Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / StaticResourceExtension.cs / 1 / StaticResourceExtension.cs
/****************************************************************************\ * * File: StaticResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Reflection; using MS.Internal; namespace System.Windows { ////// Class for Xaml markup extension for static resource references. /// [MarkupExtensionReturnType(typeof(object))] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public class StaticResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public StaticResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For StaticResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { IProvideValueTarget provideValueTarget = null; IBamlReader bamlReader = null; if( serviceProvider != null ) { provideValueTarget = serviceProvider.GetService(typeof(IProvideValueTarget)) as IProvideValueTarget; bamlReader = serviceProvider.GetService(typeof(IBamlReader)) as IBamlReader; } if( bamlReader == null ) { throw new InvalidOperationException((SR.Get(SRID.StaticResourceInXamlOnly))); } if( provideValueTarget == null ) { throw new InvalidOperationException( SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IProvideValueTarget" )); } return ProvideValueInternal( bamlReader, provideValueTarget.TargetObject, provideValueTarget.TargetProperty, false /*allowDeferredReference*/); } internal object ProvideValueInternal( IBamlReader bamlReader, object targetObject, object targetProperty, bool allowDeferredReference) { if (ResourceKey == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionResourceKey)); } // Return a DeferredReference when set on a DependencyProperty in a Style bool deferReference = allowDeferredReference && (targetObject is FrameworkElementFactory || targetObject is Setter || targetObject is SharedDp); // Get prefetchedValue DeferredResourceReference prefetchedValue = PrefetchedValue; bool isValidPrefetchedValue = prefetchedValue != null && !prefetchedValue.IsUnset; // Find value BamlRecordReader bamlRecordReader = bamlReader.GetBamlReader() as BamlRecordReader; object value; if (prefetchedValue != null) { // If we have a prefetched value then we we need to look only in the parser stack not in the App or the theme. value = bamlRecordReader.FindResourceInParserStack(ResourceKey, deferReference, false/*mustReturnDeferredResourceReference*/); } else { // If we do not have a prefetched value it is a regular staticresource lookup and hence we must look in the parser stack and the app and the theme. value = bamlRecordReader.FindResourceInParentChain(ResourceKey, deferReference, false /*mustReturnDeferredResourceReference*/); } if (value == DependencyProperty.UnsetValue) { // If both the currently fetched value and the previously fetched value indicate that the // resource has not been found then it is time to throw an exception. if (!isValidPrefetchedValue) { bamlRecordReader.ThrowException(SRID.ParserNoResource , "{" + ResourceKey.ToString() + "}"); } else { // These prefetched values need to be tested for sharedness. Eg. They // could be refering to a resource marked x:Shared="false". In those // cases each reference must yeild a new instance of the resource. prefetchedValue.CheckIfShared = true; if (!deferReference) { // Inflate the prefetched deferred resource reference, because the // current context does not support using deferred references. value = prefetchedValue.GetValue(BaseValueSourceInternal.Unknown); } else { // Use the prefetched value as is value = prefetchedValue; } } } return value; } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } ////// This value is used to resolved StaticResourceIds /// internal virtual DeferredResourceReference PrefetchedValue { get { return null; } } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StaticResourceExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Reflection; using MS.Internal; namespace System.Windows { ////// Class for Xaml markup extension for static resource references. /// [MarkupExtensionReturnType(typeof(object))] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public class StaticResourceExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticResourceExtension() { } ////// Constructor that takes the resource key that this is a static reference to. /// public StaticResourceExtension( object resourceKey) { if (resourceKey == null) { throw new ArgumentNullException("resourceKey"); } _resourceKey = resourceKey; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For StaticResourceExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { IProvideValueTarget provideValueTarget = null; IBamlReader bamlReader = null; if( serviceProvider != null ) { provideValueTarget = serviceProvider.GetService(typeof(IProvideValueTarget)) as IProvideValueTarget; bamlReader = serviceProvider.GetService(typeof(IBamlReader)) as IBamlReader; } if( bamlReader == null ) { throw new InvalidOperationException((SR.Get(SRID.StaticResourceInXamlOnly))); } if( provideValueTarget == null ) { throw new InvalidOperationException( SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IProvideValueTarget" )); } return ProvideValueInternal( bamlReader, provideValueTarget.TargetObject, provideValueTarget.TargetProperty, false /*allowDeferredReference*/); } internal object ProvideValueInternal( IBamlReader bamlReader, object targetObject, object targetProperty, bool allowDeferredReference) { if (ResourceKey == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionResourceKey)); } // Return a DeferredReference when set on a DependencyProperty in a Style bool deferReference = allowDeferredReference && (targetObject is FrameworkElementFactory || targetObject is Setter || targetObject is SharedDp); // Get prefetchedValue DeferredResourceReference prefetchedValue = PrefetchedValue; bool isValidPrefetchedValue = prefetchedValue != null && !prefetchedValue.IsUnset; // Find value BamlRecordReader bamlRecordReader = bamlReader.GetBamlReader() as BamlRecordReader; object value; if (prefetchedValue != null) { // If we have a prefetched value then we we need to look only in the parser stack not in the App or the theme. value = bamlRecordReader.FindResourceInParserStack(ResourceKey, deferReference, false/*mustReturnDeferredResourceReference*/); } else { // If we do not have a prefetched value it is a regular staticresource lookup and hence we must look in the parser stack and the app and the theme. value = bamlRecordReader.FindResourceInParentChain(ResourceKey, deferReference, false /*mustReturnDeferredResourceReference*/); } if (value == DependencyProperty.UnsetValue) { // If both the currently fetched value and the previously fetched value indicate that the // resource has not been found then it is time to throw an exception. if (!isValidPrefetchedValue) { bamlRecordReader.ThrowException(SRID.ParserNoResource , "{" + ResourceKey.ToString() + "}"); } else { // These prefetched values need to be tested for sharedness. Eg. They // could be refering to a resource marked x:Shared="false". In those // cases each reference must yeild a new instance of the resource. prefetchedValue.CheckIfShared = true; if (!deferReference) { // Inflate the prefetched deferred resource reference, because the // current context does not support using deferred references. value = prefetchedValue.GetValue(BaseValueSourceInternal.Unknown); } else { // Use the prefetched value as is value = prefetchedValue; } } } return value; } ////// The key in a Resource Dictionary used to find the object refered to by this /// Markup Extension. /// [ConstructorArgument("resourceKey")] public object ResourceKey { get { return _resourceKey; } set { if (value == null) { throw new ArgumentNullException("value"); } _resourceKey = value; } } ////// This value is used to resolved StaticResourceIds /// internal virtual DeferredResourceReference PrefetchedValue { get { return null; } } private object _resourceKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
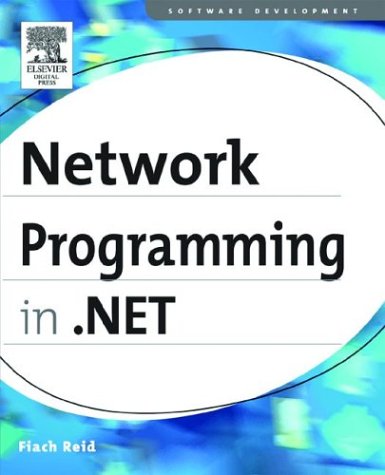
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityWithChangeTrackerStrategy.cs
- ByteStorage.cs
- CallContext.cs
- CuspData.cs
- ClientRuntime.cs
- BinarySerializer.cs
- CacheVirtualItemsEvent.cs
- CacheMode.cs
- PackWebResponse.cs
- XPathNodeList.cs
- CookielessHelper.cs
- WebPartsPersonalizationAuthorization.cs
- DataGridViewButtonCell.cs
- UICuesEvent.cs
- SqlParameterizer.cs
- SerialPort.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- GridViewSortEventArgs.cs
- XmlAutoDetectWriter.cs
- QuaternionValueSerializer.cs
- LayoutManager.cs
- FileDialogCustomPlace.cs
- DeflateStreamAsyncResult.cs
- PasswordBox.cs
- DataSourceControlBuilder.cs
- XmlHierarchicalDataSourceView.cs
- LinearKeyFrames.cs
- FrameworkReadOnlyPropertyMetadata.cs
- Suspend.cs
- AnnouncementEndpoint.cs
- EditModeSwitchButton.cs
- TreeNodeMouseHoverEvent.cs
- RectangleGeometry.cs
- SID.cs
- GeneralTransformGroup.cs
- RootBuilder.cs
- WebPartEditVerb.cs
- NegotiateStream.cs
- Column.cs
- AccessKeyManager.cs
- RootBrowserWindowProxy.cs
- ISAPIWorkerRequest.cs
- WindowsContainer.cs
- TransformFinalBlockRequest.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- GenericAuthenticationEventArgs.cs
- Statements.cs
- _NetworkingPerfCounters.cs
- ObjectSet.cs
- CharUnicodeInfo.cs
- ToolBar.cs
- SafeArrayRankMismatchException.cs
- IntAverageAggregationOperator.cs
- LOSFormatter.cs
- VectorAnimationBase.cs
- SizeChangedEventArgs.cs
- KeyGestureValueSerializer.cs
- TypeLoader.cs
- CodeExporter.cs
- ClientScriptManager.cs
- WebConfigManager.cs
- LiteralTextContainerControlBuilder.cs
- DataGridViewCellStyleConverter.cs
- MarshalByValueComponent.cs
- Publisher.cs
- PeerUnsafeNativeCryptMethods.cs
- CalendarDay.cs
- brushes.cs
- TextRangeSerialization.cs
- TextSearch.cs
- TdsParser.cs
- Recipient.cs
- LogSwitch.cs
- OAVariantLib.cs
- CodeSubDirectory.cs
- ExtensionCollection.cs
- PackageRelationship.cs
- NetworkCredential.cs
- Thread.cs
- MobileFormsAuthentication.cs
- StretchValidation.cs
- ExpressionPrefixAttribute.cs
- RestHandler.cs
- MediaContext.cs
- GiveFeedbackEvent.cs
- TypeSystem.cs
- Errors.cs
- IODescriptionAttribute.cs
- OdbcHandle.cs
- XmlCountingReader.cs
- PriorityQueue.cs
- XmlIlGenerator.cs
- RequestCachePolicy.cs
- ToolStripDropDownClosingEventArgs.cs
- CriticalFinalizerObject.cs
- localization.cs
- DeploymentSectionCache.cs
- SystemColors.cs
- XmlSchemaCompilationSettings.cs
- TextBox.cs