Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ExtensionCollection.cs / 1 / ExtensionCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Runtime.Serialization; public sealed class ExtensionCollection: SynchronizedCollection >, IExtensionCollection where T : IExtensibleObject { T owner; public ExtensionCollection(T owner) { if (owner == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("owner"); this.owner = owner; } public ExtensionCollection(T owner, object syncRoot) : base(syncRoot) { if (owner == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("owner"); this.owner = owner; } bool ICollection >.IsReadOnly { get { return false; } } protected override void ClearItems() { IExtension [] array; lock (this.SyncRoot) { array = new IExtension [this.Count]; this.CopyTo(array, 0); base.ClearItems(); foreach (IExtension extension in array) { extension.Detach(this.owner); } } } public E Find () { List > items = this.Items; lock (this.SyncRoot) { for (int i=this.Count-1; i>=0; i--) { IExtension item = items[i]; if (item is E) return (E)item; } } return default(E); } public Collection FindAll () { Collection result = new Collection (); List > items = this.Items; lock (this.SyncRoot) { for (int i=0; i item = items[i]; if (item is E) result.Add((E)item); } } return result; } protected override void InsertItem(int index, IExtension item) { if (item == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); lock (this.SyncRoot) { item.Attach(this.owner); base.InsertItem(index, item); } } protected override void RemoveItem(int index) { lock (this.SyncRoot) { this.Items[index].Detach(this.owner); base.RemoveItem(index); } } protected override void SetItem(int index, IExtension item) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxCannotSetExtensionsByIndex))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
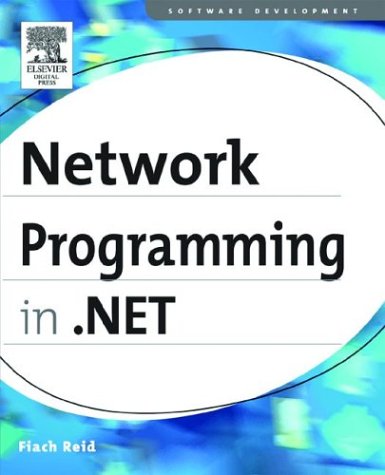
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AliasedExpr.cs
- NamespaceMapping.cs
- PopupEventArgs.cs
- XmlSchemaSimpleContent.cs
- ListViewGroup.cs
- Literal.cs
- BlockingCollection.cs
- ReadOnlyPropertyMetadata.cs
- PermissionToken.cs
- CornerRadius.cs
- Baml2006Reader.cs
- CookieHandler.cs
- EditCommandColumn.cs
- GroupItemAutomationPeer.cs
- TypefaceMetricsCache.cs
- QuadraticEase.cs
- XamlUtilities.cs
- BaseCodePageEncoding.cs
- FontDialog.cs
- MultiDataTrigger.cs
- SafeJobHandle.cs
- CookielessHelper.cs
- ApplicationSecurityManager.cs
- PerformanceCounterPermissionAttribute.cs
- LogicalExpr.cs
- X509AsymmetricSecurityKey.cs
- Light.cs
- CatalogPart.cs
- CodePageEncoding.cs
- InvalidOperationException.cs
- GenerateScriptTypeAttribute.cs
- QilInvokeEarlyBound.cs
- Stack.cs
- ExceptionHelpers.cs
- CustomPopupPlacement.cs
- ExecutedRoutedEventArgs.cs
- UriTemplateDispatchFormatter.cs
- XmlSerializableWriter.cs
- ViewGenerator.cs
- MultipleCopiesCollection.cs
- ListItemCollection.cs
- _RequestCacheProtocol.cs
- Perspective.cs
- PersonalizablePropertyEntry.cs
- BoolExpressionVisitors.cs
- Emitter.cs
- HttpChannelFactory.cs
- DbMetaDataFactory.cs
- SafeReversePInvokeHandle.cs
- MDIControlStrip.cs
- ScriptMethodAttribute.cs
- ZipIOExtraFieldPaddingElement.cs
- FileDialogCustomPlacesCollection.cs
- mansign.cs
- ColumnReorderedEventArgs.cs
- XmlBaseReader.cs
- SiteMapPath.cs
- AppDomainUnloadedException.cs
- Wizard.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- ListViewEditEventArgs.cs
- TiffBitmapEncoder.cs
- Util.cs
- XamlFilter.cs
- LineInfo.cs
- SpecialNameAttribute.cs
- SystemUnicastIPAddressInformation.cs
- LocalizedNameDescriptionPair.cs
- RowToParametersTransformer.cs
- TableRowCollection.cs
- MatrixIndependentAnimationStorage.cs
- BuildProvider.cs
- DataGridHyperlinkColumn.cs
- MasterPageCodeDomTreeGenerator.cs
- RightsManagementPermission.cs
- PingReply.cs
- AvtEvent.cs
- Thread.cs
- linebase.cs
- UnsafeNativeMethods.cs
- HierarchicalDataSourceControl.cs
- GenericEnumConverter.cs
- DeviceContext.cs
- BindUriHelper.cs
- SpellerError.cs
- InlineUIContainer.cs
- MeshGeometry3D.cs
- Console.cs
- FileChangesMonitor.cs
- NetCodeGroup.cs
- NativeMethodsCLR.cs
- XmlRootAttribute.cs
- RectAnimation.cs
- HtmlFormWrapper.cs
- InvokeMethodActivityDesigner.cs
- DesignerSerializationVisibilityAttribute.cs
- RenderDataDrawingContext.cs
- RegexCompiler.cs
- PriorityBinding.cs
- PrintPreviewGraphics.cs