Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / WebUtil.cs / 1 / WebUtil.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif ///web utility functions internal static partial class WebUtil { ///copy from one stream to another /// input stream /// output stream /// reusable buffer ///count of copied bytes internal static long CopyStream(Stream input, Stream output, ref byte[] refBuffer) { Debug.Assert(null != input, "null input stream"); Debug.Assert(null != output, "null output stream"); long total = 0; byte[] buffer = refBuffer; if (null == buffer) { refBuffer = buffer = new byte[1000]; } int count = 0; while (input.CanRead && (0 < (count = input.Read(buffer, 0, buffer.Length)))) { output.Write(buffer, 0, count); total += count; } return total; } ///get response object from possible WebException /// exception to probe /// http web respose object from exception internal static void GetHttpWebResponse(InvalidOperationException exception, ref HttpWebResponse response) { if (null == response) { WebException webexception = (exception as WebException); if (null != webexception) { response = (HttpWebResponse)webexception.Response; } } } ///is this a success status code /// status code ///true if status is between 200-299 internal static bool SuccessStatusCode(HttpStatusCode status) { return (200 <= (int)status && (int)status < 300); } ////// turn the response object headers into a dictionary /// /// response ///dictionary internal static DictionaryWrapResponseHeaders(HttpWebResponse response) { Dictionary headers = new Dictionary (); if (null != response) { foreach (string name in response.Headers.AllKeys) { headers.Add(name, response.Headers[name]); } } return headers; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif ///web utility functions internal static partial class WebUtil { ///copy from one stream to another /// input stream /// output stream /// reusable buffer ///count of copied bytes internal static long CopyStream(Stream input, Stream output, ref byte[] refBuffer) { Debug.Assert(null != input, "null input stream"); Debug.Assert(null != output, "null output stream"); long total = 0; byte[] buffer = refBuffer; if (null == buffer) { refBuffer = buffer = new byte[1000]; } int count = 0; while (input.CanRead && (0 < (count = input.Read(buffer, 0, buffer.Length)))) { output.Write(buffer, 0, count); total += count; } return total; } ///get response object from possible WebException /// exception to probe /// http web respose object from exception internal static void GetHttpWebResponse(InvalidOperationException exception, ref HttpWebResponse response) { if (null == response) { WebException webexception = (exception as WebException); if (null != webexception) { response = (HttpWebResponse)webexception.Response; } } } ///is this a success status code /// status code ///true if status is between 200-299 internal static bool SuccessStatusCode(HttpStatusCode status) { return (200 <= (int)status && (int)status < 300); } ////// turn the response object headers into a dictionary /// /// response ///dictionary internal static DictionaryWrapResponseHeaders(HttpWebResponse response) { Dictionary headers = new Dictionary (); if (null != response) { foreach (string name in response.Headers.AllKeys) { headers.Add(name, response.Headers[name]); } } return headers; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
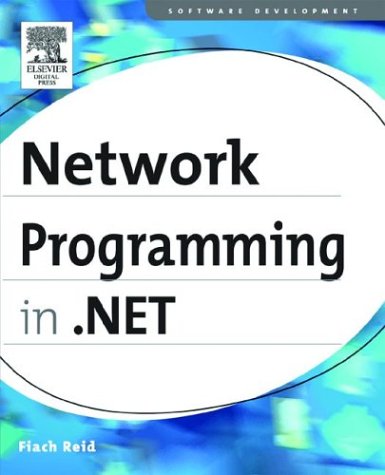
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextViewBase.cs
- Debug.cs
- _Rfc2616CacheValidators.cs
- AQNBuilder.cs
- QilTypeChecker.cs
- EpmContentSerializerBase.cs
- CancellationTokenRegistration.cs
- PixelShader.cs
- ButtonBaseAutomationPeer.cs
- StringTraceRecord.cs
- CompensationHandlingFilter.cs
- X509Certificate2Collection.cs
- WsatServiceCertificate.cs
- ComponentResourceKey.cs
- CapabilitiesState.cs
- RadioButtonPopupAdapter.cs
- EmitterCache.cs
- AuthStoreRoleProvider.cs
- GeneralTransform3DTo2D.cs
- XmlQuerySequence.cs
- FixedSOMContainer.cs
- PopOutPanel.cs
- SrgsNameValueTag.cs
- BamlResourceContent.cs
- SchemaTypeEmitter.cs
- RunInstallerAttribute.cs
- SoapExtensionImporter.cs
- SHA512.cs
- ImageResources.Designer.cs
- WindowsListViewSubItem.cs
- InputLanguageManager.cs
- WebPageTraceListener.cs
- TrackingMemoryStreamFactory.cs
- TimeSpanValidatorAttribute.cs
- PackagingUtilities.cs
- QuaternionAnimationBase.cs
- ExtendedTransformFactory.cs
- SafeNativeMethods.cs
- ProfessionalColors.cs
- RenameRuleObjectDialog.Designer.cs
- QueryCacheManager.cs
- DataGridCellInfo.cs
- WebControlAdapter.cs
- MSAAWinEventWrap.cs
- PerformanceCounterPermission.cs
- ApplicationContext.cs
- SiteMapNodeItem.cs
- httpstaticobjectscollection.cs
- IntegerValidator.cs
- FieldToken.cs
- PropertyTabChangedEvent.cs
- DynamicAttribute.cs
- DataGridComboBoxColumn.cs
- OdbcConnectionPoolProviderInfo.cs
- PropertyRef.cs
- GenericTypeParameterBuilder.cs
- selecteditemcollection.cs
- TextEndOfSegment.cs
- TimelineCollection.cs
- TabControl.cs
- AspNetSynchronizationContext.cs
- BamlRecordHelper.cs
- MasterPageParser.cs
- ToolStripItemClickedEventArgs.cs
- StreamProxy.cs
- DbProviderFactories.cs
- SettingsBindableAttribute.cs
- ValueUtilsSmi.cs
- SimpleApplicationHost.cs
- SQlBooleanStorage.cs
- CompensationParticipant.cs
- ConnectivityStatus.cs
- TokenizerHelper.cs
- HttpInputStream.cs
- BuildProvider.cs
- Line.cs
- DependencyObjectType.cs
- TypeInitializationException.cs
- Site.cs
- DataGridViewButtonCell.cs
- BidirectionalDictionary.cs
- ElementNotEnabledException.cs
- ToolStripSeparator.cs
- OutputCacheProfileCollection.cs
- SqlClientFactory.cs
- DoubleStorage.cs
- SQLDouble.cs
- CodeAccessSecurityEngine.cs
- AvTraceFormat.cs
- Int32AnimationUsingKeyFrames.cs
- BezierSegment.cs
- SiteMapProvider.cs
- RawStylusSystemGestureInputReport.cs
- DbTransaction.cs
- NetCodeGroup.cs
- TaskbarItemInfo.cs
- MorphHelper.cs
- TextRange.cs
- RadioButtonPopupAdapter.cs
- Metafile.cs