Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Data / ValueConversionAttribute.cs / 1 / ValueConversionAttribute.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: allows the author of a IValueConverter // to specify what source and target property types the // ValueConverter is capable of converting // // Specs: http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; namespace System.Windows.Data { ////// This attribute allows the author of a ////// to specify what source and target property types the ValueConverter is capable of converting. /// This meta data is useful for designer tools to help categorize and match ValueConverters. /// /// Add this custom attribute to your IValueConverter class definition. /// [AttributeUsage(AttributeTargets.Class, AllowMultiple = true)] public sealed class ValueConversionAttribute : Attribute { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// [ValueConversion(typeof(Employee), typeof(Brush))] /// class MyConverter : IValueConverter /// { /// public object Convert(object value, Type targetType, object parameter, CultureInfo culture) /// { /// if (value is Dev) return Brushes.Beige; /// if (value is Employee) return Brushes.Salmon; /// return Brushes.Yellow; /// } /// } ///
////// Creates a new ValueConversionAttribute to indicate between /// what types of a data binding source and target this ValueConverter can convert. /// /// the expected source type this ValueConverter can handle /// the target type to which this ValueConverter can convert to public ValueConversionAttribute(Type sourceType, Type targetType) { if (sourceType == null) throw new ArgumentNullException("sourceType"); if (targetType == null) throw new ArgumentNullException("targetType"); _sourceType = sourceType; _targetType = targetType; } ////// The expected source type this ValueConverter can handle. /// public Type SourceType { get { return _sourceType; } } ////// The target type to which this ValueConverter can convert to. /// public Type TargetType { get { return _targetType; } } ////// The type of the optional ValueConverter Parameter object. /// public Type ParameterType { get { return _parameterType; } set { _parameterType = value; } } ////// Returns the unique identifier for this Attribute. /// // Type ID is used to remove redundant attributes by // putting all attributes in a dictionary of [TypeId, Attribute]. // If you want AllowMultiple attributes to work with designers, // you must override TypeId. The default implementation returns // this.GetType(), which is appropriate for AllowMultiple = false, but // not for AllowMultiple = true; public override object TypeId { // the attribute itself will be used as a key to the dictionary get { return this; } } ////// Returns the hash code for this instance. /// override public int GetHashCode() { // the default implementation does some funky enumeration over its fields // we can do better and use the 2 mandatory fields source/targetType's hash codes return _sourceType.GetHashCode() + _targetType.GetHashCode(); } //-------------------------------------------- // Private members //-------------------------------------------- private Type _sourceType; private Type _targetType; private Type _parameterType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: allows the author of a IValueConverter // to specify what source and target property types the // ValueConverter is capable of converting // // Specs: http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; namespace System.Windows.Data { ////// This attribute allows the author of a ////// to specify what source and target property types the ValueConverter is capable of converting. /// This meta data is useful for designer tools to help categorize and match ValueConverters. /// /// Add this custom attribute to your IValueConverter class definition. /// [AttributeUsage(AttributeTargets.Class, AllowMultiple = true)] public sealed class ValueConversionAttribute : Attribute { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// [ValueConversion(typeof(Employee), typeof(Brush))] /// class MyConverter : IValueConverter /// { /// public object Convert(object value, Type targetType, object parameter, CultureInfo culture) /// { /// if (value is Dev) return Brushes.Beige; /// if (value is Employee) return Brushes.Salmon; /// return Brushes.Yellow; /// } /// } ///
////// Creates a new ValueConversionAttribute to indicate between /// what types of a data binding source and target this ValueConverter can convert. /// /// the expected source type this ValueConverter can handle /// the target type to which this ValueConverter can convert to public ValueConversionAttribute(Type sourceType, Type targetType) { if (sourceType == null) throw new ArgumentNullException("sourceType"); if (targetType == null) throw new ArgumentNullException("targetType"); _sourceType = sourceType; _targetType = targetType; } ////// The expected source type this ValueConverter can handle. /// public Type SourceType { get { return _sourceType; } } ////// The target type to which this ValueConverter can convert to. /// public Type TargetType { get { return _targetType; } } ////// The type of the optional ValueConverter Parameter object. /// public Type ParameterType { get { return _parameterType; } set { _parameterType = value; } } ////// Returns the unique identifier for this Attribute. /// // Type ID is used to remove redundant attributes by // putting all attributes in a dictionary of [TypeId, Attribute]. // If you want AllowMultiple attributes to work with designers, // you must override TypeId. The default implementation returns // this.GetType(), which is appropriate for AllowMultiple = false, but // not for AllowMultiple = true; public override object TypeId { // the attribute itself will be used as a key to the dictionary get { return this; } } ////// Returns the hash code for this instance. /// override public int GetHashCode() { // the default implementation does some funky enumeration over its fields // we can do better and use the 2 mandatory fields source/targetType's hash codes return _sourceType.GetHashCode() + _targetType.GetHashCode(); } //-------------------------------------------- // Private members //-------------------------------------------- private Type _sourceType; private Type _targetType; private Type _parameterType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
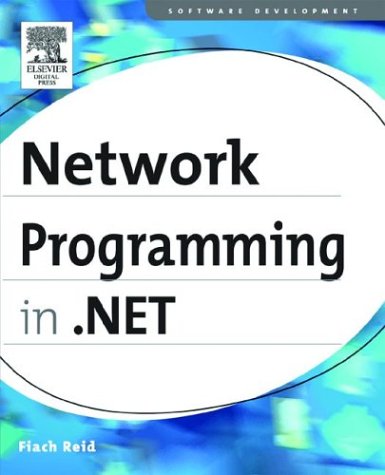
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripLocationCancelEventArgs.cs
- Currency.cs
- PageBreakRecord.cs
- WindowsHyperlink.cs
- AttributeCollection.cs
- TextEndOfLine.cs
- HtmlInputText.cs
- QueryCreatedEventArgs.cs
- CodeCatchClause.cs
- RemotingException.cs
- EmptyStringExpandableObjectConverter.cs
- TreeNodeMouseHoverEvent.cs
- IgnoreSectionHandler.cs
- XmlSchemaInclude.cs
- StylusPointPropertyId.cs
- GlobalAllocSafeHandle.cs
- SystemNetHelpers.cs
- SecurityValidationBehavior.cs
- Message.cs
- TextServicesHost.cs
- COMException.cs
- SourceFileBuildProvider.cs
- WebSysDescriptionAttribute.cs
- StringResourceManager.cs
- AutoCompleteStringCollection.cs
- ExpressionCopier.cs
- Error.cs
- iisPickupDirectory.cs
- SocketElement.cs
- PackageDigitalSignatureManager.cs
- StdRegProviderWrapper.cs
- BufferedStream.cs
- SharedMemory.cs
- DefinitionBase.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- StateRuntime.cs
- ServiceCredentialsElement.cs
- UidManager.cs
- StylusEventArgs.cs
- HtmlAnchor.cs
- ScriptReference.cs
- SettingsAttributeDictionary.cs
- EventLogEntry.cs
- IBuiltInEvidence.cs
- PerspectiveCamera.cs
- HttpContextServiceHost.cs
- RepeatInfo.cs
- ScrollBarRenderer.cs
- dataprotectionpermissionattribute.cs
- ColorTranslator.cs
- XsdValidatingReader.cs
- DetailsViewModeEventArgs.cs
- XPathException.cs
- EditorServiceContext.cs
- SectionVisual.cs
- Guid.cs
- Itemizer.cs
- FileCodeGroup.cs
- _emptywebproxy.cs
- EmptyStringExpandableObjectConverter.cs
- ActiveXHost.cs
- HttpResponseWrapper.cs
- InputScope.cs
- XmlSchemaComplexContentRestriction.cs
- Point3DCollectionConverter.cs
- WebEncodingValidatorAttribute.cs
- webproxy.cs
- EdmSchemaError.cs
- SoapClientProtocol.cs
- GeometryHitTestParameters.cs
- WorkflowServiceBuildProvider.cs
- StringStorage.cs
- ListViewItem.cs
- ForceCopyBuildProvider.cs
- SiteMapHierarchicalDataSourceView.cs
- TagPrefixCollection.cs
- XMLUtil.cs
- SystemException.cs
- TraceSwitch.cs
- DataViewListener.cs
- XmlLoader.cs
- TableLayoutStyleCollection.cs
- XmlDictionaryReaderQuotas.cs
- ScriptControlManager.cs
- ActivityWithResultConverter.cs
- LocationUpdates.cs
- ConfigViewGenerator.cs
- _NativeSSPI.cs
- OptimizedTemplateContent.cs
- TextFindEngine.cs
- MobileControlsSection.cs
- AccessDataSourceView.cs
- ClientSideQueueItem.cs
- VariableModifiersHelper.cs
- NotifyParentPropertyAttribute.cs
- WebPartConnectionCollection.cs
- WindowsToolbarAsMenu.cs
- CompositeCollection.cs
- ToolboxItemAttribute.cs
- PropertyChangedEventManager.cs