { throw new ArgumentNullException("name"); } if (description == null) { throw new ArgumentNullException("description"); } _name = name; _description = description; } ////// Read only Name property. /// public string Name { get { SecurityHelper.DemandRightsManagementPermission(); return _name; } } ////// Read only Description property. /// public string Description { get { SecurityHelper.DemandRightsManagementPermission(); return _description; } } ////// Test for equality. /// public override bool Equals(object obj) { SecurityHelper.DemandRightsManagementPermission(); if ((obj == null) || (obj.GetType() != GetType())) { return false; } LocalizedNameDescriptionPair localizedNameDescr = obj as LocalizedNameDescriptionPair; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 return (String.CompareOrdinal(localizedNameDescr.Name, Name) == 0) && (String.CompareOrdinal(localizedNameDescr.Description, Description) == 0); #pragma warning restore 6506 } ////// Compute hash code. /// public override int GetHashCode() { SecurityHelper.DemandRightsManagementPermission(); return Name.GetHashCode() ^ Description.GetHashCode(); } private string _name; private string _description; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
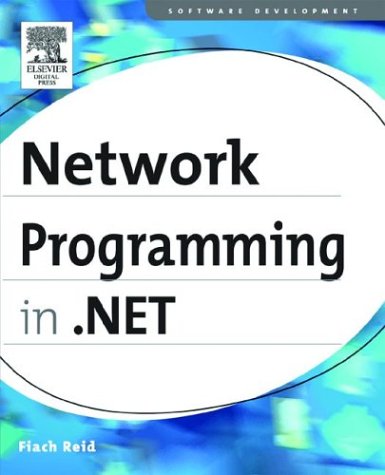
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PermissionRequestEvidence.cs
- XhtmlConformanceSection.cs
- ObjRef.cs
- PathSegmentCollection.cs
- EntitySqlQueryCacheEntry.cs
- PropertyEmitterBase.cs
- PeerNode.cs
- SpStreamWrapper.cs
- httpserverutility.cs
- WebPartsPersonalization.cs
- TdsParserSafeHandles.cs
- ByteStorage.cs
- Token.cs
- DataTemplate.cs
- DataListItem.cs
- Color.cs
- TextProviderWrapper.cs
- SoapSchemaMember.cs
- MsmqSecureHashAlgorithm.cs
- HttpAsyncResult.cs
- HatchBrush.cs
- CmsInterop.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- AlignmentYValidation.cs
- Clock.cs
- xml.cs
- StaticResourceExtension.cs
- ConditionalAttribute.cs
- ObjectStateEntryDbDataRecord.cs
- ToggleProviderWrapper.cs
- RenameRuleObjectDialog.cs
- EntityViewGenerator.cs
- PowerStatus.cs
- CustomAttributeFormatException.cs
- NewArray.cs
- DefaultSection.cs
- ReferentialConstraintRoleElement.cs
- SHA1Cng.cs
- Int64AnimationUsingKeyFrames.cs
- CachedBitmap.cs
- DataTemplateSelector.cs
- HttpListenerContext.cs
- CommandManager.cs
- UserPreference.cs
- AssemblyInfo.cs
- Queue.cs
- SiteMapNodeItem.cs
- SqlHelper.cs
- GeneratedCodeAttribute.cs
- HyperLink.cs
- DefaultPrintController.cs
- PanelDesigner.cs
- MouseButtonEventArgs.cs
- ConfigurationStrings.cs
- ResourceIDHelper.cs
- EdmScalarPropertyAttribute.cs
- ImageUrlEditor.cs
- BookmarkOptionsHelper.cs
- XmlWhitespace.cs
- EntitySqlQueryBuilder.cs
- OledbConnectionStringbuilder.cs
- FileDialogCustomPlace.cs
- FullTextBreakpoint.cs
- CodeIterationStatement.cs
- PageWrapper.cs
- ProfileSettingsCollection.cs
- XmlProcessingInstruction.cs
- ErrorInfoXmlDocument.cs
- AvTraceFormat.cs
- DropDownList.cs
- CharacterString.cs
- ECDiffieHellmanPublicKey.cs
- RelationshipConverter.cs
- PropertyPathWorker.cs
- FileDialogCustomPlace.cs
- GeometryGroup.cs
- OleDbSchemaGuid.cs
- WhitespaceRuleLookup.cs
- HiddenFieldPageStatePersister.cs
- ImportedNamespaceContextItem.cs
- PropertyIDSet.cs
- PathNode.cs
- ChangeConflicts.cs
- IDQuery.cs
- CapabilitiesPattern.cs
- XmlAttributeAttribute.cs
- Polyline.cs
- MouseEvent.cs
- DocumentApplicationJournalEntry.cs
- PathData.cs
- Style.cs
- RadioButtonAutomationPeer.cs
- UpdatePanel.cs
- PointCollection.cs
- StandardOleMarshalObject.cs
- ToolboxItemLoader.cs
- LineMetrics.cs
- AggregatePushdown.cs
- TreeNodeBindingCollection.cs
- DropTarget.cs