Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / FormDesigner.cs / 1305376 / FormDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls { using System; using System.Drawing; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Web.UI.Design.MobileControls.Util; using System.Web.UI.Design.MobileControls.Adapters; using System.Web.UI.MobileControls; using Microsoft.Win32; ////// ////// Provides design-time support for the ////// mobile control. /// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal class FormDesigner : MobileContainerDesigner { private Form _form; private TemporaryBitmapFile _backgroundBmpFile = null; private static readonly Attribute[] _emptyAttrs = new Attribute[0]; private const String _titlePropertyName = "Title"; public override String ID { get { return base.ID; } set { base.ID = value; ChangeBackgroundImage(); } } public virtual String Title { get { return _form.Title; } set { _form.Title = value; ChangeBackgroundImage(); } } private bool ValidContainment { get { return (ContainmentStatus == ContainmentStatus.AtTopLevel); } } private void ChangeBackgroundImage() { bool infoMode = false; String newMessage = GetErrorMessage(out infoMode); OnBackgroundImageChange(newMessage, infoMode); } protected override void Dispose(bool disposing) { if (disposing) { SystemEvents.UserPreferenceChanged -= new UserPreferenceChangedEventHandler(this.OnUserPreferenceChanged); } base.Dispose(disposing); } protected override String GetErrorMessage(out bool infoMode) { infoMode = false; if (!DesignerAdapterUtil.InMobileUserControl(_form)) { if (DesignerAdapterUtil.InUserControl(_form)) { infoMode = true; return MobileControlDesigner._userControlWarningMessage; } if (!DesignerAdapterUtil.InMobilePage(_form)) { return SR.GetString(SR.MobileControl_MobilePageErrorMessage); } } if (!ValidContainment) { return SR.GetString(SR.MobileControl_TopPageContainmentErrorMessage); } return null; } /// /// /// /// The control element being designed. /// ////// Initializes the designer using /// the specified component. /// ////// ////// This is called by the designer host to establish the component being /// designed. /// ///public override void Initialize(IComponent component) { Debug.Assert(component is Form, "FormDesigner.Initialize - Invalid Form Control"); // This must be called first in order to get properties from runtime control. base.Initialize(component); _form = (Form) component; if (_form.DeviceSpecific != null) { _form.DeviceSpecific = null; } SystemEvents.UserPreferenceChanged += new UserPreferenceChangedEventHandler(this.OnUserPreferenceChanged); } internal override void OnBackgroundImageChange(String message, bool infoMode) { ImageCreator.CreateBackgroundImage( ref _backgroundBmpFile, _form.ID, _form.Title, message, infoMode, GetDefaultSize().Width ); SetBehaviorStyle("backgroundImage", "url(" + _backgroundBmpFile.Url + ")"); SetBehaviorStyle( "paddingTop", _backgroundBmpFile.UnderlyingBitmap.Height + 8 ); } private void OnUserPreferenceChanged(Object sender, UserPreferenceChangedEventArgs e) { if (e.Category == UserPreferenceCategory.Color) { bool infoMode; String newMessage = GetErrorMessage(out infoMode); OnBackgroundImageChange(newMessage, infoMode); } } /// /// Adjust the appearance based on current status. /// protected override void OnContainmentChanged() { base.OnContainmentChanged(); SetBehaviorStyle("marginTop", ValidContainment ? "5px" : "3px"); SetBehaviorStyle("marginBottom", ValidContainment ? "5px" : "3px"); SetBehaviorStyle("marginRight", ValidContainment ? "30%" : "5px"); } protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); PropertyDescriptor prop = (PropertyDescriptor)properties[_titlePropertyName]; Debug.Assert(prop != null); properties[_titlePropertyName] = TypeDescriptor.CreateProperty(GetType(), prop, _emptyAttrs); } protected override void SetControlDefaultAppearance() { base.SetControlDefaultAppearance(); // Customized border style SetBehaviorStyle("borderStyle", "solid"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
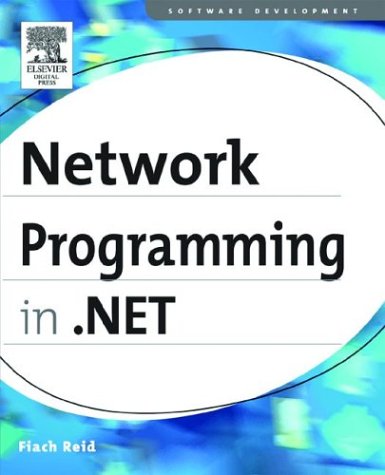
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyCollection.cs
- ComponentConverter.cs
- Filter.cs
- TypeDescriptor.cs
- BooleanConverter.cs
- AsymmetricAlgorithm.cs
- ObjectToken.cs
- DbMetaDataFactory.cs
- DataTablePropertyDescriptor.cs
- PixelShader.cs
- Border.cs
- SuppressIldasmAttribute.cs
- TextServicesManager.cs
- ClockGroup.cs
- HybridDictionary.cs
- OperandQuery.cs
- LocatorPart.cs
- RegistrySecurity.cs
- StandardBindingElement.cs
- DefaultHttpHandler.cs
- ReadOnlyCollection.cs
- SHA512.cs
- EntityDataSourceColumn.cs
- OwnerDrawPropertyBag.cs
- PropertyGrid.cs
- ToolstripProfessionalRenderer.cs
- SessionEndedEventArgs.cs
- CollectionViewProxy.cs
- XmlPreloadedResolver.cs
- TextChange.cs
- Control.cs
- TokenBasedSetEnumerator.cs
- COMException.cs
- SplashScreenNativeMethods.cs
- _UriTypeConverter.cs
- SelectorAutomationPeer.cs
- CookieProtection.cs
- TextServicesCompartmentEventSink.cs
- OptimalTextSource.cs
- HtmlControl.cs
- DataPagerFieldCollection.cs
- OrderingQueryOperator.cs
- NamespaceDisplay.xaml.cs
- PropertyItemInternal.cs
- XamlStream.cs
- PropertyEmitter.cs
- TextContainerChangedEventArgs.cs
- _Win32.cs
- Resources.Designer.cs
- BrowserCapabilitiesCompiler.cs
- ExternalDataExchangeService.cs
- XpsException.cs
- ScrollItemProviderWrapper.cs
- MobileControlPersister.cs
- TableParaClient.cs
- ILGenerator.cs
- CodeAccessSecurityEngine.cs
- TransformedBitmap.cs
- ToolStripPanelRow.cs
- TimeSpanStorage.cs
- MediaElement.cs
- OptimizerPatterns.cs
- FormViewUpdateEventArgs.cs
- RangeBaseAutomationPeer.cs
- RegexReplacement.cs
- ServiceOperationParameter.cs
- MemberDomainMap.cs
- ValidatingReaderNodeData.cs
- TextElementEditingBehaviorAttribute.cs
- StoreItemCollection.cs
- AmbientValueAttribute.cs
- PageContentAsyncResult.cs
- BrowserDefinitionCollection.cs
- CookieParameter.cs
- RepeatButtonAutomationPeer.cs
- TimeSpanMinutesConverter.cs
- RegionInfo.cs
- ExtensibleClassFactory.cs
- PageRanges.cs
- SecurityStateEncoder.cs
- Module.cs
- XmlSchemaSimpleTypeList.cs
- FileChangesMonitor.cs
- Ref.cs
- SerializationInfoEnumerator.cs
- LeaseManager.cs
- ConsoleTraceListener.cs
- CollectionsUtil.cs
- StringValueConverter.cs
- SqlOuterApplyReducer.cs
- PathStreamGeometryContext.cs
- EventLogPermissionEntryCollection.cs
- InfoCardCryptoHelper.cs
- DataServiceRequestArgs.cs
- ResumeStoryboard.cs
- LogoValidationException.cs
- OperandQuery.cs
- XmlArrayItemAttributes.cs
- PenLineCapValidation.cs
- EditorZone.cs