Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / Caching / MetadataCacheItem.cs / 2 / MetadataCacheItem.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to cache metadata information. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Caching { using System; using System.Diagnostics; using System.Collections.Generic; using System.Data.Services.Providers; ///Use this class to cache metadata for providers. internal class MetadataCacheItem { #region Private fields. ///list of top level entity sets private readonly DictionaryentitySets; /// Collection of service operations, keyed by name. private readonly DictionaryserviceOperations; /// Target type for the data provider. private readonly Type type; ///Cache of resource properties per type. private readonly DictionarytypeCache; /// Service configuration information. private DataServiceConfiguration configuration; ///If the metadata is compliant with the edm schema v1.0 other false, indicating that we need to use 1.1 schema /// 1> Edm Schema 1.0 FunctionImport returns only collection of scalars or collection of entities private bool edmVersion1Schema = true; #endregion Private fields. ///Initializes a new /// Type of data context for which metadata will be generated. internal MetadataCacheItem(Type type) { Debug.Assert(type != null, "type != null"); this.serviceOperations = new Dictionaryinstance. (); this.typeCache = new Dictionary (); this.entitySets = new Dictionary (); this.type = type; } #region Properties. /// Service configuration information. internal DataServiceConfiguration Configuration { [DebuggerStepThrough] get { return this.configuration; } set { Debug.Assert(value != null, "value != null"); Debug.Assert(this.configuration == null, "this.configuration == null -- otherwise it's being set more than once"); this.configuration = value; } } ///Collection of service operations, keyed by name. internal DictionaryServiceOperations { [DebuggerStepThrough] get { return this.serviceOperations; } } /// Cache of resource properties per type. internal DictionaryTypeCache { [DebuggerStepThrough] get { return this.typeCache; } } /// list of top level entity sets internal DictionaryEntitySets { [DebuggerStepThrough] get { return this.entitySets; } } /// Target type for the data provider. internal Type Type { [DebuggerStepThrough] get { return this.type; } } ///Returns true if the metadata adheres to edm v1 constraints otherwise returns false. internal bool EdmVersion1Schema { get { return this.edmVersion1Schema; } set { this.edmVersion1Schema = value; } } #endregion Properties. ///Seals this item instance and prevents further changes. ////// This method should be called after the configuration has been set up and before it's placed on the /// metadata cache for sharing. /// internal void Seal() { this.configuration.Seal(); foreach (ResourceType t in this.typeCache.Values) { t.TrimExcess(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to cache metadata information. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Caching { using System; using System.Diagnostics; using System.Collections.Generic; using System.Data.Services.Providers; ///Use this class to cache metadata for providers. internal class MetadataCacheItem { #region Private fields. ///list of top level entity sets private readonly DictionaryentitySets; /// Collection of service operations, keyed by name. private readonly DictionaryserviceOperations; /// Target type for the data provider. private readonly Type type; ///Cache of resource properties per type. private readonly DictionarytypeCache; /// Service configuration information. private DataServiceConfiguration configuration; ///If the metadata is compliant with the edm schema v1.0 other false, indicating that we need to use 1.1 schema /// 1> Edm Schema 1.0 FunctionImport returns only collection of scalars or collection of entities private bool edmVersion1Schema = true; #endregion Private fields. ///Initializes a new /// Type of data context for which metadata will be generated. internal MetadataCacheItem(Type type) { Debug.Assert(type != null, "type != null"); this.serviceOperations = new Dictionaryinstance. (); this.typeCache = new Dictionary (); this.entitySets = new Dictionary (); this.type = type; } #region Properties. /// Service configuration information. internal DataServiceConfiguration Configuration { [DebuggerStepThrough] get { return this.configuration; } set { Debug.Assert(value != null, "value != null"); Debug.Assert(this.configuration == null, "this.configuration == null -- otherwise it's being set more than once"); this.configuration = value; } } ///Collection of service operations, keyed by name. internal DictionaryServiceOperations { [DebuggerStepThrough] get { return this.serviceOperations; } } /// Cache of resource properties per type. internal DictionaryTypeCache { [DebuggerStepThrough] get { return this.typeCache; } } /// list of top level entity sets internal DictionaryEntitySets { [DebuggerStepThrough] get { return this.entitySets; } } /// Target type for the data provider. internal Type Type { [DebuggerStepThrough] get { return this.type; } } ///Returns true if the metadata adheres to edm v1 constraints otherwise returns false. internal bool EdmVersion1Schema { get { return this.edmVersion1Schema; } set { this.edmVersion1Schema = value; } } #endregion Properties. ///Seals this item instance and prevents further changes. ////// This method should be called after the configuration has been set up and before it's placed on the /// metadata cache for sharing. /// internal void Seal() { this.configuration.Seal(); foreach (ResourceType t in this.typeCache.Values) { t.TrimExcess(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
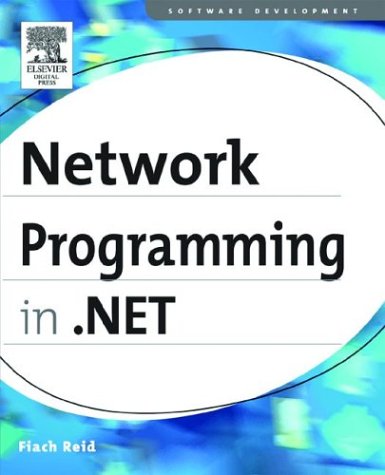
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RawStylusActions.cs
- IndexingContentUnit.cs
- AdapterUtil.cs
- ToolboxItemSnapLineBehavior.cs
- DbConnectionFactory.cs
- DataGridViewCellMouseEventArgs.cs
- XmlObjectSerializer.cs
- WebControl.cs
- ProfileProvider.cs
- unitconverter.cs
- BitmapEffectGroup.cs
- EntryPointNotFoundException.cs
- MatrixAnimationUsingPath.cs
- SystemIPGlobalProperties.cs
- FieldInfo.cs
- ImageClickEventArgs.cs
- PageAdapter.cs
- ConfigurationElement.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- ClipboardData.cs
- DeviceSpecificChoiceCollection.cs
- WebPartEventArgs.cs
- SqlCacheDependencySection.cs
- DebugView.cs
- NameObjectCollectionBase.cs
- ExcCanonicalXml.cs
- SchemaTableColumn.cs
- SecurityHelper.cs
- FlowSwitchLink.cs
- StringWriter.cs
- ListChangedEventArgs.cs
- PanelStyle.cs
- BooleanConverter.cs
- BaseParagraph.cs
- StructuredTypeEmitter.cs
- ObjRef.cs
- RecordManager.cs
- TileModeValidation.cs
- SectionVisual.cs
- SetStateDesigner.cs
- TwoPhaseCommitProxy.cs
- ValueConversionAttribute.cs
- DataListItem.cs
- StorageComplexTypeMapping.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- StorageEntityTypeMapping.cs
- LinqTreeNodeEvaluator.cs
- StateFinalizationDesigner.cs
- ObjectDataSourceChooseTypePanel.cs
- LicenseProviderAttribute.cs
- VariableQuery.cs
- FormatConvertedBitmap.cs
- SqlConnectionFactory.cs
- EntityConnection.cs
- Stack.cs
- InkCanvas.cs
- GatewayDefinition.cs
- CanExecuteRoutedEventArgs.cs
- FontSource.cs
- ServiceModelEnumValidatorAttribute.cs
- XmlLanguageConverter.cs
- X509Certificate2Collection.cs
- PersonalizationProviderCollection.cs
- TypeBuilder.cs
- RegistrySecurity.cs
- ValidationSummary.cs
- CustomErrorCollection.cs
- OutOfMemoryException.cs
- ConfigurationSchemaErrors.cs
- HTTPNotFoundHandler.cs
- TypeSystem.cs
- CodeConditionStatement.cs
- ModelFactory.cs
- SymLanguageType.cs
- MetadataArtifactLoaderResource.cs
- TreeViewItemAutomationPeer.cs
- ReferenceEqualityComparer.cs
- StylusLogic.cs
- ToolboxDataAttribute.cs
- InputBuffer.cs
- TraceData.cs
- TrustManagerMoreInformation.cs
- ReflectionUtil.cs
- ClientUrlResolverWrapper.cs
- httpstaticobjectscollection.cs
- DebuggerAttributes.cs
- DetailsViewRowCollection.cs
- SourceLineInfo.cs
- SystemIPGlobalStatistics.cs
- Overlapped.cs
- ProxyHelper.cs
- XPathSelectionIterator.cs
- AuthenticateEventArgs.cs
- GridProviderWrapper.cs
- EncoderParameters.cs
- MasterPageCodeDomTreeGenerator.cs
- ProcessStartInfo.cs
- COM2FontConverter.cs
- DataRelation.cs
- EncryptedData.cs