Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Xml / XmlBinaryReaderSession.cs / 1305376 / XmlBinaryReaderSession.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- using StringHandle = System.Int64; namespace System.Xml { using System.Xml; using System.Collections.Generic; using System.Runtime.Serialization; public class XmlBinaryReaderSession : IXmlDictionary { const int MaxArrayEntries = 2048; XmlDictionaryString[] strings; DictionarystringDict; public XmlBinaryReaderSession() { } public XmlDictionaryString Add(int id, string value) { if (id < 0) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException(SR.GetString(SR.XmlInvalidID))); if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); XmlDictionaryString xmlString; if (TryLookup(id, out xmlString)) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.XmlIDDefined))); xmlString = new XmlDictionaryString(this, value, id); if (id >= MaxArrayEntries) { if (stringDict == null) this.stringDict = new Dictionary (); this.stringDict.Add(id, xmlString); } else { if (strings == null) { strings = new XmlDictionaryString[Math.Max(id + 1, 16)]; } else if (id >= strings.Length) { XmlDictionaryString[] newStrings = new XmlDictionaryString[Math.Min(Math.Max(id + 1, strings.Length * 2), MaxArrayEntries)]; Array.Copy(strings, newStrings, strings.Length); strings = newStrings; } strings[id] = xmlString; } return xmlString; } public bool TryLookup(int key, out XmlDictionaryString result) { if (strings != null && key >= 0 && key < strings.Length) { result = strings[key]; return result != null; } else if (key >= MaxArrayEntries) { if (this.stringDict != null) return this.stringDict.TryGetValue(key, out result); } result = null; return false; } public bool TryLookup(string value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (strings != null) { for (int i = 0; i < strings.Length; i++) { XmlDictionaryString s = strings[i]; if (s != null && s.Value == value) { result = s; return true; } } } if (this.stringDict != null) { foreach (XmlDictionaryString s in this.stringDict.Values) { if (s.Value == value) { result = s; return true; } } } result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (value.Dictionary != this) { result = null; return false; } result = value; return true; } public void Clear() { if (strings != null) Array.Clear(strings, 0, strings.Length); if (this.stringDict != null) this.stringDict.Clear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- using StringHandle = System.Int64; namespace System.Xml { using System.Xml; using System.Collections.Generic; using System.Runtime.Serialization; public class XmlBinaryReaderSession : IXmlDictionary { const int MaxArrayEntries = 2048; XmlDictionaryString[] strings; Dictionary stringDict; public XmlBinaryReaderSession() { } public XmlDictionaryString Add(int id, string value) { if (id < 0) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException(SR.GetString(SR.XmlInvalidID))); if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); XmlDictionaryString xmlString; if (TryLookup(id, out xmlString)) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.XmlIDDefined))); xmlString = new XmlDictionaryString(this, value, id); if (id >= MaxArrayEntries) { if (stringDict == null) this.stringDict = new Dictionary (); this.stringDict.Add(id, xmlString); } else { if (strings == null) { strings = new XmlDictionaryString[Math.Max(id + 1, 16)]; } else if (id >= strings.Length) { XmlDictionaryString[] newStrings = new XmlDictionaryString[Math.Min(Math.Max(id + 1, strings.Length * 2), MaxArrayEntries)]; Array.Copy(strings, newStrings, strings.Length); strings = newStrings; } strings[id] = xmlString; } return xmlString; } public bool TryLookup(int key, out XmlDictionaryString result) { if (strings != null && key >= 0 && key < strings.Length) { result = strings[key]; return result != null; } else if (key >= MaxArrayEntries) { if (this.stringDict != null) return this.stringDict.TryGetValue(key, out result); } result = null; return false; } public bool TryLookup(string value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (strings != null) { for (int i = 0; i < strings.Length; i++) { XmlDictionaryString s = strings[i]; if (s != null && s.Value == value) { result = s; return true; } } } if (this.stringDict != null) { foreach (XmlDictionaryString s in this.stringDict.Values) { if (s.Value == value) { result = s; return true; } } } result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (value.Dictionary != this) { result = null; return false; } result = value; return true; } public void Clear() { if (strings != null) Array.Clear(strings, 0, strings.Length); if (this.stringDict != null) this.stringDict.Clear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
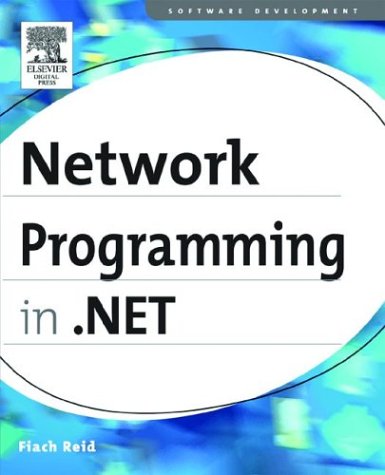
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateBamlRecordReader.cs
- UpDownEvent.cs
- ClientType.cs
- HandleExceptionArgs.cs
- ModelUIElement3D.cs
- Path.cs
- httpapplicationstate.cs
- ProtocolReflector.cs
- QuadraticBezierSegment.cs
- ObjectDataProvider.cs
- SeekStoryboard.cs
- SerializerWriterEventHandlers.cs
- FrugalMap.cs
- StylusLogic.cs
- ChannelFactoryRefCache.cs
- TypeSystem.cs
- RootBrowserWindow.cs
- ArrayHelper.cs
- QueryGenerator.cs
- ContainerControl.cs
- GcHandle.cs
- DrawingState.cs
- ThumbAutomationPeer.cs
- StartUpEventArgs.cs
- GenericPrincipal.cs
- ExtensionWindow.cs
- WindowAutomationPeer.cs
- DataGrid.cs
- FontUnit.cs
- TemplateField.cs
- LabelInfo.cs
- DataReceivedEventArgs.cs
- MenuItemCollectionEditorDialog.cs
- AnnotationStore.cs
- TableHeaderCell.cs
- WebUtil.cs
- MenuAdapter.cs
- ItemContainerGenerator.cs
- CodeSnippetExpression.cs
- GridViewSortEventArgs.cs
- DataView.cs
- Lease.cs
- CustomAttributeFormatException.cs
- CodeMemberMethod.cs
- RowsCopiedEventArgs.cs
- WSSecureConversation.cs
- HelpInfo.cs
- InternalBufferOverflowException.cs
- keycontainerpermission.cs
- Condition.cs
- WebProxyScriptElement.cs
- WebPartDisplayModeCollection.cs
- CodeCatchClause.cs
- LocalBuilder.cs
- ModuleElement.cs
- DelayedRegex.cs
- CorrelationInitializer.cs
- DbMetaDataColumnNames.cs
- BitmapEffectOutputConnector.cs
- RMEnrollmentPage2.cs
- TextRangeBase.cs
- OdbcEnvironmentHandle.cs
- ActiveXHost.cs
- ClonableStack.cs
- MdbDataFileEditor.cs
- RequestCache.cs
- DelegateHelpers.Generated.cs
- MsmqInputChannelListenerBase.cs
- TrueReadOnlyCollection.cs
- ChangeBlockUndoRecord.cs
- ListViewCancelEventArgs.cs
- ELinqQueryState.cs
- FontDriver.cs
- MgmtConfigurationRecord.cs
- DataGridColumnFloatingHeader.cs
- DocumentViewerAutomationPeer.cs
- DtdParser.cs
- ContentFilePart.cs
- DataGridViewCellMouseEventArgs.cs
- RouteItem.cs
- XmlDomTextWriter.cs
- StrokeCollection2.cs
- SecuritySessionClientSettings.cs
- GroupedContextMenuStrip.cs
- FactoryId.cs
- TypeHelpers.cs
- LeaseManager.cs
- RefreshInfo.cs
- GlyphElement.cs
- Trigger.cs
- ApplicationSettingsBase.cs
- ExpandCollapseIsCheckedConverter.cs
- TableProvider.cs
- StringDictionary.cs
- ToolboxService.cs
- ClientRoleProvider.cs
- DnsPermission.cs
- OleDbDataAdapter.cs
- ObjectDataSourceView.cs
- ItemCollection.cs