Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / StandardBindingElement.cs / 1 / StandardBindingElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System.Collections; using System.ServiceModel.Channels; using System.ComponentModel; using System.Configuration; using System.ServiceModel; using System.Security; public abstract partial class StandardBindingElement : ConfigurationElement, IBindingConfigurationElement, IConfigurationContextProviderInternal { ////// Critical - stores information used in a security decision /// [SecurityCritical] EvaluationContextHelper contextHelper; protected StandardBindingElement() : this(null) { } protected StandardBindingElement(string name) { if (!String.IsNullOrEmpty(name)) { this.Name = name; } } protected abstract Type BindingElementType { get; } [ConfigurationProperty(ConfigurationStrings.Name, Options = ConfigurationPropertyOptions.IsKey | ConfigurationPropertyOptions.IsRequired)] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[ConfigurationStrings.Name]; } set { if (String.IsNullOrEmpty(value)) { value = String.Empty; } base[ConfigurationStrings.Name] = value; } } [ConfigurationProperty(ConfigurationStrings.CloseTimeout, DefaultValue=ServiceDefaults.CloseTimeoutString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan CloseTimeout { get { return (TimeSpan)base[ConfigurationStrings.CloseTimeout]; } set { base[ConfigurationStrings.CloseTimeout] = value; } } [ConfigurationProperty(ConfigurationStrings.OpenTimeout, DefaultValue=ServiceDefaults.OpenTimeoutString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan OpenTimeout { get { return (TimeSpan)base[ConfigurationStrings.OpenTimeout]; } set { base[ConfigurationStrings.OpenTimeout] = value; } } [ConfigurationProperty(ConfigurationStrings.ReceiveTimeout, DefaultValue=ServiceDefaults.ReceiveTimeoutString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan ReceiveTimeout { get { return (TimeSpan)base[ConfigurationStrings.ReceiveTimeout]; } set { base[ConfigurationStrings.ReceiveTimeout] = value; } } [ConfigurationProperty(ConfigurationStrings.SendTimeout, DefaultValue=ServiceDefaults.SendTimeoutString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [ServiceModelTimeSpanValidator(MinValueString = ConfigurationStrings.TimeSpanZero)] public TimeSpan SendTimeout { get { return (TimeSpan)base[ConfigurationStrings.SendTimeout]; } set { base[ConfigurationStrings.SendTimeout] = value; } } public void ApplyConfiguration(Binding binding) { if (null == binding) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("binding"); } if (binding.GetType() != this.BindingElementType) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.ConfigInvalidTypeForBinding, this.BindingElementType.AssemblyQualifiedName, binding.GetType().AssemblyQualifiedName)); } // The properties binding.Name and this.Name are actually two different things: // - binding.Name corresponds to how the WSDL for this binding is surfaced, // it is used in conjunction with binding.Namespace // - this.Name is a token used as a key in the binding collection to identify // a specific bucket of configuration settings. // Thus, the Name property is skipped here. binding.CloseTimeout = this.CloseTimeout; binding.OpenTimeout = this.OpenTimeout; binding.ReceiveTimeout = this.ReceiveTimeout; binding.SendTimeout = this.SendTimeout; this.OnApplyConfiguration(binding); } protected virtual internal void InitializeFrom(Binding binding) { if (null == binding) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("binding"); } if (binding.GetType() != this.BindingElementType) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.ConfigInvalidTypeForBinding, this.BindingElementType.AssemblyQualifiedName, binding.GetType().AssemblyQualifiedName)); } // The properties binding.Name and this.Name are actually two different things: // - binding.Name corresponds to how the WSDL for this binding is surfaced, // it is used in conjunction with binding.Namespace // - this.Name is a token used as a key in the binding collection to identify // a specific bucket of configuration settings. // Thus, the Name property is skipped here. this.CloseTimeout = binding.CloseTimeout; this.OpenTimeout = binding.OpenTimeout; this.ReceiveTimeout = binding.ReceiveTimeout; this.SendTimeout = binding.SendTimeout; } protected abstract void OnApplyConfiguration(Binding binding); ////// Critical - accesses critical field contextHelper /// [SecurityCritical] protected override void Reset(ConfigurationElement parentElement) { this.contextHelper.OnReset(parentElement); base.Reset(parentElement); } ContextInformation IConfigurationContextProviderInternal.GetEvaluationContext() { return this.EvaluationContext; } ////// Critical -- accesses critical field contextHelper /// RequiresReview -- the return value will be used for a security decision -- see comment in interface definition /// [SecurityCritical] ContextInformation IConfigurationContextProviderInternal.GetOriginalEvaluationContext() { return this.contextHelper.GetOriginalContext(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
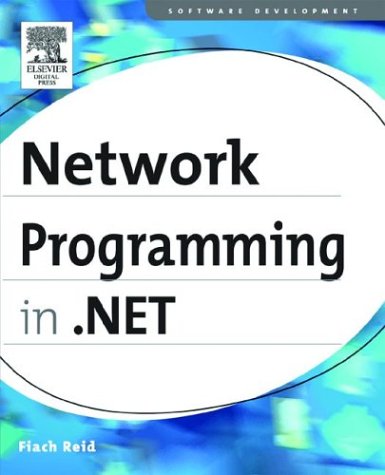
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameValueFileSectionHandler.cs
- ButtonPopupAdapter.cs
- UserControl.cs
- TransformBlockRequest.cs
- AccessorTable.cs
- AliasedExpr.cs
- Matrix.cs
- AlgoModule.cs
- _CookieModule.cs
- mongolianshape.cs
- Parameter.cs
- GeometryDrawing.cs
- ModelFactory.cs
- XsltException.cs
- path.cs
- TreePrinter.cs
- RegexReplacement.cs
- Transform.cs
- ServerIdentity.cs
- TextBox.cs
- ProfileParameter.cs
- TypeExtension.cs
- DataGridLinkButton.cs
- NestedContainer.cs
- StringArrayConverter.cs
- KeyEventArgs.cs
- ArithmeticException.cs
- ScrollEventArgs.cs
- XmlDataSource.cs
- EntityDataSourceContextCreatedEventArgs.cs
- CalendarBlackoutDatesCollection.cs
- RegisteredExpandoAttribute.cs
- ItemContainerGenerator.cs
- Stylesheet.cs
- WebPartTransformerAttribute.cs
- Context.cs
- XXXOnTypeBuilderInstantiation.cs
- ZipIOLocalFileBlock.cs
- DBSqlParserTableCollection.cs
- ThreadAbortException.cs
- ObjectConverter.cs
- WindowsRichEdit.cs
- SpotLight.cs
- RelationshipWrapper.cs
- ReaderWriterLock.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- SqlBulkCopyColumnMappingCollection.cs
- TraceEventCache.cs
- DesignerImageAdapter.cs
- BulletedListEventArgs.cs
- XmlWrappingReader.cs
- SimpleRecyclingCache.cs
- XmlSerializableWriter.cs
- EntityTransaction.cs
- TextSelection.cs
- KeySplineConverter.cs
- ScrollEvent.cs
- LogicalCallContext.cs
- RegisteredHiddenField.cs
- TimeoutException.cs
- assemblycache.cs
- TextRunTypographyProperties.cs
- FrameAutomationPeer.cs
- Transform3DGroup.cs
- TypeUtils.cs
- GridViewHeaderRowPresenter.cs
- SequenceDesigner.cs
- AlternateView.cs
- InstalledFontCollection.cs
- ComponentRenameEvent.cs
- DiscoveryInnerClientAdhocCD1.cs
- HttpConfigurationContext.cs
- WizardDesigner.cs
- MenuItemStyle.cs
- ComponentResourceKeyConverter.cs
- XDRSchema.cs
- SQLDateTimeStorage.cs
- PngBitmapDecoder.cs
- PreloadedPackages.cs
- Item.cs
- DataGridViewComboBoxEditingControl.cs
- TakeQueryOptionExpression.cs
- CaseCqlBlock.cs
- MSHTMLHost.cs
- CodeCommentStatementCollection.cs
- DataGridBeginningEditEventArgs.cs
- ProjectionPruner.cs
- FlowSwitchLink.cs
- ColorMap.cs
- ListBoxAutomationPeer.cs
- DbConvert.cs
- VirtualPathExtension.cs
- XPathNavigatorKeyComparer.cs
- FormViewRow.cs
- TimeStampChecker.cs
- FamilyTypeface.cs
- DataTransferEventArgs.cs
- IsolationInterop.cs
- DesignSurfaceManager.cs
- LocalBuilder.cs