Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / SerTrace.cs / 1305376 / SerTrace.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerTrace ** ** ** Purpose: Routine used for Debugging ** ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Security.Permissions; using System.Reflection; using System.Diagnostics; using System.Diagnostics.Contracts; #if FEATURE_PAL // To turn on tracing, add the following to the per-machine // rotor.ini file, inside the [Rotor] section: // ManagedLogFacility=0x32 // where: #else // To turn on tracing the set registry // HKEY_CURRENT_USER -> Software -> Microsoft -> .NETFramework // new DWORD value ManagedLogFacility 0x32 where #endif // 0x2 is System.Runtime.Serialization // 0x10 is Binary Formatter // 0x20 is Soap Formatter // // Turn on Logging in the jitmgr // remoting Wsdl logging ///[System.Security.SecurityCritical] // auto-generated_required [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalRM { /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("SOAP"); } } /// [System.Security.SecurityCritical] // auto-generated_required [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalST { private InternalST() { } /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("Soap"); } /// [System.Diagnostics.Conditional("SER_LOGGING")] public static void Soap(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("SOAP",messages); } /// [System.Diagnostics.Conditional("_DEBUG")] public static void SoapAssert(bool condition, String message) { Contract.Assert(condition, message); } /// public static void SerializationSetValue(FieldInfo fi, Object target, Object value) { if (fi == null) throw new ArgumentNullException("fi"); if (target == null) throw new ArgumentNullException("target"); if (value == null) throw new ArgumentNullException("value"); Contract.EndContractBlock(); FormatterServices.SerializationSetValue(fi, target, value); } /// public static Assembly LoadAssemblyFromString(String assemblyString) { return FormatterServices.LoadAssemblyFromString(assemblyString); } } internal static class SerTrace { [Conditional("_LOGGING")] internal static void InfoLog(params Object[]messages) { BCLDebug.Trace("BINARY", messages); } [Conditional("SER_LOGGING")] internal static void Log(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("BINARY",messages); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
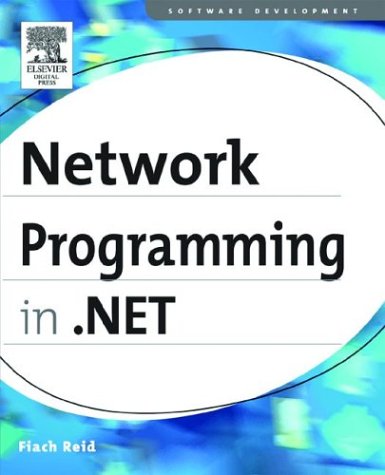
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- loginstatus.cs
- NativeMethods.cs
- SqlFactory.cs
- WebBrowserNavigatingEventHandler.cs
- CompiledQueryCacheKey.cs
- HttpPostedFile.cs
- TrackBarDesigner.cs
- EntityDesignPluralizationHandler.cs
- FlowLayoutSettings.cs
- ObjectReaderCompiler.cs
- LineSegment.cs
- DataGridViewRowsAddedEventArgs.cs
- SmtpClient.cs
- DesignTimeSiteMapProvider.cs
- ConsoleKeyInfo.cs
- Stream.cs
- ProfessionalColors.cs
- RegexCaptureCollection.cs
- DataGridAddNewRow.cs
- ProtocolReflector.cs
- CallbackValidator.cs
- WebDescriptionAttribute.cs
- EntityDataSourceChangingEventArgs.cs
- VisualBrush.cs
- Transform.cs
- MSHTMLHost.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- Int32KeyFrameCollection.cs
- UriParserTemplates.cs
- XamlReaderHelper.cs
- InstanceNotReadyException.cs
- PersonalizationDictionary.cs
- DuplicateWaitObjectException.cs
- StringComparer.cs
- TypeBuilder.cs
- Stream.cs
- HtmlAnchor.cs
- DataRelation.cs
- ImpersonateTokenRef.cs
- HttpHandlerAction.cs
- DataGridCellEditEndingEventArgs.cs
- Section.cs
- SqlConnection.cs
- ExecutionContext.cs
- ContentPresenter.cs
- unitconverter.cs
- GestureRecognitionResult.cs
- DatePickerAutomationPeer.cs
- DataComponentNameHandler.cs
- SqlComparer.cs
- EventListenerClientSide.cs
- ConfigViewGenerator.cs
- DataPointer.cs
- FormCollection.cs
- XmlDataCollection.cs
- DataServiceContext.cs
- JsonByteArrayDataContract.cs
- ResourceAttributes.cs
- IPHostEntry.cs
- ToolboxItem.cs
- CrossAppDomainChannel.cs
- FontCollection.cs
- XmlAttributeAttribute.cs
- AudioDeviceOut.cs
- CryptographicAttribute.cs
- SizeChangedInfo.cs
- DataTableReader.cs
- WSDualHttpSecurityElement.cs
- CriticalHandle.cs
- NavigationPropertyEmitter.cs
- WebBrowser.cs
- SerialErrors.cs
- SqlSelectClauseBuilder.cs
- IndicCharClassifier.cs
- SafeSystemMetrics.cs
- OracleConnectionFactory.cs
- CacheHelper.cs
- DSASignatureDeformatter.cs
- KeyGesture.cs
- WebPart.cs
- TypeToken.cs
- CompilationRelaxations.cs
- ObfuscationAttribute.cs
- HtmlInputCheckBox.cs
- StringArrayConverter.cs
- ImmutablePropertyDescriptorGridEntry.cs
- PointLight.cs
- ProxyWebPart.cs
- TraceSwitch.cs
- RequiredAttributeAttribute.cs
- ListViewUpdatedEventArgs.cs
- CallId.cs
- ForEachAction.cs
- Convert.cs
- VerticalAlignConverter.cs
- InvalidDataException.cs
- TransformedBitmap.cs
- FormsIdentity.cs
- SimpleMailWebEventProvider.cs
- WebPartHelpVerb.cs