Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / unitconverter.cs / 1 / unitconverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web.Util; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class UnitConverter : TypeConverter { ////// /// Returns a value indicating whether the unit converter can /// convert from the specified source type. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } ////// /// Returns a value indicating whether the converter can /// convert to the specified destination type. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ((destinationType == typeof(string)) || (destinationType == typeof(InstanceDescriptor))) { return true; } else { return base.CanConvertTo(context, destinationType); } } ////// /// Performs type conversion from the given value into a Unit. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; string stringValue = value as string; if (stringValue != null) { string textValue = stringValue.Trim(); if (textValue.Length == 0) { return Unit.Empty; } if (culture != null) { return Unit.Parse(textValue, culture); } else { return Unit.Parse(textValue, CultureInfo.CurrentCulture); } } else { return base.ConvertFrom(context, culture, value); } } ////// /// Performs type conversion to the specified destination type /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string)) { if ((value == null) || ((Unit)value).IsEmpty) return String.Empty; else return ((Unit)value).ToString(culture); } else if ((destinationType == typeof(InstanceDescriptor)) && (value != null)) { Unit u = (Unit)value; MemberInfo member = null; object[] args = null; if (u.IsEmpty) { member = typeof(Unit).GetField("Empty"); } else { member = typeof(Unit).GetConstructor(new Type[] { typeof(double), typeof(UnitType) }); args = new object[] { u.Value, u.Type }; } Debug.Assert(member != null, "Looks like we're missing Unit.Empty or Unit::ctor(double, UnitType)"); if (member != null) { return new InstanceDescriptor(member, args); } else { return null; } } else { return base.ConvertTo(context, culture, value, destinationType); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web.Util; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class UnitConverter : TypeConverter { ////// /// Returns a value indicating whether the unit converter can /// convert from the specified source type. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } ////// /// Returns a value indicating whether the converter can /// convert to the specified destination type. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ((destinationType == typeof(string)) || (destinationType == typeof(InstanceDescriptor))) { return true; } else { return base.CanConvertTo(context, destinationType); } } ////// /// Performs type conversion from the given value into a Unit. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; string stringValue = value as string; if (stringValue != null) { string textValue = stringValue.Trim(); if (textValue.Length == 0) { return Unit.Empty; } if (culture != null) { return Unit.Parse(textValue, culture); } else { return Unit.Parse(textValue, CultureInfo.CurrentCulture); } } else { return base.ConvertFrom(context, culture, value); } } ////// /// Performs type conversion to the specified destination type /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string)) { if ((value == null) || ((Unit)value).IsEmpty) return String.Empty; else return ((Unit)value).ToString(culture); } else if ((destinationType == typeof(InstanceDescriptor)) && (value != null)) { Unit u = (Unit)value; MemberInfo member = null; object[] args = null; if (u.IsEmpty) { member = typeof(Unit).GetField("Empty"); } else { member = typeof(Unit).GetConstructor(new Type[] { typeof(double), typeof(UnitType) }); args = new object[] { u.Value, u.Type }; } Debug.Assert(member != null, "Looks like we're missing Unit.Empty or Unit::ctor(double, UnitType)"); if (member != null) { return new InstanceDescriptor(member, args); } else { return null; } } else { return base.ConvertTo(context, culture, value, destinationType); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
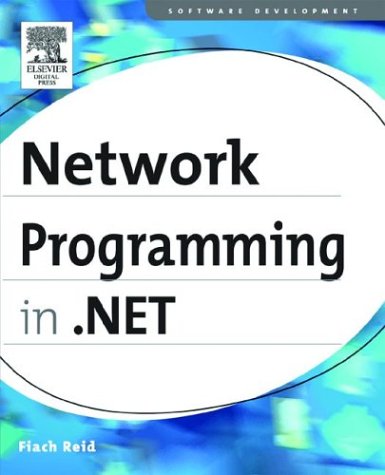
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceRequestException.cs
- Effect.cs
- NamedPipeConnectionPool.cs
- XmlSchemaSimpleTypeRestriction.cs
- DoubleLink.cs
- Stylesheet.cs
- CompositeActivityValidator.cs
- OleDbException.cs
- QilReference.cs
- NumericUpDownAcceleration.cs
- XXXInfos.cs
- DoubleLinkList.cs
- DoubleAnimationClockResource.cs
- TextCollapsingProperties.cs
- PolyBezierSegment.cs
- SaveFileDialog.cs
- MulticastDelegate.cs
- SolidColorBrush.cs
- ListBindingConverter.cs
- FixedSOMGroup.cs
- Vector3DAnimation.cs
- ClassicBorderDecorator.cs
- HWStack.cs
- WebPartDisplayModeEventArgs.cs
- DirectoryObjectSecurity.cs
- ProtectedConfigurationSection.cs
- FormClosedEvent.cs
- StreamAsIStream.cs
- DefinitionBase.cs
- SmtpMail.cs
- SortDescriptionCollection.cs
- PersonalizationProviderCollection.cs
- CodeLabeledStatement.cs
- DataMemberAttribute.cs
- HtmlForm.cs
- Marshal.cs
- KeyProperty.cs
- StorageComplexTypeMapping.cs
- AdapterDictionary.cs
- DataGridViewRowCancelEventArgs.cs
- AttributeXamlType.cs
- TextTreeText.cs
- WindowsSpinner.cs
- FontWeightConverter.cs
- EncoderNLS.cs
- XmlEntity.cs
- ArgumentsParser.cs
- IPHostEntry.cs
- CodeGen.cs
- GridItemPattern.cs
- FilteredDataSetHelper.cs
- WindowsFormsSectionHandler.cs
- DiscoveryClient.cs
- CollectionBase.cs
- xmlglyphRunInfo.cs
- PartialTrustVisibleAssembliesSection.cs
- OutputCacheSection.cs
- RangeValuePattern.cs
- ErrorStyle.cs
- SmiEventSink.cs
- MsmqInputMessage.cs
- SubMenuStyleCollection.cs
- ModelVisual3D.cs
- DeflateEmulationStream.cs
- XPathScanner.cs
- DataGridAutomationPeer.cs
- SharedConnectionWorkflowTransactionService.cs
- DragStartedEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- AxDesigner.cs
- TextTabProperties.cs
- TypedDataSetSchemaImporterExtension.cs
- EventLogger.cs
- StylusLogic.cs
- FormViewInsertedEventArgs.cs
- CornerRadius.cs
- CatalogPartChrome.cs
- _AcceptOverlappedAsyncResult.cs
- WorkflowIdleElement.cs
- EnumConverter.cs
- TcpWorkerProcess.cs
- Page.cs
- FlowDocumentView.cs
- ServiceNameElementCollection.cs
- ContractDescription.cs
- RadioButton.cs
- EventProxy.cs
- SQLSingle.cs
- WindowsScrollBar.cs
- EndGetFileNameFromUserRequest.cs
- Trigger.cs
- Event.cs
- SecureUICommand.cs
- RSAPKCS1SignatureFormatter.cs
- DataViewSettingCollection.cs
- DeobfuscatingStream.cs
- DoneReceivingAsyncResult.cs
- SmtpTransport.cs
- MatrixTransform3D.cs
- NameSpaceExtractor.cs