Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / XPathNodeIterator.cs / 1305376 / XPathNodeIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; using System.Text; namespace System.Xml.XPath { [DebuggerDisplay("Position={CurrentPosition}, Current={debuggerDisplayProxy}")] public abstract class XPathNodeIterator : ICloneable, IEnumerable { internal int count = -1; object ICloneable.Clone() { return this.Clone(); } public abstract XPathNodeIterator Clone(); public abstract bool MoveNext(); public abstract XPathNavigator Current { get; } public abstract int CurrentPosition { get; } public virtual int Count { get { if (count == -1) { XPathNodeIterator clone = this.Clone(); while(clone.MoveNext()) ; count = clone.CurrentPosition; } return count; } } public virtual IEnumerator GetEnumerator() { return new Enumerator(this); } private object debuggerDisplayProxy { get { return Current == null ? null : (object)new XPathNavigator.DebuggerDisplayProxy(Current); } } ////// Implementation of a resetable enumerator that is linked to the XPathNodeIterator used to create it. /// private class Enumerator : IEnumerator { private XPathNodeIterator original; // Keep original XPathNodeIterator in case Reset() is called private XPathNodeIterator current; private bool iterationStarted; public Enumerator(XPathNodeIterator original) { this.original = original.Clone(); } public virtual object Current { get { // 1. Do not reuse the XPathNavigator, as we do in XPathNodeIterator // 2. Throw exception if current position is before first node or after the last node if (this.iterationStarted) { // Current is null if iterator is positioned after the last node if (this.current == null) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current.Current.Clone(); } // User must call MoveNext before accessing Current property throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); } } public virtual bool MoveNext() { // Delegate to XPathNodeIterator if (!this.iterationStarted) { // Reset iteration to original position this.current = this.original.Clone(); this.iterationStarted = true; } if (this.current == null || !this.current.MoveNext()) { // Iteration complete this.current = null; return false; } return true; } public virtual void Reset() { this.iterationStarted = false; } } private struct DebuggerDisplayProxy { private XPathNodeIterator nodeIterator; public DebuggerDisplayProxy(XPathNodeIterator nodeIterator) { this.nodeIterator = nodeIterator; } public override string ToString() { // Position={CurrentPosition}, Current={Current == null ? null : (object) new XPathNavigator.DebuggerDisplayProxy(Current)} StringBuilder sb = new StringBuilder(); sb.Append("Position="); sb.Append(nodeIterator.CurrentPosition); sb.Append(", Current="); if (nodeIterator.Current == null) { sb.Append("null"); } else { sb.Append('{'); sb.Append(new XPathNavigator.DebuggerDisplayProxy(nodeIterator.Current).ToString()); sb.Append('}'); } return sb.ToString(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
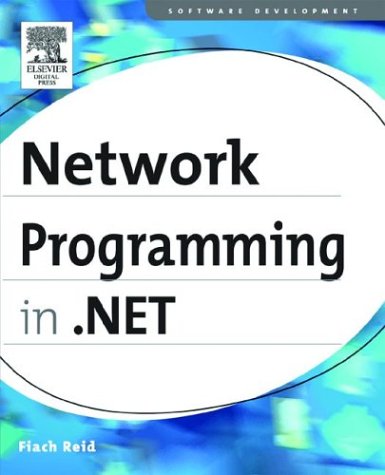
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CapacityStreamGeometryContext.cs
- control.ime.cs
- CodeEventReferenceExpression.cs
- TraceSource.cs
- StreamGeometry.cs
- DynamicILGenerator.cs
- FigureParaClient.cs
- XmlReturnWriter.cs
- XmlSchemaProviderAttribute.cs
- OptimalTextSource.cs
- RelationshipSet.cs
- AuthorizationPolicyTypeElement.cs
- DbUpdateCommandTree.cs
- _Events.cs
- DataGridViewDataErrorEventArgs.cs
- _SecureChannel.cs
- InstanceNotFoundException.cs
- MeasureItemEvent.cs
- List.cs
- PrimaryKeyTypeConverter.cs
- FilterException.cs
- EditorBrowsableAttribute.cs
- HtmlTableCellCollection.cs
- Latin1Encoding.cs
- Exceptions.cs
- SafeHandles.cs
- FormsAuthenticationEventArgs.cs
- DictionaryEntry.cs
- EntityDataSourceUtil.cs
- dsa.cs
- GorillaCodec.cs
- PageScaling.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- Point3D.cs
- Button.cs
- IriParsingElement.cs
- InstanceNotFoundException.cs
- Material.cs
- Errors.cs
- WindowsBrush.cs
- ToolStripGripRenderEventArgs.cs
- PriorityBinding.cs
- ControlBuilderAttribute.cs
- AnnotationResource.cs
- OperationContextScope.cs
- MetadataArtifactLoaderComposite.cs
- HttpCookiesSection.cs
- XPathScanner.cs
- WebBrowserUriTypeConverter.cs
- AsymmetricAlgorithm.cs
- DataGridViewSelectedRowCollection.cs
- LinearGradientBrush.cs
- SmiEventSink.cs
- DetailsViewPageEventArgs.cs
- ComPlusThreadInitializer.cs
- MultipartIdentifier.cs
- MultiSelector.cs
- RtfControls.cs
- DropShadowBitmapEffect.cs
- UnmanagedMemoryStream.cs
- SQLInt64Storage.cs
- WebPartConnectionsConfigureVerb.cs
- LinearGradientBrush.cs
- SystemColors.cs
- Misc.cs
- ApplicationServiceHelper.cs
- NativeMethods.cs
- DNS.cs
- XmlReflectionMember.cs
- PropertyPushdownHelper.cs
- EntityCommandExecutionException.cs
- SmtpTransport.cs
- XslTransform.cs
- CacheManager.cs
- ApplyImportsAction.cs
- OdbcEnvironment.cs
- XmlSchemaParticle.cs
- MD5Cng.cs
- TabPanel.cs
- FormsIdentity.cs
- HybridDictionary.cs
- Trustee.cs
- SqlConnectionString.cs
- CqlGenerator.cs
- ElementUtil.cs
- PrintController.cs
- LayoutDump.cs
- TableLayoutStyleCollection.cs
- PackageRelationshipSelector.cs
- ApplicationException.cs
- unitconverter.cs
- InputBinding.cs
- CacheDependency.cs
- UInt16Converter.cs
- DataGridAutomationPeer.cs
- InputMethodStateTypeInfo.cs
- PeerApplicationLaunchInfo.cs
- XmlNodeChangedEventArgs.cs
- UshortList2.cs
- PeerNameRecordCollection.cs