Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / TransactionTraceIdentifier.cs / 1305376 / TransactionTraceIdentifier.cs
using System; namespace System.Transactions { ////// This identifier is used in tracing to distiguish instances /// of transaction objects. This identifier is only unique within /// a given AppDomain. /// internal struct TransactionTraceIdentifier { public static readonly TransactionTraceIdentifier Empty = new TransactionTraceIdentifier(); public TransactionTraceIdentifier( string transactionIdentifier, int cloneIdentifier ) { this.transactionIdentifier = transactionIdentifier; this.cloneIdentifier = cloneIdentifier; } private string transactionIdentifier; ////// The string representation of the transaction identifier. /// public string TransactionIdentifier { get { return this.transactionIdentifier; } } private int cloneIdentifier; ////// An integer value that allows different clones of the same /// transaction to be distiguished in the tracing. /// public int CloneIdentifier { get { return this.cloneIdentifier; } } public override int GetHashCode() { return base.GetHashCode(); // Don't have anything better to do. } public override bool Equals ( object objectToCompare ) { if ( ! ( objectToCompare is TransactionTraceIdentifier ) ) { return false; } TransactionTraceIdentifier id = (TransactionTraceIdentifier) objectToCompare; if ( ( id.TransactionIdentifier != this.TransactionIdentifier ) || ( id.CloneIdentifier != this.CloneIdentifier ) ) { return false; } return true; } public static bool operator==( TransactionTraceIdentifier id1, TransactionTraceIdentifier id2 ) { return id1.Equals( id2 ); } public static bool operator!=( TransactionTraceIdentifier id1, TransactionTraceIdentifier id2 ) { return !id1.Equals( id2 ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Transactions { ////// This identifier is used in tracing to distiguish instances /// of transaction objects. This identifier is only unique within /// a given AppDomain. /// internal struct TransactionTraceIdentifier { public static readonly TransactionTraceIdentifier Empty = new TransactionTraceIdentifier(); public TransactionTraceIdentifier( string transactionIdentifier, int cloneIdentifier ) { this.transactionIdentifier = transactionIdentifier; this.cloneIdentifier = cloneIdentifier; } private string transactionIdentifier; ////// The string representation of the transaction identifier. /// public string TransactionIdentifier { get { return this.transactionIdentifier; } } private int cloneIdentifier; ////// An integer value that allows different clones of the same /// transaction to be distiguished in the tracing. /// public int CloneIdentifier { get { return this.cloneIdentifier; } } public override int GetHashCode() { return base.GetHashCode(); // Don't have anything better to do. } public override bool Equals ( object objectToCompare ) { if ( ! ( objectToCompare is TransactionTraceIdentifier ) ) { return false; } TransactionTraceIdentifier id = (TransactionTraceIdentifier) objectToCompare; if ( ( id.TransactionIdentifier != this.TransactionIdentifier ) || ( id.CloneIdentifier != this.CloneIdentifier ) ) { return false; } return true; } public static bool operator==( TransactionTraceIdentifier id1, TransactionTraceIdentifier id2 ) { return id1.Equals( id2 ); } public static bool operator!=( TransactionTraceIdentifier id1, TransactionTraceIdentifier id2 ) { return !id1.Equals( id2 ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
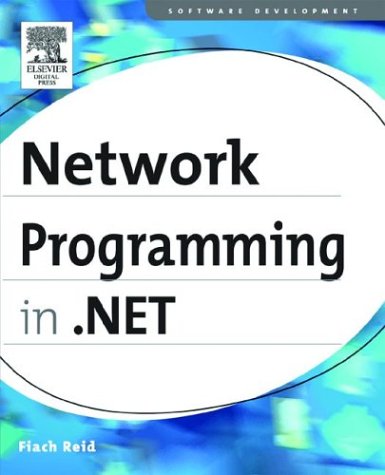
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionModeReader.cs
- TextParagraphProperties.cs
- ContextMenu.cs
- AnnotationHighlightLayer.cs
- Delegate.cs
- ExceptionDetail.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DataExpression.cs
- HttpChannelHelper.cs
- BitmapEffectDrawingContextState.cs
- ByteStorage.cs
- MeasureData.cs
- DragStartedEventArgs.cs
- IsolatedStorageFile.cs
- UnsafeNativeMethodsCLR.cs
- WebPartDeleteVerb.cs
- InProcStateClientManager.cs
- DataGridViewCellValueEventArgs.cs
- HtmlContainerControl.cs
- StateMachineSubscriptionManager.cs
- SignalGate.cs
- XmlEntity.cs
- Pair.cs
- FixedLineResult.cs
- RetrieveVirtualItemEventArgs.cs
- XsltArgumentList.cs
- EncryptedKey.cs
- CodeIdentifier.cs
- ItemsChangedEventArgs.cs
- CompoundFileIOPermission.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- StrongNameMembershipCondition.cs
- Environment.cs
- TextContainer.cs
- LocatorManager.cs
- DesignerActionPanel.cs
- UiaCoreApi.cs
- XmlMtomReader.cs
- SettingsAttributeDictionary.cs
- AutomationPattern.cs
- PropertyRef.cs
- SymbolPair.cs
- DurableErrorHandler.cs
- cookiecollection.cs
- dbenumerator.cs
- WrappedIUnknown.cs
- Deserializer.cs
- PrintPreviewControl.cs
- LinkConverter.cs
- DictionaryTraceRecord.cs
- ColumnMap.cs
- SkewTransform.cs
- DataViewManager.cs
- SiblingIterators.cs
- DependencyPropertyAttribute.cs
- DelegateArgument.cs
- EntitySqlQueryBuilder.cs
- Region.cs
- AttributeUsageAttribute.cs
- RoutedEventValueSerializer.cs
- OleDbConnectionInternal.cs
- HttpDebugHandler.cs
- MarkupObject.cs
- ToolStripDropDownMenu.cs
- __Error.cs
- SelectedCellsChangedEventArgs.cs
- cookie.cs
- RepeaterItemCollection.cs
- HttpSessionStateBase.cs
- BrowserCapabilitiesFactory35.cs
- PhysicalAddress.cs
- UpWmlPageAdapter.cs
- XamlReader.cs
- ScriptControl.cs
- LicenseException.cs
- OdbcError.cs
- ValidatingReaderNodeData.cs
- Cloud.cs
- DBConcurrencyException.cs
- ApplicationServiceManager.cs
- Currency.cs
- StorageAssociationSetMapping.cs
- TextWriterEngine.cs
- HtmlInputButton.cs
- FixedNode.cs
- IgnoreFileBuildProvider.cs
- HttpDictionary.cs
- NameValueConfigurationCollection.cs
- SelectedDatesCollection.cs
- SmiMetaDataProperty.cs
- SchemaImporterExtension.cs
- TextWriterEngine.cs
- StyleReferenceConverter.cs
- TableLayoutColumnStyleCollection.cs
- DataKeyArray.cs
- ElementsClipboardData.cs
- HttpResponseHeader.cs
- XmlNodeList.cs
- DefaultPrintController.cs
- OleDbConnectionFactory.cs