Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / EdmSchemaError.cs / 2 / EdmSchemaError.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data; using System.Data.Entity; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// This class encapsulates the error information for a schema error that was encountered. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] [Serializable] public sealed class EdmSchemaError : EdmError { #region Instance Fields private int _errorCode = 0; private EdmSchemaErrorSeverity _severity = EdmSchemaErrorSeverity.Warning; private string _schemaLocation = null; private int _line = -1; private int _column = -1; private string _stackTrace = string.Empty; #endregion #region Public Methods ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity) : this(message, errorCode, severity, null) { } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// The exception that caused the error to be filed. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, Exception exception) : base(message) { Initialize(errorCode, severity, null, -1, -1, exception); } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// /// /// internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column) : this(message, errorCode, severity, schemaLocation, line, column, null) { } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// /// /// /// The exception that caused the error to be filed. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column, Exception exception) : base(message) { if (severity < EdmSchemaErrorSeverity.Warning || severity > EdmSchemaErrorSeverity.Error) { throw new ArgumentOutOfRangeException("severity", severity, System.Data.Entity.Strings.ArgumentOutOfRange(severity)); } if (line < 0) { throw new ArgumentOutOfRangeException("line", line, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(line)); } if (column < 0) { throw new ArgumentOutOfRangeException("column", column, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(column)); } Initialize(errorCode, severity, schemaLocation, line, column, exception); } private void Initialize(int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column, Exception exception) { if (errorCode < 0) { throw new ArgumentOutOfRangeException("errorCode", errorCode, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(errorCode)); } _errorCode = errorCode; _severity = severity; _schemaLocation = schemaLocation; _line = line; _column = column; if (exception != null) { _stackTrace = exception.StackTrace; } } ////// Creates a string representation of the error. /// public override string ToString() { string text; string severity; switch (Severity) { case EdmSchemaErrorSeverity.Error: severity = System.Data.Entity.Strings.GeneratorErrorSeverityError; break; case EdmSchemaErrorSeverity.Warning: severity = System.Data.Entity.Strings.GeneratorErrorSeverityWarning; break; default: severity = System.Data.Entity.Strings.GeneratorErrorSeverityUnknown; break; } if (String.IsNullOrEmpty(SchemaName) && Line < 0 && Column < 0) { text = String.Format(System.Globalization.CultureInfo.CurrentCulture, "{0} {1:0000}: {2}", severity, ErrorCode, Message); } else { text = String.Format(System.Globalization.CultureInfo.CurrentCulture, "{0}({1},{2}) : {3} {4:0000}: {5}", (SchemaName == null) ? System.Data.Entity.Strings.SourceUriUnknown : SchemaName, Line, Column, severity, ErrorCode, Message); } return text; } #endregion #region Public Properties ////// Gets the ErrorCode. /// public int ErrorCode { get { return _errorCode; } } ////// Gets the Severity of the error. /// public EdmSchemaErrorSeverity Severity { get { return _severity; } } ////// Gets the LineNumber that the error occured on. /// public int Line { get { return _line; } } ////// Gets the column that the error occured in. /// public int Column { get { return _column; } } ////// Gets the of the schema that contains the error. /// public string SchemaLocation { get { return _schemaLocation; } } ////// Gets the of the schema that contains the error. /// public string SchemaName { get { return GetNameFromSchemaLocation(SchemaLocation); } } ////// Gets the stack trace of when the error occured. /// ///public string StackTrace { get { return _stackTrace; } } #endregion private static string GetNameFromSchemaLocation(string schemaLocation) { if (string.IsNullOrEmpty(schemaLocation)) { return schemaLocation; } int pos = Math.Max(schemaLocation.LastIndexOf('/'), schemaLocation.LastIndexOf('\\')); int start = pos + 1; if (pos < 0) { return schemaLocation; } else if (start >= schemaLocation.Length) { return string.Empty; } return schemaLocation.Substring(start); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data; using System.Data.Entity; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// This class encapsulates the error information for a schema error that was encountered. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] [Serializable] public sealed class EdmSchemaError : EdmError { #region Instance Fields private int _errorCode = 0; private EdmSchemaErrorSeverity _severity = EdmSchemaErrorSeverity.Warning; private string _schemaLocation = null; private int _line = -1; private int _column = -1; private string _stackTrace = string.Empty; #endregion #region Public Methods ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity) : this(message, errorCode, severity, null) { } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// The exception that caused the error to be filed. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, Exception exception) : base(message) { Initialize(errorCode, severity, null, -1, -1, exception); } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// /// /// internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column) : this(message, errorCode, severity, schemaLocation, line, column, null) { } ////// Constructs a EdmSchemaError object. /// /// The explanation of the error. /// The code associated with this error. /// The severity of the error. /// /// /// /// The exception that caused the error to be filed. internal EdmSchemaError(string message, int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column, Exception exception) : base(message) { if (severity < EdmSchemaErrorSeverity.Warning || severity > EdmSchemaErrorSeverity.Error) { throw new ArgumentOutOfRangeException("severity", severity, System.Data.Entity.Strings.ArgumentOutOfRange(severity)); } if (line < 0) { throw new ArgumentOutOfRangeException("line", line, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(line)); } if (column < 0) { throw new ArgumentOutOfRangeException("column", column, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(column)); } Initialize(errorCode, severity, schemaLocation, line, column, exception); } private void Initialize(int errorCode, EdmSchemaErrorSeverity severity, string schemaLocation, int line, int column, Exception exception) { if (errorCode < 0) { throw new ArgumentOutOfRangeException("errorCode", errorCode, System.Data.Entity.Strings.ArgumentOutOfRangeExpectedPostiveNumber(errorCode)); } _errorCode = errorCode; _severity = severity; _schemaLocation = schemaLocation; _line = line; _column = column; if (exception != null) { _stackTrace = exception.StackTrace; } } ////// Creates a string representation of the error. /// public override string ToString() { string text; string severity; switch (Severity) { case EdmSchemaErrorSeverity.Error: severity = System.Data.Entity.Strings.GeneratorErrorSeverityError; break; case EdmSchemaErrorSeverity.Warning: severity = System.Data.Entity.Strings.GeneratorErrorSeverityWarning; break; default: severity = System.Data.Entity.Strings.GeneratorErrorSeverityUnknown; break; } if (String.IsNullOrEmpty(SchemaName) && Line < 0 && Column < 0) { text = String.Format(System.Globalization.CultureInfo.CurrentCulture, "{0} {1:0000}: {2}", severity, ErrorCode, Message); } else { text = String.Format(System.Globalization.CultureInfo.CurrentCulture, "{0}({1},{2}) : {3} {4:0000}: {5}", (SchemaName == null) ? System.Data.Entity.Strings.SourceUriUnknown : SchemaName, Line, Column, severity, ErrorCode, Message); } return text; } #endregion #region Public Properties ////// Gets the ErrorCode. /// public int ErrorCode { get { return _errorCode; } } ////// Gets the Severity of the error. /// public EdmSchemaErrorSeverity Severity { get { return _severity; } } ////// Gets the LineNumber that the error occured on. /// public int Line { get { return _line; } } ////// Gets the column that the error occured in. /// public int Column { get { return _column; } } ////// Gets the of the schema that contains the error. /// public string SchemaLocation { get { return _schemaLocation; } } ////// Gets the of the schema that contains the error. /// public string SchemaName { get { return GetNameFromSchemaLocation(SchemaLocation); } } ////// Gets the stack trace of when the error occured. /// ///public string StackTrace { get { return _stackTrace; } } #endregion private static string GetNameFromSchemaLocation(string schemaLocation) { if (string.IsNullOrEmpty(schemaLocation)) { return schemaLocation; } int pos = Math.Max(schemaLocation.LastIndexOf('/'), schemaLocation.LastIndexOf('\\')); int start = pos + 1; if (pos < 0) { return schemaLocation; } else if (start >= schemaLocation.Length) { return string.Empty; } return schemaLocation.Substring(start); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
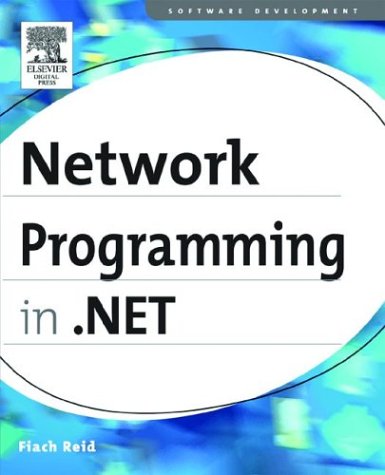
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RijndaelManaged.cs
- ManifestResourceInfo.cs
- SchemaImporterExtension.cs
- PointLightBase.cs
- NativeMethods.cs
- SqlVisitor.cs
- DbConnectionStringCommon.cs
- TypeBinaryExpression.cs
- DocumentReferenceCollection.cs
- SystemPens.cs
- XmlNode.cs
- PackagePart.cs
- Converter.cs
- ScrollBar.cs
- TextChangedEventArgs.cs
- ReferencedCollectionType.cs
- XmlValueConverter.cs
- ZipIOExtraFieldElement.cs
- SignatureDescription.cs
- BuildProviderCollection.cs
- _NtlmClient.cs
- IPipelineRuntime.cs
- FlowDocumentView.cs
- ConfigurationException.cs
- MonitoringDescriptionAttribute.cs
- ClientBuildManager.cs
- OdbcCommandBuilder.cs
- DynamicActivity.cs
- cookie.cs
- AccessDataSourceView.cs
- TextCompositionManager.cs
- sqlcontext.cs
- securitycriticaldata.cs
- webbrowsersite.cs
- TransformDescriptor.cs
- Menu.cs
- MetricEntry.cs
- PlanCompilerUtil.cs
- GradientStopCollection.cs
- FontUnitConverter.cs
- TypedTableBase.cs
- GenerateScriptTypeAttribute.cs
- ApplicationContext.cs
- SmtpReplyReaderFactory.cs
- MediaScriptCommandRoutedEventArgs.cs
- CommentEmitter.cs
- PerformanceCounterManager.cs
- DataServiceException.cs
- TraceXPathNavigator.cs
- SecurityPolicySection.cs
- RightsManagementInformation.cs
- DataServiceConfiguration.cs
- XmlException.cs
- HostedElements.cs
- ScriptModule.cs
- SpAudioStreamWrapper.cs
- SortedDictionary.cs
- DocumentViewerAutomationPeer.cs
- propertytag.cs
- Qualifier.cs
- WindowsListViewItem.cs
- SpeechRecognizer.cs
- BindingsCollection.cs
- TileModeValidation.cs
- HelpEvent.cs
- XmlSchemaImporter.cs
- CompositeScriptReferenceEventArgs.cs
- SafeTokenHandle.cs
- DesignSurfaceEvent.cs
- ConfigurationManagerInternalFactory.cs
- ImageClickEventArgs.cs
- ColorAnimationUsingKeyFrames.cs
- HealthMonitoringSection.cs
- EnterpriseServicesHelper.cs
- CompilationLock.cs
- RootBuilder.cs
- ComMethodElement.cs
- DragStartedEventArgs.cs
- PerfService.cs
- FrameworkObject.cs
- FlowLayoutSettings.cs
- CodeSnippetExpression.cs
- NameNode.cs
- DatePickerTextBox.cs
- OneToOneMappingSerializer.cs
- Mapping.cs
- DbProviderSpecificTypePropertyAttribute.cs
- EntityDataSourceReferenceGroup.cs
- DbReferenceCollection.cs
- BindingContext.cs
- WorkflowServiceHostFactory.cs
- CanonicalFontFamilyReference.cs
- UpdateException.cs
- ServiceHostFactory.cs
- UnsafeNativeMethods.cs
- WindowsListViewGroup.cs
- WeakRefEnumerator.cs
- LayoutSettings.cs
- EncodingNLS.cs
- IsolatedStorageFilePermission.cs