Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / SystemColors.cs / 1305600 / SystemColors.cs
using System; using System.Collections; using System.Windows.Media; using Microsoft.Win32; using MS.Win32; namespace System.Windows { ////// Contains properties that are queries into the system's various colors. /// public static class SystemColors { #region Colors ////// System color of the same name. /// public static Color ActiveBorderColor { get { return GetSystemColor(CacheSlot.ActiveBorder); } } ////// System color of the same name. /// public static Color ActiveCaptionColor { get { return GetSystemColor(CacheSlot.ActiveCaption); } } ////// System color of the same name. /// public static Color ActiveCaptionTextColor { get { return GetSystemColor(CacheSlot.ActiveCaptionText); } } ////// System color of the same name. /// public static Color AppWorkspaceColor { get { return GetSystemColor(CacheSlot.AppWorkspace); } } ////// System color of the same name. /// public static Color ControlColor { get { return GetSystemColor(CacheSlot.Control); } } ////// System color of the same name. /// public static Color ControlDarkColor { get { return GetSystemColor(CacheSlot.ControlDark); } } ////// System color of the same name. /// public static Color ControlDarkDarkColor { get { return GetSystemColor(CacheSlot.ControlDarkDark); } } ////// System color of the same name. /// public static Color ControlLightColor { get { return GetSystemColor(CacheSlot.ControlLight); } } ////// System color of the same name. /// public static Color ControlLightLightColor { get { return GetSystemColor(CacheSlot.ControlLightLight); } } ////// System color of the same name. /// public static Color ControlTextColor { get { return GetSystemColor(CacheSlot.ControlText); } } ////// System color of the same name. /// public static Color DesktopColor { get { return GetSystemColor(CacheSlot.Desktop); } } ////// System color of the same name. /// public static Color GradientActiveCaptionColor { get { return GetSystemColor(CacheSlot.GradientActiveCaption); } } ////// System color of the same name. /// public static Color GradientInactiveCaptionColor { get { return GetSystemColor(CacheSlot.GradientInactiveCaption); } } ////// System color of the same name. /// public static Color GrayTextColor { get { return GetSystemColor(CacheSlot.GrayText); } } ////// System color of the same name. /// public static Color HighlightColor { get { return GetSystemColor(CacheSlot.Highlight); } } ////// System color of the same name. /// public static Color HighlightTextColor { get { return GetSystemColor(CacheSlot.HighlightText); } } ////// System color of the same name. /// public static Color HotTrackColor { get { return GetSystemColor(CacheSlot.HotTrack); } } ////// System color of the same name. /// public static Color InactiveBorderColor { get { return GetSystemColor(CacheSlot.InactiveBorder); } } ////// System color of the same name. /// public static Color InactiveCaptionColor { get { return GetSystemColor(CacheSlot.InactiveCaption); } } ////// System color of the same name. /// public static Color InactiveCaptionTextColor { get { return GetSystemColor(CacheSlot.InactiveCaptionText); } } ////// System color of the same name. /// public static Color InfoColor { get { return GetSystemColor(CacheSlot.Info); } } ////// System color of the same name. /// public static Color InfoTextColor { get { return GetSystemColor(CacheSlot.InfoText); } } ////// System color of the same name. /// public static Color MenuColor { get { return GetSystemColor(CacheSlot.Menu); } } ////// System color of the same name. /// public static Color MenuBarColor { get { return GetSystemColor(CacheSlot.MenuBar); } } ////// System color of the same name. /// public static Color MenuHighlightColor { get { return GetSystemColor(CacheSlot.MenuHighlight); } } ////// System color of the same name. /// public static Color MenuTextColor { get { return GetSystemColor(CacheSlot.MenuText); } } ////// System color of the same name. /// public static Color ScrollBarColor { get { return GetSystemColor(CacheSlot.ScrollBar); } } ////// System color of the same name. /// public static Color WindowColor { get { return GetSystemColor(CacheSlot.Window); } } ////// System color of the same name. /// public static Color WindowFrameColor { get { return GetSystemColor(CacheSlot.WindowFrame); } } ////// System color of the same name. /// public static Color WindowTextColor { get { return GetSystemColor(CacheSlot.WindowText); } } #endregion [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static SystemResourceKey CreateInstance(SystemResourceKeyID KeyId) { return new SystemResourceKey(KeyId); } #region Color Keys ////// ActiveBorderColor System Resource Key /// public static ResourceKey ActiveBorderColorKey { get { if (_cacheActiveBorderColor == null) { _cacheActiveBorderColor = CreateInstance(SystemResourceKeyID.ActiveBorderColor); } return _cacheActiveBorderColor; } } ////// ActiveCaptionColor System Resource Key /// public static ResourceKey ActiveCaptionColorKey { get { if (_cacheActiveCaptionColor == null) { _cacheActiveCaptionColor = CreateInstance(SystemResourceKeyID.ActiveCaptionColor); } return _cacheActiveCaptionColor; } } ////// ActiveCaptionTextColor System Resource Key /// public static ResourceKey ActiveCaptionTextColorKey { get { if (_cacheActiveCaptionTextColor == null) { _cacheActiveCaptionTextColor = CreateInstance(SystemResourceKeyID.ActiveCaptionTextColor); } return _cacheActiveCaptionTextColor; } } ////// AppWorkspaceColor System Resource Key /// public static ResourceKey AppWorkspaceColorKey { get { if (_cacheAppWorkspaceColor == null) { _cacheAppWorkspaceColor = CreateInstance(SystemResourceKeyID.AppWorkspaceColor); } return _cacheAppWorkspaceColor; } } ////// ControlColor System Resource Key /// public static ResourceKey ControlColorKey { get { if (_cacheControlColor == null) { _cacheControlColor = CreateInstance(SystemResourceKeyID.ControlColor); } return _cacheControlColor; } } ////// ControlDarkColor System Resource Key /// public static ResourceKey ControlDarkColorKey { get { if (_cacheControlDarkColor == null) { _cacheControlDarkColor = CreateInstance(SystemResourceKeyID.ControlDarkColor); } return _cacheControlDarkColor; } } ////// ControlDarkDarkColor System Resource Key /// public static ResourceKey ControlDarkDarkColorKey { get { if (_cacheControlDarkDarkColor == null) { _cacheControlDarkDarkColor = CreateInstance(SystemResourceKeyID.ControlDarkDarkColor); } return _cacheControlDarkDarkColor; } } ////// ControlLightColor System Resource Key /// public static ResourceKey ControlLightColorKey { get { if (_cacheControlLightColor == null) { _cacheControlLightColor = CreateInstance(SystemResourceKeyID.ControlLightColor); } return _cacheControlLightColor; } } ////// ControlLightLightColor System Resource Key /// public static ResourceKey ControlLightLightColorKey { get { if (_cacheControlLightLightColor == null) { _cacheControlLightLightColor = CreateInstance(SystemResourceKeyID.ControlLightLightColor); } return _cacheControlLightLightColor; } } ////// ControlTextColor System Resource Key /// public static ResourceKey ControlTextColorKey { get { if (_cacheControlTextColor == null) { _cacheControlTextColor = CreateInstance(SystemResourceKeyID.ControlTextColor); } return _cacheControlTextColor; } } ////// DesktopColor System Resource Key /// public static ResourceKey DesktopColorKey { get { if (_cacheDesktopColor == null) { _cacheDesktopColor = CreateInstance(SystemResourceKeyID.DesktopColor); } return _cacheDesktopColor; } } ////// GradientActiveCaptionColor System Resource Key /// public static ResourceKey GradientActiveCaptionColorKey { get { if (_cacheGradientActiveCaptionColor == null) { _cacheGradientActiveCaptionColor = CreateInstance(SystemResourceKeyID.GradientActiveCaptionColor); } return _cacheGradientActiveCaptionColor; } } ////// GradientInactiveCaptionColor System Resource Key /// public static ResourceKey GradientInactiveCaptionColorKey { get { if (_cacheGradientInactiveCaptionColor == null) { _cacheGradientInactiveCaptionColor = CreateInstance(SystemResourceKeyID.GradientInactiveCaptionColor); } return _cacheGradientInactiveCaptionColor; } } ////// GrayTextColor System Resource Key /// public static ResourceKey GrayTextColorKey { get { if (_cacheGrayTextColor == null) { _cacheGrayTextColor = CreateInstance(SystemResourceKeyID.GrayTextColor); } return _cacheGrayTextColor; } } ////// HighlightColor System Resource Key /// public static ResourceKey HighlightColorKey { get { if (_cacheHighlightColor == null) { _cacheHighlightColor = CreateInstance(SystemResourceKeyID.HighlightColor); } return _cacheHighlightColor; } } ////// HighlightTextColor System Resource Key /// public static ResourceKey HighlightTextColorKey { get { if (_cacheHighlightTextColor == null) { _cacheHighlightTextColor = CreateInstance(SystemResourceKeyID.HighlightTextColor); } return _cacheHighlightTextColor; } } ////// HotTrackColor System Resource Key /// public static ResourceKey HotTrackColorKey { get { if (_cacheHotTrackColor == null) { _cacheHotTrackColor = CreateInstance(SystemResourceKeyID.HotTrackColor); } return _cacheHotTrackColor; } } ////// InactiveBorderColor System Resource Key /// public static ResourceKey InactiveBorderColorKey { get { if (_cacheInactiveBorderColor == null) { _cacheInactiveBorderColor = CreateInstance(SystemResourceKeyID.InactiveBorderColor); } return _cacheInactiveBorderColor; } } ////// InactiveCaptionColor System Resource Key /// public static ResourceKey InactiveCaptionColorKey { get { if (_cacheInactiveCaptionColor == null) { _cacheInactiveCaptionColor = CreateInstance(SystemResourceKeyID.InactiveCaptionColor); } return _cacheInactiveCaptionColor; } } ////// InactiveCaptionTextColor System Resource Key /// public static ResourceKey InactiveCaptionTextColorKey { get { if (_cacheInactiveCaptionTextColor == null) { _cacheInactiveCaptionTextColor = CreateInstance(SystemResourceKeyID.InactiveCaptionTextColor); } return _cacheInactiveCaptionTextColor; } } ////// InfoColor System Resource Key /// public static ResourceKey InfoColorKey { get { if (_cacheInfoColor == null) { _cacheInfoColor = CreateInstance(SystemResourceKeyID.InfoColor); } return _cacheInfoColor; } } ////// InfoTextColor System Resource Key /// public static ResourceKey InfoTextColorKey { get { if (_cacheInfoTextColor == null) { _cacheInfoTextColor = CreateInstance(SystemResourceKeyID.InfoTextColor); } return _cacheInfoTextColor; } } ////// MenuColor System Resource Key /// public static ResourceKey MenuColorKey { get { if (_cacheMenuColor == null) { _cacheMenuColor = CreateInstance(SystemResourceKeyID.MenuColor); } return _cacheMenuColor; } } ////// MenuBarColor System Resource Key /// public static ResourceKey MenuBarColorKey { get { if (_cacheMenuBarColor == null) { _cacheMenuBarColor = CreateInstance(SystemResourceKeyID.MenuBarColor); } return _cacheMenuBarColor; } } ////// MenuHighlightColor System Resource Key /// public static ResourceKey MenuHighlightColorKey { get { if (_cacheMenuHighlightColor == null) { _cacheMenuHighlightColor = CreateInstance(SystemResourceKeyID.MenuHighlightColor); } return _cacheMenuHighlightColor; } } ////// MenuTextColor System Resource Key /// public static ResourceKey MenuTextColorKey { get { if (_cacheMenuTextColor == null) { _cacheMenuTextColor = CreateInstance(SystemResourceKeyID.MenuTextColor); } return _cacheMenuTextColor; } } ////// ScrollBarColor System Resource Key /// public static ResourceKey ScrollBarColorKey { get { if (_cacheScrollBarColor == null) { _cacheScrollBarColor = CreateInstance(SystemResourceKeyID.ScrollBarColor); } return _cacheScrollBarColor; } } ////// WindowColor System Resource Key /// public static ResourceKey WindowColorKey { get { if (_cacheWindowColor == null) { _cacheWindowColor = CreateInstance(SystemResourceKeyID.WindowColor); } return _cacheWindowColor; } } ////// WindowFrameColor System Resource Key /// public static ResourceKey WindowFrameColorKey { get { if (_cacheWindowFrameColor == null) { _cacheWindowFrameColor = CreateInstance(SystemResourceKeyID.WindowFrameColor); } return _cacheWindowFrameColor; } } ////// WindowTextColor System Resource Key /// public static ResourceKey WindowTextColorKey { get { if (_cacheWindowTextColor == null) { _cacheWindowTextColor = CreateInstance(SystemResourceKeyID.WindowTextColor); } return _cacheWindowTextColor; } } #endregion #region Brushes ////// System color of the same name. /// public static SolidColorBrush ActiveBorderBrush { get { return MakeBrush(CacheSlot.ActiveBorder); } } ////// System color of the same name. /// public static SolidColorBrush ActiveCaptionBrush { get { return MakeBrush(CacheSlot.ActiveCaption); } } ////// System color of the same name. /// public static SolidColorBrush ActiveCaptionTextBrush { get { return MakeBrush(CacheSlot.ActiveCaptionText); } } ////// System color of the same name. /// public static SolidColorBrush AppWorkspaceBrush { get { return MakeBrush(CacheSlot.AppWorkspace); } } ////// System color of the same name. /// public static SolidColorBrush ControlBrush { get { return MakeBrush(CacheSlot.Control); } } ////// System color of the same name. /// public static SolidColorBrush ControlDarkBrush { get { return MakeBrush(CacheSlot.ControlDark); } } ////// System color of the same name. /// public static SolidColorBrush ControlDarkDarkBrush { get { return MakeBrush(CacheSlot.ControlDarkDark); } } ////// System color of the same name. /// public static SolidColorBrush ControlLightBrush { get { return MakeBrush(CacheSlot.ControlLight); } } ////// System color of the same name. /// public static SolidColorBrush ControlLightLightBrush { get { return MakeBrush(CacheSlot.ControlLightLight); } } ////// System color of the same name. /// public static SolidColorBrush ControlTextBrush { get { return MakeBrush(CacheSlot.ControlText); } } ////// System color of the same name. /// public static SolidColorBrush DesktopBrush { get { return MakeBrush(CacheSlot.Desktop); } } ////// System color of the same name. /// public static SolidColorBrush GradientActiveCaptionBrush { get { return MakeBrush(CacheSlot.GradientActiveCaption); } } ////// System color of the same name. /// public static SolidColorBrush GradientInactiveCaptionBrush { get { return MakeBrush(CacheSlot.GradientInactiveCaption); } } ////// System color of the same name. /// public static SolidColorBrush GrayTextBrush { get { return MakeBrush(CacheSlot.GrayText); } } ////// System color of the same name. /// public static SolidColorBrush HighlightBrush { get { return MakeBrush(CacheSlot.Highlight); } } ////// System color of the same name. /// public static SolidColorBrush HighlightTextBrush { get { return MakeBrush(CacheSlot.HighlightText); } } ////// System color of the same name. /// public static SolidColorBrush HotTrackBrush { get { return MakeBrush(CacheSlot.HotTrack); } } ////// System color of the same name. /// public static SolidColorBrush InactiveBorderBrush { get { return MakeBrush(CacheSlot.InactiveBorder); } } ////// System color of the same name. /// public static SolidColorBrush InactiveCaptionBrush { get { return MakeBrush(CacheSlot.InactiveCaption); } } ////// System color of the same name. /// public static SolidColorBrush InactiveCaptionTextBrush { get { return MakeBrush(CacheSlot.InactiveCaptionText); } } ////// System color of the same name. /// public static SolidColorBrush InfoBrush { get { return MakeBrush(CacheSlot.Info); } } ////// System color of the same name. /// public static SolidColorBrush InfoTextBrush { get { return MakeBrush(CacheSlot.InfoText); } } ////// System color of the same name. /// public static SolidColorBrush MenuBrush { get { return MakeBrush(CacheSlot.Menu); } } ////// System color of the same name. /// public static SolidColorBrush MenuBarBrush { get { return MakeBrush(CacheSlot.MenuBar); } } ////// System color of the same name. /// public static SolidColorBrush MenuHighlightBrush { get { return MakeBrush(CacheSlot.MenuHighlight); } } ////// System color of the same name. /// public static SolidColorBrush MenuTextBrush { get { return MakeBrush(CacheSlot.MenuText); } } ////// System color of the same name. /// public static SolidColorBrush ScrollBarBrush { get { return MakeBrush(CacheSlot.ScrollBar); } } ////// System color of the same name. /// public static SolidColorBrush WindowBrush { get { return MakeBrush(CacheSlot.Window); } } ////// System color of the same name. /// public static SolidColorBrush WindowFrameBrush { get { return MakeBrush(CacheSlot.WindowFrame); } } ////// System color of the same name. /// public static SolidColorBrush WindowTextBrush { get { return MakeBrush(CacheSlot.WindowText); } } #endregion #region Brush Keys ////// ActiveBorderBrush System Resource Key /// public static ResourceKey ActiveBorderBrushKey { get { if (_cacheActiveBorderBrush == null) { _cacheActiveBorderBrush = CreateInstance(SystemResourceKeyID.ActiveBorderBrush); } return _cacheActiveBorderBrush; } } ////// ActiveCaptionBrush System Resource Key /// public static ResourceKey ActiveCaptionBrushKey { get { if (_cacheActiveCaptionBrush == null) { _cacheActiveCaptionBrush = CreateInstance(SystemResourceKeyID.ActiveCaptionBrush); } return _cacheActiveCaptionBrush; } } ////// ActiveCaptionTextBrush System Resource Key /// public static ResourceKey ActiveCaptionTextBrushKey { get { if (_cacheActiveCaptionTextBrush == null) { _cacheActiveCaptionTextBrush = CreateInstance(SystemResourceKeyID.ActiveCaptionTextBrush); } return _cacheActiveCaptionTextBrush; } } ////// AppWorkspaceBrush System Resource Key /// public static ResourceKey AppWorkspaceBrushKey { get { if (_cacheAppWorkspaceBrush == null) { _cacheAppWorkspaceBrush = CreateInstance(SystemResourceKeyID.AppWorkspaceBrush); } return _cacheAppWorkspaceBrush; } } ////// ControlBrush System Resource Key /// public static ResourceKey ControlBrushKey { get { if (_cacheControlBrush == null) { _cacheControlBrush = CreateInstance(SystemResourceKeyID.ControlBrush); } return _cacheControlBrush; } } ////// ControlDarkBrush System Resource Key /// public static ResourceKey ControlDarkBrushKey { get { if (_cacheControlDarkBrush == null) { _cacheControlDarkBrush = CreateInstance(SystemResourceKeyID.ControlDarkBrush); } return _cacheControlDarkBrush; } } ////// ControlDarkDarkBrush System Resource Key /// public static ResourceKey ControlDarkDarkBrushKey { get { if (_cacheControlDarkDarkBrush == null) { _cacheControlDarkDarkBrush = CreateInstance(SystemResourceKeyID.ControlDarkDarkBrush); } return _cacheControlDarkDarkBrush; } } ////// ControlLightBrush System Resource Key /// public static ResourceKey ControlLightBrushKey { get { if (_cacheControlLightBrush == null) { _cacheControlLightBrush = CreateInstance(SystemResourceKeyID.ControlLightBrush); } return _cacheControlLightBrush; } } ////// ControlLightLightBrush System Resource Key /// public static ResourceKey ControlLightLightBrushKey { get { if (_cacheControlLightLightBrush == null) { _cacheControlLightLightBrush = CreateInstance(SystemResourceKeyID.ControlLightLightBrush); } return _cacheControlLightLightBrush; } } ////// ControlTextBrush System Resource Key /// public static ResourceKey ControlTextBrushKey { get { if (_cacheControlTextBrush == null) { _cacheControlTextBrush = CreateInstance(SystemResourceKeyID.ControlTextBrush); } return _cacheControlTextBrush; } } ////// DesktopBrush System Resource Key /// public static ResourceKey DesktopBrushKey { get { if (_cacheDesktopBrush == null) { _cacheDesktopBrush = CreateInstance(SystemResourceKeyID.DesktopBrush); } return _cacheDesktopBrush; } } ////// GradientActiveCaptionBrush System Resource Key /// public static ResourceKey GradientActiveCaptionBrushKey { get { if (_cacheGradientActiveCaptionBrush == null) { _cacheGradientActiveCaptionBrush = CreateInstance(SystemResourceKeyID.GradientActiveCaptionBrush); } return _cacheGradientActiveCaptionBrush; } } ////// GradientInactiveCaptionBrush System Resource Key /// public static ResourceKey GradientInactiveCaptionBrushKey { get { if (_cacheGradientInactiveCaptionBrush == null) { _cacheGradientInactiveCaptionBrush = CreateInstance(SystemResourceKeyID.GradientInactiveCaptionBrush); } return _cacheGradientInactiveCaptionBrush; } } ////// GrayTextBrush System Resource Key /// public static ResourceKey GrayTextBrushKey { get { if (_cacheGrayTextBrush == null) { _cacheGrayTextBrush = CreateInstance(SystemResourceKeyID.GrayTextBrush); } return _cacheGrayTextBrush; } } ////// HighlightBrush System Resource Key /// public static ResourceKey HighlightBrushKey { get { if (_cacheHighlightBrush == null) { _cacheHighlightBrush = CreateInstance(SystemResourceKeyID.HighlightBrush); } return _cacheHighlightBrush; } } ////// HighlightTextBrush System Resource Key /// public static ResourceKey HighlightTextBrushKey { get { if (_cacheHighlightTextBrush == null) { _cacheHighlightTextBrush = CreateInstance(SystemResourceKeyID.HighlightTextBrush); } return _cacheHighlightTextBrush; } } ////// HotTrackBrush System Resource Key /// public static ResourceKey HotTrackBrushKey { get { if (_cacheHotTrackBrush == null) { _cacheHotTrackBrush = CreateInstance(SystemResourceKeyID.HotTrackBrush); } return _cacheHotTrackBrush; } } ////// InactiveBorderBrush System Resource Key /// public static ResourceKey InactiveBorderBrushKey { get { if (_cacheInactiveBorderBrush == null) { _cacheInactiveBorderBrush = CreateInstance(SystemResourceKeyID.InactiveBorderBrush); } return _cacheInactiveBorderBrush; } } ////// InactiveCaptionBrush System Resource Key /// public static ResourceKey InactiveCaptionBrushKey { get { if (_cacheInactiveCaptionBrush == null) { _cacheInactiveCaptionBrush = CreateInstance(SystemResourceKeyID.InactiveCaptionBrush); } return _cacheInactiveCaptionBrush; } } ////// InactiveCaptionTextBrush System Resource Key /// public static ResourceKey InactiveCaptionTextBrushKey { get { if (_cacheInactiveCaptionTextBrush == null) { _cacheInactiveCaptionTextBrush = CreateInstance(SystemResourceKeyID.InactiveCaptionTextBrush); } return _cacheInactiveCaptionTextBrush; } } ////// InfoBrush System Resource Key /// public static ResourceKey InfoBrushKey { get { if (_cacheInfoBrush == null) { _cacheInfoBrush = CreateInstance(SystemResourceKeyID.InfoBrush); } return _cacheInfoBrush; } } ////// InfoTextBrush System Resource Key /// public static ResourceKey InfoTextBrushKey { get { if (_cacheInfoTextBrush == null) { _cacheInfoTextBrush = CreateInstance(SystemResourceKeyID.InfoTextBrush); } return _cacheInfoTextBrush; } } ////// MenuBrush System Resource Key /// public static ResourceKey MenuBrushKey { get { if (_cacheMenuBrush == null) { _cacheMenuBrush = CreateInstance(SystemResourceKeyID.MenuBrush); } return _cacheMenuBrush; } } ////// MenuBarBrush System Resource Key /// public static ResourceKey MenuBarBrushKey { get { if (_cacheMenuBarBrush == null) { _cacheMenuBarBrush = CreateInstance(SystemResourceKeyID.MenuBarBrush); } return _cacheMenuBarBrush; } } ////// MenuHighlightBrush System Resource Key /// public static ResourceKey MenuHighlightBrushKey { get { if (_cacheMenuHighlightBrush == null) { _cacheMenuHighlightBrush = CreateInstance(SystemResourceKeyID.MenuHighlightBrush); } return _cacheMenuHighlightBrush; } } ////// MenuTextBrush System Resource Key /// public static ResourceKey MenuTextBrushKey { get { if (_cacheMenuTextBrush == null) { _cacheMenuTextBrush = CreateInstance(SystemResourceKeyID.MenuTextBrush); } return _cacheMenuTextBrush; } } ////// ScrollBarBrush System Resource Key /// public static ResourceKey ScrollBarBrushKey { get { if (_cacheScrollBarBrush == null) { _cacheScrollBarBrush = CreateInstance(SystemResourceKeyID.ScrollBarBrush); } return _cacheScrollBarBrush; } } ////// WindowBrush System Resource Key /// public static ResourceKey WindowBrushKey { get { if (_cacheWindowBrush == null) { _cacheWindowBrush = CreateInstance(SystemResourceKeyID.WindowBrush); } return _cacheWindowBrush; } } ////// WindowFrameBrush System Resource Key /// public static ResourceKey WindowFrameBrushKey { get { if (_cacheWindowFrameBrush == null) { _cacheWindowFrameBrush = CreateInstance(SystemResourceKeyID.WindowFrameBrush); } return _cacheWindowFrameBrush; } } ////// WindowTextBrush System Resource Key /// public static ResourceKey WindowTextBrushKey { get { if (_cacheWindowTextBrush == null) { _cacheWindowTextBrush = CreateInstance(SystemResourceKeyID.WindowTextBrush); } return _cacheWindowTextBrush; } } #endregion #region Implementation internal static bool InvalidateCache() { bool color = SystemResources.ClearBitArray(_colorCacheValid); bool brush = SystemResources.ClearBitArray(_brushCacheValid); return color || brush; } // Shift count and bit mask for A, R, G, B components private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// The color slot. ///The system color. private static Color GetSystemColor(CacheSlot slot) { Color color; lock (_colorCacheValid) { if (!_colorCacheValid[(int)slot]) { uint argb; int sysColor = SafeNativeMethods.GetSysColor(SlotToFlag(slot)); argb = (uint)FromWin32Value(sysColor); color = Color.FromArgb((byte)((argb & 0xff000000) >>24), (byte)((argb & 0x00ff0000) >>16), (byte)((argb & 0x0000ff00) >>8), (byte)(argb & 0x000000ff)); _colorCache[(int)slot] = color; _colorCacheValid[(int)slot] = true; } else { color = _colorCache[(int)slot]; } } return color; } private static SolidColorBrush MakeBrush(CacheSlot slot) { SolidColorBrush brush; lock (_brushCacheValid) { if (!_brushCacheValid[(int)slot]) { brush = new SolidColorBrush(GetSystemColor(slot)); brush.Freeze(); _brushCache[(int)slot] = brush; _brushCacheValid[(int)slot] = true; } else { brush = _brushCache[(int)slot]; } } return brush; } private static int SlotToFlag(CacheSlot slot) { // FxCop: Hashtable would be overkill, using switch instead switch (slot) { case CacheSlot.ActiveBorder: return (int)NativeMethods.Win32SystemColors.ActiveBorder; case CacheSlot.ActiveCaption: return (int)NativeMethods.Win32SystemColors.ActiveCaption; case CacheSlot.ActiveCaptionText: return (int)NativeMethods.Win32SystemColors.ActiveCaptionText; case CacheSlot.AppWorkspace: return (int)NativeMethods.Win32SystemColors.AppWorkspace; case CacheSlot.Control: return (int)NativeMethods.Win32SystemColors.Control; case CacheSlot.ControlDark: return (int)NativeMethods.Win32SystemColors.ControlDark; case CacheSlot.ControlDarkDark: return (int)NativeMethods.Win32SystemColors.ControlDarkDark; case CacheSlot.ControlLight: return (int)NativeMethods.Win32SystemColors.ControlLight; case CacheSlot.ControlLightLight: return (int)NativeMethods.Win32SystemColors.ControlLightLight; case CacheSlot.ControlText: return (int)NativeMethods.Win32SystemColors.ControlText; case CacheSlot.Desktop: return (int)NativeMethods.Win32SystemColors.Desktop; case CacheSlot.GradientActiveCaption: return (int)NativeMethods.Win32SystemColors.GradientActiveCaption; case CacheSlot.GradientInactiveCaption: return (int)NativeMethods.Win32SystemColors.GradientInactiveCaption; case CacheSlot.GrayText: return (int)NativeMethods.Win32SystemColors.GrayText; case CacheSlot.Highlight: return (int)NativeMethods.Win32SystemColors.Highlight; case CacheSlot.HighlightText: return (int)NativeMethods.Win32SystemColors.HighlightText; case CacheSlot.HotTrack: return (int)NativeMethods.Win32SystemColors.HotTrack; case CacheSlot.InactiveBorder: return (int)NativeMethods.Win32SystemColors.InactiveBorder; case CacheSlot.InactiveCaption: return (int)NativeMethods.Win32SystemColors.InactiveCaption; case CacheSlot.InactiveCaptionText: return (int)NativeMethods.Win32SystemColors.InactiveCaptionText; case CacheSlot.Info: return (int)NativeMethods.Win32SystemColors.Info; case CacheSlot.InfoText: return (int)NativeMethods.Win32SystemColors.InfoText; case CacheSlot.Menu: return (int)NativeMethods.Win32SystemColors.Menu; case CacheSlot.MenuBar: return (int)NativeMethods.Win32SystemColors.MenuBar; case CacheSlot.MenuHighlight: return (int)NativeMethods.Win32SystemColors.MenuHighlight; case CacheSlot.MenuText: return (int)NativeMethods.Win32SystemColors.MenuText; case CacheSlot.ScrollBar: return (int)NativeMethods.Win32SystemColors.ScrollBar; case CacheSlot.Window: return (int)NativeMethods.Win32SystemColors.Window; case CacheSlot.WindowFrame: return (int)NativeMethods.Win32SystemColors.WindowFrame; case CacheSlot.WindowText: return (int)NativeMethods.Win32SystemColors.WindowText; } return 0; } private enum CacheSlot : int { ActiveBorder, ActiveCaption, ActiveCaptionText, AppWorkspace, Control, ControlDark, ControlDarkDark, ControlLight, ControlLightLight, ControlText, Desktop, GradientActiveCaption, GradientInactiveCaption, GrayText, Highlight, HighlightText, HotTrack, InactiveBorder, InactiveCaption, InactiveCaptionText, Info, InfoText, Menu, MenuBar, MenuHighlight, MenuText, ScrollBar, Window, WindowFrame, WindowText, NumSlots } private static BitArray _colorCacheValid = new BitArray((int)CacheSlot.NumSlots); private static Color[] _colorCache = new Color[(int)CacheSlot.NumSlots]; private static BitArray _brushCacheValid = new BitArray((int)CacheSlot.NumSlots); private static SolidColorBrush[] _brushCache = new SolidColorBrush[(int)CacheSlot.NumSlots]; private static SystemResourceKey _cacheActiveBorderBrush; private static SystemResourceKey _cacheActiveCaptionBrush; private static SystemResourceKey _cacheActiveCaptionTextBrush; private static SystemResourceKey _cacheAppWorkspaceBrush; private static SystemResourceKey _cacheControlBrush; private static SystemResourceKey _cacheControlDarkBrush; private static SystemResourceKey _cacheControlDarkDarkBrush; private static SystemResourceKey _cacheControlLightBrush; private static SystemResourceKey _cacheControlLightLightBrush; private static SystemResourceKey _cacheControlTextBrush; private static SystemResourceKey _cacheDesktopBrush; private static SystemResourceKey _cacheGradientActiveCaptionBrush; private static SystemResourceKey _cacheGradientInactiveCaptionBrush; private static SystemResourceKey _cacheGrayTextBrush; private static SystemResourceKey _cacheHighlightBrush; private static SystemResourceKey _cacheHighlightTextBrush; private static SystemResourceKey _cacheHotTrackBrush; private static SystemResourceKey _cacheInactiveBorderBrush; private static SystemResourceKey _cacheInactiveCaptionBrush; private static SystemResourceKey _cacheInactiveCaptionTextBrush; private static SystemResourceKey _cacheInfoBrush; private static SystemResourceKey _cacheInfoTextBrush; private static SystemResourceKey _cacheMenuBrush; private static SystemResourceKey _cacheMenuBarBrush; private static SystemResourceKey _cacheMenuHighlightBrush; private static SystemResourceKey _cacheMenuTextBrush; private static SystemResourceKey _cacheScrollBarBrush; private static SystemResourceKey _cacheWindowBrush; private static SystemResourceKey _cacheWindowFrameBrush; private static SystemResourceKey _cacheWindowTextBrush; private static SystemResourceKey _cacheActiveBorderColor; private static SystemResourceKey _cacheActiveCaptionColor; private static SystemResourceKey _cacheActiveCaptionTextColor; private static SystemResourceKey _cacheAppWorkspaceColor; private static SystemResourceKey _cacheControlColor; private static SystemResourceKey _cacheControlDarkColor; private static SystemResourceKey _cacheControlDarkDarkColor; private static SystemResourceKey _cacheControlLightColor; private static SystemResourceKey _cacheControlLightLightColor; private static SystemResourceKey _cacheControlTextColor; private static SystemResourceKey _cacheDesktopColor; private static SystemResourceKey _cacheGradientActiveCaptionColor; private static SystemResourceKey _cacheGradientInactiveCaptionColor; private static SystemResourceKey _cacheGrayTextColor; private static SystemResourceKey _cacheHighlightColor; private static SystemResourceKey _cacheHighlightTextColor; private static SystemResourceKey _cacheHotTrackColor; private static SystemResourceKey _cacheInactiveBorderColor; private static SystemResourceKey _cacheInactiveCaptionColor; private static SystemResourceKey _cacheInactiveCaptionTextColor; private static SystemResourceKey _cacheInfoColor; private static SystemResourceKey _cacheInfoTextColor; private static SystemResourceKey _cacheMenuColor; private static SystemResourceKey _cacheMenuBarColor; private static SystemResourceKey _cacheMenuHighlightColor; private static SystemResourceKey _cacheMenuTextColor; private static SystemResourceKey _cacheScrollBarColor; private static SystemResourceKey _cacheWindowColor; private static SystemResourceKey _cacheWindowFrameColor; private static SystemResourceKey _cacheWindowTextColor; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
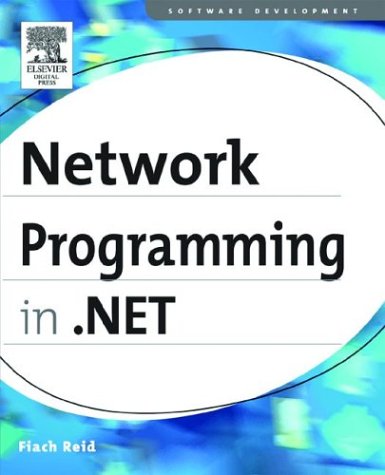
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlUserDefinedAggregateAttribute.cs
- KeyValuePair.cs
- Pkcs7Recipient.cs
- Imaging.cs
- ChildTable.cs
- KnownColorTable.cs
- BmpBitmapDecoder.cs
- NumericUpDown.cs
- DataServiceRequestOfT.cs
- DiscoveryReferences.cs
- Vector3DAnimationUsingKeyFrames.cs
- DataGridViewCellPaintingEventArgs.cs
- TokenBasedSetEnumerator.cs
- SystemParameters.cs
- TransactionContextManager.cs
- SqlVersion.cs
- TextBlockAutomationPeer.cs
- HttpHandlerActionCollection.cs
- ValueProviderWrapper.cs
- DataService.cs
- TypeConverterHelper.cs
- VectorCollection.cs
- Comparer.cs
- PrintPreviewGraphics.cs
- Lasso.cs
- RewritingProcessor.cs
- NonBatchDirectoryCompiler.cs
- Boolean.cs
- CodeAttributeArgument.cs
- Application.cs
- ToolStripPanelRow.cs
- PackUriHelper.cs
- GlyphCache.cs
- SQLUtility.cs
- MergeFilterQuery.cs
- MergeLocalizationDirectives.cs
- FormsAuthenticationCredentials.cs
- EventListenerClientSide.cs
- ActivityFunc.cs
- TypedTableBase.cs
- HtmlMeta.cs
- ControlsConfig.cs
- MessageBox.cs
- MessageSmuggler.cs
- DoubleAnimationUsingKeyFrames.cs
- KnownTypesHelper.cs
- ILGen.cs
- CorrelationService.cs
- WindowsRebar.cs
- IndexerNameAttribute.cs
- Thumb.cs
- XmlDataSourceDesigner.cs
- FrameworkTemplate.cs
- WeakRefEnumerator.cs
- DataMember.cs
- Point4D.cs
- ProxyAttribute.cs
- WindowsImpersonationContext.cs
- SafeThreadHandle.cs
- StreamingContext.cs
- AggregateNode.cs
- TraceContext.cs
- QuaternionRotation3D.cs
- XmlBaseWriter.cs
- Encoder.cs
- ToolStripDropTargetManager.cs
- StyleBamlRecordReader.cs
- SingleStorage.cs
- EmissiveMaterial.cs
- ScriptModule.cs
- FontDriver.cs
- DispatcherEventArgs.cs
- ComboBoxRenderer.cs
- _CommandStream.cs
- CustomPopupPlacement.cs
- XPathNavigator.cs
- KernelTypeValidation.cs
- EventNotify.cs
- ElementUtil.cs
- EditorZoneBase.cs
- BamlReader.cs
- IRCollection.cs
- ObjectContextServiceProvider.cs
- TypedElement.cs
- CoTaskMemHandle.cs
- Publisher.cs
- CheckBoxRenderer.cs
- TransactionTraceIdentifier.cs
- WsatConfiguration.cs
- ContractCodeDomInfo.cs
- ListViewItem.cs
- httpstaticobjectscollection.cs
- Container.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- Pair.cs
- WaitForChangedResult.cs
- UnsettableComboBox.cs
- Util.cs
- PasswordTextContainer.cs
- JsonFormatReaderGenerator.cs