Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / CaseCqlBlock.cs / 1 / CaseCqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class to capture CqlBlocks responsible for case statements -- these // are statements needed for generating the variables in the // multiconstants, i.e., complex types, entities, discriminators, etc internal class CaseCqlBlock : CqlBlock { #region Constructors // effects: Creates a case statememt CqlBlock with SELECT (slots), // FROM (child -- note has only has one child), WHERE (true), AS // (blockAliasNum). caseSlot indicates which slot in slots // corresponds to the single case statement being generated by this block internal CaseCqlBlock(SlotInfo[] slots, int caseSlot, CqlBlock child, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) : base(slots, new List(new CqlBlock[]{child}), whereClause, identifiers, blockAliasNum) { m_caseSlotInfo = slots[caseSlot]; } #endregion #region Fields private SlotInfo m_caseSlotInfo; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part StringUtil.IndentNewLine(builder, indentLevel); // Since we know that the case statement starts on a new line, we // can add a comment after SELECT Debug.Assert(m_caseSlotInfo.MemberPath != null, "We only construct real slots not boolean slots"); builder.Append("SELECT "); if (isTopLevel) { builder.Append("VALUE "); } builder.Append("-- Constructing ") .Append(m_caseSlotInfo.MemberPath.LastComponentName); Debug.Assert(Children.Count == 1, "Case can have exactly one child"); CqlBlock childBlock = Children[0]; // First emit the case statement "CASE ... END AS Alias" m_caseSlotInfo.AsCql(builder, childBlock.CqlAlias, indentLevel); if (false == isTopLevel) { builder.Append(" AS ") .Append(m_caseSlotInfo.CqlFieldAlias); } // Now get the remaining slots bool isFirst = true; // CHANGE_[....]_IMPROVE: Try to leverage GenerateProjectedtList foreach (SlotInfo slot in Slots) { if (false == slot.IsRequiredByParent || object.ReferenceEquals(slot, m_caseSlotInfo)) { continue; } if (isFirst == true) { // Add , after END and then start new line for first field builder.Append(", "); StringUtil.IndentNewLine(builder, indentLevel + 1); } else { // Simply add a comma for the next field builder.Append(", "); } slot.AsCql(builder, childBlock.CqlAlias, indentLevel); isFirst = false; } // The FROM part: FROM (ChildView) AS AliasName StringUtil.IndentNewLine(builder, indentLevel); builder.Append("FROM ("); // Get the child childBlock.AsCql(builder, false, indentLevel + 1); StringUtil.IndentNewLine(builder, indentLevel); builder.Append(") AS ") .Append(childBlock.CqlAlias); // Get the WHERE part only when the expression is not simply TRUE if (false == BoolExpression.EqualityComparer.Equals(WhereClause, BoolExpression.True)) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("WHERE "); WhereClause.AsCql(builder, childBlock.CqlAlias); } return builder; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class to capture CqlBlocks responsible for case statements -- these // are statements needed for generating the variables in the // multiconstants, i.e., complex types, entities, discriminators, etc internal class CaseCqlBlock : CqlBlock { #region Constructors // effects: Creates a case statememt CqlBlock with SELECT (slots), // FROM (child -- note has only has one child), WHERE (true), AS // (blockAliasNum). caseSlot indicates which slot in slots // corresponds to the single case statement being generated by this block internal CaseCqlBlock(SlotInfo[] slots, int caseSlot, CqlBlock child, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) : base(slots, new List(new CqlBlock[]{child}), whereClause, identifiers, blockAliasNum) { m_caseSlotInfo = slots[caseSlot]; } #endregion #region Fields private SlotInfo m_caseSlotInfo; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part StringUtil.IndentNewLine(builder, indentLevel); // Since we know that the case statement starts on a new line, we // can add a comment after SELECT Debug.Assert(m_caseSlotInfo.MemberPath != null, "We only construct real slots not boolean slots"); builder.Append("SELECT "); if (isTopLevel) { builder.Append("VALUE "); } builder.Append("-- Constructing ") .Append(m_caseSlotInfo.MemberPath.LastComponentName); Debug.Assert(Children.Count == 1, "Case can have exactly one child"); CqlBlock childBlock = Children[0]; // First emit the case statement "CASE ... END AS Alias" m_caseSlotInfo.AsCql(builder, childBlock.CqlAlias, indentLevel); if (false == isTopLevel) { builder.Append(" AS ") .Append(m_caseSlotInfo.CqlFieldAlias); } // Now get the remaining slots bool isFirst = true; // CHANGE_[....]_IMPROVE: Try to leverage GenerateProjectedtList foreach (SlotInfo slot in Slots) { if (false == slot.IsRequiredByParent || object.ReferenceEquals(slot, m_caseSlotInfo)) { continue; } if (isFirst == true) { // Add , after END and then start new line for first field builder.Append(", "); StringUtil.IndentNewLine(builder, indentLevel + 1); } else { // Simply add a comma for the next field builder.Append(", "); } slot.AsCql(builder, childBlock.CqlAlias, indentLevel); isFirst = false; } // The FROM part: FROM (ChildView) AS AliasName StringUtil.IndentNewLine(builder, indentLevel); builder.Append("FROM ("); // Get the child childBlock.AsCql(builder, false, indentLevel + 1); StringUtil.IndentNewLine(builder, indentLevel); builder.Append(") AS ") .Append(childBlock.CqlAlias); // Get the WHERE part only when the expression is not simply TRUE if (false == BoolExpression.EqualityComparer.Equals(WhereClause, BoolExpression.True)) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("WHERE "); WhereClause.AsCql(builder, childBlock.CqlAlias); } return builder; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
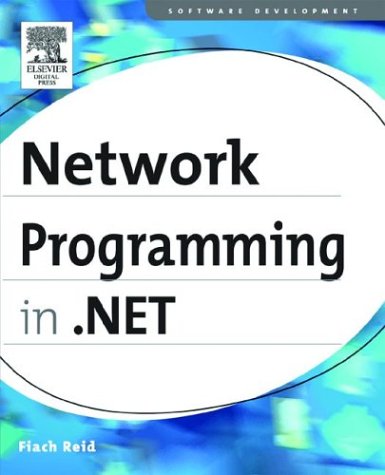
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlShimManager.cs
- BitmapFrame.cs
- OracleMonthSpan.cs
- ToolZone.cs
- CodeRegionDirective.cs
- WindowsComboBox.cs
- JapaneseCalendar.cs
- TypedDatasetGenerator.cs
- DataServiceStreamProviderWrapper.cs
- AdapterUtil.cs
- NativeMethods.cs
- mactripleDES.cs
- ImageField.cs
- DocumentEventArgs.cs
- PositiveTimeSpanValidator.cs
- ObjectCloneHelper.cs
- ToolBarPanel.cs
- SpeechEvent.cs
- BindingsCollection.cs
- ListView.cs
- DataGridColumn.cs
- Geometry3D.cs
- DescendantOverDescendantQuery.cs
- ProtectedConfigurationSection.cs
- Rights.cs
- PerformanceCountersElement.cs
- PlanCompilerUtil.cs
- SystemFonts.cs
- QueryOperationResponseOfT.cs
- ObjectParameterCollection.cs
- CharStorage.cs
- webeventbuffer.cs
- HttpCookiesSection.cs
- PlanCompilerUtil.cs
- OLEDB_Util.cs
- KeyPressEvent.cs
- X509Utils.cs
- TypeHelper.cs
- DialogDivider.cs
- SourceFileBuildProvider.cs
- WindowInteropHelper.cs
- Component.cs
- OutputCacheProfileCollection.cs
- Trace.cs
- AggregatePushdown.cs
- QilList.cs
- XmlSerializationWriter.cs
- Image.cs
- _AuthenticationState.cs
- RegexFCD.cs
- InvalidAsynchronousStateException.cs
- TokenBasedSetEnumerator.cs
- XmlDocument.cs
- OdbcCommandBuilder.cs
- ContentElement.cs
- SiteMembershipCondition.cs
- Debugger.cs
- DesigntimeLicenseContext.cs
- ObjectDataSourceFilteringEventArgs.cs
- ClientApiGenerator.cs
- DateTimeOffsetConverter.cs
- BuildProvider.cs
- SemanticKeyElement.cs
- InkCanvasAutomationPeer.cs
- XMLUtil.cs
- basecomparevalidator.cs
- HtmlHead.cs
- CompilationLock.cs
- ForwardPositionQuery.cs
- LineGeometry.cs
- ReadingWritingEntityEventArgs.cs
- SendMailErrorEventArgs.cs
- Pens.cs
- XmlWriterSettings.cs
- XmlSerializableWriter.cs
- DrawingVisual.cs
- Binding.cs
- TextTreeTextElementNode.cs
- SchemaImporter.cs
- WindowsSpinner.cs
- PathFigureCollectionConverter.cs
- SiteMapPath.cs
- InteropTrackingRecord.cs
- TypeDelegator.cs
- SafeFindHandle.cs
- SerializableAttribute.cs
- TableItemProviderWrapper.cs
- WSSecurityTokenSerializer.cs
- XslException.cs
- PageClientProxyGenerator.cs
- Win32PrintDialog.cs
- LabelEditEvent.cs
- SimpleWebHandlerParser.cs
- WindowsSecurityToken.cs
- ExtensionSimplifierMarkupObject.cs
- ExpressionLexer.cs
- Html32TextWriter.cs
- SapiRecognizer.cs
- ProfileEventArgs.cs
- InputLanguageCollection.cs