Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / BindingsCollection.cs / 1305376 / BindingsCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using Microsoft.Win32; using System.Diagnostics; using System.ComponentModel; using System.Collections; ////// /// [DefaultEvent("CollectionChanged")] public class BindingsCollection : System.Windows.Forms.BaseCollection { private ArrayList list; private CollectionChangeEventHandler onCollectionChanging; private CollectionChangeEventHandler onCollectionChanged; // internalonly internal BindingsCollection() { } ///Represents a collection of data bindings on a control. ///public override int Count { get { if (list == null) { return 0; } return base.Count; } } /// /// /// /// protected override ArrayList List { get { if (list == null) list = new ArrayList(); return list; } } ////// Gets the bindings in the collection as an object. /// ////// /// public Binding this[int index] { get { return (Binding) List[index]; } } ///Gets the ///at the specified index. // internalonly internal protected void Add(Binding binding) { CollectionChangeEventArgs ccevent = new CollectionChangeEventArgs(CollectionChangeAction.Add, binding); OnCollectionChanging(ccevent); AddCore(binding); OnCollectionChanged(ccevent); } // internalonly /// /// /// /// protected virtual void AddCore(Binding dataBinding) { if (dataBinding == null) throw new ArgumentNullException("dataBinding"); List.Add(dataBinding); } ////// Adds a ////// to the collection. /// /// /// [SRDescription(SR.collectionChangingEventDescr)] public event CollectionChangeEventHandler CollectionChanging { add { onCollectionChanging += value; } remove { onCollectionChanging -= value; } } ////// Occurs when the collection is about to change. /// ////// /// [SRDescription(SR.collectionChangedEventDescr)] public event CollectionChangeEventHandler CollectionChanged { add { onCollectionChanged += value; } remove { onCollectionChanged -= value; } } // internalonly ////// Occurs when the collection is changed. /// ///internal protected void Clear() { CollectionChangeEventArgs ccevent = new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null); OnCollectionChanging(ccevent); ClearCore(); OnCollectionChanged(ccevent); } // internalonly /// /// /// /// protected virtual void ClearCore() { List.Clear(); } ////// Clears the collection of any members. /// ////// /// protected virtual void OnCollectionChanging(CollectionChangeEventArgs e) { if (onCollectionChanging != null) { onCollectionChanging(this, e); } } ////// Raises the ///event. /// /// /// protected virtual void OnCollectionChanged(CollectionChangeEventArgs ccevent) { if (onCollectionChanged != null) { onCollectionChanged(this, ccevent); } } ////// Raises the ///event. /// // internalonly internal protected void Remove(Binding binding) { CollectionChangeEventArgs ccevent = new CollectionChangeEventArgs(CollectionChangeAction.Remove, binding); OnCollectionChanging(ccevent); RemoveCore(binding); OnCollectionChanged(ccevent); } /// // internalonly internal protected void RemoveAt(int index) { Remove(this[index]); } // internalonly /// /// /// /// protected virtual void RemoveCore(Binding dataBinding) { List.Remove(dataBinding); } ////// Removes the specified ///from the collection. /// // internalonly internal protected bool ShouldSerializeMyAll() { return Count > 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
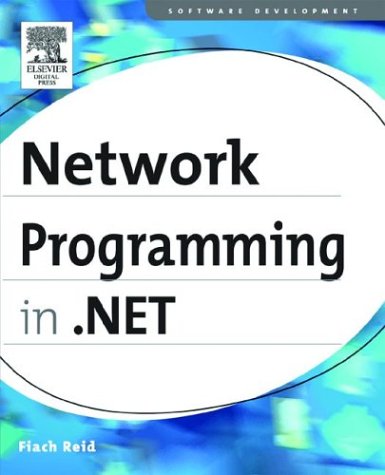
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SR.cs
- TextDecoration.cs
- TextBlockAutomationPeer.cs
- BigInt.cs
- LambdaCompiler.Logical.cs
- HtmlInputText.cs
- SudsWriter.cs
- ConfigDefinitionUpdates.cs
- URLEditor.cs
- DebugViewWriter.cs
- TagMapInfo.cs
- CompilationUtil.cs
- OleDbTransaction.cs
- PointF.cs
- ObjectTypeMapping.cs
- PackagePart.cs
- ResourcePermissionBaseEntry.cs
- ListViewPagedDataSource.cs
- SqlSelectClauseBuilder.cs
- RelationshipDetailsRow.cs
- StrokeFIndices.cs
- TypeTypeConverter.cs
- EmptyReadOnlyDictionaryInternal.cs
- HTTPNotFoundHandler.cs
- UpdateDelegates.Generated.cs
- SessionKeyExpiredException.cs
- SqlUdtInfo.cs
- EFColumnProvider.cs
- DbProviderFactoriesConfigurationHandler.cs
- EditorBrowsableAttribute.cs
- CreateParams.cs
- RequestQueue.cs
- Regex.cs
- DataGridViewComboBoxEditingControl.cs
- KnownTypesHelper.cs
- LoggedException.cs
- QueryExecutionOption.cs
- ToolStripPanelCell.cs
- HttpTransportSecurity.cs
- Int32CollectionValueSerializer.cs
- ProjectionPathBuilder.cs
- ResourceReferenceExpressionConverter.cs
- ProtocolsSection.cs
- MsmqDecodeHelper.cs
- Currency.cs
- RenderTargetBitmap.cs
- ClientTargetCollection.cs
- DescendantQuery.cs
- DragDeltaEventArgs.cs
- ConfigurationValidatorAttribute.cs
- DependencyObjectProvider.cs
- ContentPosition.cs
- EFDataModelProvider.cs
- AnimationClockResource.cs
- Sql8ExpressionRewriter.cs
- TimeSpanValidatorAttribute.cs
- PersistenceTypeAttribute.cs
- CharacterBuffer.cs
- WebResponse.cs
- BindMarkupExtensionSerializer.cs
- DbTransaction.cs
- Setter.cs
- NavigationEventArgs.cs
- SimpleTypeResolver.cs
- XmlBindingWorker.cs
- RefreshEventArgs.cs
- DataObjectEventArgs.cs
- CustomErrorCollection.cs
- RealProxy.cs
- FileStream.cs
- ClientEventManager.cs
- ResourceManagerWrapper.cs
- Material.cs
- SpellerHighlightLayer.cs
- TrustManager.cs
- WSHttpBinding.cs
- ModelTreeManager.cs
- HighlightVisual.cs
- GZipDecoder.cs
- ConfigurationException.cs
- ActivityDesigner.cs
- ToolStripArrowRenderEventArgs.cs
- CodeChecksumPragma.cs
- XmlNodeReader.cs
- CompilerTypeWithParams.cs
- ColorBlend.cs
- XmlValidatingReader.cs
- AsyncOperation.cs
- ReachVisualSerializerAsync.cs
- Inflater.cs
- Emitter.cs
- Sql8ExpressionRewriter.cs
- HostedHttpTransportManager.cs
- UnsafeNetInfoNativeMethods.cs
- UpdatePanelControlTrigger.cs
- ColorContextHelper.cs
- JsonGlobals.cs
- DataGridViewCheckBoxColumn.cs
- Int64Animation.cs
- XmlSerializerNamespaces.cs