Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / OperatorExpressions.cs / 4 / OperatorExpressions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { #region Boolean Operators ////// Represents the logical And of two Boolean arguments. /// ///DbAndExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbAndExpression : DbBinaryExpression { internal DbAndExpression(DbCommandTree commandTree, DbExpression left, DbExpression right) : base(commandTree, DbExpressionKind.And) { EntityUtil.CheckArgumentNull(left, "Left"); EntityUtil.CheckArgumentNull(right, "Right"); TypeUsage resultType = TypeHelpers.GetCommonTypeUsage(left.ResultType, right.ResultType); if (null == resultType || !TypeSemantics.IsPrimitiveType(resultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_And_BooleanArgumentsRequired); } // // Apply constraints to the left and right arguments and set initial values // this.LeftLink.SetExpectedType(left.ResultType); this.LeftLink.InitializeValue(left); this.RightLink.SetExpectedType(right.ResultType); this.RightLink.InitializeValue(right); // // Result Type is always Boolean // this.ResultType = resultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Or of two Boolean arguments. /// ///DbOrExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOrExpression : DbBinaryExpression { internal DbOrExpression(DbCommandTree commandTree, DbExpression left, DbExpression right) : base(commandTree, DbExpressionKind.Or) { EntityUtil.CheckArgumentNull(left, "Left"); EntityUtil.CheckArgumentNull(right, "Right"); TypeUsage resultType = TypeHelpers.GetCommonTypeUsage(left.ResultType, right.ResultType); if (null == resultType || !TypeSemantics.IsPrimitiveType(resultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Or_BooleanArgumentsRequired); } // // Apply constraints to the left and right arguments and set initial values // this.LeftLink.SetExpectedType(left.ResultType); this.LeftLink.InitializeValue(left); this.RightLink.SetExpectedType(right.ResultType); this.RightLink.InitializeValue(right); // // Result Type is always Boolean // this.ResultType = resultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Not of a single Boolean argument. /// ///DbNotExpression requires that its argument has a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNotExpression : DbUnaryExpression { internal DbNotExpression(DbCommandTree commandTree, DbExpression arg) : base(commandTree, DbExpressionKind.Not) { EntityUtil.CheckArgumentNull(arg, "Argument"); // // Argument to Not must have Boolean result type // if (!TypeSemantics.IsPrimitiveType(arg.ResultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Not_BooleanArgumentRequired); } this.ArgumentLink.SetExpectedType(arg.ResultType); this.ArgumentLink.InitializeValue(arg); this.ResultType = arg.ResultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #endregion /// /// Represents an arithmetic operation (addition, subtraction, multiplication, division, modulo or negation) applied to two numeric arguments. /// ///DbArithmeticExpression requires that its arguments have a common numeric result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbArithmeticExpression : DbExpression { private readonly ExpressionList _args; internal DbArithmeticExpression(DbCommandTree commandTree, DbExpressionKind kind, IListargs) : base(commandTree, kind) { EntityUtil.CheckArgumentNull(args, "args"); Debug.Assert( DbExpressionKind.Divide == kind || DbExpressionKind.Minus == kind || DbExpressionKind.Modulo == kind || DbExpressionKind.Multiply == kind || DbExpressionKind.Plus == kind || DbExpressionKind.UnaryMinus == kind, "Invalid DbExpressionKind used in DbArithmeticExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); Debug.Assert( (DbExpressionKind.UnaryMinus == kind && 1 == args.Count) || 2 == args.Count, "Incorrect number of arguments specified to DbArithmeticExpression" ); // // Construct a new ExpressionList to contain the arguments // ExpressionList argList = new ExpressionList("Arguments", commandTree, args.Count); // // Set the current elements without constraining the expected element type // argList.SetElements(args); // // Ensure that a numeric common type exists for the arguments // TypeUsage commonType = argList.GetCommonElementType(); if (TypeSemantics.IsNullOrNullType(commonType) || !TypeSemantics.IsNumericType(commonType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Arithmetic_NumericCommonType); } // // Constrain the type of elements in the list to the common numeric type // argList.SetExpectedElementType(commonType); this._args = argList; this.ResultType = commonType; } /// /// Gets the list of expressions that define the current arguments. /// ////// The public IListArguments
property returns a fixed-size list ofelements. /// requires that all elements of it's Arguments
list /// have a common numeric result type. ///Arguments { get { return _args; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a Case When...Then...Else logical operation. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCaseExpression : DbExpression { private ExpressionList _when; private ExpressionList _then; private ExpressionLink _else; internal DbCaseExpression(DbCommandTree cmdTree, IListwhens, IList thens, DbExpression elseExpr) : base(cmdTree, DbExpressionKind.Case) { // // Whens/Thens cannot be null // (nor can Else, but this is enforced when the // ExpressionLink for the Else property is created, below). // EntityUtil.CheckArgumentNull(whens, "whens"); EntityUtil.CheckArgumentNull(thens, "thens"); // // The number of 'When's must equal the number of 'Then's. // if (whens.Count != thens.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_WhensMustEqualThens); } // // There must be at least one clause in the case expression. // if (0 == whens.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_AtLeastOneClause); } // // All 'When's must produce a Boolean result, and a common (non-null) result type must exist // TypeUsage resultType = null; for(int idx = 0; idx < whens.Count; idx++) { if (null == thens[idx]) { throw EntityUtil.ArgumentNull(CommandTreeUtils.FormatIndex("Thens", idx)); } if (null == resultType) { resultType = thens[idx].ResultType; } else { resultType = TypeHelpers.GetCommonTypeUsage(thens[idx].ResultType, resultType); if (null == resultType) { break; } } } if (resultType != null && elseExpr != null) { resultType = TypeHelpers.GetCommonTypeUsage(elseExpr.ResultType, resultType); } if (TypeSemantics.IsNullOrNullType(resultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_InvalidResultType); } // // Initialize the When clauses. Elements must be promotable to Boolean. // NullType is specifically not allowed as the Type of a When element. // _when = new ExpressionList("When", cmdTree, PrimitiveTypeKind.Boolean, whens); // // Initialize the Then clauses. Elements must be promotable to the common result type. // _then = new ExpressionList("Then", cmdTree, resultType, thens); // // Initialize the Else clause. Values must be promotable to the common result type. // _else = new ExpressionLink("Else", cmdTree, resultType, elseExpr); // // The result type of DbCaseExpression is the common result type // this.ResultType = resultType; } /// /// Gets the When clauses of this DbCaseExpression. /// public IListWhen { get { return _when; } } /// /// Gets the Then clauses of this DbCaseExpression. /// public IListThen { get { return _then; } } /// /// Gets or sets the Else clause of this DbCaseExpression. /// ///The expression is null ////// The expression is not associated with the DbCaseExpression's command tree, /// or its result type is not equal or promotable to the result type of the DbCaseExpression /// public DbExpression Else { get { return _else.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _else.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a cast operation applied to a polymorphic argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCastExpression : DbUnaryExpression { internal DbCastExpression(DbCommandTree cmdTree, TypeUsage type, DbExpression arg) : base(cmdTree, DbExpressionKind.Cast) { // // Verify the type to cast to. Casting to NullType is not allowed // cmdTree.TypeHelper.CheckType(type); // // Verify that the cast is allowed // if (!TypeSemantics.IsCastAllowed(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Cast_InvalidCast(TypeHelpers.GetFullName(arg.ResultType), TypeHelpers.GetFullName(type))); } // // Set the Argument constraints and initialize the argument value // this.ArgumentLink.InitializeValue(arg); this.ArgumentLink.SetExpectedType(arg.ResultType); this.ResultType = type; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a comparison operation (equality, greater than, greather than or equal, less than, less than or equal, inequality) applied to two arguments. /// ////// DbComparisonExpression requires that its arguments have a common result type /// that is equality comparable (for [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbComparisonExpression : DbBinaryExpression { internal DbComparisonExpression(DbCommandTree commandTree, DbExpressionKind kind, DbExpression left, DbExpression right) : base(commandTree, kind) { Debug.Assert( DbExpressionKind.Equals == kind || DbExpressionKind.LessThan == kind || DbExpressionKind.LessThanOrEquals == kind || DbExpressionKind.GreaterThan == kind || DbExpressionKind.GreaterThanOrEquals == kind || DbExpressionKind.NotEquals == kind, "Invalid DbExpressionKind used in DbComparisonExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); // // Initialize the Left and Right arguments to perform Null value and NullType checking // this.LeftLink.InitializeValue(left); this.RightLink.InitializeValue(right); // // Ensure that the types of the arguments are comparable // this.CheckComparison(left, right); // // Register for notifications that the left or right argument is changing. // The arguments can be freely substituted provided that when a value is changed, // the result type of the new expression value and the result type of the unchanged argument are comparable. // this.LeftLink.ValueChanging += new ExpressionLinkConstraint(this.ArgumentChanging); this.RightLink.ValueChanging += new ExpressionLinkConstraint(this.ArgumentChanging); // // Result Type is always Boolean // this.ResultType = commandTree.TypeHelper.CreateBooleanResultType(); } ///.Equals and .NotEquals), /// order comparable (for .GreaterThan and .LessThan), /// or both (for .GreaterThanOrEquals and .LessThanOrEquals). /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } private void ArgumentChanging(ExpressionLink link, DbExpression newValue) { // // If the Left expression is changing then the Right expression should be compared // to the new value, and vice-versa. // if (this.LeftLink == link) { this.CheckComparison(newValue, this.Right); } else { this.CheckComparison(this.Left, newValue); } } private void CheckComparison(DbExpression left, DbExpression right) { // // A comparison of the specified kind must exist between the left and right arguments // bool equality = true; bool order = true; if (DbExpressionKind.GreaterThanOrEquals == this.ExpressionKind || DbExpressionKind.LessThanOrEquals == this.ExpressionKind) { equality = TypeSemantics.IsEqualComparableTo(left.ResultType, right.ResultType); order = TypeSemantics.IsOrderComparableTo(left.ResultType, right.ResultType); } else if (DbExpressionKind.Equals == this.ExpressionKind || DbExpressionKind.NotEquals == this.ExpressionKind) { equality = TypeSemantics.IsEqualComparableTo(left.ResultType, right.ResultType); } else { order = TypeSemantics.IsOrderComparableTo(left.ResultType, right.ResultType); } if(!equality || !order) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Comparison_ComparableRequired); } } } /// /// Represents empty set determination applied to a single set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsEmptyExpression : DbUnaryExpression { internal DbIsEmptyExpression(DbCommandTree cmdTree, DbExpression arg) : base(cmdTree, DbExpressionKind.IsEmpty, arg) { // // Ensure that the Argument is of a collection type // this.CheckCollectionArgument(); // // Result Type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents null determination applied to a single argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsNullExpression : DbUnaryExpression { internal DbIsNullExpression(DbCommandTree cmdTree, DbExpression arg, bool isRowTypeArgumentAllowed) : base(cmdTree, DbExpressionKind.IsNull) { // // The argument cannot be of a collection type // if (TypeSemantics.IsCollectionType(arg.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_IsNull_CollectionNotAllowed); } // // ensure argument type is valid for this operation // if (!TypeHelpers.IsValidIsNullOpType(arg.ResultType) && (!isRowTypeArgumentAllowed || !TypeSemantics.IsRowType(arg.ResultType))) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_IsNull_InvalidType); } // // Set the Argument constraints and initialize the Argument value // ArgumentLink.SetExpectedType is not called - any expression can be substituted freely for the argument expression // this.ArgumentLink.InitializeValue(arg); // // Result Type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type comparison of a single argument against the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsOfExpression : DbUnaryExpression { private TypeUsage _ofType; internal DbIsOfExpression(DbCommandTree cmdTree, DbExpressionKind isOfKind, TypeUsage type, DbExpression arg) : base(cmdTree, isOfKind) { Debug.Assert(DbExpressionKind.IsOf == this.ExpressionKind || DbExpressionKind.IsOfOnly == this.ExpressionKind, string.Format(CultureInfo.InvariantCulture, "Invalid DbExpressionKind used in DbIsOfExpression: {0}", Enum.GetName(typeof(DbExpressionKind), this.ExpressionKind))); // // Ensure the ofType is non-null, associated with the correct metadata workspace/dataspace, // is not NullType, and is polymorphic // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Verify that the IsOf operation is allowed // if (!TypeSemantics.IsValidPolymorphicCast(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } _ofType = type; // // Set the Argument constraints and initialize the Argument value // this.ArgumentLink.SetExpectedType(arg.ResultType); this.ArgumentLink.InitializeValue(arg); // // Return type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// Gets the type metadata that the type metadata of the argument should be compared to. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of elements of the specified type from the given set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOfTypeExpression : DbUnaryExpression { private TypeUsage _ofType; internal DbOfTypeExpression(DbCommandTree cmdTree, DbExpressionKind ofTypeKind, TypeUsage type, DbExpression arg) : base(cmdTree, ofTypeKind, arg) { Debug.Assert(DbExpressionKind.OfType == ofTypeKind || DbExpressionKind.OfTypeOnly == ofTypeKind, "ExpressionKind for DbOfTypeExpression must be OfType or OfTypeOnly"); // // Ensure that the type is non-null and valid - from the same metadata collection and dataspace and the command tree. // The type is also not allowed to be NullType. // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Ensure that the argument is actually of a collection type. // this.CheckCollectionArgument(); // // Verify that the OfType operation is allowed // #if DEBUG CollectionType argCollType = null; bool isCollType = TypeHelpers.TryGetEdmType(arg.ResultType, out argCollType); Debug.Assert(isCollType && argCollType != null, "DbUnaryExpression.CheckCollectionArgument allowed non-collection argument"); #endif TypeUsage elementType = null; if( !TypeHelpers.TryGetCollectionElementType(arg.ResultType, out elementType) || !TypeSemantics.IsValidPolymorphicCast(elementType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } // // The type of this DbExpression is a new collection type based on the requested element type. // this.ResultType = CommandTreeTypeHelper.CreateCollectionResultType(type); // // Assign the requested element type to the OfType property. // _ofType = type; } /// /// Gets the metadata of the type of elements that should be retrieved from the set argument. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type conversion of a single argument to the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbTreatExpression : DbUnaryExpression { internal DbTreatExpression(DbCommandTree cmdTree, TypeUsage type, DbExpression arg) : base(cmdTree, DbExpressionKind.Treat) { // // Verify the type to treat as. Treat-As (NullType) is not allowed. // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Verify that the Treat operation is allowed // if (!TypeSemantics.IsValidPolymorphicCast(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } // // Set the Argument constraints and initialize the Argument value // this.ArgumentLink.InitializeValue(arg); this.ArgumentLink.SetExpectedType(arg.ResultType); this.ResultType = type; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a string comparison against the specified pattern with an optional escape string /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLikeExpression : DbExpression { private ExpressionLink _argument; private ExpressionLink _pattern; private ExpressionLink _escape; internal DbLikeExpression(DbCommandTree cmdTree, DbExpression input, DbExpression pattern, DbExpression escape) : base(cmdTree, DbExpressionKind.Like) { _argument = new ExpressionLink("Argument", cmdTree, PrimitiveTypeKind.String, input); _pattern = new ExpressionLink("Pattern", cmdTree, PrimitiveTypeKind.String, pattern); if (null == escape) { // The default escape sequence is null - not the empty string escape = cmdTree.CreateNullExpression(pattern.ResultType); } _escape = new ExpressionLink("Escape", cmdTree, PrimitiveTypeKind.String, escape); this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// Gets or sets an expression that specifies the string to compare against the given pattern /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Argument { get { return _argument.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _argument.Expression = value; } } ////// Gets or sets an expression that specifies the pattern against which the given string should be compared /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Pattern { get { return _pattern.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _pattern.Expression = value; } } ////// Gets or sets an expression that provides an optional escape string to use for the comparison /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Escape { get { return _escape.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _escape.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a reference to the specified Entity as a Ref. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbEntityRefExpression : DbUnaryExpression { internal DbEntityRefExpression(DbCommandTree cmdTree, DbExpression entity) : base(cmdTree, DbExpressionKind.EntityRef, entity) { EntityType entityType = null; if (!TypeHelpers.TryGetEdmType(this.Argument.ResultType, out entityType) || null == entityType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetEntityRef_EntityRequired, "Argument"); } this.ResultType = CommandTreeTypeHelper.CreateReferenceResultType(entityType); } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of the key value of the specified Reference as a row. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRefKeyExpression : DbUnaryExpression { internal DbRefKeyExpression(DbCommandTree cmdTree, DbExpression reference) : base(cmdTree, DbExpressionKind.RefKey, reference) { RefType refType = null; if (!TypeHelpers.TryGetEdmType(this.Argument.ResultType, out refType) || null == refType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetRefKey_RefRequired, "Argument"); } if (null == refType.ElementType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetRefKey_InvalidRef, "Argument"); } TypeUsage result = CommandTreeTypeHelper.CreateResultType(TypeHelpers.CreateKeyRowType(refType.ElementType, cmdTree.MetadataWorkspace)); this.ResultType = result; } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { #region Boolean Operators ////// Represents the logical And of two Boolean arguments. /// ///DbAndExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbAndExpression : DbBinaryExpression { internal DbAndExpression(DbCommandTree commandTree, DbExpression left, DbExpression right) : base(commandTree, DbExpressionKind.And) { EntityUtil.CheckArgumentNull(left, "Left"); EntityUtil.CheckArgumentNull(right, "Right"); TypeUsage resultType = TypeHelpers.GetCommonTypeUsage(left.ResultType, right.ResultType); if (null == resultType || !TypeSemantics.IsPrimitiveType(resultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_And_BooleanArgumentsRequired); } // // Apply constraints to the left and right arguments and set initial values // this.LeftLink.SetExpectedType(left.ResultType); this.LeftLink.InitializeValue(left); this.RightLink.SetExpectedType(right.ResultType); this.RightLink.InitializeValue(right); // // Result Type is always Boolean // this.ResultType = resultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Or of two Boolean arguments. /// ///DbOrExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOrExpression : DbBinaryExpression { internal DbOrExpression(DbCommandTree commandTree, DbExpression left, DbExpression right) : base(commandTree, DbExpressionKind.Or) { EntityUtil.CheckArgumentNull(left, "Left"); EntityUtil.CheckArgumentNull(right, "Right"); TypeUsage resultType = TypeHelpers.GetCommonTypeUsage(left.ResultType, right.ResultType); if (null == resultType || !TypeSemantics.IsPrimitiveType(resultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Or_BooleanArgumentsRequired); } // // Apply constraints to the left and right arguments and set initial values // this.LeftLink.SetExpectedType(left.ResultType); this.LeftLink.InitializeValue(left); this.RightLink.SetExpectedType(right.ResultType); this.RightLink.InitializeValue(right); // // Result Type is always Boolean // this.ResultType = resultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Not of a single Boolean argument. /// ///DbNotExpression requires that its argument has a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNotExpression : DbUnaryExpression { internal DbNotExpression(DbCommandTree commandTree, DbExpression arg) : base(commandTree, DbExpressionKind.Not) { EntityUtil.CheckArgumentNull(arg, "Argument"); // // Argument to Not must have Boolean result type // if (!TypeSemantics.IsPrimitiveType(arg.ResultType, PrimitiveTypeKind.Boolean)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Not_BooleanArgumentRequired); } this.ArgumentLink.SetExpectedType(arg.ResultType); this.ArgumentLink.InitializeValue(arg); this.ResultType = arg.ResultType; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #endregion /// /// Represents an arithmetic operation (addition, subtraction, multiplication, division, modulo or negation) applied to two numeric arguments. /// ///DbArithmeticExpression requires that its arguments have a common numeric result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbArithmeticExpression : DbExpression { private readonly ExpressionList _args; internal DbArithmeticExpression(DbCommandTree commandTree, DbExpressionKind kind, IListargs) : base(commandTree, kind) { EntityUtil.CheckArgumentNull(args, "args"); Debug.Assert( DbExpressionKind.Divide == kind || DbExpressionKind.Minus == kind || DbExpressionKind.Modulo == kind || DbExpressionKind.Multiply == kind || DbExpressionKind.Plus == kind || DbExpressionKind.UnaryMinus == kind, "Invalid DbExpressionKind used in DbArithmeticExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); Debug.Assert( (DbExpressionKind.UnaryMinus == kind && 1 == args.Count) || 2 == args.Count, "Incorrect number of arguments specified to DbArithmeticExpression" ); // // Construct a new ExpressionList to contain the arguments // ExpressionList argList = new ExpressionList("Arguments", commandTree, args.Count); // // Set the current elements without constraining the expected element type // argList.SetElements(args); // // Ensure that a numeric common type exists for the arguments // TypeUsage commonType = argList.GetCommonElementType(); if (TypeSemantics.IsNullOrNullType(commonType) || !TypeSemantics.IsNumericType(commonType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Arithmetic_NumericCommonType); } // // Constrain the type of elements in the list to the common numeric type // argList.SetExpectedElementType(commonType); this._args = argList; this.ResultType = commonType; } /// /// Gets the list of expressions that define the current arguments. /// ////// The public IListArguments
property returns a fixed-size list ofelements. /// requires that all elements of it's Arguments
list /// have a common numeric result type. ///Arguments { get { return _args; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a Case When...Then...Else logical operation. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCaseExpression : DbExpression { private ExpressionList _when; private ExpressionList _then; private ExpressionLink _else; internal DbCaseExpression(DbCommandTree cmdTree, IListwhens, IList thens, DbExpression elseExpr) : base(cmdTree, DbExpressionKind.Case) { // // Whens/Thens cannot be null // (nor can Else, but this is enforced when the // ExpressionLink for the Else property is created, below). // EntityUtil.CheckArgumentNull(whens, "whens"); EntityUtil.CheckArgumentNull(thens, "thens"); // // The number of 'When's must equal the number of 'Then's. // if (whens.Count != thens.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_WhensMustEqualThens); } // // There must be at least one clause in the case expression. // if (0 == whens.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_AtLeastOneClause); } // // All 'When's must produce a Boolean result, and a common (non-null) result type must exist // TypeUsage resultType = null; for(int idx = 0; idx < whens.Count; idx++) { if (null == thens[idx]) { throw EntityUtil.ArgumentNull(CommandTreeUtils.FormatIndex("Thens", idx)); } if (null == resultType) { resultType = thens[idx].ResultType; } else { resultType = TypeHelpers.GetCommonTypeUsage(thens[idx].ResultType, resultType); if (null == resultType) { break; } } } if (resultType != null && elseExpr != null) { resultType = TypeHelpers.GetCommonTypeUsage(elseExpr.ResultType, resultType); } if (TypeSemantics.IsNullOrNullType(resultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Case_InvalidResultType); } // // Initialize the When clauses. Elements must be promotable to Boolean. // NullType is specifically not allowed as the Type of a When element. // _when = new ExpressionList("When", cmdTree, PrimitiveTypeKind.Boolean, whens); // // Initialize the Then clauses. Elements must be promotable to the common result type. // _then = new ExpressionList("Then", cmdTree, resultType, thens); // // Initialize the Else clause. Values must be promotable to the common result type. // _else = new ExpressionLink("Else", cmdTree, resultType, elseExpr); // // The result type of DbCaseExpression is the common result type // this.ResultType = resultType; } /// /// Gets the When clauses of this DbCaseExpression. /// public IListWhen { get { return _when; } } /// /// Gets the Then clauses of this DbCaseExpression. /// public IListThen { get { return _then; } } /// /// Gets or sets the Else clause of this DbCaseExpression. /// ///The expression is null ////// The expression is not associated with the DbCaseExpression's command tree, /// or its result type is not equal or promotable to the result type of the DbCaseExpression /// public DbExpression Else { get { return _else.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _else.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a cast operation applied to a polymorphic argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCastExpression : DbUnaryExpression { internal DbCastExpression(DbCommandTree cmdTree, TypeUsage type, DbExpression arg) : base(cmdTree, DbExpressionKind.Cast) { // // Verify the type to cast to. Casting to NullType is not allowed // cmdTree.TypeHelper.CheckType(type); // // Verify that the cast is allowed // if (!TypeSemantics.IsCastAllowed(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Cast_InvalidCast(TypeHelpers.GetFullName(arg.ResultType), TypeHelpers.GetFullName(type))); } // // Set the Argument constraints and initialize the argument value // this.ArgumentLink.InitializeValue(arg); this.ArgumentLink.SetExpectedType(arg.ResultType); this.ResultType = type; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a comparison operation (equality, greater than, greather than or equal, less than, less than or equal, inequality) applied to two arguments. /// ////// DbComparisonExpression requires that its arguments have a common result type /// that is equality comparable (for [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbComparisonExpression : DbBinaryExpression { internal DbComparisonExpression(DbCommandTree commandTree, DbExpressionKind kind, DbExpression left, DbExpression right) : base(commandTree, kind) { Debug.Assert( DbExpressionKind.Equals == kind || DbExpressionKind.LessThan == kind || DbExpressionKind.LessThanOrEquals == kind || DbExpressionKind.GreaterThan == kind || DbExpressionKind.GreaterThanOrEquals == kind || DbExpressionKind.NotEquals == kind, "Invalid DbExpressionKind used in DbComparisonExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); // // Initialize the Left and Right arguments to perform Null value and NullType checking // this.LeftLink.InitializeValue(left); this.RightLink.InitializeValue(right); // // Ensure that the types of the arguments are comparable // this.CheckComparison(left, right); // // Register for notifications that the left or right argument is changing. // The arguments can be freely substituted provided that when a value is changed, // the result type of the new expression value and the result type of the unchanged argument are comparable. // this.LeftLink.ValueChanging += new ExpressionLinkConstraint(this.ArgumentChanging); this.RightLink.ValueChanging += new ExpressionLinkConstraint(this.ArgumentChanging); // // Result Type is always Boolean // this.ResultType = commandTree.TypeHelper.CreateBooleanResultType(); } ///.Equals and .NotEquals), /// order comparable (for .GreaterThan and .LessThan), /// or both (for .GreaterThanOrEquals and .LessThanOrEquals). /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } private void ArgumentChanging(ExpressionLink link, DbExpression newValue) { // // If the Left expression is changing then the Right expression should be compared // to the new value, and vice-versa. // if (this.LeftLink == link) { this.CheckComparison(newValue, this.Right); } else { this.CheckComparison(this.Left, newValue); } } private void CheckComparison(DbExpression left, DbExpression right) { // // A comparison of the specified kind must exist between the left and right arguments // bool equality = true; bool order = true; if (DbExpressionKind.GreaterThanOrEquals == this.ExpressionKind || DbExpressionKind.LessThanOrEquals == this.ExpressionKind) { equality = TypeSemantics.IsEqualComparableTo(left.ResultType, right.ResultType); order = TypeSemantics.IsOrderComparableTo(left.ResultType, right.ResultType); } else if (DbExpressionKind.Equals == this.ExpressionKind || DbExpressionKind.NotEquals == this.ExpressionKind) { equality = TypeSemantics.IsEqualComparableTo(left.ResultType, right.ResultType); } else { order = TypeSemantics.IsOrderComparableTo(left.ResultType, right.ResultType); } if(!equality || !order) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Comparison_ComparableRequired); } } } /// /// Represents empty set determination applied to a single set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsEmptyExpression : DbUnaryExpression { internal DbIsEmptyExpression(DbCommandTree cmdTree, DbExpression arg) : base(cmdTree, DbExpressionKind.IsEmpty, arg) { // // Ensure that the Argument is of a collection type // this.CheckCollectionArgument(); // // Result Type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents null determination applied to a single argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsNullExpression : DbUnaryExpression { internal DbIsNullExpression(DbCommandTree cmdTree, DbExpression arg, bool isRowTypeArgumentAllowed) : base(cmdTree, DbExpressionKind.IsNull) { // // The argument cannot be of a collection type // if (TypeSemantics.IsCollectionType(arg.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_IsNull_CollectionNotAllowed); } // // ensure argument type is valid for this operation // if (!TypeHelpers.IsValidIsNullOpType(arg.ResultType) && (!isRowTypeArgumentAllowed || !TypeSemantics.IsRowType(arg.ResultType))) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_IsNull_InvalidType); } // // Set the Argument constraints and initialize the Argument value // ArgumentLink.SetExpectedType is not called - any expression can be substituted freely for the argument expression // this.ArgumentLink.InitializeValue(arg); // // Result Type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type comparison of a single argument against the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsOfExpression : DbUnaryExpression { private TypeUsage _ofType; internal DbIsOfExpression(DbCommandTree cmdTree, DbExpressionKind isOfKind, TypeUsage type, DbExpression arg) : base(cmdTree, isOfKind) { Debug.Assert(DbExpressionKind.IsOf == this.ExpressionKind || DbExpressionKind.IsOfOnly == this.ExpressionKind, string.Format(CultureInfo.InvariantCulture, "Invalid DbExpressionKind used in DbIsOfExpression: {0}", Enum.GetName(typeof(DbExpressionKind), this.ExpressionKind))); // // Ensure the ofType is non-null, associated with the correct metadata workspace/dataspace, // is not NullType, and is polymorphic // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Verify that the IsOf operation is allowed // if (!TypeSemantics.IsValidPolymorphicCast(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } _ofType = type; // // Set the Argument constraints and initialize the Argument value // this.ArgumentLink.SetExpectedType(arg.ResultType); this.ArgumentLink.InitializeValue(arg); // // Return type is always Boolean // this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// Gets the type metadata that the type metadata of the argument should be compared to. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of elements of the specified type from the given set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOfTypeExpression : DbUnaryExpression { private TypeUsage _ofType; internal DbOfTypeExpression(DbCommandTree cmdTree, DbExpressionKind ofTypeKind, TypeUsage type, DbExpression arg) : base(cmdTree, ofTypeKind, arg) { Debug.Assert(DbExpressionKind.OfType == ofTypeKind || DbExpressionKind.OfTypeOnly == ofTypeKind, "ExpressionKind for DbOfTypeExpression must be OfType or OfTypeOnly"); // // Ensure that the type is non-null and valid - from the same metadata collection and dataspace and the command tree. // The type is also not allowed to be NullType. // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Ensure that the argument is actually of a collection type. // this.CheckCollectionArgument(); // // Verify that the OfType operation is allowed // #if DEBUG CollectionType argCollType = null; bool isCollType = TypeHelpers.TryGetEdmType(arg.ResultType, out argCollType); Debug.Assert(isCollType && argCollType != null, "DbUnaryExpression.CheckCollectionArgument allowed non-collection argument"); #endif TypeUsage elementType = null; if( !TypeHelpers.TryGetCollectionElementType(arg.ResultType, out elementType) || !TypeSemantics.IsValidPolymorphicCast(elementType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } // // The type of this DbExpression is a new collection type based on the requested element type. // this.ResultType = CommandTreeTypeHelper.CreateCollectionResultType(type); // // Assign the requested element type to the OfType property. // _ofType = type; } /// /// Gets the metadata of the type of elements that should be retrieved from the set argument. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type conversion of a single argument to the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbTreatExpression : DbUnaryExpression { internal DbTreatExpression(DbCommandTree cmdTree, TypeUsage type, DbExpression arg) : base(cmdTree, DbExpressionKind.Treat) { // // Verify the type to treat as. Treat-As (NullType) is not allowed. // cmdTree.TypeHelper.CheckPolymorphicType(type); // // Verify that the Treat operation is allowed // if (!TypeSemantics.IsValidPolymorphicCast(arg.ResultType, type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicArgRequired(this.GetType().Name)); } // // Set the Argument constraints and initialize the Argument value // this.ArgumentLink.InitializeValue(arg); this.ArgumentLink.SetExpectedType(arg.ResultType); this.ResultType = type; } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a string comparison against the specified pattern with an optional escape string /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLikeExpression : DbExpression { private ExpressionLink _argument; private ExpressionLink _pattern; private ExpressionLink _escape; internal DbLikeExpression(DbCommandTree cmdTree, DbExpression input, DbExpression pattern, DbExpression escape) : base(cmdTree, DbExpressionKind.Like) { _argument = new ExpressionLink("Argument", cmdTree, PrimitiveTypeKind.String, input); _pattern = new ExpressionLink("Pattern", cmdTree, PrimitiveTypeKind.String, pattern); if (null == escape) { // The default escape sequence is null - not the empty string escape = cmdTree.CreateNullExpression(pattern.ResultType); } _escape = new ExpressionLink("Escape", cmdTree, PrimitiveTypeKind.String, escape); this.ResultType = cmdTree.TypeHelper.CreateBooleanResultType(); } ////// Gets or sets an expression that specifies the string to compare against the given pattern /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Argument { get { return _argument.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _argument.Expression = value; } } ////// Gets or sets an expression that specifies the pattern against which the given string should be compared /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Pattern { get { return _pattern.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _pattern.Expression = value; } } ////// Gets or sets an expression that provides an optional escape string to use for the comparison /// ///The expression is null ////// The expression is not associated with the DbLikeExpression's command tree, /// or its result type is not a string type. /// public DbExpression Escape { get { return _escape.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _escape.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a reference to the specified Entity as a Ref. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbEntityRefExpression : DbUnaryExpression { internal DbEntityRefExpression(DbCommandTree cmdTree, DbExpression entity) : base(cmdTree, DbExpressionKind.EntityRef, entity) { EntityType entityType = null; if (!TypeHelpers.TryGetEdmType(this.Argument.ResultType, out entityType) || null == entityType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetEntityRef_EntityRequired, "Argument"); } this.ResultType = CommandTreeTypeHelper.CreateReferenceResultType(entityType); } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of the key value of the specified Reference as a row. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRefKeyExpression : DbUnaryExpression { internal DbRefKeyExpression(DbCommandTree cmdTree, DbExpression reference) : base(cmdTree, DbExpressionKind.RefKey, reference) { RefType refType = null; if (!TypeHelpers.TryGetEdmType(this.Argument.ResultType, out refType) || null == refType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetRefKey_RefRequired, "Argument"); } if (null == refType.ElementType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GetRefKey_InvalidRef, "Argument"); } TypeUsage result = CommandTreeTypeHelper.CreateResultType(TypeHelpers.CreateKeyRowType(refType.ElementType, cmdTree.MetadataWorkspace)); this.ResultType = result; } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
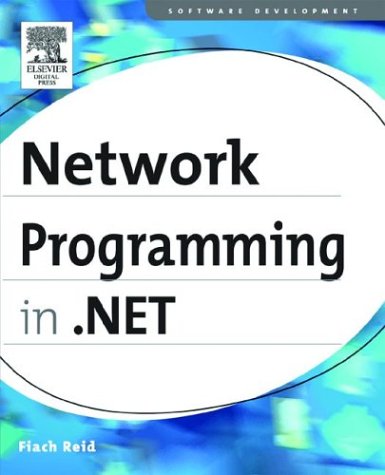
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamedElement.cs
- ComplexType.cs
- AddInIpcChannel.cs
- Camera.cs
- DuplicateWaitObjectException.cs
- BuildProvider.cs
- FormViewRow.cs
- shaper.cs
- MultiSelector.cs
- MasterPageBuildProvider.cs
- FloatUtil.cs
- FieldBuilder.cs
- ProfilePropertySettings.cs
- NavigationPropertySingletonExpression.cs
- TableAdapterManagerMethodGenerator.cs
- StrongNameMembershipCondition.cs
- XmlObjectSerializerWriteContextComplex.cs
- SerialPinChanges.cs
- XmlObjectSerializerReadContextComplexJson.cs
- DependencyObjectProvider.cs
- SocketPermission.cs
- XmlBinaryReaderSession.cs
- FixedPageProcessor.cs
- XPathDocumentIterator.cs
- CodeExpressionStatement.cs
- Geometry3D.cs
- EditBehavior.cs
- MembershipPasswordException.cs
- DocumentPaginator.cs
- VarRemapper.cs
- TextElement.cs
- GridViewCommandEventArgs.cs
- SiteMap.cs
- PrinterResolution.cs
- MemoryFailPoint.cs
- ReadOnlyCollection.cs
- BufferModeSettings.cs
- EncodingNLS.cs
- GlobalItem.cs
- XsltFunctions.cs
- ExternalFile.cs
- DynamicDocumentPaginator.cs
- XmlArrayAttribute.cs
- ADMembershipUser.cs
- Lookup.cs
- Calendar.cs
- PropertyValueUIItem.cs
- ArrangedElementCollection.cs
- AppSettingsExpressionBuilder.cs
- WeakReferenceList.cs
- MonitoringDescriptionAttribute.cs
- AncestorChangedEventArgs.cs
- ButtonFlatAdapter.cs
- InheritanceUI.cs
- LocatorPart.cs
- TraceHandler.cs
- DetailsViewRowCollection.cs
- BrowserDefinition.cs
- SQLByte.cs
- ApplicationManager.cs
- ClientUtils.cs
- AddingNewEventArgs.cs
- TextBox.cs
- CodeVariableReferenceExpression.cs
- Component.cs
- FormatConvertedBitmap.cs
- SystemBrushes.cs
- WeakReferenceEnumerator.cs
- DataGridViewColumnCollection.cs
- PeerReferralPolicy.cs
- FormatConvertedBitmap.cs
- WizardSideBarListControlItem.cs
- CustomTypeDescriptor.cs
- Splitter.cs
- PageClientProxyGenerator.cs
- BindableTemplateBuilder.cs
- XmlTextReaderImpl.cs
- IteratorDescriptor.cs
- DrawListViewColumnHeaderEventArgs.cs
- CacheHelper.cs
- MaskedTextProvider.cs
- DataShape.cs
- XPathNode.cs
- CurrentChangingEventArgs.cs
- HtmlShim.cs
- MessagePropertyDescriptionCollection.cs
- AlternateView.cs
- ReaderWriterLock.cs
- CompilerResults.cs
- CodeExporter.cs
- COM2ExtendedTypeConverter.cs
- PanelDesigner.cs
- InvalidEnumArgumentException.cs
- SessionSwitchEventArgs.cs
- DependencyPropertyChangedEventArgs.cs
- Path.cs
- OdbcConnectionFactory.cs
- SqlDataSourceView.cs
- PriorityItem.cs
- PropertyGridDesigner.cs