Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Core / Presentation / FlowSwitchLink.cs / 1407647 / FlowSwitchLink.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Activities.Core.Presentation { using System; using System.Activities.Presentation; using System.Activities.Presentation.Model; using System.Activities.Presentation.View; using System.Activities.Statements; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Runtime; using System.Collections.Generic; using System.Windows.Data; using System.Globalization; using System.Activities.Presentation.PropertyEditing; using System.Activities.Presentation.Internal.PropertyEditing.Model; sealed class FlowSwitchLink: DependencyObject, IFlowSwitchLink { static DependencyProperty caseProperty = DependencyProperty.Register("Case", typeof(T), typeof(FlowSwitchLink ), new FrameworkPropertyMetadata(new PropertyChangedCallback(FlowSwitchLink .OnCasePropertyChanged))); static DependencyProperty isDefaultCaseProperty = DependencyProperty.Register("IsDefault", typeof(bool), typeof(FlowSwitchLink ), new FrameworkPropertyMetadata(new PropertyChangedCallback(FlowSwitchLink .OnIsDefaultCasePropertyChanged))); private FlowSwitch parentFlowSwitch; bool internalChange = false; ModelItem flowSwitchModelItem; const string DefaultConnectorViewStateKey = "Default"; const string CaseViewStateKeyAppendString = "Connector"; bool internalDefaultCaseChange = false; public FlowSwitchLink(ModelItem flowSwitchMI, T caseValue, bool isDefault) { flowSwitchModelItem = flowSwitchMI; object flowSwitch = flowSwitchModelItem.GetCurrentValue(); parentFlowSwitch = (FlowSwitch )flowSwitchModelItem.GetCurrentValue(); this.internalChange = true; this.internalDefaultCaseChange = true; if (!isDefault) { this.CaseObject = caseValue; } this.IsDefaultCase = isDefault; this.internalDefaultCaseChange = false; this.internalChange = false; } [BrowsableAttribute(false)] public ModelItem ModelItem { get; set; } public CaseKeyValidationCallbackDelegate ValidateCaseKey { get { return (object obj, out string reason) => { return GenericFlowSwitchHelper.ValidateCaseKey(obj, flowSwitchModelItem.Properties["Cases"], typeof(T), out reason); }; } } [BrowsableAttribute(false)] public FlowNode ParentFlowSwitch { get { return this.parentFlowSwitch; } set { this.parentFlowSwitch = value as FlowSwitch ; } } public bool IsDefaultCase { get { return (bool) GetValue(FlowSwitchLink .isDefaultCaseProperty); } set { SetValue(FlowSwitchLink .isDefaultCaseProperty, value); } } [Browsable(false)] public string CaseName { get { object value = GetValue(FlowSwitchLink .caseProperty); return GenericFlowSwitchHelper.GetString((T)value, typeof(T)); } } [Browsable(false)] public object CaseObject { get { return GetValue(FlowSwitchLink .caseProperty); } set { SetValue(FlowSwitchLink .caseProperty, value); } } public T Case { get { return (T) GetValue(FlowSwitchLink .caseProperty); } set { SetValue(FlowSwitchLink .caseProperty, value); } } public DependencyProperty CaseProperty { get { return caseProperty; } } public DependencyProperty IsDefaultCaseProperty { get { return isDefaultCaseProperty; } } [Browsable(false)] public Type GenericType { get { return typeof(T); } } bool ContainsKey(object key) { return this.parentFlowSwitch.Cases.ContainsKey((T)key); } void OnIsDefaultPropertyChanged(DependencyPropertyChangedEventArgs e) { bool isUndoRedoInProgress; FlowSwitchDesigner designer = ((FlowSwitchDesigner)((FlowSwitchDesigner)this.flowSwitchModelItem.View)); if (designer == null) { isUndoRedoInProgress = false; } else { isUndoRedoInProgress = designer.Context.Services.GetService ().IsUndoRedoInProgress; } if (!this.internalDefaultCaseChange && !isUndoRedoInProgress) { bool value = (bool)e.NewValue; bool oldValue = (bool)e.OldValue; if (value) { if (object.Equals(flowSwitchModelItem.Properties["Default"].Value, null)) { using (EditingScope es = (EditingScope)flowSwitchModelItem.BeginEdit(SR.FlowSwitchCaseRenameEditingScopeDesc)) { ModelItem flowNodeMI = GenericFlowSwitchHelper.GetCaseModelItem(flowSwitchModelItem.Properties["Cases"], this.CaseObject); GenericFlowSwitchHelper.RemoveCase(flowSwitchModelItem.Properties["Cases"], this.CaseObject); flowSwitchModelItem.Properties["Default"].SetValue(flowNodeMI); UpdateViewState(this.CaseName + CaseViewStateKeyAppendString, DefaultConnectorViewStateKey); this.internalChange = true; es.Complete(); } } else { internalDefaultCaseChange = true; this.IsDefaultCase = oldValue; throw FxTrace.Exception.AsError(new InvalidOperationException(SR.DefaultCaseExists)); } } else { if (oldValue) { using (EditingScope es = (EditingScope)flowSwitchModelItem.BeginEdit(SR.FlowSwitchCaseRenameEditingScopeDesc)) { ModelItem defaultCase = flowSwitchModelItem.Properties["Default"].Value; object uniqueCase = null; string errorMessage = string.Empty; Type typeArgument = typeof(T); if (GenericFlowSwitchHelper.CanBeGeneratedUniquely(typeArgument)) { string caseName = GenericFlowSwitchHelper.GetCaseName(flowSwitchModelItem.Properties["Cases"], typeArgument, out errorMessage); if (!string.IsNullOrEmpty(errorMessage)) { internalDefaultCaseChange = true; this.IsDefaultCase = oldValue; throw FxTrace.Exception.AsError(new InvalidOperationException(errorMessage)); } uniqueCase = GenericFlowSwitchHelper.GetObject(caseName, typeArgument); } else { FlowSwitchCaseEditorDialog editor = new FlowSwitchCaseEditorDialog(this.flowSwitchModelItem, ((FlowSwitchDesigner)this.flowSwitchModelItem.View).Context, ((FlowSwitchDesigner)this.flowSwitchModelItem.View), SR.ChangeCaseValue, this.flowSwitchModelItem.ItemType.GetGenericArguments()[0]); editor.WindowSizeToContent = SizeToContent.WidthAndHeight; if (!editor.ShowOkCancel()) { internalDefaultCaseChange = true; this.IsDefaultCase = oldValue; return; } uniqueCase = editor.Case; if (GenericFlowSwitchHelper.ContainsCaseKey(this.flowSwitchModelItem.Properties["Cases"], uniqueCase)) { internalDefaultCaseChange = true; this.IsDefaultCase = oldValue; throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidFlowSwitchCaseMessage)); } } flowSwitchModelItem.Properties["Default"].SetValue(null); this.internalChange = true; if (typeof(string) != typeof(T)) { this.ModelItem.Properties["Case"].SetValue(uniqueCase); GenericFlowSwitchHelper.AddCase(this.flowSwitchModelItem.Properties["Cases"], uniqueCase, defaultCase.GetCurrentValue()); } else { this.ModelItem.Properties["Case"].SetValue(uniqueCase); GenericFlowSwitchHelper.AddCase(this.flowSwitchModelItem.Properties["Cases"], uniqueCase, defaultCase.GetCurrentValue()); } UpdateViewState(DefaultConnectorViewStateKey, GenericFlowSwitchHelper.GetString(uniqueCase, typeof(T)) + CaseViewStateKeyAppendString); es.Complete(); this.internalChange = false; } this.internalDefaultCaseChange = false; } } } this.internalDefaultCaseChange = false; } void OnCasePropertyChanged(DependencyPropertyChangedEventArgs e) { bool isUndoRedoInProgress; FlowSwitchDesigner designer = ((FlowSwitchDesigner)this.flowSwitchModelItem.View); if (designer == null) { isUndoRedoInProgress = false; } else { isUndoRedoInProgress = designer.Context.Services.GetService ().IsUndoRedoInProgress; } if (!internalChange && !isUndoRedoInProgress) { T oldValue = (T)e.OldValue; T newValue = (T)e.NewValue; if (newValue is string && newValue != null) { newValue = (T) ((object)((string)((object)newValue)).Trim()); } string oldViewStateKey = string.Empty; if (!ContainsKey(newValue)) { using (EditingScope es = (EditingScope)flowSwitchModelItem.BeginEdit(SR.FlowSwitchCaseRenameEditingScopeDesc)) { ModelItem flowElementMI = null; flowElementMI = GenericFlowSwitchHelper.GetCaseModelItem(flowSwitchModelItem.Properties["Cases"], oldValue); GenericFlowSwitchHelper.RemoveCase(flowSwitchModelItem.Properties["Cases"], oldValue); oldViewStateKey = GenericFlowSwitchHelper.GetString(oldValue, typeof(T)) + CaseViewStateKeyAppendString; //Add the new value GenericFlowSwitchHelper.AddCase(flowSwitchModelItem.Properties["Cases"], newValue, flowElementMI.GetCurrentValue()); //Update the viewstate for the flowswitch. UpdateViewState(oldViewStateKey, GenericFlowSwitchHelper.GetString(newValue, typeof(T)) + CaseViewStateKeyAppendString); //Making sure the value for Case is always trimmed. internalChange = true; this.ModelItem.Properties["Case"].SetValue(newValue); es.Complete(); } } else { internalChange = true; this.CaseObject = oldValue; throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidFlowSwitchCaseMessage)); } } internalChange = false; } void UpdateViewState(string oldValue, string newValue) { EditingContext context = flowSwitchModelItem.GetEditingContext(); ViewStateService viewStateService = (ViewStateService)context.Services.GetService(typeof(ViewStateService)); if (viewStateService != null) { object viewState = viewStateService.RetrieveViewState(flowSwitchModelItem, oldValue); if (viewState != null) { viewStateService.StoreViewStateWithUndo(flowSwitchModelItem, oldValue, null); viewStateService.StoreViewStateWithUndo(flowSwitchModelItem, newValue, viewState); } } } static void OnCasePropertyChanged(DependencyObject dependencyObject, DependencyPropertyChangedEventArgs e) { FlowSwitchLink link = (FlowSwitchLink )dependencyObject; link.OnCasePropertyChanged(e); } static void OnIsDefaultCasePropertyChanged(DependencyObject dependencyObject, DependencyPropertyChangedEventArgs e) { FlowSwitchLink link = (FlowSwitchLink )dependencyObject; link.OnIsDefaultPropertyChanged(e); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
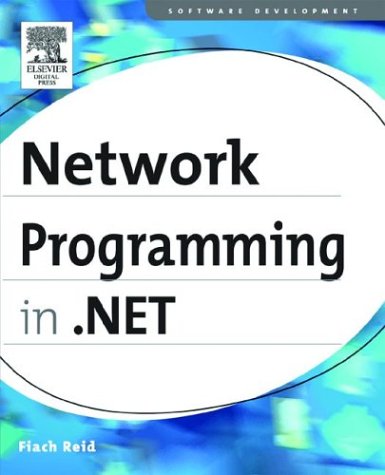
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlAliaser.cs
- ToolStripDesignerAvailabilityAttribute.cs
- ConstNode.cs
- PipeException.cs
- HierarchicalDataSourceControl.cs
- DocumentScope.cs
- ByeMessage11.cs
- DPAPIProtectedConfigurationProvider.cs
- SerializeAbsoluteContext.cs
- Animatable.cs
- QueryServiceConfigHandle.cs
- WebBrowsableAttribute.cs
- Triangle.cs
- Paragraph.cs
- SHA1Managed.cs
- InertiaRotationBehavior.cs
- ELinqQueryState.cs
- HMACSHA256.cs
- BrowserInteropHelper.cs
- DataSetUtil.cs
- TraceUtils.cs
- RayHitTestParameters.cs
- GlobalizationAssembly.cs
- HttpChannelFactory.cs
- DaylightTime.cs
- XmlIterators.cs
- DbConnectionPool.cs
- ItemCollectionEditor.cs
- ControlParameter.cs
- HtmlElementEventArgs.cs
- AlternationConverter.cs
- ConfigurationValues.cs
- ProjectedSlot.cs
- HostedHttpRequestAsyncResult.cs
- State.cs
- CustomAttributeFormatException.cs
- SoapObjectWriter.cs
- IDispatchConstantAttribute.cs
- EventSourceCreationData.cs
- DataSourceSelectArguments.cs
- PrinterResolution.cs
- FileDialog.cs
- AlphabeticalEnumConverter.cs
- CapabilitiesRule.cs
- ToolStripRenderer.cs
- TempEnvironment.cs
- ImmComposition.cs
- AttachedAnnotationChangedEventArgs.cs
- BuildManager.cs
- PolyLineSegmentFigureLogic.cs
- BamlCollectionHolder.cs
- CriticalFinalizerObject.cs
- WebDescriptionAttribute.cs
- TypeSystem.cs
- _FixedSizeReader.cs
- ThousandthOfEmRealDoubles.cs
- StatusBar.cs
- DataObjectMethodAttribute.cs
- TdsParser.cs
- While.cs
- ServiceSettingsResponseInfo.cs
- ChtmlTextBoxAdapter.cs
- HttpFileCollection.cs
- SqlConnectionManager.cs
- SiteOfOriginContainer.cs
- MenuItemStyleCollection.cs
- BaseParagraph.cs
- Int32KeyFrameCollection.cs
- ApplicationContext.cs
- Base64Decoder.cs
- HandledMouseEvent.cs
- UserControlParser.cs
- LocalizableAttribute.cs
- Math.cs
- CodeExpressionCollection.cs
- ExpressionConverter.cs
- ObjectConverter.cs
- ToolStripActionList.cs
- EventHandlerList.cs
- EdmProviderManifest.cs
- ApplicationException.cs
- HostedBindingBehavior.cs
- BindingUtils.cs
- OdbcParameterCollection.cs
- ClientSideQueueItem.cs
- WebDisplayNameAttribute.cs
- TimeSpanValidatorAttribute.cs
- HtmlInputControl.cs
- MDIWindowDialog.cs
- SmiContext.cs
- PeerTransportListenAddressValidatorAttribute.cs
- QilParameter.cs
- SoapAttributeAttribute.cs
- XMLDiffLoader.cs
- SchemaSetCompiler.cs
- ProcessManager.cs
- X509Utils.cs
- ObfuscateAssemblyAttribute.cs
- NodeFunctions.cs
- StringConcat.cs